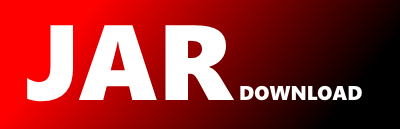
io.vertx.rxjava.ext.auth.User Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.auth;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents an authenticates User and contains operations to authorise the user.
*
* Please consult the documentation for a detailed explanation.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.User original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.auth.User.class)
public class User {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
User that = (User) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new User((io.vertx.ext.auth.User) obj),
User::getDelegate
);
private final io.vertx.ext.auth.User delegate;
public User(io.vertx.ext.auth.User delegate) {
this.delegate = delegate;
}
public User(Object delegate) {
this.delegate = (io.vertx.ext.auth.User)delegate;
}
public io.vertx.ext.auth.User getDelegate() {
return delegate;
}
/**
* Factory for user instances that are single string. The credentials will be added to the principal
* of this instance. As nothing can be said about the credentials no validation will be done.
*
* Will create a principal with a property "username"
with the name as value.
* @param username the value for this user
* @return user instance
*/
public static io.vertx.rxjava.ext.auth.User fromName(String username) {
io.vertx.rxjava.ext.auth.User ret = io.vertx.rxjava.ext.auth.User.newInstance((io.vertx.ext.auth.User)io.vertx.ext.auth.User.fromName(username));
return ret;
}
/**
* Factory for user instances that are single string. The credentials will be added to the principal
* of this instance. As nothing can be said about the credentials no validation will be done.
*
* Will create a principal with a property "access_token"
with the name as value.
* @param token the value for this user
* @return user instance
*/
public static io.vertx.rxjava.ext.auth.User fromToken(String token) {
io.vertx.rxjava.ext.auth.User ret = io.vertx.rxjava.ext.auth.User.newInstance((io.vertx.ext.auth.User)io.vertx.ext.auth.User.fromToken(token));
return ret;
}
/**
* Factory for user instances that are free form. The credentials will be added to the principal
* of this instance. As nothing can be said about the credentials no validation will be done.
* @param principal the free form json principal
* @return user instance
*/
public static io.vertx.rxjava.ext.auth.User create(JsonObject principal) {
io.vertx.rxjava.ext.auth.User ret = io.vertx.rxjava.ext.auth.User.newInstance((io.vertx.ext.auth.User)io.vertx.ext.auth.User.create(principal));
return ret;
}
/**
* Factory for user instances that are free form. The credentials will be added to the principal
* of this instance. As nothing can be said about the credentials no validation will be done.
* @param principal the free form json principal
* @param attributes the free form json attributes that further describe the principal
* @return user instance
*/
public static io.vertx.rxjava.ext.auth.User create(JsonObject principal, JsonObject attributes) {
io.vertx.rxjava.ext.auth.User ret = io.vertx.rxjava.ext.auth.User.newInstance((io.vertx.ext.auth.User)io.vertx.ext.auth.User.create(principal, attributes));
return ret;
}
/**
* Gets extra attributes of the user. Attributes contains any attributes related
* to the outcome of authenticating a user (e.g.: issued date, metadata, etc...)
* @return a json object with any relevant attribute.
*/
public JsonObject attributes() {
JsonObject ret = delegate.attributes();
return ret;
}
/**
* Flags this user object to be expired. A User is considered expired if it contains an expiration time and
* the current clock time is post the expiration date.
* @return true
if expired
*/
public boolean expired() {
boolean ret = delegate.expired();
return ret;
}
/**
* Flags this user object to be expired. Expiration takes 3 values in account:
*
*
* exp
"expiration" timestamp in seconds.
* iat
"issued at" in seconds.
* nbf
"not before" in seconds.
*
* A User is considered expired if it contains any of the above and
* the current clock time does not agree with the parameter value. If the {@link io.vertx.rxjava.ext.auth.User#principal} do not contain a key
* then {@link io.vertx.rxjava.ext.auth.User#attributes} are checked.
*
* If all of the properties are not available the user will not expire.
*
* Implementations of this interface might relax this rule to account for a leeway to safeguard against
* clock drifting.
* @param leeway a greater than zero leeway value.
* @return true
if expired
*/
public boolean expired(int leeway) {
boolean ret = delegate.expired(leeway);
return ret;
}
/**
* Returns user's authorizations that have been previously loaded by the providers.
* @return authorizations holder for the user.
*/
public io.vertx.rxjava.ext.auth.authorization.Authorizations authorizations() {
io.vertx.rxjava.ext.auth.authorization.Authorizations ret = io.vertx.rxjava.ext.auth.authorization.Authorizations.newInstance((io.vertx.ext.auth.authorization.Authorizations)delegate.authorizations());
return ret;
}
/**
* Is the user authorised to
* @param authority the authority - what this really means is determined by the specific implementation. It might represent a permission to access a resource e.g. `printers:printer34` or it might represent authority to a role in a roles based model, e.g. `role:admin`.
* @param resultHandler handler that will be called with an {@link io.vertx.core.AsyncResult} containing the value `true` if the they has the authority or `false` otherwise.
* @return the User to enable fluent use
*/
@Deprecated()
public io.vertx.rxjava.ext.auth.User isAuthorized(io.vertx.rxjava.ext.auth.authorization.Authorization authority, Handler> resultHandler) {
delegate.isAuthorized(authority.getDelegate(), resultHandler);
return this;
}
/**
* Is the user authorised to
* @param authority the authority - what this really means is determined by the specific implementation. It might represent a permission to access a resource e.g. `printers:printer34` or it might represent authority to a role in a roles based model, e.g. `role:admin`.
* @return the User to enable fluent use
*/
@Deprecated()
public io.vertx.rxjava.ext.auth.User isAuthorized(io.vertx.rxjava.ext.auth.authorization.Authorization authority) {
return
isAuthorized(authority, ar -> { });
}
/**
* Is the user authorised to
* @param authority the authority - what this really means is determined by the specific implementation. It might represent a permission to access a resource e.g. `printers:printer34` or it might represent authority to a role in a roles based model, e.g. `role:admin`.
* @return the User to enable fluent use
*/
@Deprecated()
public Single rxIsAuthorized(io.vertx.rxjava.ext.auth.authorization.Authorization authority) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
isAuthorized(authority, fut);
}));
}
/**
* Is the user authorised to
* @param authority the authority - what this really means is determined by the specific implementation. It might represent a permission to access a resource e.g. `printers:printer34` or it might represent authority to a role in a roles based model, e.g. `role:admin`.
* @param resultHandler handler that will be called with an {@link io.vertx.core.AsyncResult} containing the value `true` if the they has the authority or `false` otherwise.
* @return the User to enable fluent use
*/
@Deprecated()
public io.vertx.rxjava.ext.auth.User isAuthorized(String authority, Handler> resultHandler) {
delegate.isAuthorized(authority, resultHandler);
return this;
}
/**
* Is the user authorised to
* @param authority the authority - what this really means is determined by the specific implementation. It might represent a permission to access a resource e.g. `printers:printer34` or it might represent authority to a role in a roles based model, e.g. `role:admin`.
* @return the User to enable fluent use
*/
@Deprecated()
public io.vertx.rxjava.ext.auth.User isAuthorized(String authority) {
return
isAuthorized(authority, ar -> { });
}
/**
* Is the user authorised to
* @param authority the authority - what this really means is determined by the specific implementation. It might represent a permission to access a resource e.g. `printers:printer34` or it might represent authority to a role in a roles based model, e.g. `role:admin`.
* @return the User to enable fluent use
*/
@Deprecated()
public Single rxIsAuthorized(String authority) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
isAuthorized(authority, fut);
}));
}
/**
* The User object will cache any authorities that it knows it has to avoid hitting the
* underlying auth provider each time. Use this method if you want to clear this cache.
* @return the User to enable fluent use
*/
@Deprecated()
public io.vertx.rxjava.ext.auth.User clearCache() {
delegate.clearCache();
return this;
}
/**
* Get the underlying principal for the User. What this actually returns depends on the implementation.
* For a simple user/password based auth, it's likely to contain a JSON object with the following structure:
*
* {
* "username", "tim"
* }
*
* @return JSON representation of the Principal
*/
public JsonObject principal() {
JsonObject ret = delegate.principal();
return ret;
}
/**
* Set the auth provider for the User. This is typically used to reattach a detached User with an AuthProvider, e.g.
* after it has been deserialized.
* @param authProvider the AuthProvider - this must be the same type of AuthProvider that originally created the User
*/
@Deprecated()
public void setAuthProvider(io.vertx.rxjava.ext.auth.AuthProvider authProvider) {
delegate.setAuthProvider(authProvider.getDelegate());
}
public static User newInstance(io.vertx.ext.auth.User arg) {
return arg != null ? new User(arg) : null;
}
}