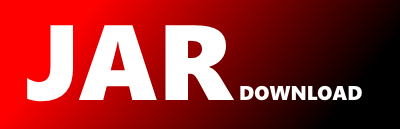
io.vertx.rxjava.core.http.Cookie Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.core.http;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents an HTTP Cookie.
*
* All cookies must have a name and a value and can optionally have other fields set such as path, domain, etc.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.Cookie original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.Cookie.class)
public class Cookie {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Cookie that = (Cookie) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new Cookie((io.vertx.core.http.Cookie) obj),
Cookie::getDelegate
);
private final io.vertx.core.http.Cookie delegate;
public Cookie(io.vertx.core.http.Cookie delegate) {
this.delegate = delegate;
}
public Cookie(Object delegate) {
this.delegate = (io.vertx.core.http.Cookie)delegate;
}
public io.vertx.core.http.Cookie getDelegate() {
return delegate;
}
/**
* Create a new cookie
* @param name the name of the cookie
* @param value the cookie value
* @return the cookie
*/
public static io.vertx.rxjava.core.http.Cookie cookie(String name, String value) {
io.vertx.rxjava.core.http.Cookie ret = io.vertx.rxjava.core.http.Cookie.newInstance((io.vertx.core.http.Cookie)io.vertx.core.http.Cookie.cookie(name, value));
return ret;
}
/**
* @return the name of this cookie
*/
public String getName() {
String ret = delegate.getName();
return ret;
}
/**
* @return the value of this cookie
*/
public String getValue() {
String ret = delegate.getValue();
return ret;
}
/**
* Sets the value of this cookie
* @param value The value to set
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.http.Cookie setValue(String value) {
delegate.setValue(value);
return this;
}
/**
* Sets the domain of this cookie
* @param domain The domain to use
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.http.Cookie setDomain(String domain) {
delegate.setDomain(domain);
return this;
}
/**
* @return the domain for the cookie
*/
public String getDomain() {
String ret = delegate.getDomain();
return ret;
}
/**
* Sets the path of this cookie.
* @param path The path to use for this cookie
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.http.Cookie setPath(String path) {
delegate.setPath(path);
return this;
}
/**
* @return the path for this cookie
*/
public String getPath() {
String ret = delegate.getPath();
return ret;
}
/**
* Sets the maximum age of this cookie in seconds.
* If an age of 0
is specified, this cookie will be
* automatically removed by browser because it will expire immediately.
* If {@link java.lang.Long} is specified, this cookie will be removed when the
* browser is closed.
* If you don't set this the cookie will be a session cookie and be removed when the browser is closed.
* @param maxAge The maximum age of this cookie in seconds
* @return
*/
public io.vertx.rxjava.core.http.Cookie setMaxAge(long maxAge) {
delegate.setMaxAge(maxAge);
return this;
}
/**
* Sets the security getStatus of this cookie
* @param secure True if this cookie is to be secure, otherwise false
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.http.Cookie setSecure(boolean secure) {
delegate.setSecure(secure);
return this;
}
/**
* @return the security status of this cookie
*/
public boolean isSecure() {
boolean ret = delegate.isSecure();
return ret;
}
/**
* Determines if this cookie is HTTP only.
* If set to true, this cookie cannot be accessed by a client
* side script. However, this works only if the browser supports it.
* For for information, please look
* here.
* @param httpOnly True if the cookie is HTTP only, otherwise false.
* @return
*/
public io.vertx.rxjava.core.http.Cookie setHttpOnly(boolean httpOnly) {
delegate.setHttpOnly(httpOnly);
return this;
}
/**
* @return the http only status of this cookie
*/
public boolean isHttpOnly() {
boolean ret = delegate.isHttpOnly();
return ret;
}
/**
* Sets the same site of this cookie.
* @param policy The policy should be one of {@link io.vertx.core.http.CookieSameSite}.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.http.Cookie setSameSite(io.vertx.core.http.CookieSameSite policy) {
delegate.setSameSite(policy);
return this;
}
/**
* @return the SameSite policy of this cookie
*/
public io.vertx.core.http.CookieSameSite getSameSite() {
io.vertx.core.http.CookieSameSite ret = delegate.getSameSite();
return ret;
}
/**
* Encode the cookie to a string. This is what is used in the Set-Cookie header
* @return the encoded cookie
*/
public String encode() {
String ret = delegate.encode();
return ret;
}
public static Cookie newInstance(io.vertx.core.http.Cookie arg) {
return arg != null ? new Cookie(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy