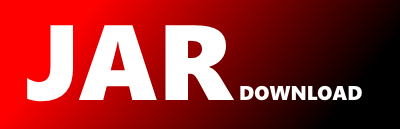
io.vertx.reactivex.sqlclient.Tuple Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.reactivex.sqlclient;
import io.vertx.reactivex.RxHelper;
import io.vertx.reactivex.ObservableHelper;
import io.vertx.reactivex.FlowableHelper;
import io.vertx.reactivex.impl.AsyncResultMaybe;
import io.vertx.reactivex.impl.AsyncResultSingle;
import io.vertx.reactivex.impl.AsyncResultCompletable;
import io.vertx.reactivex.WriteStreamObserver;
import io.vertx.reactivex.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A general purpose tuple.
*
* CAUTION: indexes start at 0, not at 1.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.sqlclient.Tuple original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.sqlclient.Tuple.class)
public class Tuple {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Tuple that = (Tuple) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new Tuple((io.vertx.sqlclient.Tuple) obj),
Tuple::getDelegate
);
private final io.vertx.sqlclient.Tuple delegate;
public Tuple(io.vertx.sqlclient.Tuple delegate) {
this.delegate = delegate;
}
public Tuple(Object delegate) {
this.delegate = (io.vertx.sqlclient.Tuple)delegate;
}
public io.vertx.sqlclient.Tuple getDelegate() {
return delegate;
}
/**
* @return a new empty tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple tuple() {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.tuple());
return ret;
}
/**
* Wrap the provided list
with a tuple.
*
* The list is not copied and is used as store for tuple elements.
* @param list
* @return the list wrapped as a tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple from(java.util.List list) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.from(list));
return ret;
}
/**
* Wrap the provided list
with a tuple.
*
* The list is not copied and is used as store for tuple elements.
*
* Note: The list might be modified and users should use {@link io.vertx.reactivex.sqlclient.Tuple#tuple} if the list is unmodifiable
* @param list
* @return the list wrapped as a tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple wrap(java.util.List list) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.wrap(list));
return ret;
}
/**
* Create a tuple of one element.
* @param elt1 the first value
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple of(java.lang.Object elt1) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.of(elt1));
return ret;
}
/**
* Create a tuple of two elements.
* @param elt1 the first value
* @param elt2 the second value
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple of(java.lang.Object elt1, java.lang.Object elt2) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.of(elt1, elt2));
return ret;
}
/**
* Create a tuple of three elements.
* @param elt1 the first value
* @param elt2 the second value
* @param elt3 the third value
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple of(java.lang.Object elt1, java.lang.Object elt2, java.lang.Object elt3) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.of(elt1, elt2, elt3));
return ret;
}
/**
* Create a tuple of four elements.
* @param elt1 the first value
* @param elt2 the second value
* @param elt3 the third value
* @param elt4 the fourth value
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple of(java.lang.Object elt1, java.lang.Object elt2, java.lang.Object elt3, java.lang.Object elt4) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.of(elt1, elt2, elt3, elt4));
return ret;
}
/**
* Create a tuple of five elements.
* @param elt1 the first value
* @param elt2 the second value
* @param elt3 the third value
* @param elt4 the fourth value
* @param elt5 the fifth value
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple of(java.lang.Object elt1, java.lang.Object elt2, java.lang.Object elt3, java.lang.Object elt4, java.lang.Object elt5) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.of(elt1, elt2, elt3, elt4, elt5));
return ret;
}
/**
* Create a tuple of six elements.
* @param elt1 the first value
* @param elt2 the second valueg
* @param elt3 the third value
* @param elt4 the fourth value
* @param elt5 the fifth value
* @param elt6 the sixth value
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple of(java.lang.Object elt1, java.lang.Object elt2, java.lang.Object elt3, java.lang.Object elt4, java.lang.Object elt5, java.lang.Object elt6) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.of(elt1, elt2, elt3, elt4, elt5, elt6));
return ret;
}
/**
* Create a tuple with the provided elements
list.
*
* The elements
list is not modified.
* @param elements the list of elements
* @return the tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple tuple(java.util.List elements) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.tuple(elements));
return ret;
}
/**
* Get an object value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Object getValue(int pos) {
java.lang.Object ret = (Object) delegate.getValue(pos);
return ret;
}
/**
* Get a boolean value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Boolean getBoolean(int pos) {
java.lang.Boolean ret = delegate.getBoolean(pos);
return ret;
}
/**
* Get a short value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Short getShort(int pos) {
java.lang.Short ret = delegate.getShort(pos);
return ret;
}
/**
* Get an integer value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Integer getInteger(int pos) {
java.lang.Integer ret = delegate.getInteger(pos);
return ret;
}
/**
* Get a long value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Long getLong(int pos) {
java.lang.Long ret = delegate.getLong(pos);
return ret;
}
/**
* Get a float value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Float getFloat(int pos) {
java.lang.Float ret = delegate.getFloat(pos);
return ret;
}
/**
* Get a double value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.Double getDouble(int pos) {
java.lang.Double ret = delegate.getDouble(pos);
return ret;
}
/**
* Get a string value at pos
.
* @param pos the position
* @return the value
*/
public java.lang.String getString(int pos) {
java.lang.String ret = delegate.getString(pos);
return ret;
}
/**
* Get a value at pos
.
* @param pos the position
* @return the value
*/
public io.vertx.core.json.JsonObject getJsonObject(int pos) {
io.vertx.core.json.JsonObject ret = delegate.getJsonObject(pos);
return ret;
}
/**
* Get a value at pos
.
* @param pos the position
* @return the value
*/
public io.vertx.core.json.JsonArray getJsonArray(int pos) {
io.vertx.core.json.JsonArray ret = delegate.getJsonArray(pos);
return ret;
}
/**
* Get a JSON element at pos
, the element might be {@link io.vertx.reactivex.sqlclient.Tuple #JSON_NULL null} or one of the following types:
*
* - String
* - Number
* - JsonObject
* - JsonArray
* - Boolean
*
* @param pos the position
* @return the value
*/
public java.lang.Object getJson(int pos) {
java.lang.Object ret = (Object) delegate.getJson(pos);
return ret;
}
/**
* Get a buffer value at pos
.
* @param pos the position
* @return the value
*/
public io.vertx.core.buffer.Buffer getBuffer(int pos) {
io.vertx.core.buffer.Buffer ret = delegate.getBuffer(pos);
return ret;
}
/**
* Add an object value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addValue(java.lang.Object value) {
delegate.addValue(value);
return this;
}
/**
* Add a boolean value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addBoolean(java.lang.Boolean value) {
delegate.addBoolean(value);
return this;
}
/**
* Add a short value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addShort(java.lang.Short value) {
delegate.addShort(value);
return this;
}
/**
* Add an integer value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addInteger(java.lang.Integer value) {
delegate.addInteger(value);
return this;
}
/**
* Add a long value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addLong(java.lang.Long value) {
delegate.addLong(value);
return this;
}
/**
* Add a float value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addFloat(java.lang.Float value) {
delegate.addFloat(value);
return this;
}
/**
* Add a double value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addDouble(java.lang.Double value) {
delegate.addDouble(value);
return this;
}
/**
* Add a string value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addString(java.lang.String value) {
delegate.addString(value);
return this;
}
/**
* Add a value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addJsonObject(io.vertx.core.json.JsonObject value) {
delegate.addJsonObject(value);
return this;
}
/**
* Add a value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addJsonArray(io.vertx.core.json.JsonArray value) {
delegate.addJsonArray(value);
return this;
}
/**
* Add a buffer value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addBuffer(io.vertx.core.buffer.Buffer value) {
delegate.addBuffer(value);
return this;
}
/**
* Get the the at the specified position
and the specified type
.
*
* The type can be one of the types returned by the row (e.g String.class
) or an array
* of the type (e.g String[].class
)).
* @param type the expected value type
* @param position the value position
* @return the value if the value is found or null.
*/
public T get(java.lang.Class type, int position) {
T ret = (T)TypeArg.of(type).wrap(delegate.get(io.vertx.lang.reactivex.Helper.unwrap(type), position));
return ret;
}
/**
* @return the tuple size
*/
public int size() {
int ret = delegate.size();
return ret;
}
public void clear() {
delegate.clear();
}
/**
* @return A String containing the {@link java.lang.Object} value of each element, separated by a comma (,) character
*/
public java.lang.String deepToString() {
java.lang.String ret = delegate.deepToString();
return ret;
}
/**
* Wrap the provided array
with a tuple.
*
* The array is not copied and is used as store for tuple elements.
* @param array
* @return the list wrapped as a tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple from(java.lang.Object[] array) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.from(array));
return ret;
}
/**
* Wrap the provided array
with a tuple.
*
* The array is not copied and is used as store for tuple elements.
* @param array
* @return the list wrapped as a tuple
*/
public static io.vertx.reactivex.sqlclient.Tuple wrap(java.lang.Object[] array) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)io.vertx.sqlclient.Tuple.wrap(array));
return ret;
}
/**
* Get value at pos
.
* @param pos the position
* @return the value
*/
public io.vertx.sqlclient.data.Numeric getNumeric(int pos) {
io.vertx.sqlclient.data.Numeric ret = delegate.getNumeric(pos);
return ret;
}
/**
* Get a {@link java.time.temporal.Temporal} value at pos
.
* @param pos the position
* @return the value
*/
public java.time.temporal.Temporal getTemporal(int pos) {
java.time.temporal.Temporal ret = delegate.getTemporal(pos);
return ret;
}
/**
* Get {@link java.time.LocalDate} value at pos
.
*
* Target element instance of LocalDateTime
will be
* coerced to LocalDate
.
* @param pos the position
* @return the value
*/
public java.time.LocalDate getLocalDate(int pos) {
java.time.LocalDate ret = delegate.getLocalDate(pos);
return ret;
}
/**
* Get {@link java.time.LocalTime} value at pos
.
*
*
Target element instance of LocalDateTime
will be
* coerced to LocalTime
.
* @param pos the position
* @return the value
*/
public java.time.LocalTime getLocalTime(int pos) {
java.time.LocalTime ret = delegate.getLocalTime(pos);
return ret;
}
/**
* Get {@link java.time.LocalDateTime} value at pos
.
* @param pos the position
* @return the value
*/
public java.time.LocalDateTime getLocalDateTime(int pos) {
java.time.LocalDateTime ret = delegate.getLocalDateTime(pos);
return ret;
}
/**
* Get {@link java.time.OffsetTime} value at pos
.
*
*
Target element instance of OffsetDateTime
will be
* coerced to OffsetTime
.
* @param pos the position
* @return the value
*/
public java.time.OffsetTime getOffsetTime(int pos) {
java.time.OffsetTime ret = delegate.getOffsetTime(pos);
return ret;
}
/**
* Get {@link java.time.OffsetDateTime} value at pos
.
* @param pos the position
* @return the value
*/
public java.time.OffsetDateTime getOffsetDateTime(int pos) {
java.time.OffsetDateTime ret = delegate.getOffsetDateTime(pos);
return ret;
}
/**
* Get {@link java.util.UUID} value at pos
.
* @param pos the position
* @return the value
*/
public java.util.UUID getUUID(int pos) {
java.util.UUID ret = delegate.getUUID(pos);
return ret;
}
/**
* Get value at pos
.
* @param pos the position
* @return the value
*/
public java.math.BigDecimal getBigDecimal(int pos) {
java.math.BigDecimal ret = delegate.getBigDecimal(pos);
return ret;
}
/**
* Get an array of {@link java.lang.Boolean} value at pos
.
*
*
Target element instance of Object[]
will be
* coerced to Boolean[]
.
* @param pos the position
* @return the value
*/
public java.lang.Boolean[] getArrayOfBooleans(int pos) {
java.lang.Boolean[] ret = delegate.getArrayOfBooleans(pos);
return ret;
}
/**
* Get an array of {@link java.lang.Short} value at pos
.
*
*
Target element instance of Number[]
or Object[]
will be
* coerced to Short[]
.
* @param pos the position
* @return the value
*/
public java.lang.Short[] getArrayOfShorts(int pos) {
java.lang.Short[] ret = delegate.getArrayOfShorts(pos);
return ret;
}
/**
* Get an array of {@link java.lang.Integer} value at pos
.
*
*
Target element instance of Number[]
or Object[]
will be
* coerced to Integer[]
.
* @param pos the position
* @return the value
*/
public java.lang.Integer[] getArrayOfIntegers(int pos) {
java.lang.Integer[] ret = delegate.getArrayOfIntegers(pos);
return ret;
}
/**
* Get an array of {@link java.lang.Long} value at pos
.
*
*
Target element instance of Number[]
or Object[]
will be
* coerced to Long[]
.
* @param pos the position
* @return the value
*/
public java.lang.Long[] getArrayOfLongs(int pos) {
java.lang.Long[] ret = delegate.getArrayOfLongs(pos);
return ret;
}
/**
* Get an array of {@link java.lang.Float} value at pos
.
*
*
Target element instance of Number[]
or Object[]
will be
* coerced to Float[]
.
* @param pos the position
* @return the value
*/
public java.lang.Float[] getArrayOfFloats(int pos) {
java.lang.Float[] ret = delegate.getArrayOfFloats(pos);
return ret;
}
/**
* Get an array of {@link java.lang.Double} value at pos
.
*
*
Target element instance of Number[]
or Object[]
will be
* coerced to Double[]
.
* @param pos the position
* @return the value
*/
public java.lang.Double[] getArrayOfDoubles(int pos) {
java.lang.Double[] ret = delegate.getArrayOfDoubles(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the column
* @return the value
*/
public io.vertx.sqlclient.data.Numeric[] getArrayOfNumerics(int pos) {
io.vertx.sqlclient.data.Numeric[] ret = delegate.getArrayOfNumerics(pos);
return ret;
}
/**
* Get an array of {@link java.lang.String} value at pos
.
*
*
Target element instance of Object[]
will be
* coerced to String[]
.
* @param pos the position
* @return the value
*/
public java.lang.String[] getArrayOfStrings(int pos) {
java.lang.String[] ret = delegate.getArrayOfStrings(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the position
* @return the value
*/
public io.vertx.core.json.JsonObject[] getArrayOfJsonObjects(int pos) {
io.vertx.core.json.JsonObject[] ret = delegate.getArrayOfJsonObjects(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the position
* @return the value
*/
public io.vertx.core.json.JsonArray[] getArrayOfJsonArrays(int pos) {
io.vertx.core.json.JsonArray[] ret = delegate.getArrayOfJsonArrays(pos);
return ret;
}
/**
* Get an array of JSON elements at pos
, the element might be {@link io.vertx.reactivex.sqlclient.Tuple #JSON_NULL null} or one of the following types:
*
* - String
* - Number
* - JsonObject
* - JsonArray
* - Boolean
*
* @param pos the position
* @return the value
*/
public java.lang.Object[] getArrayOfJsons(int pos) {
java.lang.Object[] ret = delegate.getArrayOfJsons(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the position
* @return the value
*/
public java.time.temporal.Temporal[] getArrayOfTemporals(int pos) {
java.time.temporal.Temporal[] ret = delegate.getArrayOfTemporals(pos);
return ret;
}
/**
* Get an array of value at pos
.
*
* Target element instance of LocalDateTime[]
will be
* coerced to LocalDate[]
.
* @param pos the position
* @return the value
*/
public java.time.LocalDate[] getArrayOfLocalDates(int pos) {
java.time.LocalDate[] ret = delegate.getArrayOfLocalDates(pos);
return ret;
}
/**
* Get an array of value at pos
.
*
*
Target element instance of LocalDateTime[]
will be
* coerced to LocalTime[]
.
* @param pos the position
* @return the value
*/
public java.time.LocalTime[] getArrayOfLocalTimes(int pos) {
java.time.LocalTime[] ret = delegate.getArrayOfLocalTimes(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the position
* @return the value
*/
public java.time.LocalDateTime[] getArrayOfLocalDateTimes(int pos) {
java.time.LocalDateTime[] ret = delegate.getArrayOfLocalDateTimes(pos);
return ret;
}
/**
* Get an array of value at pos
.
*
*
Target element instance of OffsetDateTime[]
will be
* coerced to OffsetTime[]
.
* @param pos the position
* @return the value
*/
public java.time.OffsetTime[] getArrayOfOffsetTimes(int pos) {
java.time.OffsetTime[] ret = delegate.getArrayOfOffsetTimes(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the position
* @return the value
*/
public java.time.OffsetDateTime[] getArrayOfOffsetDateTimes(int pos) {
java.time.OffsetDateTime[] ret = delegate.getArrayOfOffsetDateTimes(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the column
* @return the value
*/
public java.util.UUID[] getArrayOfUUIDs(int pos) {
java.util.UUID[] ret = delegate.getArrayOfUUIDs(pos);
return ret;
}
/**
* Get an array of value at pos
.
* @param pos the column
* @return the value
*/
public java.math.BigDecimal[] getArrayOfBigDecimals(int pos) {
java.math.BigDecimal[] ret = delegate.getArrayOfBigDecimals(pos);
return ret;
}
/**
* Add a {@link java.time.temporal.Temporal} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addTemporal(java.time.temporal.Temporal value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addTemporal(value));
return ret;
}
/**
* Add a {@link java.time.LocalDate} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addLocalDate(java.time.LocalDate value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addLocalDate(value));
return ret;
}
/**
* Add a {@link java.time.LocalTime} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addLocalTime(java.time.LocalTime value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addLocalTime(value));
return ret;
}
/**
* Add a {@link java.time.LocalDateTime} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addLocalDateTime(java.time.LocalDateTime value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addLocalDateTime(value));
return ret;
}
/**
* Add a {@link java.time.OffsetTime} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addOffsetTime(java.time.OffsetTime value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addOffsetTime(value));
return ret;
}
/**
* Add a {@link java.time.OffsetDateTime} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addOffsetDateTime(java.time.OffsetDateTime value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addOffsetDateTime(value));
return ret;
}
/**
* Add a {@link java.util.UUID} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addUUID(java.util.UUID value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addUUID(value));
return ret;
}
/**
* Add a value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addBigDecimal(java.math.BigDecimal value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addBigDecimal(value));
return ret;
}
/**
* Add an array of Boolean
value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfBoolean(java.lang.Boolean[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfBoolean(value));
return ret;
}
/**
* Add an array of {@link java.lang.Short} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfShort(java.lang.Short[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfShort(value));
return ret;
}
/**
* Add an array of Integer
value at the end of the tuple.
*
*
Target element instance of Number[]
will be
* coerced to Integer[]
.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfInteger(java.lang.Integer[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfInteger(value));
return ret;
}
/**
* Add an array of {@link java.lang.Long} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfLong(java.lang.Long[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfLong(value));
return ret;
}
/**
* Add an array of {@link java.lang.Float} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfFloat(java.lang.Float[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfFloat(value));
return ret;
}
/**
* Add an array of {@link java.lang.Double} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfDouble(java.lang.Double[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfDouble(value));
return ret;
}
/**
* Add an array of {@link java.lang.String} value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfString(java.lang.String[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfString(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfJsonObject(io.vertx.core.json.JsonObject[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfJsonObject(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfJsonArray(io.vertx.core.json.JsonArray[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfJsonArray(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfTemporal(java.time.temporal.Temporal[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfTemporal(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfLocalDate(java.time.LocalDate[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfLocalDate(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfLocalTime(java.time.LocalTime[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfLocalTime(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfLocalDateTime(java.time.LocalDateTime[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfLocalDateTime(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfOffsetTime(java.time.OffsetTime[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfOffsetTime(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfOffsetDateTime(java.time.OffsetDateTime[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfOffsetDateTime(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfUUID(java.util.UUID[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfUUID(value));
return ret;
}
/**
* Add an array of value at the end of the tuple.
* @param value the value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.reactivex.sqlclient.Tuple addArrayOfBigDecimal(java.math.BigDecimal[] value) {
io.vertx.reactivex.sqlclient.Tuple ret = io.vertx.reactivex.sqlclient.Tuple.newInstance((io.vertx.sqlclient.Tuple)delegate.addArrayOfBigDecimal(value));
return ret;
}
/**
* The JSON null literal value.
*
* It is used to distinguish a JSON null literal value from the Java null
value. This is only
* used when the database supports JSON types.
*/
public static final java.lang.Object JSON_NULL = (Object) io.vertx.sqlclient.Tuple.JSON_NULL;
public static Tuple newInstance(io.vertx.sqlclient.Tuple arg) {
return arg != null ? new Tuple(arg) : null;
}
}