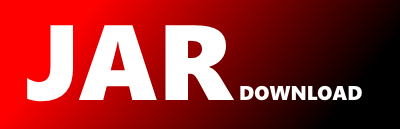
io.vertx.pgclient.data.Path Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-pg-client Show documentation
Show all versions of vertx-pg-client Show documentation
The Reactive PostgreSQL Client
package io.vertx.pgclient.data;
import io.vertx.codegen.annotations.DataObject;
import io.vertx.core.json.JsonObject;
import java.util.ArrayList;
import java.util.List;
/**
* Path data type in Postgres represented by lists of connected points.
* Paths can be open, where the first and last points in the list are considered not connected,
* or closed, where the first and last points are considered connected.
*/
public class Path {
private boolean isOpen;
private List points;
public Path() {
this(false, new ArrayList<>());
}
public Path(boolean isOpen, List points) {
this.isOpen = isOpen;
this.points = points;
}
public boolean isOpen() {
return isOpen;
}
public void setOpen(boolean open) {
isOpen = open;
}
public List getPoints() {
return points;
}
public void setPoints(List points) {
this.points = points;
}
public Path addPoint(Point point) {
if (points == null ) {
points = new ArrayList<>();
}
points.add(point);
return this;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Path path = (Path) o;
if (isOpen != path.isOpen) return false;
return points.equals(path.points);
}
@Override
public int hashCode() {
int result = (isOpen ? 1 : 0);
result = 31 * result + points.hashCode();
return result;
}
@Override
public String toString() {
String left;
String right;
if (isOpen) {
left = "[";
right = "]";
} else {
left = "(";
right = ")";
}
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append("Path");
stringBuilder.append(left);
for (int i = 0; i < points.size(); i++) {
Point point = points.get(i);
stringBuilder.append(point.toString());
if (i != points.size() - 1) {
// not the last one
stringBuilder.append(",");
}
}
stringBuilder.append(right);
return stringBuilder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy