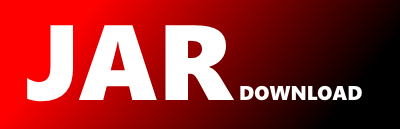
io.vertx.rxjava.core.file.AsyncFile Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.core.file;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents a file on the file-system which can be read from, or written to asynchronously.
*
* This class also implements {@link io.vertx.rxjava.core.streams.ReadStream} and
* {@link io.vertx.rxjava.core.streams.WriteStream}. This allows the data to be piped to and from
* other streams, e.g. an {@link io.vertx.rxjava.core.http.HttpClientRequest} instance,
* using the {@link io.vertx.rxjava.core.streams.Pipe} class
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.file.AsyncFile original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.file.AsyncFile.class)
public class AsyncFile implements io.vertx.rxjava.core.streams.ReadStream, io.vertx.rxjava.core.streams.WriteStream {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AsyncFile that = (AsyncFile) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new AsyncFile((io.vertx.core.file.AsyncFile) obj),
AsyncFile::getDelegate
);
private final io.vertx.core.file.AsyncFile delegate;
public AsyncFile(io.vertx.core.file.AsyncFile delegate) {
this.delegate = delegate;
}
public AsyncFile(Object delegate) {
this.delegate = (io.vertx.core.file.AsyncFile)delegate;
}
public io.vertx.core.file.AsyncFile getDelegate() {
return delegate;
}
private Observable observable;
public synchronized Observable toObservable() {
if (observable == null) {
observable = RxHelper.toObservable(this.getDelegate());
}
return observable;
}
private WriteStreamSubscriber subscriber;
public synchronized WriteStreamSubscriber toSubscriber() {
if (subscriber == null) {
subscriber = RxHelper.toSubscriber(getDelegate());
}
return subscriber;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava.core.file.AsyncFileLock.newInstance((io.vertx.core.file.AsyncFileLock)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.rxjava.core.file.AsyncFileLock.newInstance((io.vertx.core.file.AsyncFileLock)o1), o1 -> o1.getDelegate());
/**
* Pause this stream and return a to transfer the elements of this stream to a destination .
*
* The stream will be resumed when the pipe will be wired to a WriteStream
.
* @return a pipe
*/
public io.vertx.rxjava.core.streams.Pipe pipe() {
io.vertx.rxjava.core.streams.Pipe ret = io.vertx.rxjava.core.streams.Pipe.newInstance((io.vertx.core.streams.Pipe)delegate.pipe(), TypeArg.unknown());
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
* @param dst the destination write stream
* @return a future notified when the write stream will be ended with the outcome
*/
public io.vertx.core.Future pipeTo(io.vertx.rxjava.core.streams.WriteStream dst) {
io.vertx.core.Future ret = delegate.pipeTo(dst.getDelegate()).map(val -> val);
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
* @param dst the destination write stream
* @return a future notified when the write stream will be ended with the outcome
*/
public rx.Single rxPipeTo(io.vertx.rxjava.core.streams.WriteStream dst) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.pipeTo(dst).onComplete(fut);
}));
}
/**
* Write some data to the stream.
*
* The data is usually put on an internal write queue, and the write actually happens
* asynchronously. To avoid running out of memory by putting too much on the write queue,
* check the {@link io.vertx.rxjava.core.streams.WriteStream#writeQueueFull} method before writing. This is done automatically if
* using a .
*
*
When the data
is moved from the queue to the actual medium, the returned
* will be completed with the write result, e.g the future is succeeded
* when a server HTTP response buffer is written to the socket and failed if the remote
* client has closed the socket while the data was still pending for write.
* @param data the data to write
* @return a future completed with the write result
*/
public io.vertx.core.Future write(io.vertx.core.buffer.Buffer data) {
io.vertx.core.Future ret = delegate.write(data).map(val -> val);
return ret;
}
/**
* Write some data to the stream.
*
* The data is usually put on an internal write queue, and the write actually happens
* asynchronously. To avoid running out of memory by putting too much on the write queue,
* check the {@link io.vertx.rxjava.core.streams.WriteStream#writeQueueFull} method before writing. This is done automatically if
* using a .
*
*
When the data
is moved from the queue to the actual medium, the returned
* will be completed with the write result, e.g the future is succeeded
* when a server HTTP response buffer is written to the socket and failed if the remote
* client has closed the socket while the data was still pending for write.
* @param data the data to write
* @return a future completed with the write result
*/
public rx.Single rxWrite(io.vertx.core.buffer.Buffer data) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.write(data).onComplete(fut);
}));
}
/**
* Ends the stream.
*
* Once the stream has ended, it cannot be used any more.
* @return a future completed with the result
*/
public io.vertx.core.Future end() {
io.vertx.core.Future ret = delegate.end().map(val -> val);
return ret;
}
/**
* Ends the stream.
*
* Once the stream has ended, it cannot be used any more.
* @return a future completed with the result
*/
public rx.Single rxEnd() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.end().onComplete(fut);
}));
}
/**
* Same as {@link io.vertx.rxjava.core.streams.WriteStream#end} but writes some data to the stream before ending.
* @param data the data to write
* @return a future completed with the result
*/
public io.vertx.core.Future end(io.vertx.core.buffer.Buffer data) {
io.vertx.core.Future ret = delegate.end(data).map(val -> val);
return ret;
}
/**
* Same as {@link io.vertx.rxjava.core.streams.WriteStream#end} but writes some data to the stream before ending.
* @param data the data to write
* @return a future completed with the result
*/
public rx.Single rxEnd(io.vertx.core.buffer.Buffer data) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.end(data).onComplete(fut);
}));
}
/**
* This will return true
if there are more bytes in the write queue than the value set using {@link io.vertx.rxjava.core.file.AsyncFile#setWriteQueueMaxSize}
* @return true
if write queue is full
*/
public boolean writeQueueFull() {
boolean ret = delegate.writeQueueFull();
return ret;
}
public io.vertx.rxjava.core.file.AsyncFile handler(io.vertx.core.Handler handler) {
delegate.handler(handler);
return this;
}
public io.vertx.rxjava.core.file.AsyncFile pause() {
delegate.pause();
return this;
}
public io.vertx.rxjava.core.file.AsyncFile resume() {
delegate.resume();
return this;
}
public io.vertx.rxjava.core.file.AsyncFile endHandler(io.vertx.core.Handler endHandler) {
delegate.endHandler(endHandler);
return this;
}
public io.vertx.rxjava.core.file.AsyncFile setWriteQueueMaxSize(int maxSize) {
delegate.setWriteQueueMaxSize(maxSize);
return this;
}
public io.vertx.rxjava.core.file.AsyncFile drainHandler(io.vertx.core.Handler handler) {
delegate.drainHandler(handler);
return this;
}
public io.vertx.rxjava.core.file.AsyncFile exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.rxjava.core.file.AsyncFile fetch(long amount) {
delegate.fetch(amount);
return this;
}
/**
* Close the file. The actual close happens asynchronously.
* @return a future completed with the result
*/
public io.vertx.core.Future close() {
io.vertx.core.Future ret = delegate.close().map(val -> val);
return ret;
}
/**
* Close the file. The actual close happens asynchronously.
* @return a future completed with the result
*/
public rx.Single rxClose() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.close().onComplete(fut);
}));
}
/**
* Write a {@link io.vertx.core.buffer.Buffer} to the file at position position
in the file, asynchronously.
*
* If position
lies outside of the current size
* of the file, the file will be enlarged to encompass it.
*
* When multiple writes are invoked on the same file
* there are no guarantees as to order in which those writes actually occur
* @param buffer the buffer to write
* @param position the position in the file to write it at
* @return a future notified when the write is complete
*/
public io.vertx.core.Future write(io.vertx.core.buffer.Buffer buffer, long position) {
io.vertx.core.Future ret = delegate.write(buffer, position).map(val -> val);
return ret;
}
/**
* Write a {@link io.vertx.core.buffer.Buffer} to the file at position position
in the file, asynchronously.
*
* If position
lies outside of the current size
* of the file, the file will be enlarged to encompass it.
*
* When multiple writes are invoked on the same file
* there are no guarantees as to order in which those writes actually occur
* @param buffer the buffer to write
* @param position the position in the file to write it at
* @return a future notified when the write is complete
*/
public rx.Single rxWrite(io.vertx.core.buffer.Buffer buffer, long position) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.write(buffer, position).onComplete(fut);
}));
}
/**
* Reads length
bytes of data from the file at position position
in the file, asynchronously.
*
* The read data will be written into the specified Buffer buffer
at position offset
.
*
* If data is read past the end of the file then zero bytes will be read.
* When multiple reads are invoked on the same file there are no guarantees as to order in which those reads actually occur.
* @param buffer the buffer to read into
* @param offset the offset into the buffer where the data will be read
* @param position the position in the file where to start reading
* @param length the number of bytes to read
* @return a future notified when the write is complete
*/
public io.vertx.core.Future read(io.vertx.core.buffer.Buffer buffer, int offset, long position, int length) {
io.vertx.core.Future ret = delegate.read(buffer, offset, position, length).map(val -> val);
return ret;
}
/**
* Reads length
bytes of data from the file at position position
in the file, asynchronously.
*
* The read data will be written into the specified Buffer buffer
at position offset
.
*
* If data is read past the end of the file then zero bytes will be read.
* When multiple reads are invoked on the same file there are no guarantees as to order in which those reads actually occur.
* @param buffer the buffer to read into
* @param offset the offset into the buffer where the data will be read
* @param position the position in the file where to start reading
* @param length the number of bytes to read
* @return a future notified when the write is complete
*/
public rx.Single rxRead(io.vertx.core.buffer.Buffer buffer, int offset, long position, int length) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.read(buffer, offset, position, length).onComplete(fut);
}));
}
/**
* Flush any writes made to this file to underlying persistent storage.
*
* If the file was opened with flush
set to true
then calling this method will have no effect.
*
* The actual flush will happen asynchronously.
* @return a future completed with the result
*/
public io.vertx.core.Future flush() {
io.vertx.core.Future ret = delegate.flush().map(val -> val);
return ret;
}
/**
* Flush any writes made to this file to underlying persistent storage.
*
* If the file was opened with flush
set to true
then calling this method will have no effect.
*
* The actual flush will happen asynchronously.
* @return a future completed with the result
*/
public rx.Single rxFlush() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.flush().onComplete(fut);
}));
}
/**
* Sets the position from which data will be read from when using the file as a {@link io.vertx.rxjava.core.streams.ReadStream}.
* @param readPos the position in the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.AsyncFile setReadPos(long readPos) {
delegate.setReadPos(readPos);
return this;
}
/**
* Sets the number of bytes that will be read when using the file as a {@link io.vertx.rxjava.core.streams.ReadStream}.
* @param readLength the bytes that will be read from the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.AsyncFile setReadLength(long readLength) {
delegate.setReadLength(readLength);
return this;
}
/**
* @return the number of bytes that will be read when using the file as a {@link io.vertx.rxjava.core.streams.ReadStream}
*/
public long getReadLength() {
long ret = delegate.getReadLength();
return ret;
}
/**
* Sets the position from which data will be written when using the file as a {@link io.vertx.rxjava.core.streams.WriteStream}.
* @param writePos the position in the file
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.AsyncFile setWritePos(long writePos) {
delegate.setWritePos(writePos);
return this;
}
/**
* @return the current write position the file is at
*/
public long getWritePos() {
long ret = delegate.getWritePos();
return ret;
}
/**
* Sets the buffer size that will be used to read the data from the file. Changing this value will impact how much
* the data will be read at a time from the file system.
* @param readBufferSize the buffer size
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava.core.file.AsyncFile setReadBufferSize(int readBufferSize) {
delegate.setReadBufferSize(readBufferSize);
return this;
}
/**
* Like {@link io.vertx.rxjava.core.file.AsyncFile#size} but blocking.
* @return
*/
public long sizeBlocking() {
long ret = delegate.sizeBlocking();
return ret;
}
/**
* @return the size of the file
*/
public io.vertx.core.Future size() {
io.vertx.core.Future ret = delegate.size().map(val -> val);
return ret;
}
/**
* @return the size of the file
*/
public rx.Single rxSize() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.size().onComplete(fut);
}));
}
/**
* Try to acquire a non-shared lock on the entire file.
* @return the lock if it can be acquired immediately, otherwise null
*/
public io.vertx.rxjava.core.file.AsyncFileLock tryLock() {
io.vertx.rxjava.core.file.AsyncFileLock ret = io.vertx.rxjava.core.file.AsyncFileLock.newInstance((io.vertx.core.file.AsyncFileLock)delegate.tryLock());
return ret;
}
/**
* Try to acquire a lock on a portion of this file.
* @param position where the region starts
* @param size the size of the region
* @param shared whether the lock should be shared
* @return the lock if it can be acquired immediately, otherwise null
*/
public io.vertx.rxjava.core.file.AsyncFileLock tryLock(long position, long size, boolean shared) {
io.vertx.rxjava.core.file.AsyncFileLock ret = io.vertx.rxjava.core.file.AsyncFileLock.newInstance((io.vertx.core.file.AsyncFileLock)delegate.tryLock(position, size, shared));
return ret;
}
/**
* Acquire a non-shared lock on the entire file.
* @return a future indicating the completion of this operation
*/
public io.vertx.core.Future lock() {
io.vertx.core.Future ret = delegate.lock().map(val -> io.vertx.rxjava.core.file.AsyncFileLock.newInstance((io.vertx.core.file.AsyncFileLock)val));
return ret;
}
/**
* Acquire a non-shared lock on the entire file.
* @return a future indicating the completion of this operation
*/
public rx.Single rxLock() {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.lock().onComplete(fut);
}));
}
/**
* Acquire a lock on a portion of this file.
* @param position where the region starts
* @param size the size of the region
* @param shared whether the lock should be shared
* @return a future indicating the completion of this operation
*/
public io.vertx.core.Future lock(long position, long size, boolean shared) {
io.vertx.core.Future ret = delegate.lock(position, size, shared).map(val -> io.vertx.rxjava.core.file.AsyncFileLock.newInstance((io.vertx.core.file.AsyncFileLock)val));
return ret;
}
/**
* Acquire a lock on a portion of this file.
* @param position where the region starts
* @param size the size of the region
* @param shared whether the lock should be shared
* @return a future indicating the completion of this operation
*/
public rx.Single rxLock(long position, long size, boolean shared) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.lock(position, size, shared).onComplete(fut);
}));
}
/**
* Acquire a non-shared lock on the entire file.
*
* When the block
is called, the lock is already acquired, it will be released when the
* Future
returned by the block completes.
*
*
When the block
fails, the lock is released and the returned future is failed with the cause of the failure.
* @param block the code block called after lock acquisition
* @return the future returned by the block
*/
public io.vertx.core.Future withLock(java.util.function.Supplier> block) {
io.vertx.core.Future ret = delegate.withLock(new Supplier>() {
public io.vertx.core.Future get() {
io.vertx.core.Future ret = block.get();
return ret.map(val -> val);
}
}).map(val -> (T) val);
return ret;
}
/**
* Acquire a non-shared lock on the entire file.
*
* When the block
is called, the lock is already acquired, it will be released when the
* Future
returned by the block completes.
*
*
When the block
fails, the lock is released and the returned future is failed with the cause of the failure.
* @param block the code block called after lock acquisition
* @return the future returned by the block
*/
public rx.Single rxWithLock(java.util.function.Supplier> block) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.withLock(block).onComplete(fut);
}));
}
/**
* Acquire a lock on a portion of this file.
*
* When the block
is called, the lock is already acquired , it will be released when the
* Future
returned by the block completes.
*
*
When the block
fails, the lock is released and the returned future is failed with the cause of the failure.
* @param position where the region starts
* @param size the size of the region
* @param shared whether the lock should be shared
* @param block the code block called after lock acquisition
* @return the future returned by the block
*/
public io.vertx.core.Future withLock(long position, long size, boolean shared, java.util.function.Supplier> block) {
io.vertx.core.Future ret = delegate.withLock(position, size, shared, new Supplier>() {
public io.vertx.core.Future get() {
io.vertx.core.Future ret = block.get();
return ret.map(val -> val);
}
}).map(val -> (T) val);
return ret;
}
/**
* Acquire a lock on a portion of this file.
*
* When the block
is called, the lock is already acquired , it will be released when the
* Future
returned by the block completes.
*
*
When the block
fails, the lock is released and the returned future is failed with the cause of the failure.
* @param position where the region starts
* @param size the size of the region
* @param shared whether the lock should be shared
* @param block the code block called after lock acquisition
* @return the future returned by the block
*/
public rx.Single rxWithLock(long position, long size, boolean shared, java.util.function.Supplier> block) {
return Single.create(new SingleOnSubscribeAdapter<>(fut -> {
this.withLock(position, size, shared, block).onComplete(fut);
}));
}
public static AsyncFile newInstance(io.vertx.core.file.AsyncFile arg) {
return arg != null ? new AsyncFile(arg) : null;
}
}