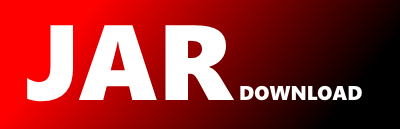
io.vertx.rxjava.ext.mongo.MongoClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.mongo;
import java.util.Map;
import rx.Observable;
import rx.Single;
import io.vertx.ext.mongo.MongoClientDeleteResult;
import io.vertx.ext.mongo.WriteOption;
import io.vertx.ext.mongo.BulkOperation;
import io.vertx.rxjava.core.Vertx;
import io.vertx.ext.mongo.MongoClientBulkWriteResult;
import io.vertx.core.json.JsonArray;
import java.util.List;
import io.vertx.ext.mongo.IndexOptions;
import io.vertx.ext.mongo.BulkWriteOptions;
import io.vertx.ext.mongo.FindOptions;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.ext.mongo.MongoClientUpdateResult;
import io.vertx.ext.mongo.UpdateOptions;
/**
* A Vert.x service used to interact with MongoDB server instances.
*
* Some of the operations might change _id field of passed document.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.mongo.MongoClient original} non RX-ified interface using Vert.x codegen.
*/
@io.vertx.lang.rxjava.RxGen(io.vertx.ext.mongo.MongoClient.class)
public class MongoClient {
public static final io.vertx.lang.rxjava.TypeArg __TYPE_ARG = new io.vertx.lang.rxjava.TypeArg<>(
obj -> new MongoClient((io.vertx.ext.mongo.MongoClient) obj),
MongoClient::getDelegate
);
private final io.vertx.ext.mongo.MongoClient delegate;
public MongoClient(io.vertx.ext.mongo.MongoClient delegate) {
this.delegate = delegate;
}
public io.vertx.ext.mongo.MongoClient getDelegate() {
return delegate;
}
/**
* Create a Mongo client which maintains its own data source.
* @param vertx the Vert.x instance
* @param config the configuration
* @return the client
*/
public static MongoClient createNonShared(Vertx vertx, JsonObject config) {
MongoClient ret = MongoClient.newInstance(io.vertx.ext.mongo.MongoClient.createNonShared(vertx.getDelegate(), config));
return ret;
}
/**
* Create a Mongo client which shares its data source with any other Mongo clients created with the same
* data source name
* @param vertx the Vert.x instance
* @param config the configuration
* @param dataSourceName the data source name
* @return the client
*/
public static MongoClient createShared(Vertx vertx, JsonObject config, String dataSourceName) {
MongoClient ret = MongoClient.newInstance(io.vertx.ext.mongo.MongoClient.createShared(vertx.getDelegate(), config, dataSourceName));
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.mongo.MongoClient#createShared} but with the default data source name
* @param vertx the Vert.x instance
* @param config the configuration
* @return the client
*/
public static MongoClient createShared(Vertx vertx, JsonObject config) {
MongoClient ret = MongoClient.newInstance(io.vertx.ext.mongo.MongoClient.createShared(vertx.getDelegate(), config));
return ret;
}
/**
* Save a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param resultHandler result handler will be provided with the id if document didn't already have one
* @return
*/
public MongoClient save(String collection, JsonObject document, Handler> resultHandler) {
delegate.save(collection, document, resultHandler);
return this;
}
/**
* Save a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
* @deprecated use {@link #rxSave} instead
*/
@Deprecated()
public Observable saveObservable(String collection, JsonObject document) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
save(collection, document, resultHandler.toHandler());
return resultHandler;
}
/**
* Save a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
*/
public Single rxSave(String collection, JsonObject document) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
save(collection, document, fut);
}));
}
/**
* Save a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @param resultHandler result handler will be provided with the id if document didn't already have one
* @return
*/
public MongoClient saveWithOptions(String collection, JsonObject document, WriteOption writeOption, Handler> resultHandler) {
delegate.saveWithOptions(collection, document, writeOption, resultHandler);
return this;
}
/**
* Save a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
* @deprecated use {@link #rxSaveWithOptions} instead
*/
@Deprecated()
public Observable saveWithOptionsObservable(String collection, JsonObject document, WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
saveWithOptions(collection, document, writeOption, resultHandler.toHandler());
return resultHandler;
}
/**
* Save a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
*/
public Single rxSaveWithOptions(String collection, JsonObject document, WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
saveWithOptions(collection, document, writeOption, fut);
}));
}
/**
* Insert a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param resultHandler result handler will be provided with the id if document didn't already have one
* @return
*/
public MongoClient insert(String collection, JsonObject document, Handler> resultHandler) {
delegate.insert(collection, document, resultHandler);
return this;
}
/**
* Insert a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
* @deprecated use {@link #rxInsert} instead
*/
@Deprecated()
public Observable insertObservable(String collection, JsonObject document) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
insert(collection, document, resultHandler.toHandler());
return resultHandler;
}
/**
* Insert a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
*/
public Single rxInsert(String collection, JsonObject document) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
insert(collection, document, fut);
}));
}
/**
* Insert a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @param resultHandler result handler will be provided with the id if document didn't already have one
* @return
*/
public MongoClient insertWithOptions(String collection, JsonObject document, WriteOption writeOption, Handler> resultHandler) {
delegate.insertWithOptions(collection, document, writeOption, resultHandler);
return this;
}
/**
* Insert a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
* @deprecated use {@link #rxInsertWithOptions} instead
*/
@Deprecated()
public Observable insertWithOptionsObservable(String collection, JsonObject document, WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
insertWithOptions(collection, document, writeOption, resultHandler.toHandler());
return resultHandler;
}
/**
* Insert a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
*/
public Single rxInsertWithOptions(String collection, JsonObject document, WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
insertWithOptions(collection, document, writeOption, fut);
}));
}
/**
* Update matching documents in the specified collection
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param resultHandler will be called when complete
* @return
*/
public MongoClient update(String collection, JsonObject query, JsonObject update, Handler> resultHandler) {
delegate.update(collection, query, update, resultHandler);
return this;
}
/**
* Update matching documents in the specified collection
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
* @deprecated use {@link #rxUpdate} instead
*/
@Deprecated()
public Observable updateObservable(String collection, JsonObject query, JsonObject update) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
update(collection, query, update, resultHandler.toHandler());
return resultHandler;
}
/**
* Update matching documents in the specified collection
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
*/
public Single rxUpdate(String collection, JsonObject query, JsonObject update) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
update(collection, query, update, fut);
}));
}
/**
* Update matching documents in the specified collection and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param resultHandler will be called when complete
* @return
*/
public MongoClient updateCollection(String collection, JsonObject query, JsonObject update, Handler> resultHandler) {
delegate.updateCollection(collection, query, update, resultHandler);
return this;
}
/**
* Update matching documents in the specified collection and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
* @deprecated use {@link #rxUpdateCollection} instead
*/
@Deprecated()
public Observable updateCollectionObservable(String collection, JsonObject query, JsonObject update) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
updateCollection(collection, query, update, resultHandler.toHandler());
return resultHandler;
}
/**
* Update matching documents in the specified collection and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
*/
public Single rxUpdateCollection(String collection, JsonObject query, JsonObject update) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
updateCollection(collection, query, update, fut);
}));
}
/**
* Update matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @param resultHandler will be called when complete
* @return
*/
public MongoClient updateWithOptions(String collection, JsonObject query, JsonObject update, UpdateOptions options, Handler> resultHandler) {
delegate.updateWithOptions(collection, query, update, options, resultHandler);
return this;
}
/**
* Update matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @return
* @deprecated use {@link #rxUpdateWithOptions} instead
*/
@Deprecated()
public Observable updateWithOptionsObservable(String collection, JsonObject query, JsonObject update, UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
updateWithOptions(collection, query, update, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Update matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @return
*/
public Single rxUpdateWithOptions(String collection, JsonObject query, JsonObject update, UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
updateWithOptions(collection, query, update, options, fut);
}));
}
/**
* Update matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @param resultHandler will be called when complete
* @return
*/
public MongoClient updateCollectionWithOptions(String collection, JsonObject query, JsonObject update, UpdateOptions options, Handler> resultHandler) {
delegate.updateCollectionWithOptions(collection, query, update, options, resultHandler);
return this;
}
/**
* Update matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @return
* @deprecated use {@link #rxUpdateCollectionWithOptions} instead
*/
@Deprecated()
public Observable updateCollectionWithOptionsObservable(String collection, JsonObject query, JsonObject update, UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
updateCollectionWithOptions(collection, query, update, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Update matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @return
*/
public Single rxUpdateCollectionWithOptions(String collection, JsonObject query, JsonObject update, UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
updateCollectionWithOptions(collection, query, update, options, fut);
}));
}
/**
* Replace matching documents in the specified collection
*
* This operation might change _id field of replace parameter
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param resultHandler will be called when complete
* @return
*/
public MongoClient replace(String collection, JsonObject query, JsonObject replace, Handler> resultHandler) {
delegate.replace(collection, query, replace, resultHandler);
return this;
}
/**
* Replace matching documents in the specified collection
*
* This operation might change _id field of replace parameter
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @return
* @deprecated use {@link #rxReplace} instead
*/
@Deprecated()
public Observable replaceObservable(String collection, JsonObject query, JsonObject replace) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replace(collection, query, replace, resultHandler.toHandler());
return resultHandler;
}
/**
* Replace matching documents in the specified collection
*
* This operation might change _id field of replace parameter
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @return
*/
public Single rxReplace(String collection, JsonObject query, JsonObject replace) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replace(collection, query, replace, fut);
}));
}
/**
* Replace matching documents in the specified collection and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param resultHandler will be called when complete
* @return
*/
public MongoClient replaceDocuments(String collection, JsonObject query, JsonObject replace, Handler> resultHandler) {
delegate.replaceDocuments(collection, query, replace, resultHandler);
return this;
}
/**
* Replace matching documents in the specified collection and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @return
* @deprecated use {@link #rxReplaceDocuments} instead
*/
@Deprecated()
public Observable replaceDocumentsObservable(String collection, JsonObject query, JsonObject replace) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replaceDocuments(collection, query, replace, resultHandler.toHandler());
return resultHandler;
}
/**
* Replace matching documents in the specified collection and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @return
*/
public Single rxReplaceDocuments(String collection, JsonObject query, JsonObject replace) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replaceDocuments(collection, query, replace, fut);
}));
}
/**
* Replace matching documents in the specified collection, specifying options
*
* This operation might change _id field of replace parameter
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @param resultHandler will be called when complete
* @return
*/
public MongoClient replaceWithOptions(String collection, JsonObject query, JsonObject replace, UpdateOptions options, Handler> resultHandler) {
delegate.replaceWithOptions(collection, query, replace, options, resultHandler);
return this;
}
/**
* Replace matching documents in the specified collection, specifying options
*
* This operation might change _id field of replace parameter
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @return
* @deprecated use {@link #rxReplaceWithOptions} instead
*/
@Deprecated()
public Observable replaceWithOptionsObservable(String collection, JsonObject query, JsonObject replace, UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replaceWithOptions(collection, query, replace, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Replace matching documents in the specified collection, specifying options
*
* This operation might change _id field of replace parameter
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @return
*/
public Single rxReplaceWithOptions(String collection, JsonObject query, JsonObject replace, UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replaceWithOptions(collection, query, replace, options, fut);
}));
}
/**
* Replace matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @param resultHandler will be called when complete
* @return
*/
public MongoClient replaceDocumentsWithOptions(String collection, JsonObject query, JsonObject replace, UpdateOptions options, Handler> resultHandler) {
delegate.replaceDocumentsWithOptions(collection, query, replace, options, resultHandler);
return this;
}
/**
* Replace matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @return
* @deprecated use {@link #rxReplaceDocumentsWithOptions} instead
*/
@Deprecated()
public Observable replaceDocumentsWithOptionsObservable(String collection, JsonObject query, JsonObject replace, UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replaceDocumentsWithOptions(collection, query, replace, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Replace matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @return
*/
public Single rxReplaceDocumentsWithOptions(String collection, JsonObject query, JsonObject replace, UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replaceDocumentsWithOptions(collection, query, replace, options, fut);
}));
}
/**
* Execute a bulk operation. Can insert, update, replace, and/or delete multiple documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @param resultHandler will be called with a {@link io.vertx.ext.mongo.MongoClientBulkWriteResult} when complete
* @return
*/
public MongoClient bulkWrite(String collection, List operations, Handler> resultHandler) {
delegate.bulkWrite(collection, operations, resultHandler);
return this;
}
/**
* Execute a bulk operation. Can insert, update, replace, and/or delete multiple documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @return
* @deprecated use {@link #rxBulkWrite} instead
*/
@Deprecated()
public Observable bulkWriteObservable(String collection, List operations) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
bulkWrite(collection, operations, resultHandler.toHandler());
return resultHandler;
}
/**
* Execute a bulk operation. Can insert, update, replace, and/or delete multiple documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @return
*/
public Single rxBulkWrite(String collection, List operations) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
bulkWrite(collection, operations, fut);
}));
}
/**
* Execute a bulk operation with the specified write options. Can insert, update, replace, and/or delete multiple
* documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @param bulkWriteOptions the write options
* @param resultHandler will be called with a {@link io.vertx.ext.mongo.MongoClientBulkWriteResult} when complete
* @return
*/
public MongoClient bulkWriteWithOptions(String collection, List operations, BulkWriteOptions bulkWriteOptions, Handler> resultHandler) {
delegate.bulkWriteWithOptions(collection, operations, bulkWriteOptions, resultHandler);
return this;
}
/**
* Execute a bulk operation with the specified write options. Can insert, update, replace, and/or delete multiple
* documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @param bulkWriteOptions the write options
* @return
* @deprecated use {@link #rxBulkWriteWithOptions} instead
*/
@Deprecated()
public Observable bulkWriteWithOptionsObservable(String collection, List operations, BulkWriteOptions bulkWriteOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
bulkWriteWithOptions(collection, operations, bulkWriteOptions, resultHandler.toHandler());
return resultHandler;
}
/**
* Execute a bulk operation with the specified write options. Can insert, update, replace, and/or delete multiple
* documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @param bulkWriteOptions the write options
* @return
*/
public Single rxBulkWriteWithOptions(String collection, List operations, BulkWriteOptions bulkWriteOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
bulkWriteWithOptions(collection, operations, bulkWriteOptions, fut);
}));
}
/**
* Find matching documents in the specified collection
* @param collection the collection
* @param query query used to match documents
* @param resultHandler will be provided with list of documents
* @return
*/
public MongoClient find(String collection, JsonObject query, Handler>> resultHandler) {
delegate.find(collection, query, resultHandler);
return this;
}
/**
* Find matching documents in the specified collection
* @param collection the collection
* @param query query used to match documents
* @return
* @deprecated use {@link #rxFind} instead
*/
@Deprecated()
public Observable> findObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture> resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
find(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Find matching documents in the specified collection
* @param collection the collection
* @param query query used to match documents
* @return
*/
public Single> rxFind(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
find(collection, query, fut);
}));
}
/**
* Find matching documents in the specified collection.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @param resultHandler will be provided with each found document
* @return
*/
public MongoClient findBatch(String collection, JsonObject query, Handler> resultHandler) {
delegate.findBatch(collection, query, resultHandler);
return this;
}
/**
* Find matching documents in the specified collection.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @return
* @deprecated use {@link #rxFindBatch} instead
*/
@Deprecated()
public Observable findBatchObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findBatch(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Find matching documents in the specified collection.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @return
*/
public Single rxFindBatch(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findBatch(collection, query, fut);
}));
}
/**
* Find matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @param resultHandler will be provided with list of documents
* @return
*/
public MongoClient findWithOptions(String collection, JsonObject query, FindOptions options, Handler>> resultHandler) {
delegate.findWithOptions(collection, query, options, resultHandler);
return this;
}
/**
* Find matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return
* @deprecated use {@link #rxFindWithOptions} instead
*/
@Deprecated()
public Observable> findWithOptionsObservable(String collection, JsonObject query, FindOptions options) {
io.vertx.rx.java.ObservableFuture> resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findWithOptions(collection, query, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Find matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return
*/
public Single> rxFindWithOptions(String collection, JsonObject query, FindOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findWithOptions(collection, query, options, fut);
}));
}
/**
* Find matching documents in the specified collection, specifying options.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @param resultHandler will be provided with each found document
* @return
*/
public MongoClient findBatchWithOptions(String collection, JsonObject query, FindOptions options, Handler> resultHandler) {
delegate.findBatchWithOptions(collection, query, options, resultHandler);
return this;
}
/**
* Find matching documents in the specified collection, specifying options.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return
* @deprecated use {@link #rxFindBatchWithOptions} instead
*/
@Deprecated()
public Observable findBatchWithOptionsObservable(String collection, JsonObject query, FindOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findBatchWithOptions(collection, query, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Find matching documents in the specified collection, specifying options.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return
*/
public Single rxFindBatchWithOptions(String collection, JsonObject query, FindOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findBatchWithOptions(collection, query, options, fut);
}));
}
/**
* Find a single matching document in the specified collection
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param fields the fields
* @param resultHandler will be provided with the document, if any
* @return
*/
public MongoClient findOne(String collection, JsonObject query, JsonObject fields, Handler> resultHandler) {
delegate.findOne(collection, query, fields, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param fields the fields
* @return
* @deprecated use {@link #rxFindOne} instead
*/
@Deprecated()
public Observable findOneObservable(String collection, JsonObject query, JsonObject fields) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOne(collection, query, fields, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param fields the fields
* @return
*/
public Single rxFindOne(String collection, JsonObject query, JsonObject fields) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOne(collection, query, fields, fut);
}));
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @param resultHandler will be provided with the document, if any
* @return
*/
public MongoClient findOneAndUpdate(String collection, JsonObject query, JsonObject update, Handler> resultHandler) {
delegate.findOneAndUpdate(collection, query, update, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @return
* @deprecated use {@link #rxFindOneAndUpdate} instead
*/
@Deprecated()
public Observable findOneAndUpdateObservable(String collection, JsonObject query, JsonObject update) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndUpdate(collection, query, update, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @return
*/
public Single rxFindOneAndUpdate(String collection, JsonObject query, JsonObject update) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndUpdate(collection, query, update, fut);
}));
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @param resultHandler will be provided with the document, if any
* @return
*/
public MongoClient findOneAndUpdateWithOptions(String collection, JsonObject query, JsonObject update, FindOptions findOptions, UpdateOptions updateOptions, Handler> resultHandler) {
delegate.findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
* @deprecated use {@link #rxFindOneAndUpdateWithOptions} instead
*/
@Deprecated()
public Observable findOneAndUpdateWithOptionsObservable(String collection, JsonObject query, JsonObject update, FindOptions findOptions, UpdateOptions updateOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
*/
public Single rxFindOneAndUpdateWithOptions(String collection, JsonObject query, JsonObject update, FindOptions findOptions, UpdateOptions updateOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, fut);
}));
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @param resultHandler will be provided with the document, if any
* @return
*/
public MongoClient findOneAndReplace(String collection, JsonObject query, JsonObject replace, Handler> resultHandler) {
delegate.findOneAndReplace(collection, query, replace, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @return
* @deprecated use {@link #rxFindOneAndReplace} instead
*/
@Deprecated()
public Observable findOneAndReplaceObservable(String collection, JsonObject query, JsonObject replace) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndReplace(collection, query, replace, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @return
*/
public Single rxFindOneAndReplace(String collection, JsonObject query, JsonObject replace) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndReplace(collection, query, replace, fut);
}));
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @param resultHandler will be provided with the document, if any
* @return
*/
public MongoClient findOneAndReplaceWithOptions(String collection, JsonObject query, JsonObject replace, FindOptions findOptions, UpdateOptions updateOptions, Handler> resultHandler) {
delegate.findOneAndReplaceWithOptions(collection, query, replace, findOptions, updateOptions, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
* @deprecated use {@link #rxFindOneAndReplaceWithOptions} instead
*/
@Deprecated()
public Observable findOneAndReplaceWithOptionsObservable(String collection, JsonObject query, JsonObject replace, FindOptions findOptions, UpdateOptions updateOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndReplaceWithOptions(collection, query, replace, findOptions, updateOptions, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
*/
public Single rxFindOneAndReplaceWithOptions(String collection, JsonObject query, JsonObject replace, FindOptions findOptions, UpdateOptions updateOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndReplaceWithOptions(collection, query, replace, findOptions, updateOptions, fut);
}));
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param resultHandler will be provided with the deleted document, if any
* @return
*/
public MongoClient findOneAndDelete(String collection, JsonObject query, Handler> resultHandler) {
delegate.findOneAndDelete(collection, query, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @return
* @deprecated use {@link #rxFindOneAndDelete} instead
*/
@Deprecated()
public Observable findOneAndDeleteObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndDelete(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @return
*/
public Single rxFindOneAndDelete(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndDelete(collection, query, fut);
}));
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param findOptions options to configure the find
* @param resultHandler will be provided with the deleted document, if any
* @return
*/
public MongoClient findOneAndDeleteWithOptions(String collection, JsonObject query, FindOptions findOptions, Handler> resultHandler) {
delegate.findOneAndDeleteWithOptions(collection, query, findOptions, resultHandler);
return this;
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param findOptions options to configure the find
* @return
* @deprecated use {@link #rxFindOneAndDeleteWithOptions} instead
*/
@Deprecated()
public Observable findOneAndDeleteWithOptionsObservable(String collection, JsonObject query, FindOptions findOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndDeleteWithOptions(collection, query, findOptions, resultHandler.toHandler());
return resultHandler;
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param findOptions options to configure the find
* @return
*/
public Single rxFindOneAndDeleteWithOptions(String collection, JsonObject query, FindOptions findOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndDeleteWithOptions(collection, query, findOptions, fut);
}));
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @param resultHandler will be provided with the number of matching documents
* @return
*/
public MongoClient count(String collection, JsonObject query, Handler> resultHandler) {
delegate.count(collection, query, resultHandler);
return this;
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @return
* @deprecated use {@link #rxCount} instead
*/
@Deprecated()
public Observable countObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
count(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @return
*/
public Single rxCount(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
count(collection, query, fut);
}));
}
/**
* Remove matching documents from a collection
* @param collection the collection
* @param query query used to match documents
* @param resultHandler will be called when complete
* @return
*/
public MongoClient remove(String collection, JsonObject query, Handler> resultHandler) {
delegate.remove(collection, query, resultHandler);
return this;
}
/**
* Remove matching documents from a collection
* @param collection the collection
* @param query query used to match documents
* @return
* @deprecated use {@link #rxRemove} instead
*/
@Deprecated()
public Observable removeObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
remove(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove matching documents from a collection
* @param collection the collection
* @param query query used to match documents
* @return
*/
public Single rxRemove(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
remove(collection, query, fut);
}));
}
/**
* Remove matching documents from a collection and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match documents
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeDocuments(String collection, JsonObject query, Handler> resultHandler) {
delegate.removeDocuments(collection, query, resultHandler);
return this;
}
/**
* Remove matching documents from a collection and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match documents
* @return
* @deprecated use {@link #rxRemoveDocuments} instead
*/
@Deprecated()
public Observable removeDocumentsObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocuments(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove matching documents from a collection and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match documents
* @return
*/
public Single rxRemoveDocuments(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocuments(collection, query, fut);
}));
}
/**
* Remove matching documents from a collection with the specified write option
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeWithOptions(String collection, JsonObject query, WriteOption writeOption, Handler> resultHandler) {
delegate.removeWithOptions(collection, query, writeOption, resultHandler);
return this;
}
/**
* Remove matching documents from a collection with the specified write option
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @return
* @deprecated use {@link #rxRemoveWithOptions} instead
*/
@Deprecated()
public Observable removeWithOptionsObservable(String collection, JsonObject query, WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove matching documents from a collection with the specified write option
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @return
*/
public Single rxRemoveWithOptions(String collection, JsonObject query, WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeWithOptions(collection, query, writeOption, fut);
}));
}
/**
* Remove matching documents from a collection with the specified write option and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeDocumentsWithOptions(String collection, JsonObject query, WriteOption writeOption, Handler> resultHandler) {
delegate.removeDocumentsWithOptions(collection, query, writeOption, resultHandler);
return this;
}
/**
* Remove matching documents from a collection with the specified write option and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @return
* @deprecated use {@link #rxRemoveDocumentsWithOptions} instead
*/
@Deprecated()
public Observable removeDocumentsWithOptionsObservable(String collection, JsonObject query, WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocumentsWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove matching documents from a collection with the specified write option and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @return
*/
public Single rxRemoveDocumentsWithOptions(String collection, JsonObject query, WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocumentsWithOptions(collection, query, writeOption, fut);
}));
}
/**
* Remove a single matching document from a collection
* @param collection the collection
* @param query query used to match document
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeOne(String collection, JsonObject query, Handler> resultHandler) {
delegate.removeOne(collection, query, resultHandler);
return this;
}
/**
* Remove a single matching document from a collection
* @param collection the collection
* @param query query used to match document
* @return
* @deprecated use {@link #rxRemoveOne} instead
*/
@Deprecated()
public Observable removeOneObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeOne(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove a single matching document from a collection
* @param collection the collection
* @param query query used to match document
* @return
*/
public Single rxRemoveOne(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeOne(collection, query, fut);
}));
}
/**
* Remove a single matching document from a collection and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match document
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeDocument(String collection, JsonObject query, Handler> resultHandler) {
delegate.removeDocument(collection, query, resultHandler);
return this;
}
/**
* Remove a single matching document from a collection and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match document
* @return
* @deprecated use {@link #rxRemoveDocument} instead
*/
@Deprecated()
public Observable removeDocumentObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocument(collection, query, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove a single matching document from a collection and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match document
* @return
*/
public Single rxRemoveDocument(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocument(collection, query, fut);
}));
}
/**
* Remove a single matching document from a collection with the specified write option
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeOneWithOptions(String collection, JsonObject query, WriteOption writeOption, Handler> resultHandler) {
delegate.removeOneWithOptions(collection, query, writeOption, resultHandler);
return this;
}
/**
* Remove a single matching document from a collection with the specified write option
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @return
* @deprecated use {@link #rxRemoveOneWithOptions} instead
*/
@Deprecated()
public Observable removeOneWithOptionsObservable(String collection, JsonObject query, WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeOneWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove a single matching document from a collection with the specified write option
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @return
*/
public Single rxRemoveOneWithOptions(String collection, JsonObject query, WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeOneWithOptions(collection, query, writeOption, fut);
}));
}
/**
* Remove a single matching document from a collection with the specified write option and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @param resultHandler will be called when complete
* @return
*/
public MongoClient removeDocumentWithOptions(String collection, JsonObject query, WriteOption writeOption, Handler> resultHandler) {
delegate.removeDocumentWithOptions(collection, query, writeOption, resultHandler);
return this;
}
/**
* Remove a single matching document from a collection with the specified write option and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @return
* @deprecated use {@link #rxRemoveDocumentWithOptions} instead
*/
@Deprecated()
public Observable removeDocumentWithOptionsObservable(String collection, JsonObject query, WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocumentWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
/**
* Remove a single matching document from a collection with the specified write option and return the handler with MongoClientDeleteResult result
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @return
*/
public Single rxRemoveDocumentWithOptions(String collection, JsonObject query, WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocumentWithOptions(collection, query, writeOption, fut);
}));
}
/**
* Create a new collection
* @param collectionName the name of the collection
* @param resultHandler will be called when complete
* @return
*/
public MongoClient createCollection(String collectionName, Handler> resultHandler) {
delegate.createCollection(collectionName, resultHandler);
return this;
}
/**
* Create a new collection
* @param collectionName the name of the collection
* @return
* @deprecated use {@link #rxCreateCollection} instead
*/
@Deprecated()
public Observable createCollectionObservable(String collectionName) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
createCollection(collectionName, resultHandler.toHandler());
return resultHandler;
}
/**
* Create a new collection
* @param collectionName the name of the collection
* @return
*/
public Single rxCreateCollection(String collectionName) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
createCollection(collectionName, fut);
}));
}
/**
* Get a list of all collections in the database.
* @param resultHandler will be called with a list of collections.
* @return
*/
public MongoClient getCollections(Handler>> resultHandler) {
delegate.getCollections(resultHandler);
return this;
}
/**
* Get a list of all collections in the database.
* @return
* @deprecated use {@link #rxGetCollections} instead
*/
@Deprecated()
public Observable> getCollectionsObservable() {
io.vertx.rx.java.ObservableFuture> resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
getCollections(resultHandler.toHandler());
return resultHandler;
}
/**
* Get a list of all collections in the database.
* @return
*/
public Single> rxGetCollections() {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
getCollections(fut);
}));
}
/**
* Drop a collection
* @param collection the collection
* @param resultHandler will be called when complete
* @return
*/
public MongoClient dropCollection(String collection, Handler> resultHandler) {
delegate.dropCollection(collection, resultHandler);
return this;
}
/**
* Drop a collection
* @param collection the collection
* @return
* @deprecated use {@link #rxDropCollection} instead
*/
@Deprecated()
public Observable dropCollectionObservable(String collection) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
dropCollection(collection, resultHandler.toHandler());
return resultHandler;
}
/**
* Drop a collection
* @param collection the collection
* @return
*/
public Single rxDropCollection(String collection) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
dropCollection(collection, fut);
}));
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @param resultHandler will be called when complete
* @return
*/
public MongoClient createIndex(String collection, JsonObject key, Handler> resultHandler) {
delegate.createIndex(collection, key, resultHandler);
return this;
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @return
* @deprecated use {@link #rxCreateIndex} instead
*/
@Deprecated()
public Observable createIndexObservable(String collection, JsonObject key) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
createIndex(collection, key, resultHandler.toHandler());
return resultHandler;
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @return
*/
public Single rxCreateIndex(String collection, JsonObject key) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
createIndex(collection, key, fut);
}));
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @param options the options for the index
* @param resultHandler will be called when complete
* @return
*/
public MongoClient createIndexWithOptions(String collection, JsonObject key, IndexOptions options, Handler> resultHandler) {
delegate.createIndexWithOptions(collection, key, options, resultHandler);
return this;
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @param options the options for the index
* @return
* @deprecated use {@link #rxCreateIndexWithOptions} instead
*/
@Deprecated()
public Observable createIndexWithOptionsObservable(String collection, JsonObject key, IndexOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
createIndexWithOptions(collection, key, options, resultHandler.toHandler());
return resultHandler;
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @param options the options for the index
* @return
*/
public Single rxCreateIndexWithOptions(String collection, JsonObject key, IndexOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
createIndexWithOptions(collection, key, options, fut);
}));
}
/**
* Get all the indexes in this collection.
* @param collection the collection
* @param resultHandler will be called when complete
* @return
*/
public MongoClient listIndexes(String collection, Handler> resultHandler) {
delegate.listIndexes(collection, resultHandler);
return this;
}
/**
* Get all the indexes in this collection.
* @param collection the collection
* @return
* @deprecated use {@link #rxListIndexes} instead
*/
@Deprecated()
public Observable listIndexesObservable(String collection) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
listIndexes(collection, resultHandler.toHandler());
return resultHandler;
}
/**
* Get all the indexes in this collection.
* @param collection the collection
* @return
*/
public Single rxListIndexes(String collection) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
listIndexes(collection, fut);
}));
}
/**
* Drops the index given its name.
* @param collection the collection
* @param indexName the name of the index to remove
* @param resultHandler will be called when complete
* @return
*/
public MongoClient dropIndex(String collection, String indexName, Handler> resultHandler) {
delegate.dropIndex(collection, indexName, resultHandler);
return this;
}
/**
* Drops the index given its name.
* @param collection the collection
* @param indexName the name of the index to remove
* @return
* @deprecated use {@link #rxDropIndex} instead
*/
@Deprecated()
public Observable dropIndexObservable(String collection, String indexName) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
dropIndex(collection, indexName, resultHandler.toHandler());
return resultHandler;
}
/**
* Drops the index given its name.
* @param collection the collection
* @param indexName the name of the index to remove
* @return
*/
public Single rxDropIndex(String collection, String indexName) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
dropIndex(collection, indexName, fut);
}));
}
/**
* Run an arbitrary MongoDB command.
* @param commandName the name of the command
* @param command the command
* @param resultHandler will be called with the result.
* @return
*/
public MongoClient runCommand(String commandName, JsonObject command, Handler> resultHandler) {
delegate.runCommand(commandName, command, resultHandler);
return this;
}
/**
* Run an arbitrary MongoDB command.
* @param commandName the name of the command
* @param command the command
* @return
* @deprecated use {@link #rxRunCommand} instead
*/
@Deprecated()
public Observable runCommandObservable(String commandName, JsonObject command) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
runCommand(commandName, command, resultHandler.toHandler());
return resultHandler;
}
/**
* Run an arbitrary MongoDB command.
* @param commandName the name of the command
* @param command the command
* @return
*/
public Single rxRunCommand(String commandName, JsonObject command) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
runCommand(commandName, command, fut);
}));
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param resultHandler will be provided with array of values.
* @return
*/
public MongoClient distinct(String collection, String fieldName, String resultClassname, Handler> resultHandler) {
delegate.distinct(collection, fieldName, resultClassname, resultHandler);
return this;
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return
* @deprecated use {@link #rxDistinct} instead
*/
@Deprecated()
public Observable distinctObservable(String collection, String fieldName, String resultClassname) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
distinct(collection, fieldName, resultClassname, resultHandler.toHandler());
return resultHandler;
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return
*/
public Single rxDistinct(String collection, String fieldName, String resultClassname) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
distinct(collection, fieldName, resultClassname, fut);
}));
}
/**
* Gets the distinct values of the specified field name.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param resultHandler will be provided with each found value
* @return
*/
public MongoClient distinctBatch(String collection, String fieldName, String resultClassname, Handler> resultHandler) {
delegate.distinctBatch(collection, fieldName, resultClassname, resultHandler);
return this;
}
/**
* Gets the distinct values of the specified field name.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return
* @deprecated use {@link #rxDistinctBatch} instead
*/
@Deprecated()
public Observable distinctBatchObservable(String collection, String fieldName, String resultClassname) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
distinctBatch(collection, fieldName, resultClassname, resultHandler.toHandler());
return resultHandler;
}
/**
* Gets the distinct values of the specified field name.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return
*/
public Single rxDistinctBatch(String collection, String fieldName, String resultClassname) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
distinctBatch(collection, fieldName, resultClassname, fut);
}));
}
/**
* Close the client and release its resources
*/
public void close() {
delegate.close();
}
public static MongoClient newInstance(io.vertx.ext.mongo.MongoClient arg) {
return arg != null ? new MongoClient(arg) : null;
}
}