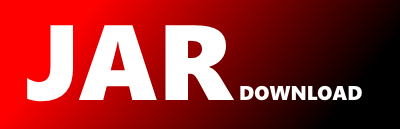
io.vertx.kotlin.ext.web.client.HttpRequest.kt Maven / Gradle / Ivy
/*
* Copyright 2019 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.kotlin.ext.web.client
import io.vertx.core.MultiMap
import io.vertx.core.buffer.Buffer
import io.vertx.core.json.JsonObject
import io.vertx.core.streams.ReadStream
import io.vertx.ext.web.client.HttpRequest
import io.vertx.ext.web.client.HttpResponse
import io.vertx.ext.web.multipart.MultipartForm
import io.vertx.kotlin.coroutines.awaitResult
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendStream]
*
* @param body the body
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendStream returning a future and chain with await()", replaceWith = ReplaceWith("sendStream(body).await()"))
suspend fun HttpRequest.sendStreamAwait(body: ReadStream): HttpResponse {
return awaitResult {
this.sendStream(body, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendBuffer]
*
* @param body the body
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendBuffer returning a future and chain with await()", replaceWith = ReplaceWith("sendBuffer(body).await()"))
suspend fun HttpRequest.sendBufferAwait(body: Buffer): HttpResponse {
return awaitResult {
this.sendBuffer(body, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendJsonObject]
*
* @param body the body
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendJsonObject returning a future and chain with await()", replaceWith = ReplaceWith("sendJsonObject(body).await()"))
suspend fun HttpRequest.sendJsonObjectAwait(body: JsonObject): HttpResponse {
return awaitResult {
this.sendJsonObject(body, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendJson]
*
* @param body the body
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendJson returning a future and chain with await()", replaceWith = ReplaceWith("sendJson(body).await()"))
suspend fun HttpRequest.sendJsonAwait(body: Any?): HttpResponse {
return awaitResult {
this.sendJson(body, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendForm]
*
* @param body the body
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendForm returning a future and chain with await()", replaceWith = ReplaceWith("sendForm(body).await()"))
suspend fun HttpRequest.sendFormAwait(body: MultiMap): HttpResponse {
return awaitResult {
this.sendForm(body, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendForm]
*
* @param body the body
* @param charset
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendForm returning a future and chain with await()", replaceWith = ReplaceWith("sendForm(body, charset).await()"))
suspend fun HttpRequest.sendFormAwait(body: MultiMap, charset: String): HttpResponse {
return awaitResult {
this.sendForm(body, charset, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.sendMultipartForm]
*
* @param body the body
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use sendMultipartForm returning a future and chain with await()", replaceWith = ReplaceWith("sendMultipartForm(body).await()"))
suspend fun HttpRequest.sendMultipartFormAwait(body: MultipartForm): HttpResponse {
return awaitResult {
this.sendMultipartForm(body, it)
}
}
/**
* Suspending version of method [io.vertx.ext.web.client.HttpRequest.send]
*
* @return [HttpResponse]
*
* NOTE: This function has been automatically generated from [io.vertx.ext.web.client.HttpRequest] using Vert.x codegen.
*/
@Deprecated(message = "Instead use send returning a future and chain with await()", replaceWith = ReplaceWith("send().await()"))
suspend fun HttpRequest.sendAwait(): HttpResponse {
return awaitResult {
this.send(it)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy