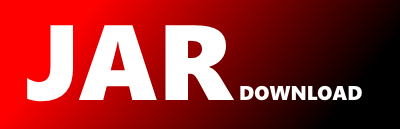
io.vertx.reactivex.ext.stomp.StompClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.ext.stomp;
import io.vertx.reactivex.RxHelper;
import io.vertx.reactivex.ObservableHelper;
import io.vertx.reactivex.FlowableHelper;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.AsyncResultSingle;
import io.vertx.reactivex.impl.AsyncResultCompletable;
import io.vertx.reactivex.WriteStreamObserver;
import io.vertx.reactivex.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Defines a STOMP client.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.stomp.StompClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.stomp.StompClient.class)
public class StompClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
StompClient that = (StompClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new StompClient((io.vertx.ext.stomp.StompClient) obj),
StompClient::getDelegate
);
private final io.vertx.ext.stomp.StompClient delegate;
public StompClient(io.vertx.ext.stomp.StompClient delegate) {
this.delegate = delegate;
}
public StompClient(Object delegate) {
this.delegate = (io.vertx.ext.stomp.StompClient)delegate;
}
public io.vertx.ext.stomp.StompClient getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.reactivex.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.reactivex.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)o1), o1 -> o1.getDelegate());
/**
* Creates a {@link io.vertx.reactivex.ext.stomp.StompClient} using the default implementation.
* @param vertx the vert.x instance to use
* @return the created {@link io.vertx.reactivex.ext.stomp.StompClient}
*/
public static io.vertx.reactivex.ext.stomp.StompClient create(io.vertx.reactivex.core.Vertx vertx) {
io.vertx.reactivex.ext.stomp.StompClient ret = io.vertx.reactivex.ext.stomp.StompClient.newInstance((io.vertx.ext.stomp.StompClient)io.vertx.ext.stomp.StompClient.create(vertx.getDelegate()));
return ret;
}
/**
* Creates a {@link io.vertx.reactivex.ext.stomp.StompClient} using the default implementation.
* @param vertx the vert.x instance to use
* @param options the options
* @return the created {@link io.vertx.reactivex.ext.stomp.StompClient}
*/
public static io.vertx.reactivex.ext.stomp.StompClient create(io.vertx.reactivex.core.Vertx vertx, io.vertx.ext.stomp.StompClientOptions options) {
io.vertx.reactivex.ext.stomp.StompClient ret = io.vertx.reactivex.ext.stomp.StompClient.newInstance((io.vertx.ext.stomp.StompClient)io.vertx.ext.stomp.StompClient.create(vertx.getDelegate(), options));
return ret;
}
/**
* Connects to the server.
* @param port the server port
* @param host the server host
* @return a future notified with the connection result
*/
public io.vertx.core.Future connect(int port, java.lang.String host) {
io.vertx.core.Future ret = delegate.connect(port, host).map(val -> io.vertx.reactivex.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)val));
return ret;
}
/**
* Connects to the server.
* @param port the server port
* @param host the server host
* @return a future notified with the connection result
*/
public io.reactivex.Single rxConnect(int port, java.lang.String host) {
return AsyncResultSingle.toSingle($handler -> {
this.connect(port, host).onComplete($handler);
});
}
/**
* Connects to the server using the host and port configured in the client's options.
* @return a future notified with the connection result. A failure will be sent to the handler if a TCP level issue happen before the `CONNECTED` frame is received.
*/
public io.vertx.core.Future connect() {
io.vertx.core.Future ret = delegate.connect().map(val -> io.vertx.reactivex.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)val));
return ret;
}
/**
* Connects to the server using the host and port configured in the client's options.
* @return a future notified with the connection result. A failure will be sent to the handler if a TCP level issue happen before the `CONNECTED` frame is received.
*/
public io.reactivex.Single rxConnect() {
return AsyncResultSingle.toSingle($handler -> {
this.connect().onComplete($handler);
});
}
/**
* Configures a received handler that gets notified when a STOMP frame is received by the client.
* This handler can be used for logging, debugging or ad-hoc behavior. The frame can still be modified at the time.
*
* When a connection is created, the handler is used as
* {@link io.vertx.reactivex.ext.stomp.StompClientConnection#receivedFrameHandler}.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompClient}
*/
public io.vertx.reactivex.ext.stomp.StompClient receivedFrameHandler(io.vertx.core.Handler handler) {
delegate.receivedFrameHandler(handler);
return this;
}
/**
* Configures a writing handler that gets notified when a STOMP frame is written on the wire.
* This handler can be used for logging, debugging or ad-hoc behavior. The frame can still be modified at the time.
*
* When a connection is created, the handler is used as
* {@link io.vertx.reactivex.ext.stomp.StompClientConnection#writingFrameHandler}.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompClient}
*/
public io.vertx.reactivex.ext.stomp.StompClient writingFrameHandler(io.vertx.core.Handler handler) {
delegate.writingFrameHandler(handler);
return this;
}
/**
* A general error frame handler. It can be used to catch ERROR
frame emitted during the connection process
* (wrong authentication). This error handler will be pass to all {@link io.vertx.reactivex.ext.stomp.StompClientConnection} created from this
* client. Obviously, the client can override it when the connection is established.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompClient}
*/
public io.vertx.reactivex.ext.stomp.StompClient errorFrameHandler(io.vertx.core.Handler handler) {
delegate.errorFrameHandler(handler);
return this;
}
/**
* Sets an exception handler notified for TCP-level errors.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompClient}
*/
public io.vertx.reactivex.ext.stomp.StompClient exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
/**
* Closes the client.
* @return
*/
public io.vertx.core.Future close() {
io.vertx.core.Future ret = delegate.close().map(val -> val);
return ret;
}
/**
* Closes the client.
* @return
*/
public io.reactivex.Completable rxClose() {
return AsyncResultCompletable.toCompletable($handler -> {
this.close().onComplete($handler);
});
}
/**
* @return the client's options.
*/
public io.vertx.ext.stomp.StompClientOptions options() {
io.vertx.ext.stomp.StompClientOptions ret = delegate.options();
return ret;
}
/**
* @return the vert.x instance used by the client.
*/
public io.vertx.reactivex.core.Vertx vertx() {
io.vertx.reactivex.core.Vertx ret = io.vertx.reactivex.core.Vertx.newInstance((io.vertx.core.Vertx)delegate.vertx());
return ret;
}
/**
* @return whether or not the client is connected to the server.
*/
public boolean isClosed() {
boolean ret = delegate.isClosed();
return ret;
}
public static StompClient newInstance(io.vertx.ext.stomp.StompClient arg) {
return arg != null ? new StompClient(arg) : null;
}
}