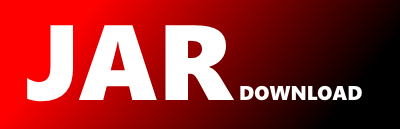
io.vertx.rxjava.redis.client.RedisAPI Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.redis.client;
import rx.Observable;
import rx.Single;
import io.vertx.rx.java.RxHelper;
import io.vertx.rx.java.WriteStreamSubscriber;
import io.vertx.rx.java.SingleOnSubscribeAdapter;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Auto generated Redis API client wrapper.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.redis.client.RedisAPI original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.redis.client.RedisAPI.class)
public class RedisAPI {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RedisAPI that = (RedisAPI) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new RedisAPI((io.vertx.redis.client.RedisAPI) obj),
RedisAPI::getDelegate
);
private final io.vertx.redis.client.RedisAPI delegate;
public RedisAPI(io.vertx.redis.client.RedisAPI delegate) {
this.delegate = delegate;
}
public RedisAPI(Object delegate) {
this.delegate = (io.vertx.redis.client.RedisAPI)delegate;
}
public io.vertx.redis.client.RedisAPI getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_3 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_4 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_5 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_6 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_7 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_8 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_9 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_10 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_11 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_12 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_13 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_14 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_15 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_16 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_17 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_18 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_19 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_20 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_21 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_22 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_23 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_24 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_25 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_26 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_27 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_28 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_29 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_30 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_31 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_32 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_33 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_34 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_35 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_36 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_37 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_38 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_39 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_40 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_41 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_42 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_43 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_44 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_45 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_46 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_47 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_48 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_49 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_50 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_51 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_52 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_53 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_54 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_55 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_56 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_57 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_58 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_59 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_60 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_61 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_62 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_63 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_64 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_65 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_66 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_67 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_68 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_69 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_70 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_71 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_72 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_73 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_74 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_75 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_76 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_77 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_78 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_79 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_80 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_81 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_82 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_83 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_84 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_85 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_86 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_87 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_88 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_89 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_90 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_91 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_92 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_93 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_94 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_95 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_96 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_97 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_98 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_99 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_100 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_101 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_102 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_103 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_104 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_105 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_106 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_107 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_108 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_109 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_110 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_111 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_112 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_113 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_114 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_115 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_116 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_117 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_118 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_119 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_120 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_121 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_122 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_123 = new TypeArg(o1 -> io.vertx.rxjava.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
private static final TypeArg