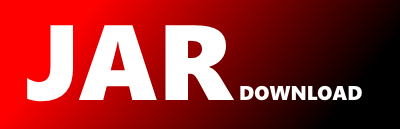
io.vertx.rxjava.ext.auth.authentication.Credentials Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.auth.authentication;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Abstract representation of a Credentials object. All implementations of this interface will define the
* required types and parameters for the specific implementation.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.authentication.Credentials original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.auth.authentication.Credentials.class)
public interface Credentials {
io.vertx.ext.auth.authentication.Credentials getDelegate();
/**
* Implementors should override this method to perform validation. An argument is allowed to
* allow custom validation, for example, when given a configuration property, a specific
* property may be allowed to be null.
* @param arg optional argument or null.
*/
public void checkValid(V arg);
/**
* Simple interop to downcast back to JSON for backwards compatibility.
* @return JSON representation of this credential.
*/
public JsonObject toJson();
/**
* Applies the HTTP Authorization challenge to this Credential instance. The internal state can change to reflect
* the extra properties the challenge conveys.
*
* See https://tools.ietf.org/html/rfc7235 for more information.
* @param challenge the challenge is the WWW-Authenticate
header response from a 401 request. Null challenges are allowed, and in this case, no verification will be performed, however it is up to the implementation to permit this.
* @param method The http method this response is responding.
* @param uri The http uri this response is responding.
* @param nc The client internal counter (optional).
* @param cnonce The client internal nonce (optional).
* @return fluent self.
*/
public io.vertx.rxjava.ext.auth.authentication.Credentials applyHttpChallenge(String challenge, io.vertx.core.http.HttpMethod method, String uri, Integer nc, String cnonce);
/**
* Applies the HTTP Authorization challenge to this Credential instance. The internal state can change to reflect
* the extra properties the challenge conveys.
*
* See https://tools.ietf.org/html/rfc7235 for more information.
* @param challenge the challenge is the WWW-Authenticate
header response from a 401 request. Null challenges are allowed, and in this case, no verification will be performed, however it is up to the implementation to permit this.
* @param method The http method this response is responding.
* @param uri The http uri this response is responding.
* @return fluent self.
*/
public io.vertx.rxjava.ext.auth.authentication.Credentials applyHttpChallenge(String challenge, io.vertx.core.http.HttpMethod method, String uri);
/**
* Applies the HTTP Authorization challenge to this Credential instance. The internal state can change to reflect
* the extra properties the challenge conveys.
*
* See https://tools.ietf.org/html/rfc7235 for more information.
* @param challenge the challenge is the WWW-Authenticate
header response from a 401 request. Null challenges are allowed, and in this case, no verification will be performed, however it is up to the implementation to permit this.
* @return fluent self.
*/
public io.vertx.rxjava.ext.auth.authentication.Credentials applyHttpChallenge(String challenge);
/**
* Encodes this credential as an HTTP Authorization https://tools.ietf.org/html/rfc7235.
*
* Calls to this method, expect that {@link io.vertx.rxjava.ext.auth.authentication.Credentials#applyHttpChallenge} has
* been prior executed. For some Authentication schemes, this isn't a requirement but doing so ensures that the
* object is on the right state.
* @return HTTP header including scheme.
*/
public String toHttpAuthorization();
public static Credentials newInstance(io.vertx.ext.auth.authentication.Credentials arg) {
return arg != null ? new CredentialsImpl(arg) : null;
}
}
class CredentialsImpl implements Credentials {
private final io.vertx.ext.auth.authentication.Credentials delegate;
public CredentialsImpl(io.vertx.ext.auth.authentication.Credentials delegate) {
this.delegate = delegate;
}
public CredentialsImpl(Object delegate) {
this.delegate = (io.vertx.ext.auth.authentication.Credentials)delegate;
}
public io.vertx.ext.auth.authentication.Credentials getDelegate() {
return delegate;
}
/**
* Implementors should override this method to perform validation. An argument is allowed to
* allow custom validation, for example, when given a configuration property, a specific
* property may be allowed to be null.
* @param arg optional argument or null.
*/
public void checkValid(V arg) {
delegate.checkValid(arg);
}
/**
* Simple interop to downcast back to JSON for backwards compatibility.
* @return JSON representation of this credential.
*/
public JsonObject toJson() {
JsonObject ret = delegate.toJson();
return ret;
}
/**
* Applies the HTTP Authorization challenge to this Credential instance. The internal state can change to reflect
* the extra properties the challenge conveys.
*
* See https://tools.ietf.org/html/rfc7235 for more information.
* @param challenge the challenge is the WWW-Authenticate
header response from a 401 request. Null challenges are allowed, and in this case, no verification will be performed, however it is up to the implementation to permit this.
* @param method The http method this response is responding.
* @param uri The http uri this response is responding.
* @param nc The client internal counter (optional).
* @param cnonce The client internal nonce (optional).
* @return fluent self.
*/
public io.vertx.rxjava.ext.auth.authentication.Credentials applyHttpChallenge(String challenge, io.vertx.core.http.HttpMethod method, String uri, Integer nc, String cnonce) {
delegate.applyHttpChallenge(challenge, method, uri, nc, cnonce);
return this;
}
/**
* Applies the HTTP Authorization challenge to this Credential instance. The internal state can change to reflect
* the extra properties the challenge conveys.
*
* See https://tools.ietf.org/html/rfc7235 for more information.
* @param challenge the challenge is the WWW-Authenticate
header response from a 401 request. Null challenges are allowed, and in this case, no verification will be performed, however it is up to the implementation to permit this.
* @param method The http method this response is responding.
* @param uri The http uri this response is responding.
* @return fluent self.
*/
public io.vertx.rxjava.ext.auth.authentication.Credentials applyHttpChallenge(String challenge, io.vertx.core.http.HttpMethod method, String uri) {
io.vertx.rxjava.ext.auth.authentication.Credentials ret = io.vertx.rxjava.ext.auth.authentication.Credentials.newInstance((io.vertx.ext.auth.authentication.Credentials)delegate.applyHttpChallenge(challenge, method, uri));
return ret;
}
/**
* Applies the HTTP Authorization challenge to this Credential instance. The internal state can change to reflect
* the extra properties the challenge conveys.
*
* See https://tools.ietf.org/html/rfc7235 for more information.
* @param challenge the challenge is the WWW-Authenticate
header response from a 401 request. Null challenges are allowed, and in this case, no verification will be performed, however it is up to the implementation to permit this.
* @return fluent self.
*/
public io.vertx.rxjava.ext.auth.authentication.Credentials applyHttpChallenge(String challenge) {
io.vertx.rxjava.ext.auth.authentication.Credentials ret = io.vertx.rxjava.ext.auth.authentication.Credentials.newInstance((io.vertx.ext.auth.authentication.Credentials)delegate.applyHttpChallenge(challenge));
return ret;
}
/**
* Encodes this credential as an HTTP Authorization https://tools.ietf.org/html/rfc7235.
*
* Calls to this method, expect that {@link io.vertx.rxjava.ext.auth.authentication.Credentials#applyHttpChallenge} has
* been prior executed. For some Authentication schemes, this isn't a requirement but doing so ensures that the
* object is on the right state.
* @return HTTP header including scheme.
*/
public String toHttpAuthorization() {
String ret = delegate.toHttpAuthorization();
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy