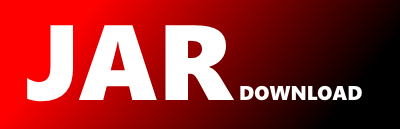
io.vertx.openapi.contract.impl.SecurityRequirementImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2023, SAP SE
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the Apache License, Version 2.0
* which is available at https://www.apache.org/licenses/LICENSE-2.0.
*
* SPDX-License-Identifier: EPL-2.0 OR Apache-2.0
*
*/
package io.vertx.openapi.contract.impl;
import io.vertx.core.json.JsonObject;
import io.vertx.json.schema.JsonSchema;
import io.vertx.openapi.contract.SecurityRequirement;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import static io.vertx.openapi.impl.Utils.EMPTY_JSON_ARRAY;
import static java.util.Collections.emptyMap;
import static java.util.Collections.emptySet;
import static java.util.Collections.unmodifiableMap;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.toList;
import static java.util.stream.Collectors.toUnmodifiableSet;
public class SecurityRequirementImpl implements SecurityRequirement {
private final JsonObject securityRequirementModel;
private final Set names;
private final Map> scopes;
public SecurityRequirementImpl(JsonObject securityRequirementModel) {
this.securityRequirementModel = securityRequirementModel;
if (securityRequirementModel.isEmpty()) {
this.names = emptySet();
this.scopes = emptyMap();
} else {
this.names = securityRequirementModel
.fieldNames()
.stream()
.filter(JsonSchema.EXCLUDE_ANNOTATIONS).collect(toUnmodifiableSet());
this.scopes = unmodifiableMap(
this.names
.stream()
.collect(Collectors.toMap(identity(), name -> extractScopes(securityRequirementModel, name))));
}
}
private static List extractScopes(JsonObject securityRequirementModel, String name) {
return securityRequirementModel.getJsonArray(name, EMPTY_JSON_ARRAY).stream().map(Object::toString)
.collect(toList());
}
@Override
public JsonObject getOpenAPIModel() {
return securityRequirementModel;
}
@Override
public Set getNames() {
return names;
}
@Override
public List getScopes(String name) {
if (scopes.containsKey(name)) {
return scopes.get(name);
}
throw new IllegalArgumentException("No security requirement with name " + name);
}
@Override
public boolean isEmpty() {
return names.isEmpty();
}
@Override
public int size() {
return names.size();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy