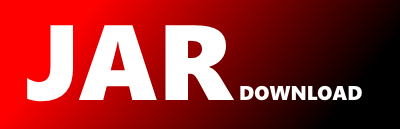
io.vertx.kotlin.core.net.PemKeyCertOptions.kt Maven / Gradle / Ivy
/*
* Copyright 2019 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.kotlin.core.net
import io.vertx.core.net.PemKeyCertOptions
/**
* A function providing a DSL for building [io.vertx.core.net.PemKeyCertOptions] objects.
*
* Key store options configuring a list of private key and its certificate based on
* Privacy-enhanced Electronic Email (PEM) files.
*
*
* A key file must contain a non encrypted private key in PKCS8 format wrapped in a PEM
* block, for example:
*
*
*
* -----BEGIN PRIVATE KEY-----
* MIIEvgIBADANBgkqhkiG9w0BAQEFAASCBKgwggSkAgEAAoIBAQDV6zPk5WqLwS0a
* ...
* K5xBhtm1AhdnZjx5KfW3BecE
* -----END PRIVATE KEY-----
*
*
* Or contain a non encrypted private key in PKCS1 format wrapped in a PEM
* block, for example:
*
*
*
* -----BEGIN RSA PRIVATE KEY-----
* MIIEowIBAAKCAQEAlO4gbHeFb/fmbUF/tOJfNPJumJUEqgzAzx8MBXv9Acyw9IRa
* ...
* zJ14Yd+t2fsLYVs2H0gxaA4DW6neCzgY3eKpSU0EBHUCFSXp/1+/
* -----END RSA PRIVATE KEY-----
*
*
* A certificate file must contain an X.509 certificate wrapped in a PEM block, for example:
*
*
*
* -----BEGIN CERTIFICATE-----
* MIIDezCCAmOgAwIBAgIEZOI/3TANBgkqhkiG9w0BAQsFADBuMRAwDgYDVQQGEwdV
* ...
* +tmLSvYS39O2nqIzzAUfztkYnUlZmB0l/mKkVqbGJA==
* -----END CERTIFICATE-----
*
*
* Keys and certificates can either be loaded by Vert.x from the filesystem:
*
*
* HttpServerOptions options = new HttpServerOptions();
* options.setPemKeyCertOptions(new PemKeyCertOptions().setKeyPath("/mykey.pem").setCertPath("/mycert.pem"));
*
*
* Or directly provided as a buffer:
*
*
* Buffer key = vertx.fileSystem().readFileBlocking("/mykey.pem");
* Buffer cert = vertx.fileSystem().readFileBlocking("/mycert.pem");
* options.setPemKeyCertOptions(new PemKeyCertOptions().setKeyValue(key).setCertValue(cert));
*
*
* Several key/certificate pairs can be used:
*
*
* HttpServerOptions options = new HttpServerOptions();
* options.setPemKeyCertOptions(new PemKeyCertOptions()
* .addKeyPath("/mykey1.pem").addCertPath("/mycert1.pem")
* .addKeyPath("/mykey2.pem").addCertPath("/mycert2.pem"));
*
*
* @param certPath Set the path of the first certificate, replacing the previous certificates paths
* @param certPaths Set all the paths to the certificates files
* @param certValue Set the first certificate as a buffer, replacing the previous certificates buffers
* @param certValues Set all the certificates as a list of buffer
* @param keyPath Set the path of the first key file, replacing the keys paths
* @param keyPaths Set all the paths to the keys files
* @param keyValue Set the first key a a buffer, replacing the previous keys buffers
* @param keyValues Set all the keys as a list of buffer
*
*
* NOTE: This function has been automatically generated from the [io.vertx.core.net.PemKeyCertOptions original] using Vert.x codegen.
*/
fun pemKeyCertOptionsOf(
certPath: String? = null,
certPaths: Iterable? = null,
certValue: io.vertx.core.buffer.Buffer? = null,
certValues: Iterable? = null,
keyPath: String? = null,
keyPaths: Iterable? = null,
keyValue: io.vertx.core.buffer.Buffer? = null,
keyValues: Iterable? = null): PemKeyCertOptions = io.vertx.core.net.PemKeyCertOptions().apply {
if (certPath != null) {
this.setCertPath(certPath)
}
if (certPaths != null) {
this.setCertPaths(certPaths.toList())
}
if (certValue != null) {
this.setCertValue(certValue)
}
if (certValues != null) {
this.setCertValues(certValues.toList())
}
if (keyPath != null) {
this.setKeyPath(keyPath)
}
if (keyPaths != null) {
this.setKeyPaths(keyPaths.toList())
}
if (keyValue != null) {
this.setKeyValue(keyValue)
}
if (keyValues != null) {
this.setKeyValues(keyValues.toList())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy