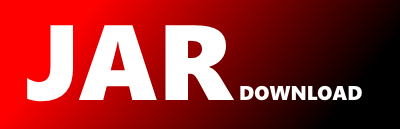
io.vertx.rxjava.ext.web.client.WebClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.web.client;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* An asynchronous HTTP / HTTP/2 client called WebClient
.
*
* The web client makes easy to do HTTP request/response interactions with a web server, and provides advanced
* features like:
*
* - Json body encoding / decoding
* - request/response pumping
* - error handling
*
*
* The web client does not deprecate the , it is actually based on it and therefore inherits
* its configuration and great features like pooling. The HttpClient
should be used when fine grained control over the HTTP
* requests/response is necessary.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.client.WebClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.web.client.WebClient.class)
public class WebClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
WebClient that = (WebClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new WebClient((io.vertx.ext.web.client.WebClient) obj),
WebClient::getDelegate
);
private final io.vertx.ext.web.client.WebClient delegate;
public WebClient(io.vertx.ext.web.client.WebClient delegate) {
this.delegate = delegate;
}
public WebClient(Object delegate) {
this.delegate = (io.vertx.ext.web.client.WebClient)delegate;
}
public io.vertx.ext.web.client.WebClient getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_3 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_4 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_5 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_6 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_7 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_8 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_9 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_10 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_11 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_12 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_13 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_14 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_15 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_16 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_17 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_18 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_19 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_20 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_21 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_22 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_23 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_24 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_25 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_26 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_27 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_28 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_29 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_30 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_31 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_32 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_33 = new TypeArg(o1 -> io.vertx.rxjava.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
/**
* Create a web client using the provided vertx
instance and default options.
* @param vertx the vertx instance
* @return the created web client
*/
public static io.vertx.rxjava.ext.web.client.WebClient create(io.vertx.rxjava.core.Vertx vertx) {
io.vertx.rxjava.ext.web.client.WebClient ret = io.vertx.rxjava.ext.web.client.WebClient.newInstance((io.vertx.ext.web.client.WebClient)io.vertx.ext.web.client.WebClient.create(vertx.getDelegate()));
return ret;
}
/**
* Create a web client using the provided vertx
instance.
* @param vertx the vertx instance
* @param options the Web Client options
* @return the created web client
*/
public static io.vertx.rxjava.ext.web.client.WebClient create(io.vertx.rxjava.core.Vertx vertx, io.vertx.ext.web.client.WebClientOptions options) {
io.vertx.rxjava.ext.web.client.WebClient ret = io.vertx.rxjava.ext.web.client.WebClient.newInstance((io.vertx.ext.web.client.WebClient)io.vertx.ext.web.client.WebClient.create(vertx.getDelegate(), options));
return ret;
}
/**
* Wrap an httpClient
with a web client and default options.
* @param httpClient the to wrap
* @return the web client
*/
public static io.vertx.rxjava.ext.web.client.WebClient wrap(io.vertx.rxjava.core.http.HttpClient httpClient) {
io.vertx.rxjava.ext.web.client.WebClient ret = io.vertx.rxjava.ext.web.client.WebClient.newInstance((io.vertx.ext.web.client.WebClient)io.vertx.ext.web.client.WebClient.wrap(httpClient.getDelegate()));
return ret;
}
/**
* Wrap an httpClient
with a web client and default options.
*
* Only the specific web client portion of the options
is used, the {@link io.vertx.core.http.HttpClientOptions}
* of the httpClient
is reused.
* @param httpClient the to wrap
* @param options the Web Client options
* @return the web client
*/
public static io.vertx.rxjava.ext.web.client.WebClient wrap(io.vertx.rxjava.core.http.HttpClient httpClient, io.vertx.ext.web.client.WebClientOptions options) {
io.vertx.rxjava.ext.web.client.WebClient ret = io.vertx.rxjava.ext.web.client.WebClient.newInstance((io.vertx.ext.web.client.WebClient)io.vertx.ext.web.client.WebClient.wrap(httpClient.getDelegate(), options));
return ret;
}
/**
* Create an HTTP request to send to the server at the specified host and port.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, port, host, requestURI), TYPE_ARG_0);
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.WebClient#request} using the serverAddress
parameter to connect to the
* server instead of the port
and host
parameters.
*
* The request host header will still be created from the port
and host
parameters.
*
* Use to connect to a unix domain socket server.
* @param method
* @param serverAddress
* @param port
* @param host
* @param requestURI
* @return
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, io.vertx.rxjava.core.net.SocketAddress serverAddress, int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, serverAddress.getDelegate(), port, host, requestURI), TYPE_ARG_1);
return ret;
}
/**
* Create an HTTP request to send to the server at the specified host and default port.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, host, requestURI), TYPE_ARG_2);
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.WebClient#request} using the serverAddress
parameter to connect to the
* server instead of the default port and host
parameter.
*
* The request host header will still be created from the default port and host
parameter.
*
* Use to connect to a unix domain socket server.
* @param method
* @param serverAddress
* @param host
* @param requestURI
* @return
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, io.vertx.rxjava.core.net.SocketAddress serverAddress, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, serverAddress.getDelegate(), host, requestURI), TYPE_ARG_3);
return ret;
}
/**
* Create an HTTP request to send to the server at the default host and port.
* @param method the HTTP method
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, requestURI), TYPE_ARG_4);
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.WebClient#request} using the serverAddress
parameter to connect to the
* server instead of the default port and default host.
*
* The request host header will still be created from the default port and default host.
*
* Use to connect to a unix domain socket server.
* @param method
* @param serverAddress
* @param requestURI
* @return
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, io.vertx.rxjava.core.net.SocketAddress serverAddress, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, serverAddress.getDelegate(), requestURI), TYPE_ARG_5);
return ret;
}
/**
* Create an HTTP request to send to the server at the specified host and port.
* @param method the HTTP method
* @param options the request options
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, io.vertx.core.http.RequestOptions options) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, options), TYPE_ARG_6);
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.WebClient#request} using the serverAddress
parameter to connect to the
* server instead of the options
parameter.
*
* The request host header will still be created from the options
parameter.
*
* Use to connect to a unix domain socket server.
* @param method
* @param serverAddress
* @param options
* @return
*/
public io.vertx.rxjava.ext.web.client.HttpRequest request(io.vertx.core.http.HttpMethod method, io.vertx.rxjava.core.net.SocketAddress serverAddress, io.vertx.core.http.RequestOptions options) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.request(method, serverAddress.getDelegate(), options), TYPE_ARG_7);
return ret;
}
/**
* Create an HTTP request to send to the server using an absolute URI
* @param method the HTTP method
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest requestAbs(io.vertx.core.http.HttpMethod method, String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.requestAbs(method, absoluteURI), TYPE_ARG_8);
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.WebClient#requestAbs} using the serverAddress
parameter to connect to the
* server instead of the absoluteURI
parameter.
*
* The request host header will still be created from the absoluteURI
parameter.
*
* Use to connect to a unix domain socket server.
* @param method
* @param serverAddress
* @param absoluteURI
* @return
*/
public io.vertx.rxjava.ext.web.client.HttpRequest requestAbs(io.vertx.core.http.HttpMethod method, io.vertx.rxjava.core.net.SocketAddress serverAddress, String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.requestAbs(method, serverAddress.getDelegate(), absoluteURI), TYPE_ARG_9);
return ret;
}
/**
* Create an HTTP GET request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest get(String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.get(requestURI), TYPE_ARG_10);
return ret;
}
/**
* Create an HTTP GET request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest get(int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.get(port, host, requestURI), TYPE_ARG_11);
return ret;
}
/**
* Create an HTTP GET request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest get(String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.get(host, requestURI), TYPE_ARG_12);
return ret;
}
/**
* Create an HTTP GET request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest getAbs(String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.getAbs(absoluteURI), TYPE_ARG_13);
return ret;
}
/**
* Create an HTTP POST request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest post(String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.post(requestURI), TYPE_ARG_14);
return ret;
}
/**
* Create an HTTP POST request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest post(int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.post(port, host, requestURI), TYPE_ARG_15);
return ret;
}
/**
* Create an HTTP POST request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest post(String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.post(host, requestURI), TYPE_ARG_16);
return ret;
}
/**
* Create an HTTP POST request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest postAbs(String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.postAbs(absoluteURI), TYPE_ARG_17);
return ret;
}
/**
* Create an HTTP PUT request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest put(String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.put(requestURI), TYPE_ARG_18);
return ret;
}
/**
* Create an HTTP PUT request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest put(int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.put(port, host, requestURI), TYPE_ARG_19);
return ret;
}
/**
* Create an HTTP PUT request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest put(String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.put(host, requestURI), TYPE_ARG_20);
return ret;
}
/**
* Create an HTTP PUT request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest putAbs(String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.putAbs(absoluteURI), TYPE_ARG_21);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest delete(String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.delete(requestURI), TYPE_ARG_22);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest delete(int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.delete(port, host, requestURI), TYPE_ARG_23);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest delete(String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.delete(host, requestURI), TYPE_ARG_24);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest deleteAbs(String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.deleteAbs(absoluteURI), TYPE_ARG_25);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest patch(String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.patch(requestURI), TYPE_ARG_26);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest patch(int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.patch(port, host, requestURI), TYPE_ARG_27);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest patch(String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.patch(host, requestURI), TYPE_ARG_28);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest patchAbs(String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.patchAbs(absoluteURI), TYPE_ARG_29);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest head(String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.head(requestURI), TYPE_ARG_30);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest head(int port, String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.head(port, host, requestURI), TYPE_ARG_31);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest head(String host, String requestURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.head(host, requestURI), TYPE_ARG_32);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public io.vertx.rxjava.ext.web.client.HttpRequest headAbs(String absoluteURI) {
io.vertx.rxjava.ext.web.client.HttpRequest ret = io.vertx.rxjava.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.headAbs(absoluteURI), TYPE_ARG_33);
return ret;
}
/**
* Close the client. Closing will close down any pooled connections.
* Clients should always be closed after use.
*/
public void close() {
delegate.close();
}
public static WebClient newInstance(io.vertx.ext.web.client.WebClient arg) {
return arg != null ? new WebClient(arg) : null;
}
}