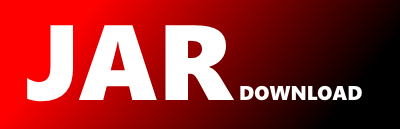
io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.auth.jdbc;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Determines how the hashing is computed in the implementation
*
* You can implement this to provide a different hashing strategy to the default.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.jdbc.JDBCHashStrategy original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.auth.jdbc.JDBCHashStrategy.class)
public class JDBCHashStrategy {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
JDBCHashStrategy that = (JDBCHashStrategy) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new JDBCHashStrategy((io.vertx.ext.auth.jdbc.JDBCHashStrategy) obj),
JDBCHashStrategy::getDelegate
);
private final io.vertx.ext.auth.jdbc.JDBCHashStrategy delegate;
public JDBCHashStrategy(io.vertx.ext.auth.jdbc.JDBCHashStrategy delegate) {
this.delegate = delegate;
}
public JDBCHashStrategy(Object delegate) {
this.delegate = (io.vertx.ext.auth.jdbc.JDBCHashStrategy)delegate;
}
public io.vertx.ext.auth.jdbc.JDBCHashStrategy getDelegate() {
return delegate;
}
/**
* This is the current backwards compatible hashing implementation, new applications should prefer the
* PBKDF2 implementation, unless the tradeoff between security and CPU usage is an option.
* @param vertx the vert.x instance
* @return the implementation.
*/
@Deprecated()
public static io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy createSHA512(io.vertx.rxjava.core.Vertx vertx) {
io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy ret = io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy.newInstance((io.vertx.ext.auth.jdbc.JDBCHashStrategy)io.vertx.ext.auth.jdbc.JDBCHashStrategy.createSHA512(vertx.getDelegate()));
return ret;
}
/**
* Implements a Hashing Strategy as per https://www.owasp.org/index.php/Password_Storage_Cheat_Sheet (2018-01-17).
*
* New deployments should use this strategy instead of the default one (which was the previous OWASP recommendation).
*
* The work factor can be updated by using the nonces json array.
* @param vertx the vert.x instance
* @return the implementation.
*/
@Deprecated()
public static io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy createPBKDF2(io.vertx.rxjava.core.Vertx vertx) {
io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy ret = io.vertx.rxjava.ext.auth.jdbc.JDBCHashStrategy.newInstance((io.vertx.ext.auth.jdbc.JDBCHashStrategy)io.vertx.ext.auth.jdbc.JDBCHashStrategy.createPBKDF2(vertx.getDelegate()));
return ret;
}
/**
* Compute a random salt.
* @return a non null salt value
*/
@Deprecated()
public String generateSalt() {
String ret = delegate.generateSalt();
return ret;
}
/**
* Compute the hashed password given the unhashed password and the salt
* @param password the unhashed password
* @param salt the salt
* @param version the nonce version to use
* @return the hashed password
*/
@Deprecated()
public String computeHash(String password, String salt, int version) {
String ret = delegate.computeHash(password, salt, version);
return ret;
}
/**
* Retrieve the hashed password from the result of the authentication query
* @param row the row
* @return the hashed password
*/
@Deprecated()
public String getHashedStoredPwd(JsonArray row) {
String ret = delegate.getHashedStoredPwd(row);
return ret;
}
/**
* Retrieve the salt from the result of the authentication query
* @param row the row
* @return the salt
*/
@Deprecated()
public String getSalt(JsonArray row) {
String ret = delegate.getSalt(row);
return ret;
}
/**
* Sets a ordered list of nonces where each position corresponds to a version.
*
* The nonces are supposed not to be stored in the underlying jdbc storage but to
* be provided as a application configuration. The idea is to add one extra variable
* to the hash function in order to make breaking the passwords using rainbow tables
* or precomputed hashes harder. Leaving the attacker only with the brute force
* approach.
*
* Nonces are dependent on the implementation. E.g.: for the SHA512 they are extra salt
* used during the hashing, for the PBKDF2 they map the number of iterations the algorithm
* should take
* @param nonces a json array.
*/
@Deprecated()
public void setNonces(JsonArray nonces) {
delegate.setNonces(nonces);
}
/**
* Time constant string comparision to avoid timming attacks.
* @param hasha hash a to compare
* @param hashb hash b to compare
* @return true if equal
*/
@Deprecated()
public static boolean isEqual(String hasha, String hashb) {
boolean ret = io.vertx.ext.auth.jdbc.JDBCHashStrategy.isEqual(hasha, hashb);
return ret;
}
public static JDBCHashStrategy newInstance(io.vertx.ext.auth.jdbc.JDBCHashStrategy arg) {
return arg != null ? new JDBCHashStrategy(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy