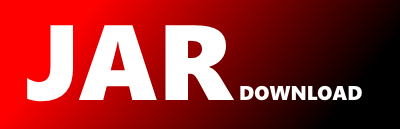
io.vertx.rxjava.ext.web.handler.APIKeyHandler Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.web.handler;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* An authentication handler that provides API Key support.
*
* API keys can be extracted from HTTP headers/query parameters/cookies
.
*
* By default this handler will extract the API key from an HTTP header named X-API-KEY
.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.handler.APIKeyHandler original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.web.handler.APIKeyHandler.class)
public class APIKeyHandler implements io.vertx.rxjava.ext.web.handler.AuthenticationHandler, Handler {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
APIKeyHandler that = (APIKeyHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new APIKeyHandler((io.vertx.ext.web.handler.APIKeyHandler) obj),
APIKeyHandler::getDelegate
);
private final io.vertx.ext.web.handler.APIKeyHandler delegate;
public APIKeyHandler(io.vertx.ext.web.handler.APIKeyHandler delegate) {
this.delegate = delegate;
}
public APIKeyHandler(Object delegate) {
this.delegate = (io.vertx.ext.web.handler.APIKeyHandler)delegate;
}
public io.vertx.ext.web.handler.APIKeyHandler getDelegate() {
return delegate;
}
/**
* Something has happened, so handle it.
* @param event the event to handle
*/
public void handle(io.vertx.rxjava.ext.web.RoutingContext event) {
delegate.handle(event.getDelegate());
}
/**
* Create an API Key authentication handler
* @param authProvider the auth provider to use
* @return the auth handler
*/
public static io.vertx.rxjava.ext.web.handler.APIKeyHandler create(io.vertx.rxjava.ext.auth.authentication.AuthenticationProvider authProvider) {
io.vertx.rxjava.ext.web.handler.APIKeyHandler ret = io.vertx.rxjava.ext.web.handler.APIKeyHandler.newInstance((io.vertx.ext.web.handler.APIKeyHandler)io.vertx.ext.web.handler.APIKeyHandler.create(authProvider.getDelegate()));
return ret;
}
/**
* Specify the source for the api key extraction as an HTTP header with the given name.
* @param headerName the header name containing the API key
* @return fluent self
*/
public io.vertx.rxjava.ext.web.handler.APIKeyHandler header(java.lang.String headerName) {
delegate.header(headerName);
return this;
}
/**
* Specify the source for the api key extraction as an HTTP query parameter with the given name.
* @param paramName the parameter name containing the API key
* @return fluent self
*/
public io.vertx.rxjava.ext.web.handler.APIKeyHandler parameter(java.lang.String paramName) {
delegate.parameter(paramName);
return this;
}
/**
* Specify the source for the api key extraction as an HTTP cookie with the given name.
* @param cookieName the cookie name containing the API key
* @return fluent self
*/
public io.vertx.rxjava.ext.web.handler.APIKeyHandler cookie(java.lang.String cookieName) {
delegate.cookie(cookieName);
return this;
}
/**
* Transform from user's token format to the AuthenticationHandler's format.
* @param tokenExtractor extract the token from the origin payload
* @return fluent self
*/
public io.vertx.rxjava.ext.web.handler.APIKeyHandler tokenExtractor(java.util.function.Function> tokenExtractor) {
delegate.tokenExtractor(new Function>() {
public io.vertx.core.Future apply(java.lang.String arg) {
io.vertx.core.Future ret = tokenExtractor.apply(arg);
return ret.map(val -> val);
}
});
return this;
}
public static APIKeyHandler newInstance(io.vertx.ext.web.handler.APIKeyHandler arg) {
return arg != null ? new APIKeyHandler(arg) : null;
}
}