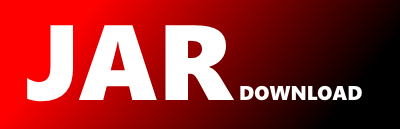
io.vertx.reactivex.ext.auth.webauthn4j.CredentialStorage Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.ext.auth.webauthn4j;
import io.vertx.reactivex.RxHelper;
import io.vertx.reactivex.ObservableHelper;
import io.vertx.reactivex.FlowableHelper;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.AsyncResultSingle;
import io.vertx.reactivex.impl.AsyncResultCompletable;
import io.vertx.reactivex.WriteStreamObserver;
import io.vertx.reactivex.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Used to represent persistent storage of credentials, this gives you a way to abstract
* how you want to store them (in memory, database, other).
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.webauthn4j.CredentialStorage original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.auth.webauthn4j.CredentialStorage.class)
public class CredentialStorage {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
CredentialStorage that = (CredentialStorage) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new CredentialStorage((io.vertx.ext.auth.webauthn4j.CredentialStorage) obj),
CredentialStorage::getDelegate
);
private final io.vertx.ext.auth.webauthn4j.CredentialStorage delegate;
public CredentialStorage(io.vertx.ext.auth.webauthn4j.CredentialStorage delegate) {
this.delegate = delegate;
}
public CredentialStorage(Object delegate) {
this.delegate = (io.vertx.ext.auth.webauthn4j.CredentialStorage)delegate;
}
public io.vertx.ext.auth.webauthn4j.CredentialStorage getDelegate() {
return delegate;
}
/**
* Finds an existing list of credentials for a given user name and credential ID.
*
* Both the userName
and credentialId
parameters are optional
* but at least one of them must be specified. If only one is specified, it must match
* the results. If both are specified, then both must match the result at the same time.
*
* This may return more than one element if one of the parameters is not set. If both are
* set, then the returned list of credentials must contain at maximum 1 element.
* If the user is not known or does not allow any authenticator, returns an empty list.
* @param userName the user name (may be null
, but must match if specified)
* @param credentialId the credential ID (must match the results)
* @return the list of authenticators allowed for the given userName and credential ID, or an empty list.
*/
public io.vertx.core.Future> find(java.lang.String userName, java.lang.String credentialId) {
io.vertx.core.Future> ret = delegate.find(userName, credentialId).map(val -> val);
return ret;
}
/**
* Finds an existing list of credentials for a given user name and credential ID.
*
* Both the userName
and credentialId
parameters are optional
* but at least one of them must be specified. If only one is specified, it must match
* the results. If both are specified, then both must match the result at the same time.
*
* This may return more than one element if one of the parameters is not set. If both are
* set, then the returned list of credentials must contain at maximum 1 element.
* If the user is not known or does not allow any authenticator, returns an empty list.
* @param userName the user name (may be null
, but must match if specified)
* @param credentialId the credential ID (must match the results)
* @return the list of authenticators allowed for the given userName and credential ID, or an empty list.
*/
public io.reactivex.Single> rxFind(java.lang.String userName, java.lang.String credentialId) {
return AsyncResultSingle.toSingle($handler -> {
this.find(userName, credentialId).onComplete($handler);
});
}
/**
* Persists a new credential, bound by its user name (may be null
) and credential ID
* (cannot be null
, must be unique).
*
* If attempting to store a credential with a credId
that is not unique, you should return
* a failed Future
.
*
* If attempting to store a credential with a userName
that already exists, you should
* first make sure that the current user is already logged in under the same userName
, because
* this will in practice add a new credential to identify the existing user, so this must be restricted
* to the already existing user, otherwise you will allow anyone to gain access to existing users.
*
* If attempting to store a credential with a userName
that already exists, and the current
* user is not logged in, or the logged in user does not have the same userName
, you should
* return a failed Future
.
* @param authenticator the new credential to persist
* @return a future of nothing, or a failed future if the credId
already exists, or if the userName
already exists and does not represent the currently logged in user.
*/
public io.vertx.core.Future storeCredential(io.vertx.ext.auth.webauthn4j.Authenticator authenticator) {
io.vertx.core.Future ret = delegate.storeCredential(authenticator).map(val -> val);
return ret;
}
/**
* Persists a new credential, bound by its user name (may be null
) and credential ID
* (cannot be null
, must be unique).
*
* If attempting to store a credential with a credId
that is not unique, you should return
* a failed Future
.
*
* If attempting to store a credential with a userName
that already exists, you should
* first make sure that the current user is already logged in under the same userName
, because
* this will in practice add a new credential to identify the existing user, so this must be restricted
* to the already existing user, otherwise you will allow anyone to gain access to existing users.
*
* If attempting to store a credential with a userName
that already exists, and the current
* user is not logged in, or the logged in user does not have the same userName
, you should
* return a failed Future
.
* @param authenticator the new credential to persist
* @return a future of nothing, or a failed future if the credId
already exists, or if the userName
already exists and does not represent the currently logged in user.
*/
public io.reactivex.Completable rxStoreCredential(io.vertx.ext.auth.webauthn4j.Authenticator authenticator) {
return AsyncResultCompletable.toCompletable($handler -> {
this.storeCredential(authenticator).onComplete($handler);
});
}
/**
* Updates a previously stored credential counter, as identified by its user name (may be null
) and credential ID
* (cannot be null
, must be unique).
* @param authenticator the credential to update
* @return a future of nothing
*/
public io.vertx.core.Future updateCounter(io.vertx.ext.auth.webauthn4j.Authenticator authenticator) {
io.vertx.core.Future ret = delegate.updateCounter(authenticator).map(val -> val);
return ret;
}
/**
* Updates a previously stored credential counter, as identified by its user name (may be null
) and credential ID
* (cannot be null
, must be unique).
* @param authenticator the credential to update
* @return a future of nothing
*/
public io.reactivex.Completable rxUpdateCounter(io.vertx.ext.auth.webauthn4j.Authenticator authenticator) {
return AsyncResultCompletable.toCompletable($handler -> {
this.updateCounter(authenticator).onComplete($handler);
});
}
public static CredentialStorage newInstance(io.vertx.ext.auth.webauthn4j.CredentialStorage arg) {
return arg != null ? new CredentialStorage(arg) : null;
}
}