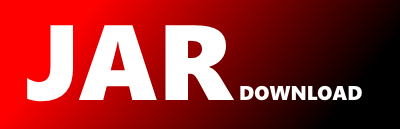
io.vertx.pgclient.PgException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-pg-client Show documentation
Show all versions of vertx-pg-client Show documentation
The Reactive PostgreSQL Client
/*
* Copyright (C) 2017 Julien Viet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package io.vertx.pgclient;
import io.vertx.core.json.Json;
/**
* PostgreSQL error including all fields
* of the ErrorResponse message of the PostgreSQL frontend/backend protocol.
*
* @author Julien Viet
*/
public class PgException extends RuntimeException {
private static String formatMessage(String errorMessage, String severity, String code) {
StringBuilder sb = new StringBuilder();
if (severity != null) {
sb.append(severity).append(":");
}
if (errorMessage != null) {
if (sb.length() > 0) {
sb.append(' ');
}
sb.append(errorMessage);
}
if (code != null) {
if (sb.length() > 0) {
sb.append(' ');
}
sb.append('(').append(code).append(')');
}
return sb.toString();
}
private final String errorMessage;
private final String severity;
private final String code;
private final String detail;
private final String hint;
private final String position;
private final String internalPosition;
private final String internalQuery;
private final String where;
private final String file;
private final String line;
private final String routine;
private final String schema;
private final String table;
private final String column;
private final String dataType;
private final String constraint;
public PgException(String errorMessage, String severity, String code, String detail) {
super(formatMessage(errorMessage, severity, code));
this.errorMessage = errorMessage;
this.severity = severity;
this.code = code;
this.detail = detail;
this.hint = null;
this.position = null;
this.internalPosition = null;
this.internalQuery = null;
this.where = null;
this.file = null;
this.line = null;
this.routine = null;
this.schema = null;
this.table = null;
this.column = null;
this.dataType = null;
this.constraint = null;
}
public PgException(String errorMessage, String severity, String code, String detail, String hint, String position,
String internalPosition, String internalQuery, String where, String file, String line, String routine,
String schema, String table, String column, String dataType, String constraint) {
super(formatMessage(errorMessage, severity, code));
this.errorMessage = errorMessage;
this.severity = severity;
this.code = code;
this.detail = detail;
this.hint = hint;
this.position = position;
this.internalPosition = internalPosition;
this.internalQuery = internalQuery;
this.where = where;
this.file = file;
this.line = line;
this.routine = routine;
this.schema = schema;
this.table = table;
this.column = column;
this.dataType = dataType;
this.constraint = constraint;
}
/**
* @return the primary human-readable error message
* ('M' field)
*/
public String getErrorMessage() {
// getErrorMessage() avoids name clash with RuntimeException#getMessage()
return errorMessage;
}
/**
* @return the severity: ERROR, FATAL, or PANIC, or a localized translation of one of these
* ('S' field)
*/
public String getSeverity() {
return severity;
}
/**
* @return the SQLSTATE code for the error
* ('S' field,
* value list),
* it is never localized
*/
public String getCode() {
return code;
}
/**
* @return an optional secondary error message carrying more detail about the problem
* ('D' field),
* a newline indicates paragraph break.
*/
public String getDetail() {
return detail;
}
/**
* @return an optional suggestion (advice) what to do about the problem
* ('H' field),
* a newline indicates paragraph break.
*/
public String getHint() {
return hint;
}
/**
* @return a decimal ASCII integer, indicating an error cursor position as an index into the original
* query string. ('P' field).
* The first character has index 1, and positions are measured in characters not bytes.
*/
public String getPosition() {
return position;
}
/**
* @return an indication of the context in which the error occurred
* ('W' field).
* Presently this includes a call stack traceback of active procedural language functions and
* internally-generated queries. The trace is one entry per line, most recent first.
*/
public String getWhere() {
return where;
}
/**
* @return file name of the source-code location where the error was reported
* ('F' field).
*/
public String getFile() {
return file;
}
/**
* @return line number of the source-code location where the error was reported
* ('L' field).
*/
public String getLine() {
return line;
}
/**
* @return name of the source-code routine reporting the error
* ('R' field).
*/
public String getRoutine() {
return routine;
}
/**
* @return if the error was associated with a specific database object, the name of the schema containing
* that object, if any
* ('s' field).
*/
public String getSchema() {
return schema;
}
/**
* @return if the error was associated with a specific table, the name of the table
* ('t' field).
*/
public String getTable() {
return table;
}
/**
* @return if the error was associated with a specific table column, the name of the column
* ('c' field).
*/
public String getColumn() {
return column;
}
/**
* @return if the error was associated with a specific data type, the name of the data type
* ('d' field).
*/
public String getDataType() {
return dataType;
}
/**
* @return if the error was associated with a specific constraint, the name of the constraint
* ('n' field).
*/
public String getConstraint() {
return constraint;
}
/**
* @return a decimal ASCII integer, indicating an error cursor position
* ('p' field)
* as an index into the internally generated command (see 'q' field).
*/
public String getInternalPosition() {
return internalPosition;
}
/**
* @return the text of a failed internally-generated command
* ('q' field).
*/
public String getInternalQuery() {
return internalQuery;
}
private static void append(StringBuffer stringBuffer, String key, String value) {
if (value != null) {
stringBuffer.append(", \"").append(key).append("\": ").append(Json.encode(value));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy