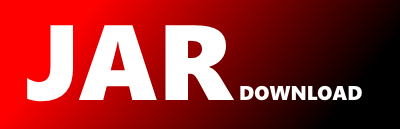
io.vertx.rxjava.ext.unit.TestSuite Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.unit;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A named suite of test cases that are executed altogether. The suite suite is created with
* the {@link io.vertx.rxjava.ext.unit.TestSuite#create} and the returned suite contains initially no tests.
*
* The suite can declare a callback before the suite with {@link io.vertx.rxjava.ext.unit.TestSuite#before} or after
* the suite with {@link io.vertx.rxjava.ext.unit.TestSuite#after}.
*
* The suite can declare a callback before each test with {@link io.vertx.rxjava.ext.unit.TestSuite#beforeEach} or after
* each test with {@link io.vertx.rxjava.ext.unit.TestSuite#afterEach}.
*
* Each test case of the suite is declared by calling the {@link io.vertx.rxjava.ext.unit.TestSuite#test} method.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.unit.TestSuite original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.unit.TestSuite.class)
public class TestSuite {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TestSuite that = (TestSuite) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new TestSuite((io.vertx.ext.unit.TestSuite) obj),
TestSuite::getDelegate
);
private final io.vertx.ext.unit.TestSuite delegate;
public TestSuite(io.vertx.ext.unit.TestSuite delegate) {
this.delegate = delegate;
}
public TestSuite(Object delegate) {
this.delegate = (io.vertx.ext.unit.TestSuite)delegate;
}
public io.vertx.ext.unit.TestSuite getDelegate() {
return delegate;
}
/**
* Create and return a new test suite.
* @param name the test suite name
* @return the created test suite
*/
public static io.vertx.rxjava.ext.unit.TestSuite create(String name) {
io.vertx.rxjava.ext.unit.TestSuite ret = io.vertx.rxjava.ext.unit.TestSuite.newInstance((io.vertx.ext.unit.TestSuite)io.vertx.ext.unit.TestSuite.create(name));
return ret;
}
/**
* Set a callback executed before the tests.
* @param callback the callback
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.ext.unit.TestSuite before(Handler callback) {
delegate.before(new Handler() {
public void handle(io.vertx.ext.unit.TestContext event) {
callback.handle(io.vertx.rxjava.ext.unit.TestContext.newInstance((io.vertx.ext.unit.TestContext)event));
}
});
return this;
}
/**
* Set a callback executed before each test and after the suite before
callback.
* @param callback the callback
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.ext.unit.TestSuite beforeEach(Handler callback) {
delegate.beforeEach(new Handler() {
public void handle(io.vertx.ext.unit.TestContext event) {
callback.handle(io.vertx.rxjava.ext.unit.TestContext.newInstance((io.vertx.ext.unit.TestContext)event));
}
});
return this;
}
/**
* Set a callback executed after the tests.
* @param callback the callback
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.ext.unit.TestSuite after(Handler callback) {
delegate.after(new Handler() {
public void handle(io.vertx.ext.unit.TestContext event) {
callback.handle(io.vertx.rxjava.ext.unit.TestContext.newInstance((io.vertx.ext.unit.TestContext)event));
}
});
return this;
}
/**
* Set a callback executed after each test and before the suite after
callback.
* @param callback the callback
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.ext.unit.TestSuite afterEach(Handler callback) {
delegate.afterEach(new Handler() {
public void handle(io.vertx.ext.unit.TestContext event) {
callback.handle(io.vertx.rxjava.ext.unit.TestContext.newInstance((io.vertx.ext.unit.TestContext)event));
}
});
return this;
}
/**
* Add a new test case to the suite.
* @param name the test case name
* @param testCase the test case
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.ext.unit.TestSuite test(String name, Handler testCase) {
delegate.test(name, new Handler() {
public void handle(io.vertx.ext.unit.TestContext event) {
testCase.handle(io.vertx.rxjava.ext.unit.TestContext.newInstance((io.vertx.ext.unit.TestContext)event));
}
});
return this;
}
/**
* Add a new test case to the suite.
* @param name the test case name
* @param repeat the number of times the test should be repeated
* @param testCase the test case
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.ext.unit.TestSuite test(String name, int repeat, Handler testCase) {
delegate.test(name, repeat, new Handler() {
public void handle(io.vertx.ext.unit.TestContext event) {
testCase.handle(io.vertx.rxjava.ext.unit.TestContext.newInstance((io.vertx.ext.unit.TestContext)event));
}
});
return this;
}
/**
* Run the testsuite with the default options.
*
* When the test suite is executed in a Vertx context (i.e `Vertx.currentContext()` returns a context) this
* context's event loop is used for running the test suite. Otherwise it is executed in the current thread.
*
* The returned {@link io.vertx.rxjava.ext.unit.Completion} object can be used to get a completion callback.
* @return the related test completion
*/
public io.vertx.rxjava.ext.unit.TestCompletion run() {
io.vertx.rxjava.ext.unit.TestCompletion ret = io.vertx.rxjava.ext.unit.TestCompletion.newInstance((io.vertx.ext.unit.TestCompletion)delegate.run());
return ret;
}
/**
* Run the testsuite with the specified options
.
*
* When the test suite is executed in a Vertx context (i.e `Vertx.currentContext()` returns a context) this
* context's event loop is used for running the test suite unless the {@link io.vertx.ext.unit.TestOptions}
* is set to false
. In this case it is executed by the current thread.
*
* Otherwise, the test suite will be executed in the current thread when {@link io.vertx.ext.unit.TestOptions} is
* set to false
or null
. If the value is true
, this methods throws an IllegalStateException
.
*
* The returned {@link io.vertx.rxjava.ext.unit.Completion} object can be used to get a completion callback.
* @param options the test options
* @return the related test completion
*/
public io.vertx.rxjava.ext.unit.TestCompletion run(io.vertx.ext.unit.TestOptions options) {
io.vertx.rxjava.ext.unit.TestCompletion ret = io.vertx.rxjava.ext.unit.TestCompletion.newInstance((io.vertx.ext.unit.TestCompletion)delegate.run(options));
return ret;
}
/**
* Run the testsuite with the default options and the specified vertx
instance.
*
* The test suite will be executed on the event loop provided by the vertx
argument. The returned
* {@link io.vertx.rxjava.ext.unit.Completion} object can be used to get a completion callback.
* @param vertx the vertx instance
* @return the related test completion
*/
public io.vertx.rxjava.ext.unit.TestCompletion run(io.vertx.rxjava.core.Vertx vertx) {
io.vertx.rxjava.ext.unit.TestCompletion ret = io.vertx.rxjava.ext.unit.TestCompletion.newInstance((io.vertx.ext.unit.TestCompletion)delegate.run(vertx.getDelegate()));
return ret;
}
/**
* Run the testsuite with the specified options
and the specified vertx
instance.
*
* The test suite will be executed on the event loop provided by the vertx
argument when
* {@link io.vertx.ext.unit.TestOptions} is not set to false
. The returned
* {@link io.vertx.rxjava.ext.unit.Completion} object can be used to get a completion callback.
* @param vertx the vertx instance
* @param options the test options
* @return the related test completion
*/
public io.vertx.rxjava.ext.unit.TestCompletion run(io.vertx.rxjava.core.Vertx vertx, io.vertx.ext.unit.TestOptions options) {
io.vertx.rxjava.ext.unit.TestCompletion ret = io.vertx.rxjava.ext.unit.TestCompletion.newInstance((io.vertx.ext.unit.TestCompletion)delegate.run(vertx.getDelegate(), options));
return ret;
}
public static TestSuite newInstance(io.vertx.ext.unit.TestSuite arg) {
return arg != null ? new TestSuite(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy