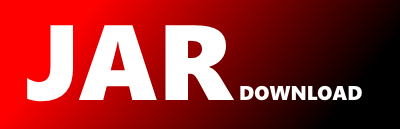
io.vertx.rxjava.core.CompositeFuture Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.core;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* The composite future wraps a list of {@link io.vertx.rxjava.core.Future futures}, it is useful when several futures
* needs to be coordinated.
* The handlers set for the coordinated futures are overridden by the handler of the composite future.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.CompositeFuture original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.CompositeFuture.class)
public class CompositeFuture extends io.vertx.rxjava.core.Future {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CompositeFuture that = (CompositeFuture) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new CompositeFuture((io.vertx.core.CompositeFuture) obj),
CompositeFuture::getDelegate
);
private final io.vertx.core.CompositeFuture delegate;
public CompositeFuture(io.vertx.core.CompositeFuture delegate) {
super(delegate);
this.delegate = delegate;
}
public CompositeFuture(Object delegate) {
super((io.vertx.core.CompositeFuture)delegate);
this.delegate = (io.vertx.core.CompositeFuture)delegate;
}
public io.vertx.core.CompositeFuture getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_3 = new TypeArg(o1 -> io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)o1), o1 -> o1.getDelegate());
/**
* The result of the operation. This will be null if the operation failed.
* @return the result or null if the operation failed.
*/
public io.vertx.rxjava.core.CompositeFuture result() {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)delegate.result());
return ret;
}
/**
* Alias for {@link io.vertx.rxjava.core.Future#compose}.
* @param mapper
* @return
*/
public io.vertx.rxjava.core.Future flatMap(Function> mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.flatMap(new Function>() {
public io.vertx.core.Future apply(io.vertx.core.CompositeFuture arg) {
io.vertx.rxjava.core.Future ret = mapper.apply(io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)arg));
return ret.getDelegate();
}
}), TypeArg.unknown());
return ret;
}
/**
* Compose this future with a mapper
function.
*
* When this future (the one on which compose
is called) succeeds, the mapper
will be called with
* the completed value and this mapper returns another future object. This returned future completion will complete
* the future returned by this method call.
*
* If the mapper
throws an exception, the returned future will be failed with this exception.
*
* When this future fails, the failure will be propagated to the returned future and the mapper
* will not be called.
* @param mapper the mapper function
* @return the composed future
*/
public io.vertx.rxjava.core.Future compose(Function> mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.compose(new Function>() {
public io.vertx.core.Future apply(io.vertx.core.CompositeFuture arg) {
io.vertx.rxjava.core.Future ret = mapper.apply(io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)arg));
return ret.getDelegate();
}
}), TypeArg.unknown());
return ret;
}
/**
* Handles a failure of this Future by returning the result of another Future.
* If the mapper fails, then the returned future will be failed with this failure.
* @param mapper A function which takes the exception of a failure and returns a new future.
* @return A recovered future
*/
public io.vertx.rxjava.core.Future recover(Function> mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.recover(new Function>() {
public io.vertx.core.Future apply(java.lang.Throwable arg) {
io.vertx.rxjava.core.Future ret = mapper.apply(arg);
return ret.getDelegate();
}
}), TYPE_ARG_0);
return ret;
}
/**
* Compose this future with a successMapper
and failureMapper
functions.
*
* When this future (the one on which compose
is called) succeeds, the successMapper
will be called with
* the completed value and this mapper returns another future object. This returned future completion will complete
* the future returned by this method call.
*
* When this future (the one on which compose
is called) fails, the failureMapper
will be called with
* the failure and this mapper returns another future object. This returned future completion will complete
* the future returned by this method call.
*
* If any mapper function throws an exception, the returned future will be failed with this exception.
* @param successMapper the function mapping the success
* @param failureMapper the function mapping the failure
* @return the composed future
*/
public io.vertx.rxjava.core.Future compose(Function> successMapper, Function> failureMapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.compose(new Function>() {
public io.vertx.core.Future apply(io.vertx.core.CompositeFuture arg) {
io.vertx.rxjava.core.Future ret = successMapper.apply(io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)arg));
return ret.getDelegate();
}
}, new Function>() {
public io.vertx.core.Future apply(java.lang.Throwable arg) {
io.vertx.rxjava.core.Future ret = failureMapper.apply(arg);
return ret.getDelegate();
}
}), TypeArg.unknown());
return ret;
}
/**
* Apply a mapper
function on this future.
*
* When this future succeeds, the mapper
will be called with the completed value and this mapper
* returns a value. This value will complete the future returned by this method call.
*
* If the mapper
throws an exception, the returned future will be failed with this exception.
*
* When this future fails, the failure will be propagated to the returned future and the mapper
* will not be called.
* @param mapper the mapper function
* @return the mapped future
*/
public io.vertx.rxjava.core.Future map(Function mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.map(new Function() {
public U apply(io.vertx.core.CompositeFuture arg) {
U ret = mapper.apply(io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)arg));
return ret;
}
}), TypeArg.unknown());
return ret;
}
/**
* Apply a mapper
function on this future.
*
* When this future fails, the mapper
will be called with the completed value and this mapper
* returns a value. This value will complete the future returned by this method call.
*
* If the mapper
throws an exception, the returned future will be failed with this exception.
*
* When this future succeeds, the result will be propagated to the returned future and the mapper
* will not be called.
* @param mapper the mapper function
* @return the mapped future
*/
public io.vertx.rxjava.core.Future otherwise(Function mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.otherwise(new Function() {
public io.vertx.core.CompositeFuture apply(java.lang.Throwable arg) {
io.vertx.rxjava.core.CompositeFuture ret = mapper.apply(arg);
return ret.getDelegate();
}
}), TYPE_ARG_1);
return ret;
}
/**
* Map the failure of a future to a specific value
.
*
* When this future fails, this value
will complete the future returned by this method call.
*
* When this future succeeds, the result will be propagated to the returned future.
* @param value the value that eventually completes the mapped future
* @return the mapped future
*/
public io.vertx.rxjava.core.Future otherwise(io.vertx.rxjava.core.CompositeFuture value) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.otherwise(value.getDelegate()), TYPE_ARG_2);
return ret;
}
/**
* Map the failure of a future to null
.
*
* This is a convenience for future.otherwise((T) null)
.
*
* When this future fails, the null
value will complete the future returned by this method call.
*
* When this future succeeds, the result will be propagated to the returned future.
* @return the mapped future
*/
public io.vertx.rxjava.core.Future otherwiseEmpty() {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.otherwiseEmpty(), TYPE_ARG_3);
return ret;
}
/**
* Return a composite future, succeeded when all futures are succeeded, failed when any future is failed.
*
* The returned future fails as soon as one of f1
or f2
fails.
* @param f1 future
* @param f2 future
* @return the composite future
*/
public static io.vertx.rxjava.core.CompositeFuture all(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.all(f1.getDelegate(), f2.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#all} but with 3 futures.
* @param f1
* @param f2
* @param f3
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture all(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.all(f1.getDelegate(), f2.getDelegate(), f3.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#all} but with 4 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture all(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.all(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#all} but with 5 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture all(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4, io.vertx.rxjava.core.Future f5) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.all(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate(), f5.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#all} but with 6 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @param f6
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture all(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4, io.vertx.rxjava.core.Future f5, io.vertx.rxjava.core.Future f6) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.all(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate(), f5.getDelegate(), f6.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#all} but with a list of futures.
*
* When the list is empty, the returned future will be already completed.
* @param futures
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture all(List futures) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.all(futures.stream().map(elt -> elt.getDelegate()).collect(Collectors.toList())));
return ret;
}
/**
* Return a composite future, succeeded when any futures is succeeded, failed when all futures are failed.
*
* The returned future succeeds as soon as one of f1
or f2
succeeds.
* @param f1 future
* @param f2 future
* @return the composite future
*/
public static io.vertx.rxjava.core.CompositeFuture any(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.any(f1.getDelegate(), f2.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#any} but with 3 futures.
* @param f1
* @param f2
* @param f3
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture any(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.any(f1.getDelegate(), f2.getDelegate(), f3.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#any} but with 4 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture any(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.any(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#any} but with 5 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture any(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4, io.vertx.rxjava.core.Future f5) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.any(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate(), f5.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#any} but with 6 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @param f6
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture any(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4, io.vertx.rxjava.core.Future f5, io.vertx.rxjava.core.Future f6) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.any(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate(), f5.getDelegate(), f6.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#any} but with a list of futures.
*
* When the list is empty, the returned future will be already completed.
* @param futures
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture any(List futures) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.any(futures.stream().map(elt -> elt.getDelegate()).collect(Collectors.toList())));
return ret;
}
/**
* Return a composite future, succeeded when all futures are succeeded, failed when any future is failed.
*
* It always wait until all its futures are completed and will not fail as soon as one of f1
or f2
fails.
* @param f1 future
* @param f2 future
* @return the composite future
*/
public static io.vertx.rxjava.core.CompositeFuture join(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.join(f1.getDelegate(), f2.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#join} but with 3 futures.
* @param f1
* @param f2
* @param f3
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture join(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.join(f1.getDelegate(), f2.getDelegate(), f3.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#join} but with 4 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture join(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.join(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#join} but with 5 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture join(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4, io.vertx.rxjava.core.Future f5) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.join(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate(), f5.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#join} but with 6 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @param f6
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture join(io.vertx.rxjava.core.Future f1, io.vertx.rxjava.core.Future f2, io.vertx.rxjava.core.Future f3, io.vertx.rxjava.core.Future f4, io.vertx.rxjava.core.Future f5, io.vertx.rxjava.core.Future f6) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.join(f1.getDelegate(), f2.getDelegate(), f3.getDelegate(), f4.getDelegate(), f5.getDelegate(), f6.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava.core.CompositeFuture#join} but with a list of futures.
*
* When the list is empty, the returned future will be already completed.
* @param futures
* @return
*/
public static io.vertx.rxjava.core.CompositeFuture join(List futures) {
io.vertx.rxjava.core.CompositeFuture ret = io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)io.vertx.core.CompositeFuture.join(futures.stream().map(elt -> elt.getDelegate()).collect(Collectors.toList())));
return ret;
}
public io.vertx.rxjava.core.CompositeFuture onComplete(Handler> handler) {
delegate.onComplete(new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
public io.vertx.rxjava.core.CompositeFuture onComplete() {
return
onComplete(ar -> { });
}
public Single rxOnComplete() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
onComplete(fut);
}));
}
public io.vertx.rxjava.core.CompositeFuture onSuccess(Handler handler) {
delegate.onSuccess(new Handler() {
public void handle(io.vertx.core.CompositeFuture event) {
handler.handle(io.vertx.rxjava.core.CompositeFuture.newInstance((io.vertx.core.CompositeFuture)event));
}
});
return this;
}
public io.vertx.rxjava.core.CompositeFuture onFailure(Handler handler) {
delegate.onFailure(handler);
return this;
}
/**
* Returns a cause of a wrapped future
* @param index the wrapped future index
* @return
*/
public java.lang.Throwable cause(int index) {
java.lang.Throwable ret = delegate.cause(index);
return ret;
}
/**
* Returns true if a wrapped future is succeeded
* @param index the wrapped future index
* @return
*/
public boolean succeeded(int index) {
boolean ret = delegate.succeeded(index);
return ret;
}
/**
* Returns true if a wrapped future is failed
* @param index the wrapped future index
* @return
*/
public boolean failed(int index) {
boolean ret = delegate.failed(index);
return ret;
}
/**
* Returns true if a wrapped future is completed
* @param index the wrapped future index
* @return
*/
public boolean isComplete(int index) {
boolean ret = delegate.isComplete(index);
return ret;
}
/**
* Returns the result of a wrapped future
* @param index the wrapped future index
* @return
*/
public T resultAt(int index) {
T ret = (T) delegate.resultAt(index);
return ret;
}
/**
* @return the number of wrapped future
*/
public int size() {
int ret = delegate.size();
return ret;
}
public static CompositeFuture newInstance(io.vertx.core.CompositeFuture arg) {
return arg != null ? new CompositeFuture(arg) : null;
}
}