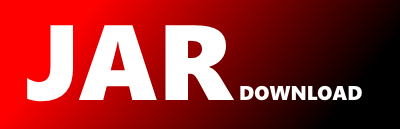
io.vertx.rxjava.core.Future Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.core;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents the result of an action that may, or may not, have occurred yet.
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.Future original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.Future.class)
public class Future {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Future that = (Future) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new Future((io.vertx.core.Future) obj),
Future::getDelegate
);
private final io.vertx.core.Future delegate;
public final TypeArg __typeArg_0;
public Future(io.vertx.core.Future delegate) {
this.delegate = delegate;
this.__typeArg_0 = TypeArg.unknown(); }
public Future(Object delegate, TypeArg typeArg_0) {
this.delegate = (io.vertx.core.Future)delegate;
this.__typeArg_0 = typeArg_0;
}
public io.vertx.core.Future getDelegate() {
return delegate;
}
/**
* Create a future that hasn't completed yet and that is passed to the handler
before it is returned.
* @param handler the handler
* @return the future.
*/
public static io.vertx.rxjava.core.Future future(Handler> handler) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)io.vertx.core.Future.future(new Handler>() {
public void handle(io.vertx.core.Promise event) {
handler.handle(io.vertx.rxjava.core.Promise.newInstance((io.vertx.core.Promise)event, TypeArg.unknown()));
}
}), TypeArg.unknown());
return ret;
}
/**
* Create a succeeded future with a null result
* @return the future
*/
public static io.vertx.rxjava.core.Future succeededFuture() {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)io.vertx.core.Future.succeededFuture(), TypeArg.unknown());
return ret;
}
/**
* Created a succeeded future with the specified result.
* @param result the result
* @return the future
*/
public static io.vertx.rxjava.core.Future succeededFuture(T result) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)io.vertx.core.Future.succeededFuture(result), TypeArg.unknown());
return ret;
}
/**
* Create a failed future with the specified failure cause.
* @param t the failure cause as a Throwable
* @return the future
*/
public static io.vertx.rxjava.core.Future failedFuture(java.lang.Throwable t) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)io.vertx.core.Future.failedFuture(t), TypeArg.unknown());
return ret;
}
/**
* Create a failed future with the specified failure message.
* @param failureMessage the failure message
* @return the future
*/
public static io.vertx.rxjava.core.Future failedFuture(String failureMessage) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)io.vertx.core.Future.failedFuture(failureMessage), TypeArg.unknown());
return ret;
}
/**
* Has the future completed?
*
* It's completed if it's either succeeded or failed.
* @return true if completed, false if not
*/
public boolean isComplete() {
boolean ret = delegate.isComplete();
return ret;
}
/**
* Add a handler to be notified of the result.
*
* @param handler the handler that will be called with the result
* @return a reference to this, so it can be used fluently
*/
public io.vertx.rxjava.core.Future onComplete(Handler> handler) {
delegate.onComplete(new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture((T)__typeArg_0.wrap(ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Add a handler to be notified of the result.
*
* @return a reference to this, so it can be used fluently
*/
public io.vertx.rxjava.core.Future onComplete() {
return
onComplete(ar -> { });
}
/**
* Add a handler to be notified of the result.
*
* @return a reference to this, so it can be used fluently
*/
public Single rxOnComplete() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
onComplete(fut);
}));
}
/**
* Add a handler to be notified of the succeeded result.
*
* @param handler the handler that will be called with the succeeded result
* @return a reference to this, so it can be used fluently
*/
public io.vertx.rxjava.core.Future onSuccess(Handler handler) {
delegate.onSuccess(new Handler() {
public void handle(T event) {
handler.handle((T)__typeArg_0.wrap(event));
}
});
return this;
}
/**
* Add a handler to be notified of the failed result.
*
* @param handler the handler that will be called with the failed result
* @return a reference to this, so it can be used fluently
*/
public io.vertx.rxjava.core.Future onFailure(Handler handler) {
delegate.onFailure(handler);
return this;
}
/**
* The result of the operation. This will be null if the operation failed.
* @return the result or null if the operation failed.
*/
public T result() {
T ret = (T)__typeArg_0.wrap(delegate.result());
return ret;
}
/**
* A Throwable describing failure. This will be null if the operation succeeded.
* @return the cause or null if the operation succeeded.
*/
public java.lang.Throwable cause() {
java.lang.Throwable ret = delegate.cause();
return ret;
}
/**
* Did it succeed?
* @return true if it succeded or false otherwise
*/
public boolean succeeded() {
boolean ret = delegate.succeeded();
return ret;
}
/**
* Did it fail?
* @return true if it failed or false otherwise
*/
public boolean failed() {
boolean ret = delegate.failed();
return ret;
}
/**
* Alias for {@link io.vertx.rxjava.core.Future#compose}.
* @param mapper
* @return
*/
public io.vertx.rxjava.core.Future flatMap(Function> mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.flatMap(new Function>() {
public io.vertx.core.Future apply(T arg) {
io.vertx.rxjava.core.Future ret = mapper.apply((T)__typeArg_0.wrap(arg));
return ret.getDelegate();
}
}), TypeArg.unknown());
return ret;
}
/**
* Compose this future with a mapper
function.
*
* When this future (the one on which compose
is called) succeeds, the mapper
will be called with
* the completed value and this mapper returns another future object. This returned future completion will complete
* the future returned by this method call.
*
* If the mapper
throws an exception, the returned future will be failed with this exception.
*
* When this future fails, the failure will be propagated to the returned future and the mapper
* will not be called.
* @param mapper the mapper function
* @return the composed future
*/
public io.vertx.rxjava.core.Future compose(Function> mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.compose(new Function>() {
public io.vertx.core.Future apply(T arg) {
io.vertx.rxjava.core.Future ret = mapper.apply((T)__typeArg_0.wrap(arg));
return ret.getDelegate();
}
}), TypeArg.unknown());
return ret;
}
/**
* Handles a failure of this Future by returning the result of another Future.
* If the mapper fails, then the returned future will be failed with this failure.
* @param mapper A function which takes the exception of a failure and returns a new future.
* @return A recovered future
*/
public io.vertx.rxjava.core.Future recover(Function> mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.recover(new Function>() {
public io.vertx.core.Future apply(java.lang.Throwable arg) {
io.vertx.rxjava.core.Future ret = mapper.apply(arg);
return ret.getDelegate();
}
}), __typeArg_0);
return ret;
}
/**
* Compose this future with a successMapper
and failureMapper
functions.
*
* When this future (the one on which compose
is called) succeeds, the successMapper
will be called with
* the completed value and this mapper returns another future object. This returned future completion will complete
* the future returned by this method call.
*
* When this future (the one on which compose
is called) fails, the failureMapper
will be called with
* the failure and this mapper returns another future object. This returned future completion will complete
* the future returned by this method call.
*
* If any mapper function throws an exception, the returned future will be failed with this exception.
* @param successMapper the function mapping the success
* @param failureMapper the function mapping the failure
* @return the composed future
*/
public io.vertx.rxjava.core.Future compose(Function> successMapper, Function> failureMapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.compose(new Function>() {
public io.vertx.core.Future apply(T arg) {
io.vertx.rxjava.core.Future ret = successMapper.apply((T)__typeArg_0.wrap(arg));
return ret.getDelegate();
}
}, new Function>() {
public io.vertx.core.Future apply(java.lang.Throwable arg) {
io.vertx.rxjava.core.Future ret = failureMapper.apply(arg);
return ret.getDelegate();
}
}), TypeArg.unknown());
return ret;
}
/**
* Apply a mapper
function on this future.
*
* When this future succeeds, the mapper
will be called with the completed value and this mapper
* returns a value. This value will complete the future returned by this method call.
*
* If the mapper
throws an exception, the returned future will be failed with this exception.
*
* When this future fails, the failure will be propagated to the returned future and the mapper
* will not be called.
* @param mapper the mapper function
* @return the mapped future
*/
public io.vertx.rxjava.core.Future map(Function mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.map(new Function() {
public U apply(T arg) {
U ret = mapper.apply((T)__typeArg_0.wrap(arg));
return ret;
}
}), TypeArg.unknown());
return ret;
}
/**
* Map the result of a future to a specific value
.
*
* When this future succeeds, this value
will complete the future returned by this method call.
*
* When this future fails, the failure will be propagated to the returned future.
* @param value the value that eventually completes the mapped future
* @return the mapped future
*/
public io.vertx.rxjava.core.Future map(V value) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.map(value), TypeArg.unknown());
return ret;
}
/**
* Map the result of a future to null
.
*
* This is a conveniency for future.map((T) null)
or future.map((Void) null)
.
*
* When this future succeeds, null
will complete the future returned by this method call.
*
* When this future fails, the failure will be propagated to the returned future.
* @return the mapped future
*/
public io.vertx.rxjava.core.Future mapEmpty() {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.mapEmpty(), TypeArg.unknown());
return ret;
}
/**
* Apply a mapper
function on this future.
*
* When this future fails, the mapper
will be called with the completed value and this mapper
* returns a value. This value will complete the future returned by this method call.
*
* If the mapper
throws an exception, the returned future will be failed with this exception.
*
* When this future succeeds, the result will be propagated to the returned future and the mapper
* will not be called.
* @param mapper the mapper function
* @return the mapped future
*/
public io.vertx.rxjava.core.Future otherwise(Function mapper) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.otherwise(new Function() {
public T apply(java.lang.Throwable arg) {
T ret = mapper.apply(arg);
return __typeArg_0.unwrap(ret);
}
}), __typeArg_0);
return ret;
}
/**
* Map the failure of a future to a specific value
.
*
* When this future fails, this value
will complete the future returned by this method call.
*
* When this future succeeds, the result will be propagated to the returned future.
* @param value the value that eventually completes the mapped future
* @return the mapped future
*/
public io.vertx.rxjava.core.Future otherwise(T value) {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.otherwise(__typeArg_0.unwrap(value)), __typeArg_0);
return ret;
}
/**
* Map the failure of a future to null
.
*
* This is a convenience for future.otherwise((T) null)
.
*
* When this future fails, the null
value will complete the future returned by this method call.
*
* When this future succeeds, the result will be propagated to the returned future.
* @return the mapped future
*/
public io.vertx.rxjava.core.Future otherwiseEmpty() {
io.vertx.rxjava.core.Future ret = io.vertx.rxjava.core.Future.newInstance((io.vertx.core.Future)delegate.otherwiseEmpty(), __typeArg_0);
return ret;
}
public static Future newInstance(io.vertx.core.Future arg) {
return arg != null ? new Future(arg) : null;
}
public static Future newInstance(io.vertx.core.Future arg, TypeArg __typeArg_T) {
return arg != null ? new Future(arg, __typeArg_T) : null;
}
}