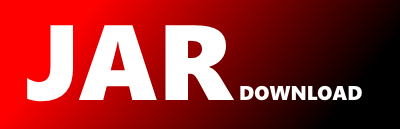
io.vertx.rxjava.ext.sql.SQLClientHelper Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2018 Contributors to the Eclipse Foundation
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the Apache License, Version 2.0
* which is available at https://www.apache.org/licenses/LICENSE-2.0.
*
* SPDX-License-Identifier: EPL-2.0 OR Apache-2.0
*/
package io.vertx.rxjava.ext.sql;
import io.vertx.rxjava.ext.sql.impl.InTransactionCompletable;
import io.vertx.rxjava.ext.sql.impl.InTransactionObservable;
import io.vertx.rxjava.ext.sql.impl.InTransactionSingle;
import rx.Completable;
import rx.Observable;
import rx.Single;
import rx.exceptions.Exceptions;
import java.util.function.Function;
/**
* Utilities for generating observables with a {@link SQLClient}.
*
* @author Thomas Segismont
*/
public class SQLClientHelper {
/**
* Creates a {@link Observable.Transformer} decorating an {@link Observable} with transaction management for a given {@link SQLConnection}.
*
* If the upstream {@link Observable} completes (onComplete), the transaction is committed.
* If the upstream {@link Observable} emits an error (onError), the transaction is rollbacked.
*
* Eventually, the given {@link SQLConnection} is put back in autocommit mode.
*
* @param sqlConnection the {@link SQLConnection} used for database operations and transaction management
* @param the type of the items emitted by the upstream {@link Observable}
* @return a {@link Observable.Transformer} decorating an {@link Observable} with transaction management
*/
public static Observable.Transformer txObservableTransformer(SQLConnection sqlConnection) {
return new InTransactionObservable<>(sqlConnection);
}
/**
* Generates a {@link Observable} from {@link SQLConnection} operations executed inside a transaction.
*
* @param client the {@link SQLClient}
* @param sourceSupplier a user-provided function returning a {@link Observable} generated by interacting with the given {@link SQLConnection}
* @param the type of the items emitted by the {@link Observable}
* @return an {@link Observable} generated from {@link SQLConnection} operations executed inside a transaction
*/
public static Observable inTransactionObservable(SQLClient client, Function> sourceSupplier) {
return usingConnectionObservable(client, conn -> sourceSupplier.apply(conn).compose(txObservableTransformer(conn)));
}
/**
* Creates a {@link Single.Transformer} decorating a {@link Single} with transaction management for a given {@link SQLConnection}.
*
* If the upstream {@link Single} emits a value (onSuccess), the transaction is committed.
* If the upstream {@link Single} emits an error (onError), the transaction is rollbacked.
*
* Eventually, the given {@link SQLConnection} is put back in autocommit mode.
*
* @param sqlConnection the {@link SQLConnection} used for database operations and transaction management
* @param the type of the item emitted by the upstream {@link Single}
* @return a {@link Single.Transformer} decorating a {@link Single} with transaction management
*/
public static Single.Transformer txSingleTransformer(SQLConnection sqlConnection) {
return new InTransactionSingle<>(sqlConnection);
}
/**
* Generates a {@link Single} from {@link SQLConnection} operations executed inside a transaction.
*
* @param client the {@link SQLClient}
* @param sourceSupplier a user-provided function returning a {@link Single} generated by interacting with the given {@link SQLConnection}
* @param the type of the item emitted by the {@link Single}
* @return a {@link Single} generated from {@link SQLConnection} operations executed inside a transaction
*/
public static Single inTransactionSingle(SQLClient client, Function> sourceSupplier) {
return usingConnectionSingle(client, conn -> sourceSupplier.apply(conn).compose(txSingleTransformer(conn)));
}
/**
* Creates a {@link Completable.Transformer} decorating a {@link Completable} with transaction management for a given {@link SQLConnection}.
*
* If the upstream {@link Completable} completes (onComplete), the transaction is committed.
* If the upstream {@link Completable} emits an error (onError), the transaction is rollbacked.
*
* Eventually, the given {@link SQLConnection} is put back in autocommit mode.
*
* @param sqlConnection the {@link SQLConnection} used for database operations and transaction management
* @return a {@link Completable.Transformer} decorating a {@link Completable} with transaction management
*/
public static Completable.Transformer txCompletableTransformer(SQLConnection sqlConnection) {
return new InTransactionCompletable(sqlConnection);
}
/**
* Generates a {@link Completable} from {@link SQLConnection} operations executed inside a transaction.
*
* @param client the {@link SQLClient}
* @param sourceSupplier a user-provided function returning a {@link Completable} generated by interacting with the given {@link SQLConnection}
* @return a {@link Completable} generated from {@link SQLConnection} operations executed inside a transaction
*/
public static Completable inTransactionCompletable(SQLClient client, Function sourceSupplier) {
return usingConnectionCompletable(client, conn -> sourceSupplier.apply(conn).compose(txCompletableTransformer(conn)));
}
/**
* Generates a {@link Observable} from {@link SQLConnection} operations.
*
* @param client the {@link SQLClient}
* @param sourceSupplier a user-provided function returning a {@link Observable} generated by interacting with the given {@link SQLConnection}
* @param the type of the items emitted by the {@link Observable}
* @return an {@link Observable} generated from {@link SQLConnection} operations
*/
public static Observable usingConnectionObservable(SQLClient client, Function> sourceSupplier) {
return client.rxGetConnection().flatMapObservable(conn -> {
try {
return sourceSupplier.apply(conn).doAfterTerminate(conn::close);
} catch (Throwable t) {
Exceptions.throwIfFatal(t);
conn.close();
return Observable.error(t);
}
});
}
/**
* Generates a {@link Single} from {@link SQLConnection} operations.
*
* @param client the {@link SQLClient}
* @param sourceSupplier a user-provided function returning a {@link Single} generated by interacting with the given {@link SQLConnection}
* @param the type of the item emitted by the {@link Single}
* @return a {@link Single} generated from {@link SQLConnection} operations
*/
public static Single usingConnectionSingle(SQLClient client, Function> sourceSupplier) {
return client.rxGetConnection().flatMap(conn -> {
try {
return sourceSupplier.apply(conn).doAfterTerminate(conn::close);
} catch (Throwable t) {
Exceptions.throwIfFatal(t);
conn.close();
return Single.error(t);
}
});
}
/**
* Generates a {@link Completable} from {@link SQLConnection} operations.
*
* @param client the {@link SQLClient}
* @param sourceSupplier a user-provided function returning a {@link Completable} generated by interacting with the given {@link SQLConnection}
* @return a {@link Completable} generated from {@link SQLConnection} operations
*/
public static Completable usingConnectionCompletable(SQLClient client, Function sourceSupplier) {
return client.rxGetConnection().flatMapCompletable(conn -> {
try {
return sourceSupplier.apply(conn).doAfterTerminate(conn::close);
} catch (Throwable t) {
Exceptions.throwIfFatal(t);
conn.close();
return Completable.error(t);
}
});
}
private SQLClientHelper() {
// Utility
}
}