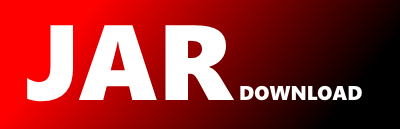
io.vertx.rxjava.ext.mongo.MongoService Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.mongo;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.mongo.MongoService original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.mongo.MongoService.class)
public class MongoService extends io.vertx.rxjava.ext.mongo.MongoClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MongoService that = (MongoService) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new MongoService((io.vertx.ext.mongo.MongoService) obj),
MongoService::getDelegate
);
private final io.vertx.ext.mongo.MongoService delegate;
public MongoService(io.vertx.ext.mongo.MongoService delegate) {
super(delegate);
this.delegate = delegate;
}
public MongoService(Object delegate) {
super((io.vertx.ext.mongo.MongoService)delegate);
this.delegate = (io.vertx.ext.mongo.MongoService)delegate;
}
public io.vertx.ext.mongo.MongoService getDelegate() {
return delegate;
}
/**
* Create a proxy to a service that is deployed somewhere on the event bus
* @param vertx the Vert.x instance
* @param address the address the service is listening on on the event bus
* @return the service
*/
public static io.vertx.rxjava.ext.mongo.MongoService createEventBusProxy(io.vertx.rxjava.core.Vertx vertx, String address) {
io.vertx.rxjava.ext.mongo.MongoService ret = io.vertx.rxjava.ext.mongo.MongoService.newInstance((io.vertx.ext.mongo.MongoService)io.vertx.ext.mongo.MongoService.createEventBusProxy(vertx.getDelegate(), address));
return ret;
}
public io.vertx.rxjava.ext.mongo.MongoService save(String collection, JsonObject document, Handler> resultHandler) {
delegate.save(collection, document, resultHandler);
return this;
}
@Deprecated()
public Observable saveObservable(String collection, JsonObject document) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
save(collection, document, resultHandler.toHandler());
return resultHandler;
}
public Single rxSave(String collection, JsonObject document) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
save(collection, document, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService saveWithOptions(String collection, JsonObject document, io.vertx.ext.mongo.WriteOption writeOption, Handler> resultHandler) {
delegate.saveWithOptions(collection, document, writeOption, resultHandler);
return this;
}
@Deprecated()
public Observable saveWithOptionsObservable(String collection, JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
saveWithOptions(collection, document, writeOption, resultHandler.toHandler());
return resultHandler;
}
public Single rxSaveWithOptions(String collection, JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
saveWithOptions(collection, document, writeOption, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService insert(String collection, JsonObject document, Handler> resultHandler) {
delegate.insert(collection, document, resultHandler);
return this;
}
@Deprecated()
public Observable insertObservable(String collection, JsonObject document) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
insert(collection, document, resultHandler.toHandler());
return resultHandler;
}
public Single rxInsert(String collection, JsonObject document) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
insert(collection, document, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService insertWithOptions(String collection, JsonObject document, io.vertx.ext.mongo.WriteOption writeOption, Handler> resultHandler) {
delegate.insertWithOptions(collection, document, writeOption, resultHandler);
return this;
}
@Deprecated()
public Observable insertWithOptionsObservable(String collection, JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
insertWithOptions(collection, document, writeOption, resultHandler.toHandler());
return resultHandler;
}
public Single rxInsertWithOptions(String collection, JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
insertWithOptions(collection, document, writeOption, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService update(String collection, JsonObject query, JsonObject update, Handler> resultHandler) {
delegate.update(collection, query, update, resultHandler);
return this;
}
@Deprecated()
public Observable updateObservable(String collection, JsonObject query, JsonObject update) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
update(collection, query, update, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxUpdate(String collection, JsonObject query, JsonObject update) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
update(collection, query, update, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService updateCollection(String collection, JsonObject query, JsonObject update, Handler> resultHandler) {
delegate.updateCollection(collection, query, update, resultHandler);
return this;
}
@Deprecated()
public Observable updateCollectionObservable(String collection, JsonObject query, JsonObject update) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
updateCollection(collection, query, update, resultHandler.toHandler());
return resultHandler;
}
public Single rxUpdateCollection(String collection, JsonObject query, JsonObject update) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
updateCollection(collection, query, update, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService updateWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.UpdateOptions options, Handler> resultHandler) {
delegate.updateWithOptions(collection, query, update, options, resultHandler);
return this;
}
@Deprecated()
public Observable updateWithOptionsObservable(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
updateWithOptions(collection, query, update, options, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxUpdateWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
updateWithOptions(collection, query, update, options, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService updateCollectionWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.UpdateOptions options, Handler> resultHandler) {
delegate.updateCollectionWithOptions(collection, query, update, options, resultHandler);
return this;
}
@Deprecated()
public Observable updateCollectionWithOptionsObservable(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
updateCollectionWithOptions(collection, query, update, options, resultHandler.toHandler());
return resultHandler;
}
public Single rxUpdateCollectionWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
updateCollectionWithOptions(collection, query, update, options, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService replace(String collection, JsonObject query, JsonObject replace, Handler> resultHandler) {
delegate.replace(collection, query, replace, resultHandler);
return this;
}
@Deprecated()
public Observable replaceObservable(String collection, JsonObject query, JsonObject replace) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replace(collection, query, replace, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxReplace(String collection, JsonObject query, JsonObject replace) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replace(collection, query, replace, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService replaceDocuments(String collection, JsonObject query, JsonObject replace, Handler> resultHandler) {
delegate.replaceDocuments(collection, query, replace, resultHandler);
return this;
}
@Deprecated()
public Observable replaceDocumentsObservable(String collection, JsonObject query, JsonObject replace) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replaceDocuments(collection, query, replace, resultHandler.toHandler());
return resultHandler;
}
public Single rxReplaceDocuments(String collection, JsonObject query, JsonObject replace) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replaceDocuments(collection, query, replace, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService replaceWithOptions(String collection, JsonObject query, JsonObject replace, io.vertx.ext.mongo.UpdateOptions options, Handler> resultHandler) {
delegate.replaceWithOptions(collection, query, replace, options, resultHandler);
return this;
}
@Deprecated()
public Observable replaceWithOptionsObservable(String collection, JsonObject query, JsonObject replace, io.vertx.ext.mongo.UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replaceWithOptions(collection, query, replace, options, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxReplaceWithOptions(String collection, JsonObject query, JsonObject replace, io.vertx.ext.mongo.UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replaceWithOptions(collection, query, replace, options, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService replaceDocumentsWithOptions(String collection, JsonObject query, JsonObject replace, io.vertx.ext.mongo.UpdateOptions options, Handler> resultHandler) {
delegate.replaceDocumentsWithOptions(collection, query, replace, options, resultHandler);
return this;
}
@Deprecated()
public Observable replaceDocumentsWithOptionsObservable(String collection, JsonObject query, JsonObject replace, io.vertx.ext.mongo.UpdateOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
replaceDocumentsWithOptions(collection, query, replace, options, resultHandler.toHandler());
return resultHandler;
}
public Single rxReplaceDocumentsWithOptions(String collection, JsonObject query, JsonObject replace, io.vertx.ext.mongo.UpdateOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
replaceDocumentsWithOptions(collection, query, replace, options, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService bulkWrite(String collection, List operations, Handler> resultHandler) {
delegate.bulkWrite(collection, operations, resultHandler);
return this;
}
@Deprecated()
public Observable bulkWriteObservable(String collection, List operations) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
bulkWrite(collection, operations, resultHandler.toHandler());
return resultHandler;
}
public Single rxBulkWrite(String collection, List operations) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
bulkWrite(collection, operations, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService bulkWriteWithOptions(String collection, List operations, io.vertx.ext.mongo.BulkWriteOptions bulkWriteOptions, Handler> resultHandler) {
delegate.bulkWriteWithOptions(collection, operations, bulkWriteOptions, resultHandler);
return this;
}
@Deprecated()
public Observable bulkWriteWithOptionsObservable(String collection, List operations, io.vertx.ext.mongo.BulkWriteOptions bulkWriteOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
bulkWriteWithOptions(collection, operations, bulkWriteOptions, resultHandler.toHandler());
return resultHandler;
}
public Single rxBulkWriteWithOptions(String collection, List operations, io.vertx.ext.mongo.BulkWriteOptions bulkWriteOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
bulkWriteWithOptions(collection, operations, bulkWriteOptions, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService find(String collection, JsonObject query, Handler>> resultHandler) {
delegate.find(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable> findObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture> resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
find(collection, query, resultHandler.toHandler());
return resultHandler;
}
public Single> rxFind(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
find(collection, query, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.FindOptions options, Handler>> resultHandler) {
delegate.findWithOptions(collection, query, options, resultHandler);
return this;
}
@Deprecated()
public Observable> findWithOptionsObservable(String collection, JsonObject query, io.vertx.ext.mongo.FindOptions options) {
io.vertx.rx.java.ObservableFuture> resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findWithOptions(collection, query, options, resultHandler.toHandler());
return resultHandler;
}
public Single> rxFindWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.FindOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findWithOptions(collection, query, options, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOne(String collection, JsonObject query, JsonObject fields, Handler> resultHandler) {
delegate.findOne(collection, query, fields, resultHandler);
return this;
}
@Deprecated()
public Observable findOneObservable(String collection, JsonObject query, JsonObject fields) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOne(collection, query, fields, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOne(String collection, JsonObject query, JsonObject fields) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOne(collection, query, fields, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOneAndUpdate(String collection, JsonObject query, JsonObject update, Handler> resultHandler) {
delegate.findOneAndUpdate(collection, query, update, resultHandler);
return this;
}
@Deprecated()
public Observable findOneAndUpdateObservable(String collection, JsonObject query, JsonObject update) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndUpdate(collection, query, update, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOneAndUpdate(String collection, JsonObject query, JsonObject update) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndUpdate(collection, query, update, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOneAndUpdateWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions, Handler> resultHandler) {
delegate.findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, resultHandler);
return this;
}
@Deprecated()
public Observable findOneAndUpdateWithOptionsObservable(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOneAndUpdateWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOneAndReplace(String collection, JsonObject query, JsonObject replace, Handler> resultHandler) {
delegate.findOneAndReplace(collection, query, replace, resultHandler);
return this;
}
@Deprecated()
public Observable findOneAndReplaceObservable(String collection, JsonObject query, JsonObject replace) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndReplace(collection, query, replace, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOneAndReplace(String collection, JsonObject query, JsonObject replace) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndReplace(collection, query, replace, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOneAndReplaceWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions, Handler> resultHandler) {
delegate.findOneAndReplaceWithOptions(collection, query, update, findOptions, updateOptions, resultHandler);
return this;
}
@Deprecated()
public Observable findOneAndReplaceWithOptionsObservable(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndReplaceWithOptions(collection, query, update, findOptions, updateOptions, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOneAndReplaceWithOptions(String collection, JsonObject query, JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndReplaceWithOptions(collection, query, update, findOptions, updateOptions, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOneAndDelete(String collection, JsonObject query, Handler> resultHandler) {
delegate.findOneAndDelete(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable findOneAndDeleteObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndDelete(collection, query, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOneAndDelete(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndDelete(collection, query, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService findOneAndDeleteWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.FindOptions findOptions, Handler> resultHandler) {
delegate.findOneAndDeleteWithOptions(collection, query, findOptions, resultHandler);
return this;
}
@Deprecated()
public Observable findOneAndDeleteWithOptionsObservable(String collection, JsonObject query, io.vertx.ext.mongo.FindOptions findOptions) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
findOneAndDeleteWithOptions(collection, query, findOptions, resultHandler.toHandler());
return resultHandler;
}
public Single rxFindOneAndDeleteWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.FindOptions findOptions) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
findOneAndDeleteWithOptions(collection, query, findOptions, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService count(String collection, JsonObject query, Handler> resultHandler) {
delegate.count(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable countObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
count(collection, query, resultHandler.toHandler());
return resultHandler;
}
public Single rxCount(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
count(collection, query, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService remove(String collection, JsonObject query, Handler> resultHandler) {
delegate.remove(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable removeObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
remove(collection, query, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxRemove(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
remove(collection, query, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService removeDocuments(String collection, JsonObject query, Handler> resultHandler) {
delegate.removeDocuments(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable removeDocumentsObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocuments(collection, query, resultHandler.toHandler());
return resultHandler;
}
public Single rxRemoveDocuments(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocuments(collection, query, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService removeWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption, Handler> resultHandler) {
delegate.removeWithOptions(collection, query, writeOption, resultHandler);
return this;
}
@Deprecated()
public Observable removeWithOptionsObservable(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxRemoveWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeWithOptions(collection, query, writeOption, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService removeDocumentsWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption, Handler> resultHandler) {
delegate.removeDocumentsWithOptions(collection, query, writeOption, resultHandler);
return this;
}
@Deprecated()
public Observable removeDocumentsWithOptionsObservable(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocumentsWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
public Single rxRemoveDocumentsWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocumentsWithOptions(collection, query, writeOption, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService removeOne(String collection, JsonObject query, Handler> resultHandler) {
delegate.removeOne(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable removeOneObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeOne(collection, query, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxRemoveOne(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeOne(collection, query, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService removeDocument(String collection, JsonObject query, Handler> resultHandler) {
delegate.removeDocument(collection, query, resultHandler);
return this;
}
@Deprecated()
public Observable removeDocumentObservable(String collection, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocument(collection, query, resultHandler.toHandler());
return resultHandler;
}
public Single rxRemoveDocument(String collection, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocument(collection, query, fut);
}));
}
@Deprecated()
public io.vertx.rxjava.ext.mongo.MongoService removeOneWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption, Handler> resultHandler) {
delegate.removeOneWithOptions(collection, query, writeOption, resultHandler);
return this;
}
@Deprecated()
public Observable removeOneWithOptionsObservable(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeOneWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
@Deprecated()
public Single rxRemoveOneWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeOneWithOptions(collection, query, writeOption, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService removeDocumentWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption, Handler> resultHandler) {
delegate.removeDocumentWithOptions(collection, query, writeOption, resultHandler);
return this;
}
@Deprecated()
public Observable removeDocumentWithOptionsObservable(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
removeDocumentWithOptions(collection, query, writeOption, resultHandler.toHandler());
return resultHandler;
}
public Single rxRemoveDocumentWithOptions(String collection, JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
removeDocumentWithOptions(collection, query, writeOption, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService createCollection(String collectionName, Handler> resultHandler) {
delegate.createCollection(collectionName, resultHandler);
return this;
}
@Deprecated()
public Observable createCollectionObservable(String collectionName) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
createCollection(collectionName, resultHandler.toHandler());
return resultHandler;
}
public Single rxCreateCollection(String collectionName) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
createCollection(collectionName, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService getCollections(Handler>> resultHandler) {
delegate.getCollections(resultHandler);
return this;
}
@Deprecated()
public Observable> getCollectionsObservable() {
io.vertx.rx.java.ObservableFuture> resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
getCollections(resultHandler.toHandler());
return resultHandler;
}
public Single> rxGetCollections() {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
getCollections(fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService dropCollection(String collection, Handler> resultHandler) {
delegate.dropCollection(collection, resultHandler);
return this;
}
@Deprecated()
public Observable dropCollectionObservable(String collection) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
dropCollection(collection, resultHandler.toHandler());
return resultHandler;
}
public Single rxDropCollection(String collection) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
dropCollection(collection, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService createIndex(String collection, JsonObject key, Handler> resultHandler) {
delegate.createIndex(collection, key, resultHandler);
return this;
}
@Deprecated()
public Observable createIndexObservable(String collection, JsonObject key) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
createIndex(collection, key, resultHandler.toHandler());
return resultHandler;
}
public Single rxCreateIndex(String collection, JsonObject key) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
createIndex(collection, key, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService createIndexWithOptions(String collection, JsonObject key, io.vertx.ext.mongo.IndexOptions options, Handler> resultHandler) {
delegate.createIndexWithOptions(collection, key, options, resultHandler);
return this;
}
@Deprecated()
public Observable createIndexWithOptionsObservable(String collection, JsonObject key, io.vertx.ext.mongo.IndexOptions options) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
createIndexWithOptions(collection, key, options, resultHandler.toHandler());
return resultHandler;
}
public Single rxCreateIndexWithOptions(String collection, JsonObject key, io.vertx.ext.mongo.IndexOptions options) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
createIndexWithOptions(collection, key, options, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService listIndexes(String collection, Handler> resultHandler) {
delegate.listIndexes(collection, resultHandler);
return this;
}
@Deprecated()
public Observable listIndexesObservable(String collection) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
listIndexes(collection, resultHandler.toHandler());
return resultHandler;
}
public Single rxListIndexes(String collection) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
listIndexes(collection, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService dropIndex(String collection, String indexName, Handler> resultHandler) {
delegate.dropIndex(collection, indexName, resultHandler);
return this;
}
@Deprecated()
public Observable dropIndexObservable(String collection, String indexName) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
dropIndex(collection, indexName, resultHandler.toHandler());
return resultHandler;
}
public Single rxDropIndex(String collection, String indexName) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
dropIndex(collection, indexName, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService runCommand(String commandName, JsonObject command, Handler> resultHandler) {
delegate.runCommand(commandName, command, resultHandler);
return this;
}
@Deprecated()
public Observable runCommandObservable(String commandName, JsonObject command) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
runCommand(commandName, command, resultHandler.toHandler());
return resultHandler;
}
public Single rxRunCommand(String commandName, JsonObject command) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
runCommand(commandName, command, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService distinct(String collection, String fieldName, String resultClassname, Handler> resultHandler) {
delegate.distinct(collection, fieldName, resultClassname, resultHandler);
return this;
}
@Deprecated()
public Observable distinctObservable(String collection, String fieldName, String resultClassname) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
distinct(collection, fieldName, resultClassname, resultHandler.toHandler());
return resultHandler;
}
public Single rxDistinct(String collection, String fieldName, String resultClassname) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
distinct(collection, fieldName, resultClassname, fut);
}));
}
public io.vertx.rxjava.ext.mongo.MongoService distinctWithQuery(String collection, String fieldName, String resultClassname, JsonObject query, Handler> resultHandler) {
delegate.distinctWithQuery(collection, fieldName, resultClassname, query, resultHandler);
return this;
}
@Deprecated()
public Observable distinctWithQueryObservable(String collection, String fieldName, String resultClassname, JsonObject query) {
io.vertx.rx.java.ObservableFuture resultHandler = io.vertx.rx.java.RxHelper.observableFuture();
distinctWithQuery(collection, fieldName, resultClassname, query, resultHandler.toHandler());
return resultHandler;
}
public Single rxDistinctWithQuery(String collection, String fieldName, String resultClassname, JsonObject query) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
distinctWithQuery(collection, fieldName, resultClassname, query, fut);
}));
}
public void close() {
delegate.close();
}
/**
* The name of the default pool
*/
public static final String DEFAULT_POOL_NAME = io.vertx.ext.mongo.MongoService.DEFAULT_POOL_NAME;
/**
* The name of the default database
*/
public static final String DEFAULT_DB_NAME = io.vertx.ext.mongo.MongoService.DEFAULT_DB_NAME;
public static MongoService newInstance(io.vertx.ext.mongo.MongoService arg) {
return arg != null ? new MongoService(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy