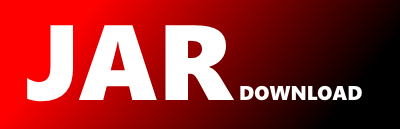
io.vertx.reactivex.core.http.HttpServerRequest Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.core.http;
import io.vertx.reactivex.RxHelper;
import io.vertx.reactivex.ObservableHelper;
import io.vertx.reactivex.FlowableHelper;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.AsyncResultSingle;
import io.vertx.reactivex.impl.AsyncResultCompletable;
import io.vertx.reactivex.WriteStreamObserver;
import io.vertx.reactivex.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents a server-side HTTP request.
*
* Instances are created for each request and passed to the user via a handler.
*
* Each instance of this class is associated with a corresponding {@link io.vertx.reactivex.core.http.HttpServerResponse} instance via
* {@link io.vertx.reactivex.core.http.HttpServerRequest#response}.
* It implements {@link io.vertx.reactivex.core.streams.ReadStream} so it can be used with
* {@link io.vertx.reactivex.core.streams.Pipe} to pipe data with flow control.
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.HttpServerRequest original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.HttpServerRequest.class)
public class HttpServerRequest implements io.vertx.reactivex.core.streams.ReadStream {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpServerRequest that = (HttpServerRequest) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpServerRequest((io.vertx.core.http.HttpServerRequest) obj),
HttpServerRequest::getDelegate
);
private final io.vertx.core.http.HttpServerRequest delegate;
public HttpServerRequest(io.vertx.core.http.HttpServerRequest delegate) {
this.delegate = delegate;
}
public HttpServerRequest(Object delegate) {
this.delegate = (io.vertx.core.http.HttpServerRequest)delegate;
}
public io.vertx.core.http.HttpServerRequest getDelegate() {
return delegate;
}
private io.reactivex.Observable observable;
private io.reactivex.Flowable flowable;
public synchronized io.reactivex.Observable toObservable() {
if (observable == null) {
observable = ObservableHelper.toObservable(this.getDelegate());
}
return observable;
}
public synchronized io.reactivex.Flowable toFlowable() {
if (flowable == null) {
flowable = FlowableHelper.toFlowable(this.getDelegate());
}
return flowable;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.reactivex.core.net.NetSocket.newInstance((io.vertx.core.net.NetSocket)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.reactivex.core.http.ServerWebSocket.newInstance((io.vertx.core.http.ServerWebSocket)o1), o1 -> o1.getDelegate());
/**
* Pause this stream and return a to transfer the elements of this stream to a destination .
*
* The stream will be resumed when the pipe will be wired to a WriteStream
.
* @return a pipe
*/
public io.vertx.reactivex.core.streams.Pipe pipe() {
io.vertx.reactivex.core.streams.Pipe ret = io.vertx.reactivex.core.streams.Pipe.newInstance((io.vertx.core.streams.Pipe)delegate.pipe(), TypeArg.unknown());
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
* @param dst the destination write stream
* @return a future notified when the write stream will be ended with the outcome
*/
public io.vertx.core.Future pipeTo(io.vertx.reactivex.core.streams.WriteStream dst) {
io.vertx.core.Future ret = delegate.pipeTo(dst.getDelegate()).map(val -> val);
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
* @param dst the destination write stream
* @return a future notified when the write stream will be ended with the outcome
*/
public io.reactivex.Completable rxPipeTo(io.vertx.reactivex.core.streams.WriteStream dst) {
return AsyncResultCompletable.toCompletable($handler -> {
this.pipeTo(dst).onComplete($handler);
});
}
public io.vertx.reactivex.core.http.HttpServerRequest exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.reactivex.core.http.HttpServerRequest handler(io.vertx.core.Handler handler) {
delegate.handler(handler);
return this;
}
public io.vertx.reactivex.core.http.HttpServerRequest pause() {
delegate.pause();
return this;
}
public io.vertx.reactivex.core.http.HttpServerRequest resume() {
delegate.resume();
return this;
}
public io.vertx.reactivex.core.http.HttpServerRequest fetch(long amount) {
delegate.fetch(amount);
return this;
}
public io.vertx.reactivex.core.http.HttpServerRequest endHandler(io.vertx.core.Handler endHandler) {
delegate.endHandler(endHandler);
return this;
}
/**
* @return the HTTP version of the request
*/
public io.vertx.core.http.HttpVersion version() {
io.vertx.core.http.HttpVersion ret = delegate.version();
return ret;
}
/**
* @return the HTTP method for the request.
*/
public io.vertx.core.http.HttpMethod method() {
io.vertx.core.http.HttpMethod ret = delegate.method();
return ret;
}
/**
* @return true if this {@link io.vertx.reactivex.core.net.NetSocket} is encrypted via SSL/TLS
*/
public boolean isSSL() {
boolean ret = delegate.isSSL();
return ret;
}
/**
* @return the scheme of the request
*/
public java.lang.String scheme() {
java.lang.String ret = delegate.scheme();
return ret;
}
/**
* @return the URI of the request. This is usually a relative URI
*/
public java.lang.String uri() {
java.lang.String ret = delegate.uri();
return ret;
}
/**
* @return The path part of the uri. For example /somepath/somemorepath/someresource.foo
*/
public java.lang.String path() {
java.lang.String ret = delegate.path();
return ret;
}
/**
* @return the query part of the uri. For example someparam=32&someotherparam=x
*/
public java.lang.String query() {
java.lang.String ret = delegate.query();
return ret;
}
/**
* @return the request authority. For HTTP/2 the pseudo header is returned, for HTTP/1.x the header is returned or null
when no such header is present. When the authority string does not carry a port, the returns -1
to indicate the scheme port is prevalent.
*/
public io.vertx.core.net.HostAndPort authority() {
io.vertx.core.net.HostAndPort ret = delegate.authority();
return ret;
}
/**
* @return the total number of bytes read for the body of the request.
*/
public long bytesRead() {
long ret = delegate.bytesRead();
return ret;
}
/**
* @return the response. Each instance of this class has an {@link io.vertx.reactivex.core.http.HttpServerResponse} instance attached to it. This is used to send the response back to the client.
*/
public io.vertx.reactivex.core.http.HttpServerResponse response() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.reactivex.core.http.HttpServerResponse ret = io.vertx.reactivex.core.http.HttpServerResponse.newInstance((io.vertx.core.http.HttpServerResponse)delegate.response());
cached_0 = ret;
return ret;
}
/**
* @return the headers in the request.
*/
public io.vertx.core.MultiMap headers() {
if (cached_1 != null) {
return cached_1;
}
io.vertx.core.MultiMap ret = delegate.headers();
cached_1 = ret;
return ret;
}
/**
* Return the first header value with the specified name
* @param headerName the header name
* @return the header value
*/
public java.lang.String getHeader(java.lang.String headerName) {
java.lang.String ret = delegate.getHeader(headerName);
return ret;
}
/**
* Override the charset to use for decoding the query parameter map, when none is set, UTF8
is used.
* @param charset the charset to use for decoding query params
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.core.http.HttpServerRequest setParamsCharset(java.lang.String charset) {
delegate.setParamsCharset(charset);
return this;
}
/**
* @return the charset used for decoding query parameters
*/
public java.lang.String getParamsCharset() {
java.lang.String ret = delegate.getParamsCharset();
return ret;
}
/**
* @return the query parameters in the request
*/
public io.vertx.core.MultiMap params() {
if (cached_2 != null) {
return cached_2;
}
io.vertx.core.MultiMap ret = delegate.params();
cached_2 = ret;
return ret;
}
/**
* @param semicolonIsNormalChar whether semicolon is treated as a normal character or a query parameter separator
* @return the query parameters in the request
*/
public io.vertx.core.MultiMap params(boolean semicolonIsNormalChar) {
io.vertx.core.MultiMap ret = delegate.params(semicolonIsNormalChar);
return ret;
}
/**
* Return the first param value with the specified name
* @param paramName the param name
* @return the param value
*/
public java.lang.String getParam(java.lang.String paramName) {
java.lang.String ret = delegate.getParam(paramName);
return ret;
}
/**
* Return the first param value with the specified name or defaultValue
when the query param is not present
* @param paramName the param name
* @param defaultValue the default value, must be non-null
* @return the param value or defaultValue
when not present
*/
public java.lang.String getParam(java.lang.String paramName, java.lang.String defaultValue) {
java.lang.String ret = delegate.getParam(paramName, defaultValue);
return ret;
}
/**
* @return the remote address for this connection, possibly null
(e.g a server bound on a domain socket). If useProxyProtocol
is set to true
, the address returned will be of the actual connecting client.
*/
public io.vertx.core.net.SocketAddress remoteAddress() {
if (cached_3 != null) {
return cached_3;
}
io.vertx.core.net.SocketAddress ret = delegate.remoteAddress();
cached_3 = ret;
return ret;
}
/**
* @return the local address for this connection, possibly null
(e.g a server bound on a domain socket) If useProxyProtocol
is set to true
, the address returned will be of the proxy.
*/
public io.vertx.core.net.SocketAddress localAddress() {
if (cached_4 != null) {
return cached_4;
}
io.vertx.core.net.SocketAddress ret = delegate.localAddress();
cached_4 = ret;
return ret;
}
/**
* @return the absolute URI corresponding to the HTTP request
*/
public java.lang.String absoluteURI() {
java.lang.String ret = delegate.absoluteURI();
return ret;
}
/**
* Convenience method for receiving the entire request body in one piece.
*
* This saves the user having to manually setting a data and end handler and append the chunks of the body until
* the whole body received. Don't use this if your request body is large - you could potentially run out of RAM.
* @param bodyHandler This handler will be called after all the body has been received
* @return
*/
public io.vertx.reactivex.core.http.HttpServerRequest bodyHandler(io.vertx.core.Handler bodyHandler) {
delegate.bodyHandler(bodyHandler);
return this;
}
/**
* Convenience method for receiving the entire request body in one piece.
*
* This saves you having to manually set a dataHandler and an endHandler and append the chunks of the body until
* the whole body received. Don't use this if your request body is large - you could potentially run out of RAM.
* @return a future completed with the body result
*/
public io.vertx.core.Future body() {
io.vertx.core.Future ret = delegate.body().map(val -> val);
return ret;
}
/**
* Convenience method for receiving the entire request body in one piece.
*
* This saves you having to manually set a dataHandler and an endHandler and append the chunks of the body until
* the whole body received. Don't use this if your request body is large - you could potentially run out of RAM.
* @return a future completed with the body result
*/
public io.reactivex.Single rxBody() {
return AsyncResultSingle.toSingle($handler -> {
this.body().onComplete($handler);
});
}
/**
* Returns a future signaling when the request has been fully received successfully or failed.
* @return a future completed with the body result
*/
public io.vertx.core.Future end() {
io.vertx.core.Future ret = delegate.end().map(val -> val);
return ret;
}
/**
* Returns a future signaling when the request has been fully received successfully or failed.
* @return a future completed with the body result
*/
public io.reactivex.Completable rxEnd() {
return AsyncResultCompletable.toCompletable($handler -> {
this.end().onComplete($handler);
});
}
/**
* Establish a TCP tunnel with the client.
*
* This must be called only for CONNECT
HTTP method or for HTTP connection upgrade, before any response is sent.
*
*
Calling this sends a 200
response for a CONNECT
or a 101
for a connection upgrade wit
* no content-length
header set and then provides the NetSocket
for handling the created tunnel.
* Any HTTP header set on the response before calling this method will be sent.
*
*
* server.requestHandler(req -> {
* if (req.method() == HttpMethod.CONNECT) {
* // Send a 200 response to accept the connect
* NetSocket socket = req.netSocket();
* socket.handler(buff -> {
* socket.write(buff);
* });
* }
* ...
* });
*
* @return a future notified with the upgraded socket
*/
public io.vertx.core.Future toNetSocket() {
io.vertx.core.Future ret = delegate.toNetSocket().map(val -> io.vertx.reactivex.core.net.NetSocket.newInstance((io.vertx.core.net.NetSocket)val));
return ret;
}
/**
* Establish a TCP tunnel with the client.
*
* This must be called only for CONNECT
HTTP method or for HTTP connection upgrade, before any response is sent.
*
*
Calling this sends a 200
response for a CONNECT
or a 101
for a connection upgrade wit
* no content-length
header set and then provides the NetSocket
for handling the created tunnel.
* Any HTTP header set on the response before calling this method will be sent.
*
*
* server.requestHandler(req -> {
* if (req.method() == HttpMethod.CONNECT) {
* // Send a 200 response to accept the connect
* NetSocket socket = req.netSocket();
* socket.handler(buff -> {
* socket.write(buff);
* });
* }
* ...
* });
*
* @return a future notified with the upgraded socket
*/
public io.reactivex.Single rxToNetSocket() {
return AsyncResultSingle.toSingle($handler -> {
this.toNetSocket().onComplete($handler);
});
}
/**
* Call this with true if you are expecting a multi-part body to be submitted in the request.
* This must be called before the body of the request has been received
* @param expect true - if you are expecting a multi-part body
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.core.http.HttpServerRequest setExpectMultipart(boolean expect) {
delegate.setExpectMultipart(expect);
return this;
}
/**
* @return true if we are expecting a multi-part body for this request. See {@link io.vertx.reactivex.core.http.HttpServerRequest#setExpectMultipart}.
*/
public boolean isExpectMultipart() {
boolean ret = delegate.isExpectMultipart();
return ret;
}
/**
* Set an upload handler. The handler will get notified once a new file upload was received to allow you to deal
* with the file upload.
* @param uploadHandler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.core.http.HttpServerRequest uploadHandler(io.vertx.core.Handler uploadHandler) {
delegate.uploadHandler(new io.vertx.lang.rx.DelegatingHandler<>(uploadHandler, event -> io.vertx.reactivex.core.http.HttpServerFileUpload.newInstance((io.vertx.core.http.HttpServerFileUpload)event)));
return this;
}
/**
* Returns a map of all form attributes in the request.
*
* Be aware that the attributes will only be available after the whole body has been received, i.e. after
* the request end handler has been called.
*
* {@link io.vertx.reactivex.core.http.HttpServerRequest#setExpectMultipart} must be called first before trying to get the form attributes.
* @return the form attributes
*/
public io.vertx.core.MultiMap formAttributes() {
if (cached_5 != null) {
return cached_5;
}
io.vertx.core.MultiMap ret = delegate.formAttributes();
cached_5 = ret;
return ret;
}
/**
* Return the first form attribute value with the specified name
* @param attributeName the attribute name
* @return the attribute value
*/
public java.lang.String getFormAttribute(java.lang.String attributeName) {
java.lang.String ret = delegate.getFormAttribute(attributeName);
return ret;
}
/**
* @return the id of the stream of this request, when it is not yet determined, i.e the request has not been yet sent or it is not supported HTTP/1.x
*/
public int streamId() {
if (cached_6 != null) {
return cached_6;
}
int ret = delegate.streamId();
cached_6 = ret;
return ret;
}
/**
* @return whether this request can be upgraded to a WebSocket, implying it uses HTTP/1.x and presents the correct characteristics for a proper upgrade.
*/
public boolean canUpgradeToWebSocket() {
boolean ret = delegate.canUpgradeToWebSocket();
return ret;
}
/**
* Upgrade the connection of the current request to a WebSocket.
*
* This is an alternative way of handling WebSockets and can only be used if no WebSocket handler is set on the
* HttpServer
, and can only be used during the upgrade request during the WebSocket handshake.
*
*
Both {@link io.vertx.reactivex.core.http.HttpServerRequest#handler} and {@link io.vertx.reactivex.core.http.HttpServerRequest#endHandler} will be set to get the full body of the
* request that is necessary to perform the WebSocket handshake.
*
*
If you need to do an asynchronous upgrade, i.e not performed immediately in your request handler,
* you need to {@link io.vertx.reactivex.core.http.HttpServerRequest#pause} the request in order to not lose HTTP events necessary to upgrade the
* request.
* @return a future notified with the upgraded WebSocket
*/
public io.vertx.core.Future toWebSocket() {
io.vertx.core.Future ret = delegate.toWebSocket().map(val -> io.vertx.reactivex.core.http.ServerWebSocket.newInstance((io.vertx.core.http.ServerWebSocket)val));
return ret;
}
/**
* Upgrade the connection of the current request to a WebSocket.
*
* This is an alternative way of handling WebSockets and can only be used if no WebSocket handler is set on the
* HttpServer
, and can only be used during the upgrade request during the WebSocket handshake.
*
*
Both {@link io.vertx.reactivex.core.http.HttpServerRequest#handler} and {@link io.vertx.reactivex.core.http.HttpServerRequest#endHandler} will be set to get the full body of the
* request that is necessary to perform the WebSocket handshake.
*
*
If you need to do an asynchronous upgrade, i.e not performed immediately in your request handler,
* you need to {@link io.vertx.reactivex.core.http.HttpServerRequest#pause} the request in order to not lose HTTP events necessary to upgrade the
* request.
* @return a future notified with the upgraded WebSocket
*/
public io.reactivex.Single rxToWebSocket() {
return AsyncResultSingle.toSingle($handler -> {
this.toWebSocket().onComplete($handler);
});
}
/**
* Has the request ended? I.e. has the entire request, including the body been read?
* @return true if ended
*/
public boolean isEnded() {
boolean ret = delegate.isEnded();
return ret;
}
/**
* Set a custom frame handler. The handler will get notified when the http stream receives an custom HTTP/2
* frame. HTTP/2 permits extension of the protocol.
* @param handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.core.http.HttpServerRequest customFrameHandler(io.vertx.core.Handler handler) {
delegate.customFrameHandler(handler);
return this;
}
/**
* @return the {@link io.vertx.reactivex.core.http.HttpConnection} associated with this request
*/
public io.vertx.reactivex.core.http.HttpConnection connection() {
if (cached_7 != null) {
return cached_7;
}
io.vertx.reactivex.core.http.HttpConnection ret = io.vertx.reactivex.core.http.HttpConnection.newInstance((io.vertx.core.http.HttpConnection)delegate.connection());
cached_7 = ret;
return ret;
}
/**
* @return the priority of the associated HTTP/2 stream for HTTP/2 otherwise null
*/
public io.vertx.core.http.StreamPriority streamPriority() {
io.vertx.core.http.StreamPriority ret = delegate.streamPriority();
return ret;
}
/**
* Set an handler for stream priority changes
*
* This is not implemented for HTTP/1.x.
* @param handler the handler to be called when stream priority changes
* @return
*/
public io.vertx.reactivex.core.http.HttpServerRequest streamPriorityHandler(io.vertx.core.Handler handler) {
delegate.streamPriorityHandler(handler);
return this;
}
/**
* Get the cookie with the specified name.
*
* NOTE: this will return just the 1st {@link io.vertx.core.http.Cookie} that matches the given name, to get all cookies for this name
* see: {@link io.vertx.reactivex.core.http.HttpServerRequest#cookies}
* @param name the cookie name
* @return the cookie or null
if not found.
*/
public io.vertx.core.http.Cookie getCookie(java.lang.String name) {
io.vertx.core.http.Cookie ret = delegate.getCookie(name);
return ret;
}
/**
* Get the cookie with the specified
.
* @param name the cookie name
* @param domain the cookie domain
* @param path the cookie path
* @return the cookie or null
if not found.
*/
public io.vertx.core.http.Cookie getCookie(java.lang.String name, java.lang.String domain, java.lang.String path) {
io.vertx.core.http.Cookie ret = delegate.getCookie(name, domain, path);
return ret;
}
/**
* @return the number of cookies in the cookie jar.
*/
public int cookieCount() {
int ret = delegate.cookieCount();
return ret;
}
/**
* @return a map of all the cookies.
*/
@Deprecated()
public java.util.Map cookieMap() {
java.util.Map ret = delegate.cookieMap();
return ret;
}
/**
* Returns a read only set of parsed cookies that match the given name, or an empty set. Several cookies may share the
* same name but have different keys. A cookie is unique by its
tuple.
*
* The set entries are references to the request original set. This means that performing property changes in the
* cookie objects will affect the original object too.
*
* NOTE: the returned is read-only. This means any attempt to modify (add or remove to the set), will
* throw {@link java.lang.UnsupportedOperationException}.
* @param name the name to be matches
* @return the matching cookies or empty set
*/
public java.util.Set cookies(java.lang.String name) {
java.util.Set ret = delegate.cookies(name);
return ret;
}
/**
* Returns a modifiable set of parsed cookies from the COOKIE
header. Several cookies may share the
* same name but have different keys. A cookie is unique by its
tuple.
*
* Request cookies are directly linked to response cookies. Any modification to a cookie object in the returned set
* will mark the cookie to be included in the HTTP response. Removing a cookie from the set, will also mean that it
* will be removed from the response, regardless if it was modified or not.
* @return a set with all cookies in the cookie jar.
*/
public java.util.Set cookies() {
java.util.Set ret = delegate.cookies();
return ret;
}
/**
* Marks this request as being routed to the given route. This is purely informational and is
* being provided to metrics.
* @param route The route this request has been routed to.
* @return
*/
public io.vertx.reactivex.core.http.HttpServerRequest routed(java.lang.String route) {
delegate.routed(route);
return this;
}
/**
* Return the first header value with the specified name
* @param headerName the header name
* @return the header value
*/
public java.lang.String getHeader(java.lang.CharSequence headerName) {
java.lang.String ret = delegate.getHeader(headerName);
return ret;
}
/**
* @return SSLSession associated with the underlying socket. Returns null if connection is not SSL.
*/
public javax.net.ssl.SSLSession sslSession() {
javax.net.ssl.SSLSession ret = delegate.sslSession();
return ret;
}
private io.vertx.reactivex.core.http.HttpServerResponse cached_0;
private io.vertx.core.MultiMap cached_1;
private io.vertx.core.MultiMap cached_2;
private io.vertx.core.net.SocketAddress cached_3;
private io.vertx.core.net.SocketAddress cached_4;
private io.vertx.core.MultiMap cached_5;
private java.lang.Integer cached_6;
private io.vertx.reactivex.core.http.HttpConnection cached_7;
public static HttpServerRequest newInstance(io.vertx.core.http.HttpServerRequest arg) {
return arg != null ? new HttpServerRequest(arg) : null;
}
}