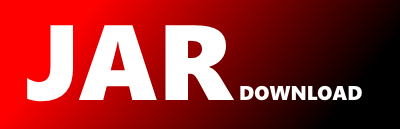
io.vertx.ext.mongo.MongoGridFsClient Maven / Gradle / Ivy
package io.vertx.ext.mongo;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.codegen.annotations.VertxGen;
import io.vertx.core.AsyncResult;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.buffer.Buffer;
import io.vertx.core.json.JsonObject;
import io.vertx.core.streams.ReadStream;
import io.vertx.core.streams.WriteStream;
import java.util.List;
@VertxGen
public interface MongoGridFsClient {
/**
* Deletes a file by it's ID
*
* @param id the identifier of the file
* @param resultHandler will be called when the file is deleted
*/
@Fluent
MongoGridFsClient delete(String id, Handler> resultHandler);
/**
* Like {@link #delete(String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future delete(String id);
@Fluent
MongoGridFsClient downloadByFileName(WriteStream stream, String fileName, Handler> resultHandler);
/**
* Like {@link #downloadByFileName(WriteStream, String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future downloadByFileName(WriteStream stream, String fileName);
@Fluent
MongoGridFsClient downloadByFileNameWithOptions(WriteStream stream, String fileName, GridFsDownloadOptions options, Handler> resultHandler);
/**
* Like {@link #downloadByFileNameWithOptions(WriteStream, String, GridFsDownloadOptions, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future downloadByFileNameWithOptions(WriteStream stream, String fileName, GridFsDownloadOptions options);
@Fluent
MongoGridFsClient downloadById(WriteStream stream, String id, Handler> resultHandler);
/**
* Like {@link #downloadById(WriteStream, String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future downloadById(WriteStream stream, String id);
/**
* Downloads a file.
*
* @param fileName the name of the file to download
* @param resultHandler called when the file is downloaded and returns the length in bytes
*/
@Fluent
MongoGridFsClient downloadFile(String fileName, Handler> resultHandler);
/**
* Like {@link #downloadFile(String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future downloadFile(String fileName);
/**
* Downloads a file and gives it a new name.
*
* @param fileName the name of the file to download
* @param newFileName the name the file should be saved as
* @param resultHandler called when the file is downloaded and returns the length in bytes
*/
@Fluent
MongoGridFsClient downloadFileAs(String fileName, String newFileName, Handler> resultHandler);
/**
* Like {@link #downloadFileAs(String, String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future downloadFileAs(String fileName, String newFileName);
/**
* Downloads a file using the ID generated by GridFs.
*
* @param id the GridFs Object ID of the file to download
* @param resultHandler called when the file is downloaded and returns the length in bytes
*/
@Fluent
MongoGridFsClient downloadFileByID(String id, String fileName, Handler> resultHandler);
/**
* Like {@link #downloadFileByID(String, String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future downloadFileByID(String id, String fileName);
/**
* Drops the entire file bucket with all of its contents
*
* @param resultHandler called when the bucket is dropped
*/
@Fluent
MongoGridFsClient drop(Handler> resultHandler);
/**
* Like {@link #drop(Handler)} but returns a {@code Future} of the asynchronous result
*/
Future drop();
/**
* Finds all file ids in the bucket
*
* @param resultHandler called when the list of file ids is available
*/
@Fluent
MongoGridFsClient findAllIds(Handler>> resultHandler);
/**
* Like {@link #findAllIds(Handler)} but returns a {@code Future} of the asynchronous result
*/
Future> findAllIds();
/**
* Finds all file ids that match a query.
*
* @param query a bson query expressed as json that will be used to match files
* @param resultHandler called when the list of file ids is available
*/
@Fluent
MongoGridFsClient findIds(JsonObject query, Handler>> resultHandler);
/**
* Like {@link #findIds(JsonObject, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future> findIds(JsonObject query);
@Fluent
MongoGridFsClient uploadByFileName(ReadStream stream, String fileName, Handler> resultHandler);
/**
* Like {@link #uploadByFileName(ReadStream, String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future uploadByFileName(ReadStream stream, String fileName);
@Fluent
MongoGridFsClient uploadByFileNameWithOptions(ReadStream stream, String fileName, GridFsUploadOptions options, Handler> resultHandler);
/**
* Like {@link #uploadByFileNameWithOptions(ReadStream, String, GridFsUploadOptions, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future uploadByFileNameWithOptions(ReadStream stream, String fileName, GridFsUploadOptions options);
/**
* Upload a file to gridfs
*
* @param fileName the name of the file to store in gridfs
* @param resultHandler the id of the file that was uploaded
*/
@Fluent
MongoGridFsClient uploadFile(String fileName, Handler> resultHandler);
/**
* Like {@link #uploadFile(String, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future uploadFile(String fileName);
/**
* Upload a file to gridfs with options
*
* @param fileName the name of the file to store in gridfs
* @param options {@link GridFsUploadOptions} for specifying metadata and chunk size
* @param resultHandler the id of the file that was uploaded
*/
@Fluent
MongoGridFsClient uploadFileWithOptions(String fileName, GridFsUploadOptions options, Handler> resultHandler);
/**
* Like {@link #uploadFileWithOptions(String, GridFsUploadOptions, Handler)} but returns a {@code Future} of the asynchronous result
*/
Future uploadFileWithOptions(String fileName, GridFsUploadOptions options);
/**
* Close the client and release its resources
*/
void close();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy