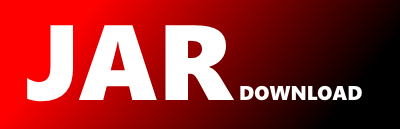
io.vertx.pgclient.impl.PgConnectionImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vertx-pg-client Show documentation
Show all versions of vertx-pg-client Show documentation
The Reactive PostgreSQL Client
/*
* Copyright (C) 2017 Julien Viet
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package io.vertx.pgclient.impl;
import io.vertx.core.impl.ContextInternal;
import io.vertx.core.spi.metrics.ClientMetrics;
import io.vertx.pgclient.PgConnectOptions;
import io.vertx.pgclient.PgConnection;
import io.vertx.pgclient.PgNotice;
import io.vertx.pgclient.PgNotification;
import io.vertx.pgclient.impl.codec.NoticeResponse;
import io.vertx.pgclient.spi.PgDriver;
import io.vertx.sqlclient.impl.Connection;
import io.vertx.sqlclient.impl.Notification;
import io.vertx.sqlclient.impl.SocketConnectionBase;
import io.vertx.sqlclient.impl.SqlConnectionBase;
import io.vertx.core.AsyncResult;
import io.vertx.core.Context;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.Vertx;
import io.vertx.pgclient.impl.codec.TxFailedEvent;
import io.vertx.sqlclient.impl.tracing.QueryTracer;
public class PgConnectionImpl extends SqlConnectionBase implements PgConnection {
public static Future connect(ContextInternal context, PgConnectOptions options) {
if (options.isUsingDomainSocket() && !context.owner().isNativeTransportEnabled()) {
return context.failedFuture("Native transport is not available");
} else {
PgConnectionFactory client;
try {
client = new PgConnectionFactory(context.owner(), options);
} catch (Exception e) {
return context.failedFuture(e);
}
context.addCloseHook(client);
return (Future) client.connect(context);
}
}
private volatile Handler notificationHandler;
private volatile Handler noticeHandler;
public PgConnectionImpl(PgConnectionFactory factory, ContextInternal context, Connection conn, QueryTracer tracer, ClientMetrics metrics) {
super(context, factory, conn, PgDriver.INSTANCE, tracer, metrics);
}
@Override
public PgConnection notificationHandler(Handler handler) {
notificationHandler = handler;
return this;
}
public void handleEvent(Object event) {
if (event instanceof Notification) {
Handler handler = notificationHandler;
if (handler != null) {
Notification notification = (Notification) event;
handler.handle(new PgNotification()
.setChannel(notification.getChannel())
.setProcessId(notification.getProcessId())
.setPayload(notification.getPayload()));
}
} else if (event instanceof NoticeResponse) {
Handler handler = noticeHandler;
NoticeResponse noticeEvent = (NoticeResponse) event;
PgNotice notice = new PgNotice()
.setSeverity(noticeEvent.getSeverity())
.setCode(noticeEvent.getCode())
.setMessage(noticeEvent.getMessage())
.setDetail(noticeEvent.getDetail())
.setHint(noticeEvent.getHint())
.setPosition(noticeEvent.getPosition())
.setInternalPosition(noticeEvent.getInternalPosition())
.setInternalQuery(noticeEvent.getInternalQuery())
.setWhere(noticeEvent.getWhere())
.setFile(noticeEvent.getFile())
.setLine(noticeEvent.getLine())
.setRoutine(noticeEvent.getRoutine())
.setSchema(noticeEvent.getSchema())
.setTable(noticeEvent.getTable())
.setColumn(noticeEvent.getColumn())
.setDataType(noticeEvent.getDataType())
.setConstraint(noticeEvent.getConstraint());
if (handler != null) {
handler.handle(notice
);
} else {
notice.log(SocketConnectionBase.logger);
}
} else if (event instanceof TxFailedEvent) {
if (tx != null) {
tx.fail();
}
}
}
@Override
public PgConnection noticeHandler(Handler handler) {
noticeHandler = handler;
return this;
}
@Override
public int processId() {
return conn.getProcessId();
}
@Override
public int secretKey() {
return conn.getSecretKey();
}
@Override
public PgConnection cancelRequest(Handler> handler) {
Context current = Vertx.currentContext();
if (current == context) {
((PgConnectionFactory)factory).cancelRequest(conn.server(), this.processId(), this.secretKey(), handler);
} else {
context.runOnContext(v -> cancelRequest(handler));
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy