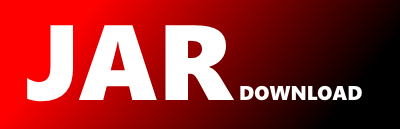
io.vertx.rxjava.core.http.HttpHeaders Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.core.http;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Contains a bunch of useful HTTP headers stuff:
*
*
* - methods for creating instances
* - often used Header names
* - method to create optimized {@link java.lang.CharSequence} which can be used as header name and value
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.HttpHeaders original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.HttpHeaders.class)
public class HttpHeaders {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpHeaders that = (HttpHeaders) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpHeaders((io.vertx.core.http.HttpHeaders) obj),
HttpHeaders::getDelegate
);
private final io.vertx.core.http.HttpHeaders delegate;
public HttpHeaders(io.vertx.core.http.HttpHeaders delegate) {
this.delegate = delegate;
}
public HttpHeaders(Object delegate) {
this.delegate = (io.vertx.core.http.HttpHeaders)delegate;
}
public io.vertx.core.http.HttpHeaders getDelegate() {
return delegate;
}
public static io.vertx.rxjava.core.MultiMap headers() {
io.vertx.rxjava.core.MultiMap ret = io.vertx.rxjava.core.MultiMap.newInstance((io.vertx.core.MultiMap)io.vertx.core.http.HttpHeaders.headers());
return ret;
}
public static io.vertx.rxjava.core.MultiMap set(String name, String value) {
io.vertx.rxjava.core.MultiMap ret = io.vertx.rxjava.core.MultiMap.newInstance((io.vertx.core.MultiMap)io.vertx.core.http.HttpHeaders.set(name, value));
return ret;
}
/**
* Create an optimized {@link java.lang.CharSequence} which can be used as header name or value.
* This should be used if you expect to use it multiple times liked for example adding the same header name or value
* for multiple responses or requests.
* @param value
* @return
*/
public static java.lang.CharSequence createOptimized(String value) {
java.lang.CharSequence ret = io.vertx.core.http.HttpHeaders.createOptimized(value);
return ret;
}
public static io.vertx.rxjava.core.MultiMap set(java.lang.CharSequence name, java.lang.CharSequence value) {
io.vertx.rxjava.core.MultiMap ret = io.vertx.rxjava.core.MultiMap.newInstance((io.vertx.core.MultiMap)io.vertx.core.http.HttpHeaders.set(name, value));
return ret;
}
/**
*JVM system property that disables HTTP headers validation, don't use this in production.
*/
public static final String DISABLE_HTTP_HEADERS_VALIDATION_PROP_NAME = io.vertx.core.http.HttpHeaders.DISABLE_HTTP_HEADERS_VALIDATION_PROP_NAME;
/**
*Constant that disables HTTP headers validation, this is a constant so the JIT can eliminate validation code.
*/
public static final boolean DISABLE_HTTP_HEADERS_VALIDATION = io.vertx.core.http.HttpHeaders.DISABLE_HTTP_HEADERS_VALIDATION;
/**
* Accept header name
*/
public static final java.lang.CharSequence ACCEPT = io.vertx.core.http.HttpHeaders.ACCEPT;
/**
* Accept-Charset header name
*/
public static final java.lang.CharSequence ACCEPT_CHARSET = io.vertx.core.http.HttpHeaders.ACCEPT_CHARSET;
/**
* Accept-Encoding header name
*/
public static final java.lang.CharSequence ACCEPT_ENCODING = io.vertx.core.http.HttpHeaders.ACCEPT_ENCODING;
/**
* Accept-Language header name
*/
public static final java.lang.CharSequence ACCEPT_LANGUAGE = io.vertx.core.http.HttpHeaders.ACCEPT_LANGUAGE;
/**
* Accept-Ranges header name
*/
public static final java.lang.CharSequence ACCEPT_RANGES = io.vertx.core.http.HttpHeaders.ACCEPT_RANGES;
/**
* Accept-Patch header name
*/
public static final java.lang.CharSequence ACCEPT_PATCH = io.vertx.core.http.HttpHeaders.ACCEPT_PATCH;
/**
* Access-Control-Allow-Credentials header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_ALLOW_CREDENTIALS = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_ALLOW_CREDENTIALS;
/**
* Access-Control-Allow-Headers header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_ALLOW_HEADERS = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_ALLOW_HEADERS;
/**
* Access-Control-Allow-Methods header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_ALLOW_METHODS = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_ALLOW_METHODS;
/**
* Access-Control-Allow-Origin header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_ALLOW_ORIGIN = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_ALLOW_ORIGIN;
/**
* Access-Control-Expose-Headers header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_EXPOSE_HEADERS = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_EXPOSE_HEADERS;
/**
* Access-Control-Max-Age header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_MAX_AGE = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_MAX_AGE;
/**
* Access-Control-Request-Headers header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_REQUEST_HEADERS = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_REQUEST_HEADERS;
/**
* Access-Control-Request-Method header name
*/
public static final java.lang.CharSequence ACCESS_CONTROL_REQUEST_METHOD = io.vertx.core.http.HttpHeaders.ACCESS_CONTROL_REQUEST_METHOD;
/**
* Age header name
*/
public static final java.lang.CharSequence AGE = io.vertx.core.http.HttpHeaders.AGE;
/**
* Allow header name
*/
public static final java.lang.CharSequence ALLOW = io.vertx.core.http.HttpHeaders.ALLOW;
/**
* Authorization header name
*/
public static final java.lang.CharSequence AUTHORIZATION = io.vertx.core.http.HttpHeaders.AUTHORIZATION;
/**
* Cache-Control header name
*/
public static final java.lang.CharSequence CACHE_CONTROL = io.vertx.core.http.HttpHeaders.CACHE_CONTROL;
/**
* Connection header name
*/
public static final java.lang.CharSequence CONNECTION = io.vertx.core.http.HttpHeaders.CONNECTION;
/**
* Content-Base header name
*/
public static final java.lang.CharSequence CONTENT_BASE = io.vertx.core.http.HttpHeaders.CONTENT_BASE;
/**
* Content-Disposition header name
*/
public static final java.lang.CharSequence CONTENT_DISPOSITION = io.vertx.core.http.HttpHeaders.CONTENT_DISPOSITION;
/**
* Content-Encoding header name
*/
public static final java.lang.CharSequence CONTENT_ENCODING = io.vertx.core.http.HttpHeaders.CONTENT_ENCODING;
/**
* Content-Language header name
*/
public static final java.lang.CharSequence CONTENT_LANGUAGE = io.vertx.core.http.HttpHeaders.CONTENT_LANGUAGE;
/**
* Content-Length header name
*/
public static final java.lang.CharSequence CONTENT_LENGTH = io.vertx.core.http.HttpHeaders.CONTENT_LENGTH;
/**
* Content-Location header name
*/
public static final java.lang.CharSequence CONTENT_LOCATION = io.vertx.core.http.HttpHeaders.CONTENT_LOCATION;
/**
* Content-Transfer-Encoding header name
*/
public static final java.lang.CharSequence CONTENT_TRANSFER_ENCODING = io.vertx.core.http.HttpHeaders.CONTENT_TRANSFER_ENCODING;
/**
* Content-MD5 header name
*/
public static final java.lang.CharSequence CONTENT_MD5 = io.vertx.core.http.HttpHeaders.CONTENT_MD5;
/**
* Content-Rage header name
*/
public static final java.lang.CharSequence CONTENT_RANGE = io.vertx.core.http.HttpHeaders.CONTENT_RANGE;
/**
* Content-Type header name
*/
public static final java.lang.CharSequence CONTENT_TYPE = io.vertx.core.http.HttpHeaders.CONTENT_TYPE;
/**
* Content-Cookie header name
*/
public static final java.lang.CharSequence COOKIE = io.vertx.core.http.HttpHeaders.COOKIE;
/**
* Date header name
*/
public static final java.lang.CharSequence DATE = io.vertx.core.http.HttpHeaders.DATE;
/**
* Etag header name
*/
public static final java.lang.CharSequence ETAG = io.vertx.core.http.HttpHeaders.ETAG;
/**
* Expect header name
*/
public static final java.lang.CharSequence EXPECT = io.vertx.core.http.HttpHeaders.EXPECT;
/**
* Expires header name
*/
public static final java.lang.CharSequence EXPIRES = io.vertx.core.http.HttpHeaders.EXPIRES;
/**
* From header name
*/
public static final java.lang.CharSequence FROM = io.vertx.core.http.HttpHeaders.FROM;
/**
* Host header name
*/
public static final java.lang.CharSequence HOST = io.vertx.core.http.HttpHeaders.HOST;
/**
* If-Match header name
*/
public static final java.lang.CharSequence IF_MATCH = io.vertx.core.http.HttpHeaders.IF_MATCH;
/**
* If-Modified-Since header name
*/
public static final java.lang.CharSequence IF_MODIFIED_SINCE = io.vertx.core.http.HttpHeaders.IF_MODIFIED_SINCE;
/**
* If-None-Match header name
*/
public static final java.lang.CharSequence IF_NONE_MATCH = io.vertx.core.http.HttpHeaders.IF_NONE_MATCH;
/**
* Last-Modified header name
*/
public static final java.lang.CharSequence LAST_MODIFIED = io.vertx.core.http.HttpHeaders.LAST_MODIFIED;
/**
* Location header name
*/
public static final java.lang.CharSequence LOCATION = io.vertx.core.http.HttpHeaders.LOCATION;
/**
* Origin header name
*/
public static final java.lang.CharSequence ORIGIN = io.vertx.core.http.HttpHeaders.ORIGIN;
/**
* Proxy-Authenticate header name
*/
public static final java.lang.CharSequence PROXY_AUTHENTICATE = io.vertx.core.http.HttpHeaders.PROXY_AUTHENTICATE;
/**
* Proxy-Authorization header name
*/
public static final java.lang.CharSequence PROXY_AUTHORIZATION = io.vertx.core.http.HttpHeaders.PROXY_AUTHORIZATION;
/**
* Referer header name
*/
public static final java.lang.CharSequence REFERER = io.vertx.core.http.HttpHeaders.REFERER;
/**
* Retry-After header name
*/
public static final java.lang.CharSequence RETRY_AFTER = io.vertx.core.http.HttpHeaders.RETRY_AFTER;
/**
* Server header name
*/
public static final java.lang.CharSequence SERVER = io.vertx.core.http.HttpHeaders.SERVER;
/**
* Transfer-Encoding header name
*/
public static final java.lang.CharSequence TRANSFER_ENCODING = io.vertx.core.http.HttpHeaders.TRANSFER_ENCODING;
/**
* User-Agent header name
*/
public static final java.lang.CharSequence USER_AGENT = io.vertx.core.http.HttpHeaders.USER_AGENT;
/**
* Set-Cookie header name
*/
public static final java.lang.CharSequence SET_COOKIE = io.vertx.core.http.HttpHeaders.SET_COOKIE;
/**
* application/x-www-form-urlencoded header value
*/
public static final java.lang.CharSequence APPLICATION_X_WWW_FORM_URLENCODED = io.vertx.core.http.HttpHeaders.APPLICATION_X_WWW_FORM_URLENCODED;
/**
* chunked header value
*/
public static final java.lang.CharSequence CHUNKED = io.vertx.core.http.HttpHeaders.CHUNKED;
/**
* close header value
*/
public static final java.lang.CharSequence CLOSE = io.vertx.core.http.HttpHeaders.CLOSE;
/**
* 100-continue header value
*/
public static final java.lang.CharSequence CONTINUE = io.vertx.core.http.HttpHeaders.CONTINUE;
/**
* identity header value
*/
public static final java.lang.CharSequence IDENTITY = io.vertx.core.http.HttpHeaders.IDENTITY;
/**
* keep-alive header value
*/
public static final java.lang.CharSequence KEEP_ALIVE = io.vertx.core.http.HttpHeaders.KEEP_ALIVE;
/**
* Upgrade header value
*/
public static final java.lang.CharSequence UPGRADE = io.vertx.core.http.HttpHeaders.UPGRADE;
/**
* WebSocket header value
*/
public static final java.lang.CharSequence WEBSOCKET = io.vertx.core.http.HttpHeaders.WEBSOCKET;
/**
* deflate,gzip header value
*/
public static final java.lang.CharSequence DEFLATE_GZIP = io.vertx.core.http.HttpHeaders.DEFLATE_GZIP;
/**
* text/html header value
*/
public static final java.lang.CharSequence TEXT_HTML = io.vertx.core.http.HttpHeaders.TEXT_HTML;
/**
* GET header value
*/
public static final java.lang.CharSequence GET = io.vertx.core.http.HttpHeaders.GET;
/**
* Vary header name
*/
public static final java.lang.CharSequence VARY = io.vertx.core.http.HttpHeaders.VARY;
public static HttpHeaders newInstance(io.vertx.core.http.HttpHeaders arg) {
return arg != null ? new HttpHeaders(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy