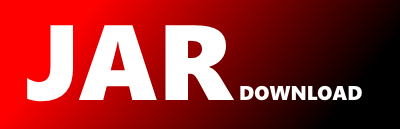
io.vertx.kotlin.ext.sql.SQLConnection.kt Maven / Gradle / Ivy
/*
* Copyright 2019 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.kotlin.ext.sql
import io.vertx.core.json.JsonArray
import io.vertx.ext.sql.ResultSet
import io.vertx.ext.sql.SQLConnection
import io.vertx.ext.sql.SQLRowStream
import io.vertx.ext.sql.TransactionIsolation
import io.vertx.ext.sql.UpdateResult
import io.vertx.kotlin.coroutines.awaitResult
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.querySingle]
*
* @param sql the statement to execute
* @return [JsonArray?]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.querySingleAwait(sql: String): JsonArray? {
return awaitResult {
this.querySingle(sql, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.querySingleWithParams]
*
* @param sql the statement to execute
* @param arguments the arguments
* @return [JsonArray?]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.querySingleWithParamsAwait(sql: String, arguments: JsonArray): JsonArray? {
return awaitResult {
this.querySingleWithParams(sql, arguments, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.setAutoCommit]
*
* @param autoCommit the autoCommit flag, true by default.
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.setAutoCommitAwait(autoCommit: Boolean): Unit {
return awaitResult {
this.setAutoCommit(autoCommit, io.vertx.core.Handler { ar -> it.handle(ar.mapEmpty()) })
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.execute]
*
* @param sql the SQL to execute. For example CREATE TABLE IF EXISTS table ...
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.executeAwait(sql: String): Unit {
return awaitResult {
this.execute(sql, io.vertx.core.Handler { ar -> it.handle(ar.mapEmpty()) })
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.query]
*
* @param sql the SQL to execute. For example SELECT * FROM table ...
.
* @return [ResultSet]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.queryAwait(sql: String): ResultSet {
return awaitResult {
this.query(sql, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.queryStream]
*
* @param sql the SQL to execute. For example SELECT * FROM table ...
.
* @return [SQLRowStream]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.queryStreamAwait(sql: String): SQLRowStream {
return awaitResult {
this.queryStream(sql, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.queryWithParams]
*
* @param sql the SQL to execute. For example SELECT * FROM table ...
.
* @param params these are the parameters to fill the statement.
* @return [ResultSet]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.queryWithParamsAwait(sql: String, params: JsonArray): ResultSet {
return awaitResult {
this.queryWithParams(sql, params, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.queryStreamWithParams]
*
* @param sql the SQL to execute. For example SELECT * FROM table ...
.
* @param params these are the parameters to fill the statement.
* @return [SQLRowStream]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.queryStreamWithParamsAwait(sql: String, params: JsonArray): SQLRowStream {
return awaitResult {
this.queryStreamWithParams(sql, params, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.update]
*
* @param sql the SQL to execute. For example INSERT INTO table ...
* @return [UpdateResult]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.updateAwait(sql: String): UpdateResult {
return awaitResult {
this.update(sql, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.updateWithParams]
*
* @param sql the SQL to execute. For example INSERT INTO table ...
* @param params these are the parameters to fill the statement.
* @return [UpdateResult]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.updateWithParamsAwait(sql: String, params: JsonArray): UpdateResult {
return awaitResult {
this.updateWithParams(sql, params, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.call]
*
* @param sql the SQL to execute. For example {call getEmpName}
.
* @return [ResultSet]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.callAwait(sql: String): ResultSet {
return awaitResult {
this.call(sql, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.callWithParams]
*
* @param sql the SQL to execute. For example {call getEmpName (?, ?)}
.
* @param params these are the parameters to fill the statement.
* @param outputs these are the outputs to fill the statement.
* @return [ResultSet]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.callWithParamsAwait(sql: String, params: JsonArray, outputs: JsonArray): ResultSet {
return awaitResult {
this.callWithParams(sql, params, outputs, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.close]
*
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.closeAwait(): Unit {
return awaitResult {
this.close(io.vertx.core.Handler { ar -> it.handle(ar.mapEmpty()) })
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.commit]
*
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.commitAwait(): Unit {
return awaitResult {
this.commit(io.vertx.core.Handler { ar -> it.handle(ar.mapEmpty()) })
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.rollback]
*
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.rollbackAwait(): Unit {
return awaitResult {
this.rollback(io.vertx.core.Handler { ar -> it.handle(ar.mapEmpty()) })
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.batch]
*
* @param sqlStatements sql statement
* @return [List]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.batchAwait(sqlStatements: List): List {
return awaitResult {
this.batch(sqlStatements, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.batchWithParams]
*
* @param sqlStatement sql statement
* @param args the prepared statement arguments
* @return [List]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.batchWithParamsAwait(sqlStatement: String, args: List): List {
return awaitResult {
this.batchWithParams(sqlStatement, args, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.batchCallableWithParams]
*
* @param sqlStatement sql statement
* @param inArgs the callable statement input arguments
* @param outArgs the callable statement output arguments
* @return [List]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.batchCallableWithParamsAwait(sqlStatement: String, inArgs: List, outArgs: List): List {
return awaitResult {
this.batchCallableWithParams(sqlStatement, inArgs, outArgs, it)
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.setTransactionIsolation]
*
* @param isolation the level of isolation
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.setTransactionIsolationAwait(isolation: TransactionIsolation): Unit {
return awaitResult {
this.setTransactionIsolation(isolation, io.vertx.core.Handler { ar -> it.handle(ar.mapEmpty()) })
}
}
/**
* Suspending version of method [io.vertx.ext.sql.SQLConnection.getTransactionIsolation]
*
* @return [TransactionIsolation]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.sql.SQLConnection] using Vert.x codegen.
*/
suspend fun SQLConnection.getTransactionIsolationAwait(): TransactionIsolation {
return awaitResult {
this.getTransactionIsolation(it)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy