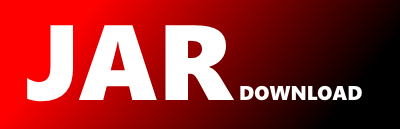
io.vertx.reactivex.MaybeHelper Maven / Gradle / Ivy
package io.vertx.reactivex;
import com.fasterxml.jackson.core.ObjectCodec;
import com.fasterxml.jackson.core.type.TypeReference;
import io.reactivex.Maybe;
import io.reactivex.MaybeObserver;
import io.reactivex.MaybeTransformer;
import io.reactivex.Single;
import io.reactivex.annotations.NonNull;
import io.reactivex.disposables.Disposable;
import io.vertx.core.AsyncResult;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.Promise;
import io.vertx.core.buffer.Buffer;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.MaybeUnmarshaller;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* @author Julien Viet
*/
public class MaybeHelper {
/**
* Returns a {@link Maybe} that, when subscribed, uses the provided {@code handler} to adapt a callback-based asynchronous method.
*
* For example:
*
{@code
* io.vertx.core.Vertx vertx = Vertx.vertx();
* Maybe blockingMethodResult = MaybeHelper.toMaybe(handler -> vertx.executeBlocking(fut -> fut.complete(invokeBlocking()), handler));
* }
*
* This is useful when using RxJava without the Vert.x Rxified API or your own asynchronous methods.
*
* @param handler the code executed when the returned {@link Maybe} is subscribed
*/
public static Maybe toMaybe(Consumer>> handler) {
return AsyncResultMaybe.toMaybe(handler);
}
/**
* Adapts an Vert.x {@code Handler>} to an RxJava2 {@link MaybeObserver}.
*
* The returned observer can be subscribed to an {@link Maybe#subscribe(MaybeObserver)}.
*
* @param handler the handler to adapt
* @return the observer
*/
public static MaybeObserver toObserver(Handler> handler) {
AtomicBoolean completed = new AtomicBoolean();
return new MaybeObserver() {
@Override
public void onSubscribe(@NonNull Disposable d) {
}
@Override
public void onComplete() {
if (completed.compareAndSet(false, true)) {
handler.handle(io.vertx.core.Future.succeededFuture());
}
}
@Override
public void onSuccess(@NonNull T item) {
if (completed.compareAndSet(false, true)) {
handler.handle(io.vertx.core.Future.succeededFuture(item));
}
}
@Override
public void onError(Throwable error) {
if (completed.compareAndSet(false, true)) {
handler.handle(io.vertx.core.Future.failedFuture(error));
}
}
};
}
/**
* Adapts an RxJava2 {@code Maybe} to a Vert.x {@link Future}.
*
* The maybe will be immediately subscribed and the returned future will
* be updated with the result of the single.
*
* @param maybe the single to adapt
* @return the future
*/
public static Future toFuture(Maybe maybe) {
Promise promise = Promise.promise();
maybe.subscribe(promise::complete, promise::fail, promise::complete);
return promise.future();
}
/**
* Like {@link MaybeHelper#toFuture(Maybe)} but with an {@code adapter} of the result.
*/
public static Future toFuture(Maybe maybe, Function adapter) {
return toFuture(maybe.map(adapter::apply));
}
public static MaybeTransformer unmarshaller(Class mappedType) {
return new MaybeUnmarshaller<>(java.util.function.Function.identity(), mappedType);
}
public static MaybeTransformer unmarshaller(TypeReference mappedTypeRef) {
return new MaybeUnmarshaller<>(java.util.function.Function.identity(), mappedTypeRef);
}
public static MaybeTransformer unmarshaller(Class mappedType, ObjectCodec mapper) {
return new MaybeUnmarshaller<>(java.util.function.Function.identity(), mappedType, mapper);
}
public static MaybeTransformer unmarshaller(TypeReference mappedTypeRef, ObjectCodec mapper) {
return new MaybeUnmarshaller<>(java.util.function.Function.identity(), mappedTypeRef, mapper);
}
}