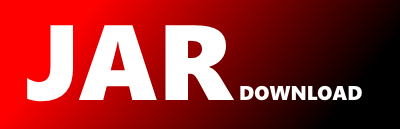
io.vertx.rx.java.UnmarshallerOperator Maven / Gradle / Ivy
package io.vertx.rx.java;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.ObjectCodec;
import com.fasterxml.jackson.core.type.TypeReference;
import io.vertx.core.buffer.Buffer;
import io.vertx.core.json.Json;
import io.vertx.core.json.jackson.JacksonFactory;
import rx.Observable;
import rx.Subscriber;
import static java.util.Objects.nonNull;
/**
* An operator
*
* @author Julien Viet
*/
public abstract class UnmarshallerOperator implements Observable.Operator {
private final Class mappedType;
private final TypeReference mappedTypeRef;
private ObjectCodec mapper;
public UnmarshallerOperator(Class mappedType) {
this.mappedType = mappedType;
this.mapper = null;
this.mappedTypeRef = null;
}
public UnmarshallerOperator(Class mappedType, ObjectCodec mapper) {
this.mappedType = mappedType;
this.mapper = mapper;
this.mappedTypeRef = null;
}
public UnmarshallerOperator(TypeReference mappedTypeRef) {
this.mappedType = null;
this.mapper = null;
this.mappedTypeRef = mappedTypeRef;
}
public UnmarshallerOperator(TypeReference mappedTypeRef, ObjectCodec mapper) {
this.mappedType = null;
this.mapper = mapper;
this.mappedTypeRef = mappedTypeRef;
}
public abstract Buffer unwrap(B buffer);
@Override
public Subscriber super B> call(Subscriber super T> subscriber) {
final Buffer buffer = Buffer.buffer();
return new Subscriber(subscriber) {
@Override
public void onCompleted() {
try {
T obj = null;
if (buffer.length() > 0) {
if (mapper != null) {
JsonParser parser = mapper.getFactory().createParser(buffer.getBytes());
obj = nonNull(mappedType) ? mapper.readValue(parser, mappedType) :
mapper.readValue(parser, mappedTypeRef);
} else {
obj = nonNull(mappedType) ? Json.CODEC.fromBuffer(buffer, mappedType) :
JacksonFactory.CODEC.fromBuffer(buffer, mappedTypeRef);
}
}
subscriber.onNext(obj);
subscriber.onCompleted();
} catch (Exception e) {
onError(e);
}
}
@Override
public void onError(Throwable e) {
subscriber.onError(e);
}
@Override
public void onNext(B item) {
buffer.appendBuffer(unwrap(item));
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy