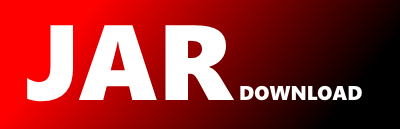
io.vertx.kotlin.redis.client.RedisOptions.kt Maven / Gradle / Ivy
/*
* Copyright 2019 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.kotlin.redis.client
import io.vertx.redis.client.RedisOptions
import io.vertx.core.net.NetClientOptions
import io.vertx.core.tracing.TracingPolicy
import io.vertx.redis.client.ProtocolVersion
import io.vertx.redis.client.RedisClientType
import io.vertx.redis.client.RedisClusterTransactions
import io.vertx.redis.client.RedisReplicas
import io.vertx.redis.client.RedisRole
import io.vertx.redis.client.RedisTopology
/**
* A function providing a DSL for building [io.vertx.redis.client.RedisOptions] objects.
*
* Redis Client Configuration options.
*
* @param type Set the desired client type to be created.
* @param netClientOptions Set the net client options to be used while connecting to the Redis server. Use this to tune your connection.
* @param topology Set how the should be obtained. By default, the topology is automatically. This is only meaningful in case of a Redis client. In case of a and Redis client, topology is currently always discovered automatically and the topology mode is ignored.
* @param endpoints Set the endpoints to use while connecting to the Redis server. Only the cluster mode will consider more than 1 element. If more are provided, they are not considered by the client when in single server mode.
* @param maxWaitingHandlers The client will always work on pipeline mode, this means that messages can start queueing. You can control how much backlog you're willing to accept. This methods sets how much handlers is the client willing to queue.
* @param masterName Set the name of the master set. This is only meaningful in case of a Redis client and is ignored otherwise.
* @param role Set the client role; that is, to which kind of node should the connection be established. This is only meaningful in case of a Redis client and is ignored otherwise.
* @param useReplicas Set whether to use replica nodes for read only queries. This is only meaningful in case of a and Redis client and is ignored otherwise.
* @param clusterTransactions Set how Redis transactions are handled in cluster mode.
* @param maxNestedArrays Tune how much nested arrays are allowed on a Redis response. This affects the parser performance.
* @param tracingPolicy Set the tracing policy for the client behavior when Vert.x has tracing enabled.
* @param poolCleanerInterval Set how often the connection pool will be cleaned. Cleaning the connection pool means scanning for unused and invalid connections and if any are found, they are forcibly closed and evicted from the pool. A connection is marked invalid if it enters a exception or fatal state. It is marked unused if it is unused for longer than the .
The value is in milliseconds. By default, the cleaning interval is 30 seconds. The value of -1 means connection pool cleaning is disabled.
* @param maxPoolSize Set the maximum size of the connection pool.
By default, the maximum pool size is 6.
When working with cluster or sentinel, this value should be at least the total number of cluster member (or number of sentinels + 1).
* @param maxPoolWaiting Set the maximum number of requests waiting for a connection from the pool.
By default, the maximum number of waiting requests size is 24.
* @param poolRecycleTimeout Set how long a connection can stay unused before it is recycled during connection pool .
The value is in milliseconds. By default, the recycle timeout is 3 minutes. The value of -1 means connection recycling is disabled.
* @param user Set the default username for Redis connections.
* @param password Set the default password for Redis connections.
* @param protocolNegotiation Should the client perform RESP
protocol negotiation during the connection acquire. By default, this is true
, but there are situations when using broken servers it may be useful to skip this and always fallback to RESP2
without using the HELLO
command.
* @param preferredProtocolVersion Sets the preferred protocol version to be used during protocol negotiation. When not set, defaults to RESP 3. When protocol negotiation is disabled, this setting has no effect.
* @param poolName Set the connection pool name to be used for metrics reporting. The default name is a random UUID.
* @param metricsName Set the metrics name identifying the reported metrics, useful for grouping metrics with the same name.
* @param hashSlotCacheTTL Sets the TTL of the hash slot cache. The TTL is expressed in milliseconds. Defaults to 1000 millis (1 second).
This is only meaningful in case of a Redis client and is ignored otherwise.
* @param autoFailover Returns whether automatic failover is enabled. This only makes sense for sentinel clients with role of and is ignored otherwise. If enabled, the sentinel client will additionally create a connection to one sentinel node and watch for failover events. When new master is elected, all connections to the old master are automatically closed and new connections to the new master are created. Note that these new connections will not have the same event handlers (, and ), will not be in the same streaming mode (, and ), and will not watch the same subscriptions (SUBSCRIBE
, PSUBSCRIBE
, etc.) as the old ones. In other words, automatic failover makes sense for connections executing regular commands, but not for connections used to subscribe to Redis pub/sub channels.
Note that there is a brief period of time between the old master failing and the new master being elected when the existing connections will temporarily fail all operations. After the new master is elected, the connections will automatically fail over and start working again.
*
*
* NOTE: This function has been automatically generated from the [io.vertx.redis.client.RedisOptions original] using Vert.x codegen.
*/
fun redisOptionsOf(
type: RedisClientType? = null,
netClientOptions: io.vertx.core.net.NetClientOptions? = null,
topology: RedisTopology? = null,
endpoints: Iterable? = null,
maxWaitingHandlers: Int? = null,
masterName: String? = null,
role: RedisRole? = null,
useReplicas: RedisReplicas? = null,
clusterTransactions: RedisClusterTransactions? = null,
maxNestedArrays: Int? = null,
tracingPolicy: TracingPolicy? = null,
poolCleanerInterval: Int? = null,
maxPoolSize: Int? = null,
maxPoolWaiting: Int? = null,
poolRecycleTimeout: Int? = null,
user: String? = null,
password: String? = null,
protocolNegotiation: Boolean? = null,
preferredProtocolVersion: ProtocolVersion? = null,
poolName: String? = null,
metricsName: String? = null,
hashSlotCacheTTL: Long? = null,
autoFailover: Boolean? = null): RedisOptions = io.vertx.redis.client.RedisOptions().apply {
if (type != null) {
this.setType(type)
}
if (netClientOptions != null) {
this.setNetClientOptions(netClientOptions)
}
if (topology != null) {
this.setTopology(topology)
}
if (endpoints != null) {
this.setEndpoints(endpoints.toList())
}
if (maxWaitingHandlers != null) {
this.setMaxWaitingHandlers(maxWaitingHandlers)
}
if (masterName != null) {
this.setMasterName(masterName)
}
if (role != null) {
this.setRole(role)
}
if (useReplicas != null) {
this.setUseReplicas(useReplicas)
}
if (clusterTransactions != null) {
this.setClusterTransactions(clusterTransactions)
}
if (maxNestedArrays != null) {
this.setMaxNestedArrays(maxNestedArrays)
}
if (tracingPolicy != null) {
this.setTracingPolicy(tracingPolicy)
}
if (poolCleanerInterval != null) {
this.setPoolCleanerInterval(poolCleanerInterval)
}
if (maxPoolSize != null) {
this.setMaxPoolSize(maxPoolSize)
}
if (maxPoolWaiting != null) {
this.setMaxPoolWaiting(maxPoolWaiting)
}
if (poolRecycleTimeout != null) {
this.setPoolRecycleTimeout(poolRecycleTimeout)
}
if (user != null) {
this.setUser(user)
}
if (password != null) {
this.setPassword(password)
}
if (protocolNegotiation != null) {
this.setProtocolNegotiation(protocolNegotiation)
}
if (preferredProtocolVersion != null) {
this.setPreferredProtocolVersion(preferredProtocolVersion)
}
if (poolName != null) {
this.setPoolName(poolName)
}
if (metricsName != null) {
this.setMetricsName(metricsName)
}
if (hashSlotCacheTTL != null) {
this.setHashSlotCacheTTL(hashSlotCacheTTL)
}
if (autoFailover != null) {
this.setAutoFailover(autoFailover)
}
}