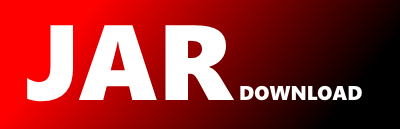
io.vertx.reactivex.core.http.HttpClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.core.http;
import io.vertx.reactivex.RxHelper;
import io.vertx.reactivex.ObservableHelper;
import io.vertx.reactivex.FlowableHelper;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.AsyncResultSingle;
import io.vertx.reactivex.impl.AsyncResultCompletable;
import io.vertx.reactivex.WriteStreamObserver;
import io.vertx.reactivex.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* The API to interacts with an HTTP server.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.HttpClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.HttpClient.class)
public interface HttpClient {
io.vertx.core.http.HttpClient getDelegate();
/**
* Create an HTTP request to send to the server with the default host and port of the client.
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request();
/**
* Create an HTTP request to send to the server with the default host and port of the client.
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest();
/**
* Create an HTTP request to send to the server.
* @param options the request options
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.RequestOptions options);
/**
* Create an HTTP request to send to the server.
* @param options the request options
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.RequestOptions options);
/**
* Create an HTTP request to send to the server at the host
and port
.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.HttpMethod method, int port, java.lang.String host, java.lang.String requestURI);
/**
* Create an HTTP request to send to the server at the host
and port
.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.HttpMethod method, int port, java.lang.String host, java.lang.String requestURI);
/**
* Create an HTTP request to send to the server at the host
and default port.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.HttpMethod method, java.lang.String host, java.lang.String requestURI);
/**
* Create an HTTP request to send to the server at the host
and default port.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.HttpMethod method, java.lang.String host, java.lang.String requestURI);
/**
* Create an HTTP request to send to the server at the default host and port.
* @param method the HTTP method
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.HttpMethod method, java.lang.String requestURI);
/**
* Create an HTTP request to send to the server at the default host and port.
* @param method the HTTP method
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.HttpMethod method, java.lang.String requestURI);
/**
* Shutdown with a 30 seconds timeout (shutdown(30, TimeUnit.SECONDS)
).
* @return a future completed when shutdown has completed
*/
public io.vertx.core.Future shutdown();
/**
* Shutdown with a 30 seconds timeout (shutdown(30, TimeUnit.SECONDS)
).
* @return a future completed when shutdown has completed
*/
public io.reactivex.Completable rxShutdown();
/**
* Close immediately (shutdown(0, TimeUnit.SECONDS
).
* @return a future notified when the client is closed
*/
public io.vertx.core.Future close();
/**
* Close immediately (shutdown(0, TimeUnit.SECONDS
).
* @return a future notified when the client is closed
*/
public io.reactivex.Completable rxClose();
/**
* Initiate the client shutdown sequence.
*
* Connections are taken out of service and closed when all inflight requests are processed, client connection are
* immediately removed from the pool. When all connections are closed the client is closed. When the timeout
* expires, all unclosed connections are immediately closed.
*
*
* - HTTP/2 connections will send a go away frame immediately to signal the other side the connection will close
* - HTTP/1.x client connection will be closed after the current response is received
*
* @param timeout the amount of time after which all resources are forcibly closed
* @param unit the of the timeout
* @return a future notified when the client is closed
*/
public io.vertx.core.Future shutdown(long timeout, java.util.concurrent.TimeUnit unit);
/**
* Initiate the client shutdown sequence.
*
* Connections are taken out of service and closed when all inflight requests are processed, client connection are
* immediately removed from the pool. When all connections are closed the client is closed. When the timeout
* expires, all unclosed connections are immediately closed.
*
*
* - HTTP/2 connections will send a go away frame immediately to signal the other side the connection will close
* - HTTP/1.x client connection will be closed after the current response is received
*
* @param timeout the amount of time after which all resources are forcibly closed
* @param unit the of the timeout
* @return a future notified when the client is closed
*/
public io.reactivex.Completable rxShutdown(long timeout, java.util.concurrent.TimeUnit unit);
public static HttpClient newInstance(io.vertx.core.http.HttpClient arg) {
return arg != null ? new HttpClientImpl(arg) : null;
}
}
class HttpClientImpl implements HttpClient {
private final io.vertx.core.http.HttpClient delegate;
public HttpClientImpl(io.vertx.core.http.HttpClient delegate) {
this.delegate = delegate;
}
public HttpClientImpl(Object delegate) {
this.delegate = (io.vertx.core.http.HttpClient)delegate;
}
public io.vertx.core.http.HttpClient getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_3 = new TypeArg(o1 -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_4 = new TypeArg(o1 -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)o1), o1 -> o1.getDelegate());
/**
* Create an HTTP request to send to the server with the default host and port of the client.
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request() {
io.vertx.core.Future ret = delegate.request().map(val -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)val));
return ret;
}
/**
* Create an HTTP request to send to the server with the default host and port of the client.
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest() {
return AsyncResultSingle.toSingle($handler -> {
this.request().onComplete($handler);
});
}
/**
* Create an HTTP request to send to the server.
* @param options the request options
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.RequestOptions options) {
io.vertx.core.Future ret = delegate.request(options).map(val -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)val));
return ret;
}
/**
* Create an HTTP request to send to the server.
* @param options the request options
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.RequestOptions options) {
return AsyncResultSingle.toSingle($handler -> {
this.request(options).onComplete($handler);
});
}
/**
* Create an HTTP request to send to the server at the host
and port
.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.HttpMethod method, int port, java.lang.String host, java.lang.String requestURI) {
io.vertx.core.Future ret = delegate.request(method, port, host, requestURI).map(val -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)val));
return ret;
}
/**
* Create an HTTP request to send to the server at the host
and port
.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.HttpMethod method, int port, java.lang.String host, java.lang.String requestURI) {
return AsyncResultSingle.toSingle($handler -> {
this.request(method, port, host, requestURI).onComplete($handler);
});
}
/**
* Create an HTTP request to send to the server at the host
and default port.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.HttpMethod method, java.lang.String host, java.lang.String requestURI) {
io.vertx.core.Future ret = delegate.request(method, host, requestURI).map(val -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)val));
return ret;
}
/**
* Create an HTTP request to send to the server at the host
and default port.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.HttpMethod method, java.lang.String host, java.lang.String requestURI) {
return AsyncResultSingle.toSingle($handler -> {
this.request(method, host, requestURI).onComplete($handler);
});
}
/**
* Create an HTTP request to send to the server at the default host and port.
* @param method the HTTP method
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.vertx.core.Future request(io.vertx.core.http.HttpMethod method, java.lang.String requestURI) {
io.vertx.core.Future ret = delegate.request(method, requestURI).map(val -> io.vertx.reactivex.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)val));
return ret;
}
/**
* Create an HTTP request to send to the server at the default host and port.
* @param method the HTTP method
* @param requestURI the relative URI
* @return a future notified when the request is ready to be sent
*/
public io.reactivex.Single rxRequest(io.vertx.core.http.HttpMethod method, java.lang.String requestURI) {
return AsyncResultSingle.toSingle($handler -> {
this.request(method, requestURI).onComplete($handler);
});
}
/**
* Shutdown with a 30 seconds timeout (shutdown(30, TimeUnit.SECONDS)
).
* @return a future completed when shutdown has completed
*/
public io.vertx.core.Future shutdown() {
io.vertx.core.Future ret = delegate.shutdown().map(val -> val);
return ret;
}
/**
* Shutdown with a 30 seconds timeout (shutdown(30, TimeUnit.SECONDS)
).
* @return a future completed when shutdown has completed
*/
public io.reactivex.Completable rxShutdown() {
return AsyncResultCompletable.toCompletable($handler -> {
this.shutdown().onComplete($handler);
});
}
/**
* Close immediately (shutdown(0, TimeUnit.SECONDS
).
* @return a future notified when the client is closed
*/
public io.vertx.core.Future close() {
io.vertx.core.Future ret = delegate.close().map(val -> val);
return ret;
}
/**
* Close immediately (shutdown(0, TimeUnit.SECONDS
).
* @return a future notified when the client is closed
*/
public io.reactivex.Completable rxClose() {
return AsyncResultCompletable.toCompletable($handler -> {
this.close().onComplete($handler);
});
}
/**
* Initiate the client shutdown sequence.
*
* Connections are taken out of service and closed when all inflight requests are processed, client connection are
* immediately removed from the pool. When all connections are closed the client is closed. When the timeout
* expires, all unclosed connections are immediately closed.
*
*
* - HTTP/2 connections will send a go away frame immediately to signal the other side the connection will close
* - HTTP/1.x client connection will be closed after the current response is received
*
* @param timeout the amount of time after which all resources are forcibly closed
* @param unit the of the timeout
* @return a future notified when the client is closed
*/
public io.vertx.core.Future shutdown(long timeout, java.util.concurrent.TimeUnit unit) {
io.vertx.core.Future ret = delegate.shutdown(timeout, unit).map(val -> val);
return ret;
}
/**
* Initiate the client shutdown sequence.
*
* Connections are taken out of service and closed when all inflight requests are processed, client connection are
* immediately removed from the pool. When all connections are closed the client is closed. When the timeout
* expires, all unclosed connections are immediately closed.
*
*
* - HTTP/2 connections will send a go away frame immediately to signal the other side the connection will close
* - HTTP/1.x client connection will be closed after the current response is received
*
* @param timeout the amount of time after which all resources are forcibly closed
* @param unit the of the timeout
* @return a future notified when the client is closed
*/
public io.reactivex.Completable rxShutdown(long timeout, java.util.concurrent.TimeUnit unit) {
return AsyncResultCompletable.toCompletable($handler -> {
this.shutdown(timeout, unit).onComplete($handler);
});
}
}