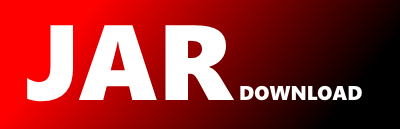
io.vertx.rxjava.core.file.FileSystem Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.core.file;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Contains a broad set of operations for manipulating files on the file system.
*
* A (potential) blocking and non blocking version of each operation is provided.
*
* The non blocking versions take a handler which is called when the operation completes or an error occurs.
*
* The blocking versions are named xxxBlocking
and return the results, or throw exceptions directly.
* In many cases, depending on the operating system and file system some of the potentially blocking operations
* can return quickly, which is why we provide them, but it's highly recommended that you test how long they take to
* return in your particular application before using them on an event loop.
*
* Please consult the documentation for more information on file system support.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.file.FileSystem original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.file.FileSystem.class)
public class FileSystem {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FileSystem that = (FileSystem) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new FileSystem((io.vertx.core.file.FileSystem) obj),
FileSystem::getDelegate
);
private final io.vertx.core.file.FileSystem delegate;
public FileSystem(io.vertx.core.file.FileSystem delegate) {
this.delegate = delegate;
}
public FileSystem(Object delegate) {
this.delegate = (io.vertx.core.file.FileSystem)delegate;
}
public io.vertx.core.file.FileSystem getDelegate() {
return delegate;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* The copy will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem copy(String from, String to, Handler> handler) {
delegate.copy(from, to, handler);
return this;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* The copy will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem copy(String from, String to) {
return
copy(from, to, ar -> { });
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* The copy will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public Single rxCopy(String from, String to) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
copy(from, to, fut);
}));
}
/**
* Copy a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem copy(String from, String to, io.vertx.core.file.CopyOptions options, Handler> handler) {
delegate.copy(from, to, options, handler);
return this;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem copy(String from, String to, io.vertx.core.file.CopyOptions options) {
return
copy(from, to, options, ar -> { });
}
/**
* Copy a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public Single rxCopy(String from, String to, io.vertx.core.file.CopyOptions options) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
copy(from, to, options, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#copy}
* @param from
* @param to
* @return
*/
public io.vertx.rxjava.core.file.FileSystem copyBlocking(String from, String to) {
delegate.copyBlocking(from, to);
return this;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* If recursive
is true
and from
represents a directory, then the directory and its contents
* will be copied recursively to the destination to
.
*
* The copy will fail if the destination if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param recursive
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem copyRecursive(String from, String to, boolean recursive, Handler> handler) {
delegate.copyRecursive(from, to, recursive, handler);
return this;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* If recursive
is true
and from
represents a directory, then the directory and its contents
* will be copied recursively to the destination to
.
*
* The copy will fail if the destination if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param recursive
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem copyRecursive(String from, String to, boolean recursive) {
return
copyRecursive(from, to, recursive, ar -> { });
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* If recursive
is true
and from
represents a directory, then the directory and its contents
* will be copied recursively to the destination to
.
*
* The copy will fail if the destination if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param recursive
* @return a reference to this, so the API can be used fluently
*/
public Single rxCopyRecursive(String from, String to, boolean recursive) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
copyRecursive(from, to, recursive, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#copyRecursive}
* @param from
* @param to
* @param recursive
* @return
*/
public io.vertx.rxjava.core.file.FileSystem copyRecursiveBlocking(String from, String to, boolean recursive) {
delegate.copyRecursiveBlocking(from, to, recursive);
return this;
}
/**
* Move a file from the path from
to path to
, asynchronously.
*
* The move will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem move(String from, String to, Handler> handler) {
delegate.move(from, to, handler);
return this;
}
/**
* Move a file from the path from
to path to
, asynchronously.
*
* The move will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem move(String from, String to) {
return
move(from, to, ar -> { });
}
/**
* Move a file from the path from
to path to
, asynchronously.
*
* The move will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public Single rxMove(String from, String to) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
move(from, to, fut);
}));
}
/**
* Move a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem move(String from, String to, io.vertx.core.file.CopyOptions options, Handler> handler) {
delegate.move(from, to, options, handler);
return this;
}
/**
* Move a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem move(String from, String to, io.vertx.core.file.CopyOptions options) {
return
move(from, to, options, ar -> { });
}
/**
* Move a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public Single rxMove(String from, String to, io.vertx.core.file.CopyOptions options) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
move(from, to, options, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#move}
* @param from
* @param to
* @return
*/
public io.vertx.rxjava.core.file.FileSystem moveBlocking(String from, String to) {
delegate.moveBlocking(from, to);
return this;
}
/**
* Truncate the file represented by path
to length len
in bytes, asynchronously.
*
* The operation will fail if the file does not exist or len
is less than zero
.
* @param path the path to the file
* @param len the length to truncate it to
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem truncate(String path, long len, Handler> handler) {
delegate.truncate(path, len, handler);
return this;
}
/**
* Truncate the file represented by path
to length len
in bytes, asynchronously.
*
* The operation will fail if the file does not exist or len
is less than zero
.
* @param path the path to the file
* @param len the length to truncate it to
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem truncate(String path, long len) {
return
truncate(path, len, ar -> { });
}
/**
* Truncate the file represented by path
to length len
in bytes, asynchronously.
*
* The operation will fail if the file does not exist or len
is less than zero
.
* @param path the path to the file
* @param len the length to truncate it to
* @return a reference to this, so the API can be used fluently
*/
public Single rxTruncate(String path, long len) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
truncate(path, len, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#truncate}
* @param path
* @param len
* @return
*/
public io.vertx.rxjava.core.file.FileSystem truncateBlocking(String path, long len) {
delegate.truncateBlocking(path, len);
return this;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
*
* The permission String takes the form rwxr-x--- as
* specified here.
* @param path the path to the file
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem chmod(String path, String perms, Handler> handler) {
delegate.chmod(path, perms, handler);
return this;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
*
* The permission String takes the form rwxr-x--- as
* specified here.
* @param path the path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem chmod(String path, String perms) {
return
chmod(path, perms, ar -> { });
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
*
* The permission String takes the form rwxr-x--- as
* specified here.
* @param path the path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxChmod(String path, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
chmod(path, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem #chmod(String, String, Handler)}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava.core.file.FileSystem chmodBlocking(String path, String perms) {
delegate.chmodBlocking(path, perms);
return this;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
* The permission String takes the form rwxr-x--- as
* specified in {here}.
*
* If the file is directory then all contents will also have their permissions changed recursively. Any directory permissions will
* be set to dirPerms
, whilst any normal file permissions will be set to perms
.
* @param path the path to the file
* @param perms the permissions string
* @param dirPerms the directory permissions
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem chmodRecursive(String path, String perms, String dirPerms, Handler> handler) {
delegate.chmodRecursive(path, perms, dirPerms, handler);
return this;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
* The permission String takes the form rwxr-x--- as
* specified in {here}.
*
* If the file is directory then all contents will also have their permissions changed recursively. Any directory permissions will
* be set to dirPerms
, whilst any normal file permissions will be set to perms
.
* @param path the path to the file
* @param perms the permissions string
* @param dirPerms the directory permissions
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem chmodRecursive(String path, String perms, String dirPerms) {
return
chmodRecursive(path, perms, dirPerms, ar -> { });
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
* The permission String takes the form rwxr-x--- as
* specified in {here}.
*
* If the file is directory then all contents will also have their permissions changed recursively. Any directory permissions will
* be set to dirPerms
, whilst any normal file permissions will be set to perms
.
* @param path the path to the file
* @param perms the permissions string
* @param dirPerms the directory permissions
* @return a reference to this, so the API can be used fluently
*/
public Single rxChmodRecursive(String path, String perms, String dirPerms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
chmodRecursive(path, perms, dirPerms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#chmodRecursive}
* @param path
* @param perms
* @param dirPerms
* @return
*/
public io.vertx.rxjava.core.file.FileSystem chmodRecursiveBlocking(String path, String perms, String dirPerms) {
delegate.chmodRecursiveBlocking(path, perms, dirPerms);
return this;
}
/**
* Change the ownership on the file represented by path
to user
and {code group}, asynchronously.
* @param path the path to the file
* @param user the user name, null
will not change the user name
* @param group the user group, null
will not change the user group name
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem chown(String path, String user, String group, Handler> handler) {
delegate.chown(path, user, group, handler);
return this;
}
/**
* Change the ownership on the file represented by path
to user
and {code group}, asynchronously.
* @param path the path to the file
* @param user the user name, null
will not change the user name
* @param group the user group, null
will not change the user group name
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem chown(String path, String user, String group) {
return
chown(path, user, group, ar -> { });
}
/**
* Change the ownership on the file represented by path
to user
and {code group}, asynchronously.
* @param path the path to the file
* @param user the user name, null
will not change the user name
* @param group the user group, null
will not change the user group name
* @return a reference to this, so the API can be used fluently
*/
public Single rxChown(String path, String user, String group) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
chown(path, user, group, fut);
}));
}
/**
* Blocking version of
*
* @param path
* @param user
* @param group
* @return
*/
public io.vertx.rxjava.core.file.FileSystem chownBlocking(String path, String user, String group) {
delegate.chownBlocking(path, user, group);
return this;
}
/**
* Obtain properties for the file represented by path
, asynchronously.
*
* If the file is a link, the link will be followed.
* @param path the path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem props(String path, Handler> handler) {
delegate.props(path, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.rxjava.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Obtain properties for the file represented by path
, asynchronously.
*
* If the file is a link, the link will be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem props(String path) {
return
props(path, ar -> { });
}
/**
* Obtain properties for the file represented by path
, asynchronously.
*
* If the file is a link, the link will be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxProps(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
props(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#props}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileProps propsBlocking(String path) {
io.vertx.rxjava.core.file.FileProps ret = io.vertx.rxjava.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)delegate.propsBlocking(path));
return ret;
}
/**
* Obtain properties for the link represented by path
, asynchronously.
*
* The link will not be followed.
* @param path the path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem lprops(String path, Handler> handler) {
delegate.lprops(path, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.rxjava.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Obtain properties for the link represented by path
, asynchronously.
*
* The link will not be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem lprops(String path) {
return
lprops(path, ar -> { });
}
/**
* Obtain properties for the link represented by path
, asynchronously.
*
* The link will not be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxLprops(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
lprops(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#lprops}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileProps lpropsBlocking(String path) {
io.vertx.rxjava.core.file.FileProps ret = io.vertx.rxjava.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)delegate.lpropsBlocking(path));
return ret;
}
/**
* Create a hard link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem link(String link, String existing, Handler> handler) {
delegate.link(link, existing, handler);
return this;
}
/**
* Create a hard link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem link(String link, String existing) {
return
link(link, existing, ar -> { });
}
/**
* Create a hard link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public Single rxLink(String link, String existing) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
link(link, existing, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#link}
* @param link
* @param existing
* @return
*/
public io.vertx.rxjava.core.file.FileSystem linkBlocking(String link, String existing) {
delegate.linkBlocking(link, existing);
return this;
}
/**
* Create a symbolic link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem symlink(String link, String existing, Handler> handler) {
delegate.symlink(link, existing, handler);
return this;
}
/**
* Create a symbolic link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem symlink(String link, String existing) {
return
symlink(link, existing, ar -> { });
}
/**
* Create a symbolic link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public Single rxSymlink(String link, String existing) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
symlink(link, existing, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#link}
* @param link
* @param existing
* @return
*/
public io.vertx.rxjava.core.file.FileSystem symlinkBlocking(String link, String existing) {
delegate.symlinkBlocking(link, existing);
return this;
}
/**
* Unlinks the link on the file system represented by the path link
, asynchronously.
* @param link the link
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem unlink(String link, Handler> handler) {
delegate.unlink(link, handler);
return this;
}
/**
* Unlinks the link on the file system represented by the path link
, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem unlink(String link) {
return
unlink(link, ar -> { });
}
/**
* Unlinks the link on the file system represented by the path link
, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public Single rxUnlink(String link) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
unlink(link, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#unlink}
* @param link
* @return
*/
public io.vertx.rxjava.core.file.FileSystem unlinkBlocking(String link) {
delegate.unlinkBlocking(link);
return this;
}
/**
* Returns the path representing the file that the symbolic link specified by link
points to, asynchronously.
* @param link the link
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readSymlink(String link, Handler> handler) {
delegate.readSymlink(link, handler);
return this;
}
/**
* Returns the path representing the file that the symbolic link specified by link
points to, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readSymlink(String link) {
return
readSymlink(link, ar -> { });
}
/**
* Returns the path representing the file that the symbolic link specified by link
points to, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public Single rxReadSymlink(String link) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
readSymlink(link, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#readSymlink}
* @param link
* @return
*/
public String readSymlinkBlocking(String link) {
String ret = delegate.readSymlinkBlocking(link);
return ret;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem delete(String path, Handler> handler) {
delegate.delete(path, handler);
return this;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem delete(String path) {
return
delete(path, ar -> { });
}
/**
* Deletes the file represented by the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxDelete(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
delete(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#delete}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileSystem deleteBlocking(String path) {
delegate.deleteBlocking(path);
return this;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
*
* If the path represents a directory and recursive = true
then the directory and its contents will be
* deleted recursively.
* @param path path to the file
* @param recursive delete recursively?
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem deleteRecursive(String path, boolean recursive, Handler> handler) {
delegate.deleteRecursive(path, recursive, handler);
return this;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
*
* If the path represents a directory and recursive = true
then the directory and its contents will be
* deleted recursively.
* @param path path to the file
* @param recursive delete recursively?
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem deleteRecursive(String path, boolean recursive) {
return
deleteRecursive(path, recursive, ar -> { });
}
/**
* Deletes the file represented by the specified path
, asynchronously.
*
* If the path represents a directory and recursive = true
then the directory and its contents will be
* deleted recursively.
* @param path path to the file
* @param recursive delete recursively?
* @return a reference to this, so the API can be used fluently
*/
public Single rxDeleteRecursive(String path, boolean recursive) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
deleteRecursive(path, recursive, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#deleteRecursive}
* @param path
* @param recursive
* @return
*/
public io.vertx.rxjava.core.file.FileSystem deleteRecursiveBlocking(String path, boolean recursive) {
delegate.deleteRecursiveBlocking(path, recursive);
return this;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdir(String path, Handler> handler) {
delegate.mkdir(path, handler);
return this;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdir(String path) {
return
mkdir(path, ar -> { });
}
/**
* Create the directory represented by path
, asynchronously.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxMkdir(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
mkdir(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#mkdir}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileSystem mkdirBlocking(String path) {
delegate.mkdirBlocking(path);
return this;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdir(String path, String perms, Handler> handler) {
delegate.mkdir(path, perms, handler);
return this;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdir(String path, String perms) {
return
mkdir(path, perms, ar -> { });
}
/**
* Create the directory represented by path
, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxMkdir(String path, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
mkdir(path, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#mkdir}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava.core.file.FileSystem mkdirBlocking(String path, String perms) {
delegate.mkdirBlocking(path, perms);
return this;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdirs(String path, Handler> handler) {
delegate.mkdirs(path, handler);
return this;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdirs(String path) {
return
mkdirs(path, ar -> { });
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxMkdirs(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
mkdirs(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#mkdirs}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileSystem mkdirsBlocking(String path) {
delegate.mkdirsBlocking(path);
return this;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdirs(String path, String perms, Handler> handler) {
delegate.mkdirs(path, perms, handler);
return this;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem mkdirs(String path, String perms) {
return
mkdirs(path, perms, ar -> { });
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxMkdirs(String path, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
mkdirs(path, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#mkdirs}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava.core.file.FileSystem mkdirsBlocking(String path, String perms) {
delegate.mkdirsBlocking(path, perms);
return this;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readDir(String path, Handler>> handler) {
delegate.readDir(path, handler);
return this;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readDir(String path) {
return
readDir(path, ar -> { });
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single> rxReadDir(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
readDir(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#readDir}
* @param path
* @return
*/
public List readDirBlocking(String path) {
List ret = delegate.readDirBlocking(path);
return ret;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The parameter filter
is a regular expression. If filter
is specified then only the paths that
* match @{filter}will be returned.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the directory
* @param filter the filter expression
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readDir(String path, String filter, Handler>> handler) {
delegate.readDir(path, filter, handler);
return this;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The parameter filter
is a regular expression. If filter
is specified then only the paths that
* match @{filter}will be returned.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the directory
* @param filter the filter expression
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readDir(String path, String filter) {
return
readDir(path, filter, ar -> { });
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The parameter filter
is a regular expression. If filter
is specified then only the paths that
* match @{filter}will be returned.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the directory
* @param filter the filter expression
* @return a reference to this, so the API can be used fluently
*/
public Single> rxReadDir(String path, String filter) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
readDir(path, filter, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#readDir}
* @param path
* @param filter
* @return
*/
public List readDirBlocking(String path, String filter) {
List ret = delegate.readDirBlocking(path, filter);
return ret;
}
/**
* Reads the entire file as represented by the path path
as a , asynchronously.
*
* Do not use this method to read very large files or you risk running out of available RAM.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readFile(String path, Handler> handler) {
delegate.readFile(path, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Reads the entire file as represented by the path path
as a , asynchronously.
*
* Do not use this method to read very large files or you risk running out of available RAM.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem readFile(String path) {
return
readFile(path, ar -> { });
}
/**
* Reads the entire file as represented by the path path
as a , asynchronously.
*
* Do not use this method to read very large files or you risk running out of available RAM.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxReadFile(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
readFile(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#readFile}
* @param path
* @return
*/
public io.vertx.rxjava.core.buffer.Buffer readFileBlocking(String path) {
io.vertx.rxjava.core.buffer.Buffer ret = io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.readFileBlocking(path));
return ret;
}
/**
* Creates the file, and writes the specified Buffer data
to the file represented by the path path
,
* asynchronously.
* @param path path to the file
* @param data
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem writeFile(String path, io.vertx.rxjava.core.buffer.Buffer data, Handler> handler) {
delegate.writeFile(path, data.getDelegate(), handler);
return this;
}
/**
* Creates the file, and writes the specified Buffer data
to the file represented by the path path
,
* asynchronously.
* @param path path to the file
* @param data
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem writeFile(String path, io.vertx.rxjava.core.buffer.Buffer data) {
return
writeFile(path, data, ar -> { });
}
/**
* Creates the file, and writes the specified Buffer data
to the file represented by the path path
,
* asynchronously.
* @param path path to the file
* @param data
* @return a reference to this, so the API can be used fluently
*/
public Single rxWriteFile(String path, io.vertx.rxjava.core.buffer.Buffer data) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
writeFile(path, data, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#writeFile}
* @param path
* @param data
* @return
*/
public io.vertx.rxjava.core.file.FileSystem writeFileBlocking(String path, io.vertx.rxjava.core.buffer.Buffer data) {
delegate.writeFileBlocking(path, data.getDelegate());
return this;
}
/**
* Open the file represented by path
, asynchronously.
*
* The file is opened for both reading and writing. If the file does not already exist it will be created.
* @param path path to the file
* @param options options describing how the file should be opened
* @param handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem open(String path, io.vertx.core.file.OpenOptions options, Handler> handler) {
delegate.open(path, options, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.rxjava.core.file.AsyncFile.newInstance((io.vertx.core.file.AsyncFile)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Open the file represented by path
, asynchronously.
*
* The file is opened for both reading and writing. If the file does not already exist it will be created.
* @param path path to the file
* @param options options describing how the file should be opened
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem open(String path, io.vertx.core.file.OpenOptions options) {
return
open(path, options, ar -> { });
}
/**
* Open the file represented by path
, asynchronously.
*
* The file is opened for both reading and writing. If the file does not already exist it will be created.
* @param path path to the file
* @param options options describing how the file should be opened
* @return a reference to this, so the API can be used fluently
*/
public Single rxOpen(String path, io.vertx.core.file.OpenOptions options) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
open(path, options, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#open}
* @param path
* @param options
* @return
*/
public io.vertx.rxjava.core.file.AsyncFile openBlocking(String path, io.vertx.core.file.OpenOptions options) {
io.vertx.rxjava.core.file.AsyncFile ret = io.vertx.rxjava.core.file.AsyncFile.newInstance((io.vertx.core.file.AsyncFile)delegate.openBlocking(path, options));
return ret;
}
/**
* Creates an empty file with the specified path
, asynchronously.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createFile(String path, Handler> handler) {
delegate.createFile(path, handler);
return this;
}
/**
* Creates an empty file with the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createFile(String path) {
return
createFile(path, ar -> { });
}
/**
* Creates an empty file with the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateFile(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createFile(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createFile}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileSystem createFileBlocking(String path) {
delegate.createFileBlocking(path);
return this;
}
/**
* Creates an empty file with the specified path
and permissions perms
, asynchronously.
* @param path path to the file
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createFile(String path, String perms, Handler> handler) {
delegate.createFile(path, perms, handler);
return this;
}
/**
* Creates an empty file with the specified path
and permissions perms
, asynchronously.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createFile(String path, String perms) {
return
createFile(path, perms, ar -> { });
}
/**
* Creates an empty file with the specified path
and permissions perms
, asynchronously.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateFile(String path, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createFile(path, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createFile}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava.core.file.FileSystem createFileBlocking(String path, String perms) {
delegate.createFileBlocking(path, perms);
return this;
}
/**
* Determines whether the file as specified by the path path
exists, asynchronously.
* @param path path to the file
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem exists(String path, Handler> handler) {
delegate.exists(path, handler);
return this;
}
/**
* Determines whether the file as specified by the path path
exists, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem exists(String path) {
return
exists(path, ar -> { });
}
/**
* Determines whether the file as specified by the path path
exists, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public Single rxExists(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
exists(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#exists}
* @param path
* @return
*/
public boolean existsBlocking(String path) {
boolean ret = delegate.existsBlocking(path);
return ret;
}
/**
* Returns properties of the file-system being used by the specified path
, asynchronously.
* @param path path to anywhere on the filesystem
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem fsProps(String path, Handler> handler) {
delegate.fsProps(path, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.rxjava.core.file.FileSystemProps.newInstance((io.vertx.core.file.FileSystemProps)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
return this;
}
/**
* Returns properties of the file-system being used by the specified path
, asynchronously.
* @param path path to anywhere on the filesystem
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem fsProps(String path) {
return
fsProps(path, ar -> { });
}
/**
* Returns properties of the file-system being used by the specified path
, asynchronously.
* @param path path to anywhere on the filesystem
* @return a reference to this, so the API can be used fluently
*/
public Single rxFsProps(String path) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
fsProps(path, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#fsProps}
* @param path
* @return
*/
public io.vertx.rxjava.core.file.FileSystemProps fsPropsBlocking(String path) {
io.vertx.rxjava.core.file.FileSystemProps ret = io.vertx.rxjava.core.file.FileSystemProps.newInstance((io.vertx.core.file.FileSystemProps)delegate.fsPropsBlocking(path));
return ret;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempDirectory(String prefix, Handler> handler) {
delegate.createTempDirectory(prefix, handler);
return this;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempDirectory(String prefix) {
return
createTempDirectory(prefix, ar -> { });
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateTempDirectory(String prefix) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createTempDirectory(prefix, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createTempDirectory}
* @param prefix
* @return
*/
public String createTempDirectoryBlocking(String prefix) {
String ret = delegate.createTempDirectoryBlocking(prefix);
return ret;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempDirectory(String prefix, String perms, Handler> handler) {
delegate.createTempDirectory(prefix, perms, handler);
return this;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempDirectory(String prefix, String perms) {
return
createTempDirectory(prefix, perms, ar -> { });
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateTempDirectory(String prefix, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createTempDirectory(prefix, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createTempDirectory}
* @param prefix
* @param perms
* @return
*/
public String createTempDirectoryBlocking(String prefix, String perms) {
String ret = delegate.createTempDirectoryBlocking(prefix, perms);
return ret;
}
/**
* Creates a new directory in the directory provided by the path path
, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempDirectory(String dir, String prefix, String perms, Handler> handler) {
delegate.createTempDirectory(dir, prefix, perms, handler);
return this;
}
/**
* Creates a new directory in the directory provided by the path path
, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempDirectory(String dir, String prefix, String perms) {
return
createTempDirectory(dir, prefix, perms, ar -> { });
}
/**
* Creates a new directory in the directory provided by the path path
, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateTempDirectory(String dir, String prefix, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createTempDirectory(dir, prefix, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createTempDirectory}
* @param dir
* @param prefix
* @param perms
* @return
*/
public String createTempDirectoryBlocking(String dir, String prefix, String perms) {
String ret = delegate.createTempDirectoryBlocking(dir, prefix, perms);
return ret;
}
/**
* Creates a new file in the default temporary-file directory, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempFile(String prefix, String suffix, Handler> handler) {
delegate.createTempFile(prefix, suffix, handler);
return this;
}
/**
* Creates a new file in the default temporary-file directory, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempFile(String prefix, String suffix) {
return
createTempFile(prefix, suffix, ar -> { });
}
/**
* Creates a new file in the default temporary-file directory, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateTempFile(String prefix, String suffix) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createTempFile(prefix, suffix, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createTempFile}
* @param prefix
* @param suffix
* @return
*/
public String createTempFileBlocking(String prefix, String suffix) {
String ret = delegate.createTempFileBlocking(prefix, suffix);
return ret;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempFile(String prefix, String suffix, String perms, Handler> handler) {
delegate.createTempFile(prefix, suffix, perms, handler);
return this;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempFile(String prefix, String suffix, String perms) {
return
createTempFile(prefix, suffix, perms, ar -> { });
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateTempFile(String prefix, String suffix, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createTempFile(prefix, suffix, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createTempFile}
* @param prefix
* @param suffix
* @param perms
* @return
*/
public String createTempFileBlocking(String prefix, String suffix, String perms) {
String ret = delegate.createTempFileBlocking(prefix, suffix, perms);
return ret;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms the permissions string
* @param handler the handler that will be called on completion
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempFile(String dir, String prefix, String suffix, String perms, Handler> handler) {
delegate.createTempFile(dir, prefix, suffix, perms, handler);
return this;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.FileSystem createTempFile(String dir, String prefix, String suffix, String perms) {
return
createTempFile(dir, prefix, suffix, perms, ar -> { });
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public Single rxCreateTempFile(String dir, String prefix, String suffix, String perms) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
createTempFile(dir, prefix, suffix, perms, fut);
}));
}
/**
* Blocking version of {@link io.vertx.rxjava.core.file.FileSystem#createTempFile}
* @param dir
* @param prefix
* @param suffix
* @param perms
* @return
*/
public String createTempFileBlocking(String dir, String prefix, String suffix, String perms) {
String ret = delegate.createTempFileBlocking(dir, prefix, suffix, perms);
return ret;
}
public static FileSystem newInstance(io.vertx.core.file.FileSystem arg) {
return arg != null ? new FileSystem(arg) : null;
}
}