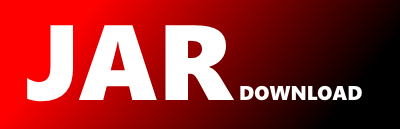
io.vertx.reactivex.ext.stomp.StompServerHandler Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.ext.stomp;
import io.vertx.reactivex.RxHelper;
import io.vertx.reactivex.ObservableHelper;
import io.vertx.reactivex.FlowableHelper;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.AsyncResultSingle;
import io.vertx.reactivex.impl.AsyncResultCompletable;
import io.vertx.reactivex.WriteStreamObserver;
import io.vertx.reactivex.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* STOMP server handler implements the behavior of the STOMP server when a specific event occurs. For instance, if
* let customize the behavior when specific STOMP frames arrives or when a connection is closed. This class has been
* designed to let you customize the server behavior. The default implementation is compliant with the STOMP
* specification. In this default implementation, not acknowledge frames are dropped.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.stomp.StompServerHandler original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.stomp.StompServerHandler.class)
public class StompServerHandler implements Handler {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
StompServerHandler that = (StompServerHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new StompServerHandler((io.vertx.ext.stomp.StompServerHandler) obj),
StompServerHandler::getDelegate
);
private final io.vertx.ext.stomp.StompServerHandler delegate;
public StompServerHandler(io.vertx.ext.stomp.StompServerHandler delegate) {
this.delegate = delegate;
}
public StompServerHandler(Object delegate) {
this.delegate = (io.vertx.ext.stomp.StompServerHandler)delegate;
}
public io.vertx.ext.stomp.StompServerHandler getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.reactivex.ext.stomp.Destination.newInstance((io.vertx.ext.stomp.Destination)o1), o1 -> o1.getDelegate());
/**
* Something has happened, so handle it.
* @param event the event to handle
*/
public void handle(io.vertx.reactivex.ext.stomp.ServerFrame event) {
delegate.handle(event.getDelegate());
}
/**
* Creates an instance of {@link io.vertx.reactivex.ext.stomp.StompServerHandler} using the default (compliant) implementation.
* @param vertx the vert.x instance to use
* @return the created {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public static io.vertx.reactivex.ext.stomp.StompServerHandler create(io.vertx.reactivex.core.Vertx vertx) {
io.vertx.reactivex.ext.stomp.StompServerHandler ret = io.vertx.reactivex.ext.stomp.StompServerHandler.newInstance((io.vertx.ext.stomp.StompServerHandler)io.vertx.ext.stomp.StompServerHandler.create(vertx.getDelegate()));
return ret;
}
/**
* Configures a handler that get notified when a STOMP frame is received by the server.
* This handler can be used for logging, debugging or ad-hoc behavior.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler receivedFrameHandler(io.vertx.core.Handler handler) {
delegate.receivedFrameHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a CONNECT
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler connectHandler(io.vertx.core.Handler handler) {
delegate.connectHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a STOMP
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler stompHandler(io.vertx.core.Handler handler) {
delegate.stompHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a SUBSCRIBE
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler subscribeHandler(io.vertx.core.Handler handler) {
delegate.subscribeHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a UNSUBSCRIBE
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler unsubscribeHandler(io.vertx.core.Handler handler) {
delegate.unsubscribeHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a SEND
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler sendHandler(io.vertx.core.Handler handler) {
delegate.sendHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a connection with the client is closed.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler closeHandler(io.vertx.core.Handler handler) {
delegate.closeHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.StompServerConnection.newInstance((io.vertx.ext.stomp.StompServerConnection)event)));
return this;
}
/**
* Called when the connection is closed. This method executes a default behavior and must calls the configured
* {@link io.vertx.reactivex.ext.stomp.StompServerHandler#closeHandler} if any.
* @param connection the connection
*/
public void onClose(io.vertx.reactivex.ext.stomp.StompServerConnection connection) {
delegate.onClose(connection.getDelegate());
}
/**
* Configures the action to execute when a COMMIT
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler commitHandler(io.vertx.core.Handler handler) {
delegate.commitHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a ABORT
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler abortHandler(io.vertx.core.Handler handler) {
delegate.abortHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a BEGIN
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler beginHandler(io.vertx.core.Handler handler) {
delegate.beginHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a DISCONNECT
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler disconnectHandler(io.vertx.core.Handler handler) {
delegate.disconnectHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a ACK
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler ackHandler(io.vertx.core.Handler handler) {
delegate.ackHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Configures the action to execute when a NACK
frame is received.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler nackHandler(io.vertx.core.Handler handler) {
delegate.nackHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.ServerFrame.newInstance((io.vertx.ext.stomp.ServerFrame)event)));
return this;
}
/**
* Called when the client connects to a server requiring authentication. It invokes the configured
* using {@link io.vertx.reactivex.ext.stomp.StompServerHandler#authProvider}.
* @param connection server connection that contains session ID
* @param login the login
* @param passcode the password
* @return a future notified with the authentication result
*/
public io.vertx.core.Future onAuthenticationRequest(io.vertx.reactivex.ext.stomp.StompServerConnection connection, java.lang.String login, java.lang.String passcode) {
io.vertx.core.Future ret = delegate.onAuthenticationRequest(connection.getDelegate(), login, passcode).map(val -> val);
return ret;
}
/**
* Called when the client connects to a server requiring authentication. It invokes the configured
* using {@link io.vertx.reactivex.ext.stomp.StompServerHandler#authProvider}.
* @param connection server connection that contains session ID
* @param login the login
* @param passcode the password
* @return a future notified with the authentication result
*/
public io.reactivex.Single rxOnAuthenticationRequest(io.vertx.reactivex.ext.stomp.StompServerConnection connection, java.lang.String login, java.lang.String passcode) {
return AsyncResultSingle.toSingle($handler -> {
this.onAuthenticationRequest(connection, login, passcode).onComplete($handler);
});
}
/**
* Provides for authorization matches on a destination level, this will return the User created by the .
* @param session session ID for the server connection.
* @return null if not authenticated.
*/
public io.vertx.reactivex.ext.auth.User getUserBySession(java.lang.String session) {
io.vertx.reactivex.ext.auth.User ret = io.vertx.reactivex.ext.auth.User.newInstance((io.vertx.ext.auth.User)delegate.getUserBySession(session));
return ret;
}
/**
* Configures the to be used to authenticate the user.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler authProvider(io.vertx.reactivex.ext.auth.authentication.AuthenticationProvider handler) {
delegate.authProvider(handler.getDelegate());
return this;
}
/**
* @return the list of destination managed by the STOMP server. Don't forget the STOMP interprets destination as opaque Strings.
*/
public java.util.List getDestinations() {
java.util.List ret = delegate.getDestinations().stream().map(elt -> io.vertx.reactivex.ext.stomp.Destination.newInstance((io.vertx.ext.stomp.Destination)elt)).collect(Collectors.toList());
return ret;
}
/**
* Gets the destination with the given name.
* @param destination the destination
* @return the {@link io.vertx.reactivex.ext.stomp.Destination}, null
if not existing.
*/
public io.vertx.reactivex.ext.stomp.Destination getDestination(java.lang.String destination) {
io.vertx.reactivex.ext.stomp.Destination ret = io.vertx.reactivex.ext.stomp.Destination.newInstance((io.vertx.ext.stomp.Destination)delegate.getDestination(destination));
return ret;
}
/**
* Method called by single message (client-individual policy) or a set of message (client policy) are acknowledged.
* Implementations must call the handler configured using {@link io.vertx.reactivex.ext.stomp.StompServerHandler#onAckHandler}.
* @param connection the connection
* @param subscribe the SUBSCRIBE
frame
* @param messages the acknowledge messages
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler onAck(io.vertx.reactivex.ext.stomp.StompServerConnection connection, io.vertx.ext.stomp.Frame subscribe, java.util.List messages) {
delegate.onAck(connection.getDelegate(), subscribe, messages);
return this;
}
/**
* Method called by single message (client-individual policy) or a set of message (client policy) are
* not acknowledged. Not acknowledgment can result from a NACK
frame or from a timeout (no
* ACK
frame received in a given time. Implementations must call the handler configured using
* {@link io.vertx.reactivex.ext.stomp.StompServerHandler#onNackHandler}.
* @param connection the connection
* @param subscribe the SUBSCRIBE
frame
* @param messages the acknowledge messages
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler onNack(io.vertx.reactivex.ext.stomp.StompServerConnection connection, io.vertx.ext.stomp.Frame subscribe, java.util.List messages) {
delegate.onNack(connection.getDelegate(), subscribe, messages);
return this;
}
/**
* Configures the action to execute when messages are acknowledged.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler onAckHandler(io.vertx.core.Handler handler) {
delegate.onAckHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.Acknowledgement.newInstance((io.vertx.ext.stomp.Acknowledgement)event)));
return this;
}
/**
* Configures the action to execute when messages are not acknowledged.
* @param handler the handler
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler onNackHandler(io.vertx.core.Handler handler) {
delegate.onNackHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.Acknowledgement.newInstance((io.vertx.ext.stomp.Acknowledgement)event)));
return this;
}
/**
* Allows customizing the action to do when the server needs to send a `PING` to the client. By default it send a
* frame containing EOL
(specification). However, you can customize this and send another frame. However,
* be aware that this may requires a custom client.
*
* The handler will only be called if the connection supports heartbeats.
* @param handler the action to execute when a `PING` needs to be sent.
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler pingHandler(io.vertx.core.Handler handler) {
delegate.pingHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.reactivex.ext.stomp.StompServerConnection.newInstance((io.vertx.ext.stomp.StompServerConnection)event)));
return this;
}
/**
* Gets a {@link io.vertx.reactivex.ext.stomp.Destination} object if existing, or create a new one. The creation is delegated to the
* {@link io.vertx.reactivex.ext.stomp.DestinationFactory}.
* @param destination the destination
* @return the {@link io.vertx.reactivex.ext.stomp.Destination} instance, may have been created.
*/
public io.vertx.reactivex.ext.stomp.Destination getOrCreateDestination(java.lang.String destination) {
io.vertx.reactivex.ext.stomp.Destination ret = io.vertx.reactivex.ext.stomp.Destination.newInstance((io.vertx.ext.stomp.Destination)delegate.getOrCreateDestination(destination));
return ret;
}
/**
* Configures the {@link io.vertx.reactivex.ext.stomp.DestinationFactory} used to create {@link io.vertx.reactivex.ext.stomp.Destination} objects.
* @param factory the factory
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}.
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler destinationFactory(io.vertx.reactivex.ext.stomp.DestinationFactory factory) {
delegate.destinationFactory(factory.getDelegate());
return this;
}
/**
* Configures the STOMP server to act as a bridge with the Vert.x event bus.
* @param options the configuration options
* @return the current {@link io.vertx.reactivex.ext.stomp.StompServerHandler}.
*/
public io.vertx.reactivex.ext.stomp.StompServerHandler bridge(io.vertx.ext.stomp.BridgeOptions options) {
delegate.bridge(options);
return this;
}
public static StompServerHandler newInstance(io.vertx.ext.stomp.StompServerHandler arg) {
return arg != null ? new StompServerHandler(arg) : null;
}
}