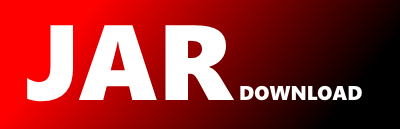
io.vertx.groovy.core.http.HttpServerResponse.groovy Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.groovy.core.http;
import groovy.transform.CompileStatic
import io.vertx.lang.groovy.InternalHelper
import io.vertx.core.json.JsonObject
import io.vertx.groovy.core.buffer.Buffer
import io.vertx.groovy.core.streams.WriteStream
import io.vertx.groovy.core.MultiMap
import io.vertx.core.AsyncResult
import io.vertx.core.Handler
/**
* Represents a server-side HTTP response.
*
* An instance of this is created and associated to every instance of
* {@link io.vertx.groovy.core.http.HttpServerRequest} that.
*
* It allows the developer to control the HTTP response that is sent back to the
* client for a particular HTTP request.
*
* It contains methods that allow HTTP headers and trailers to be set, and for a body to be written out to the response.
*
* It also allows files to be streamed by the kernel directly from disk to the
* outgoing HTTP connection, bypassing user space altogether (where supported by
* the underlying operating system). This is a very efficient way of
* serving files from the server since buffers do not have to be read one by one
* from the file and written to the outgoing socket.
*
* It implements {@link io.vertx.groovy.core.streams.WriteStream} so it can be used with
* {@link io.vertx.groovy.core.streams.Pump} to pump data with flow control.
*/
@CompileStatic
public class HttpServerResponse implements WriteStream {
private final def io.vertx.core.http.HttpServerResponse delegate;
public HttpServerResponse(Object delegate) {
this.delegate = (io.vertx.core.http.HttpServerResponse) delegate;
}
public Object getDelegate() {
return delegate;
}
/**
* This will return true
if there are more bytes in the write queue than the value set using {@link io.vertx.groovy.core.http.HttpServerResponse#setWriteQueueMaxSize}
* @return true if write queue is full
*/
public boolean writeQueueFull() {
def ret = ((io.vertx.core.streams.WriteStream) this.delegate).writeQueueFull();
return ret;
}
public HttpServerResponse exceptionHandler(Handler handler) {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.http.HttpServerResponse) this.delegate).exceptionHandler(handler);
return this;
}
public HttpServerResponse write(Buffer data) {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.http.HttpServerResponse) this.delegate).write((io.vertx.core.buffer.Buffer)data.getDelegate());
return this;
}
public HttpServerResponse setWriteQueueMaxSize(int maxSize) {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.http.HttpServerResponse) this.delegate).setWriteQueueMaxSize(maxSize);
return this;
}
public HttpServerResponse drainHandler(Handler handler) {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.http.HttpServerResponse) this.delegate).drainHandler(handler);
return this;
}
/**
* @return the HTTP status code of the response. The default is 200
representing OK
.
* @return
*/
public int getStatusCode() {
def ret = this.delegate.getStatusCode();
return ret;
}
/**
* Set the status code. If the status message hasn't been explicitly set, a default status message corresponding
* to the code will be looked-up and used.
* @param statusCode
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse setStatusCode(int statusCode) {
this.delegate.setStatusCode(statusCode);
return this;
}
/**
* @return the HTTP status message of the response. If this is not specified a default value will be used depending on what
* {@link io.vertx.groovy.core.http.HttpServerResponse#setStatusCode} has been set to.
* @return
*/
public String getStatusMessage() {
def ret = this.delegate.getStatusMessage();
return ret;
}
/**
* Set the status message
* @param statusMessage
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse setStatusMessage(String statusMessage) {
this.delegate.setStatusMessage(statusMessage);
return this;
}
/**
* If chunked
is true
, this response will use HTTP chunked encoding, and each call to write to the body
* will correspond to a new HTTP chunk sent on the wire.
*
* If chunked encoding is used the HTTP header Transfer-Encoding
with a value of Chunked
will be
* automatically inserted in the response.
*
* If chunked
is false
, this response will not use HTTP chunked encoding, and therefore the total size
* of any data that is written in the respone body must be set in the Content-Length
header before any
* data is written out.
*
* An HTTP chunked response is typically used when you do not know the total size of the request body up front.
* @param chunked
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse setChunked(boolean chunked) {
this.delegate.setChunked(chunked);
return this;
}
/**
* @return is the response chunked?
* @return
*/
public boolean isChunked() {
def ret = this.delegate.isChunked();
return ret;
}
/**
* @return The HTTP headers
* @return
*/
public MultiMap headers() {
if (cached_0 != null) {
return cached_0;
}
def ret= InternalHelper.safeCreate(this.delegate.headers(), io.vertx.groovy.core.MultiMap.class);
cached_0 = ret;
return ret;
}
/**
* Put an HTTP header
* @param name the header name
* @param value the header value.
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse putHeader(String name, String value) {
this.delegate.putHeader(name, value);
return this;
}
/**
* @return The HTTP trailers
* @return
*/
public MultiMap trailers() {
if (cached_1 != null) {
return cached_1;
}
def ret= InternalHelper.safeCreate(this.delegate.trailers(), io.vertx.groovy.core.MultiMap.class);
cached_1 = ret;
return ret;
}
/**
* Put an HTTP trailer
* @param name the trailer name
* @param value the trailer value
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse putTrailer(String name, String value) {
this.delegate.putTrailer(name, value);
return this;
}
/**
* Set a close handler for the response. This will be called if the underlying connection closes before the response
* is complete.
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse closeHandler(Handler handler) {
this.delegate.closeHandler(handler);
return this;
}
/**
* Write a {@link java.lang.String} to the response body, encoded using the encoding enc
.
* @param chunk the string to write
* @param enc the encoding to use
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse write(String chunk, String enc) {
this.delegate.write(chunk, enc);
return this;
}
/**
* Write a {@link java.lang.String} to the response body, encoded in UTF-8.
* @param chunk the string to write
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse write(String chunk) {
this.delegate.write(chunk);
return this;
}
/**
* Used to write an interim 100 Continue response to signify that the client should send the rest of the request.
* Must only be used if the request contains an "Expect:100-Continue" header
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse writeContinue() {
this.delegate.writeContinue();
return this;
}
/**
* Same as {@link io.vertx.groovy.core.http.HttpServerResponse#end} but writes a String in UTF-8 encoding before ending the response.
* @param chunk the string to write before ending the response
*/
public void end(String chunk) {
this.delegate.end(chunk);
}
/**
* Same as {@link io.vertx.groovy.core.http.HttpServerResponse#end} but writes a String with the specified encoding before ending the response.
* @param chunk the string to write before ending the response
* @param enc the encoding to use
*/
public void end(String chunk, String enc) {
this.delegate.end(chunk, enc);
}
/**
* Same as {@link io.vertx.groovy.core.http.HttpServerResponse#end} but writes some data to the response body before ending. If the response is not chunked and
* no other data has been written then the @code{Content-Length} header will be automatically set.
* @param chunk the buffer to write before ending the response
*/
public void end(Buffer chunk) {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.http.HttpServerResponse) this.delegate).end((io.vertx.core.buffer.Buffer)chunk.getDelegate());
}
/**
* Ends the response. If no data has been written to the response body,
* the actual response won't get written until this method gets called.
*
* Once the response has ended, it cannot be used any more.
*/
public void end() {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.http.HttpServerResponse) this.delegate).end();
}
/**
* Same as {@link io.vertx.groovy.core.http.HttpServerResponse#sendFile} using offset @code{0} which means starting from the beginning of the file.
* @param filename path to the file to serve
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse sendFile(String filename) {
this.delegate.sendFile(filename);
return this;
}
/**
* Same as {@link io.vertx.groovy.core.http.HttpServerResponse#sendFile} using length @code{Long.MAX_VALUE} which means until the end of the
* file.
* @param filename path to the file to serve
* @param offset offset to start serving from
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse sendFile(String filename, long offset) {
this.delegate.sendFile(filename, offset);
return this;
}
/**
* Ask the OS to stream a file as specified by filename
directly
* from disk to the outgoing connection, bypassing userspace altogether
* (where supported by the underlying operating system.
* This is a very efficient way to serve files.
* The actual serve is asynchronous and may not complete until some time after this method has returned.
* @param filename path to the file to serve
* @param offset offset to start serving from
* @param length length to serve to
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse sendFile(String filename, long offset, long length) {
this.delegate.sendFile(filename, offset, length);
return this;
}
/**
* Like {@link io.vertx.groovy.core.http.HttpServerResponse#sendFile} but providing a handler which will be notified once the file has been completely
* written to the wire.
* @param filename path to the file to serve
* @param resultHandler handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse sendFile(String filename, Handler> resultHandler) {
this.delegate.sendFile(filename, resultHandler);
return this;
}
/**
* Like {@link io.vertx.groovy.core.http.HttpServerResponse#sendFile} but providing a handler which will be notified once the file has been completely
* written to the wire.
* @param filename path to the file to serve
* @param offset the offset to serve from
* @param resultHandler handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse sendFile(String filename, long offset, Handler> resultHandler) {
this.delegate.sendFile(filename, offset, resultHandler);
return this;
}
/**
* Like {@link io.vertx.groovy.core.http.HttpServerResponse#sendFile} but providing a handler which will be notified once the file has been
* completely written to the wire.
* @param filename path to the file to serve
* @param offset the offset to serve from
* @param length the length to serve to
* @param resultHandler handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse sendFile(String filename, long offset, long length, Handler> resultHandler) {
this.delegate.sendFile(filename, offset, length, resultHandler);
return this;
}
/**
* Close the underlying TCP connection corresponding to the request.
*/
public void close() {
this.delegate.close();
}
/**
* @return has the response already ended?
* @return
*/
public boolean ended() {
def ret = this.delegate.ended();
return ret;
}
/**
* @return has the underlying TCP connection corresponding to the request already been closed?
* @return
*/
public boolean closed() {
def ret = this.delegate.closed();
return ret;
}
/**
* @return have the headers for the response already been written?
* @return
*/
public boolean headWritten() {
def ret = this.delegate.headWritten();
return ret;
}
/**
* Provide a handler that will be called just before the headers are written to the wire.
* This provides a hook allowing you to add any more headers or do any more operations before this occurs.
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse headersEndHandler(Handler handler) {
this.delegate.headersEndHandler(handler);
return this;
}
/**
* Provide a handler that will be called just before the last part of the body is written to the wire
* and the response is ended.
* This provides a hook allowing you to do any more operations before this occurs.
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
public HttpServerResponse bodyEndHandler(Handler handler) {
this.delegate.bodyEndHandler(handler);
return this;
}
/**
* @return the total number of bytes written for the body of the response.
* @return
*/
public long bytesWritten() {
def ret = this.delegate.bytesWritten();
return ret;
}
private MultiMap cached_0;
private MultiMap cached_1;
}