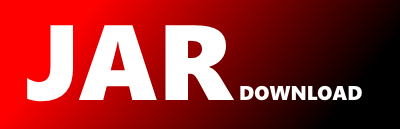
io.vertx.rxjava3.amqp.AmqpConnection Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.amqp;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Once connected to the broker or router, you get a connection. This connection is automatically opened.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.amqp.AmqpConnection original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.amqp.AmqpConnection.class)
public class AmqpConnection {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AmqpConnection that = (AmqpConnection) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new AmqpConnection((io.vertx.amqp.AmqpConnection) obj),
AmqpConnection::getDelegate
);
private final io.vertx.amqp.AmqpConnection delegate;
public AmqpConnection(io.vertx.amqp.AmqpConnection delegate) {
this.delegate = delegate;
}
public AmqpConnection(Object delegate) {
this.delegate = (io.vertx.amqp.AmqpConnection)delegate;
}
public io.vertx.amqp.AmqpConnection getDelegate() {
return delegate;
}
/**
* Registers a handler called on disconnection.
* @param handler the exception handler.
* @return the connection
*/
public io.vertx.rxjava3.amqp.AmqpConnection exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
/**
* Closes the AMQP connection, i.e. allows the Close frame to be emitted.
* @return the connection
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Closes the AMQP connection, i.e. allows the Close frame to be emitted.
* @return the connection
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( done -> {
delegate.close(done);
});
}
/**
* Creates a receiver used to consume messages from the given address. The receiver has no handler and won't
* start receiving messages until a handler is explicitly configured.
* @param address The source address to attach the consumer to, must not be null
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single createReceiver(java.lang.String address) {
io.reactivex.rxjava3.core.Single ret = rxCreateReceiver(address);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a receiver used to consume messages from the given address. The receiver has no handler and won't
* start receiving messages until a handler is explicitly configured.
* @param address The source address to attach the consumer to, must not be null
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single rxCreateReceiver(java.lang.String address) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createReceiver(address, new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> io.vertx.rxjava3.amqp.AmqpReceiver.newInstance((io.vertx.amqp.AmqpReceiver)event))));
});
}
/**
* Creates a receiver used to consumer messages from the given address.
* @param address The source address to attach the consumer to.
* @param receiverOptions The options for this receiver.
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single createReceiver(java.lang.String address, io.vertx.amqp.AmqpReceiverOptions receiverOptions) {
io.reactivex.rxjava3.core.Single ret = rxCreateReceiver(address, receiverOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a receiver used to consumer messages from the given address.
* @param address The source address to attach the consumer to.
* @param receiverOptions The options for this receiver.
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single rxCreateReceiver(java.lang.String address, io.vertx.amqp.AmqpReceiverOptions receiverOptions) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createReceiver(address, receiverOptions, new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> io.vertx.rxjava3.amqp.AmqpReceiver.newInstance((io.vertx.amqp.AmqpReceiver)event))));
});
}
/**
* Creates a dynamic receiver. The address is provided by the broker and is available in the completionHandler
,
* using the {@link io.vertx.rxjava3.amqp.AmqpReceiver#address} method. this method is useful for request-reply to generate a unique
* reply address.
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single createDynamicReceiver() {
io.reactivex.rxjava3.core.Single ret = rxCreateDynamicReceiver();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a dynamic receiver. The address is provided by the broker and is available in the completionHandler
,
* using the {@link io.vertx.rxjava3.amqp.AmqpReceiver#address} method. this method is useful for request-reply to generate a unique
* reply address.
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single rxCreateDynamicReceiver() {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createDynamicReceiver(new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> io.vertx.rxjava3.amqp.AmqpReceiver.newInstance((io.vertx.amqp.AmqpReceiver)event))));
});
}
/**
* Creates a sender used to send messages to the given address. The address must be set. For anonymous sender, check
* {@link io.vertx.rxjava3.amqp.AmqpConnection#createAnonymousSender}.
* @param address The target address to attach to, must not be null
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single createSender(java.lang.String address) {
io.reactivex.rxjava3.core.Single ret = rxCreateSender(address);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a sender used to send messages to the given address. The address must be set. For anonymous sender, check
* {@link io.vertx.rxjava3.amqp.AmqpConnection#createAnonymousSender}.
* @param address The target address to attach to, must not be null
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single rxCreateSender(java.lang.String address) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createSender(address, new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> io.vertx.rxjava3.amqp.AmqpSender.newInstance((io.vertx.amqp.AmqpSender)event))));
});
}
/**
* Creates a sender used to send messages to the given address. The address must be set. For anonymous sender, check
* {@link io.vertx.rxjava3.amqp.AmqpConnection#createAnonymousSender}.
* @param address The target address to attach to, allowed to be null
if the options
configures the sender to be attached to a dynamic address (provided by the broker).
* @param options The AMQP sender options
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single createSender(java.lang.String address, io.vertx.amqp.AmqpSenderOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCreateSender(address, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a sender used to send messages to the given address. The address must be set. For anonymous sender, check
* {@link io.vertx.rxjava3.amqp.AmqpConnection#createAnonymousSender}.
* @param address The target address to attach to, allowed to be null
if the options
configures the sender to be attached to a dynamic address (provided by the broker).
* @param options The AMQP sender options
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single rxCreateSender(java.lang.String address, io.vertx.amqp.AmqpSenderOptions options) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createSender(address, options, new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> io.vertx.rxjava3.amqp.AmqpSender.newInstance((io.vertx.amqp.AmqpSender)event))));
});
}
/**
* Creates an anonymous sender.
*
* Unlike "regular" sender, this sender is not associated to a specific address, and each message sent must provide
* an address. This method can be used in request-reply scenarios where you create a sender to send the reply,
* but you don't know the address, as the reply address is passed into the message you are going to receive.
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single createAnonymousSender() {
io.reactivex.rxjava3.core.Single ret = rxCreateAnonymousSender();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates an anonymous sender.
*
* Unlike "regular" sender, this sender is not associated to a specific address, and each message sent must provide
* an address. This method can be used in request-reply scenarios where you create a sender to send the reply,
* but you don't know the address, as the reply address is passed into the message you are going to receive.
* @return the connection.
*/
public io.reactivex.rxjava3.core.Single rxCreateAnonymousSender() {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createAnonymousSender(new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> io.vertx.rxjava3.amqp.AmqpSender.newInstance((io.vertx.amqp.AmqpSender)event))));
});
}
/**
* @return whether the connection has been disconnected.
*/
public boolean isDisconnected() {
boolean ret = delegate.isDisconnected();
return ret;
}
/**
* @return a future completed when the connection is closed
*/
public io.vertx.core.Future closeFuture() {
io.vertx.core.Future ret = delegate.closeFuture().map(val -> val);
return ret;
}
/**
* @return the underlying ProtonConnection.
*/
public io.vertx.proton.ProtonConnection unwrap() {
io.vertx.proton.ProtonConnection ret = delegate.unwrap();
return ret;
}
public static AmqpConnection newInstance(io.vertx.amqp.AmqpConnection arg) {
return arg != null ? new AmqpConnection(arg) : null;
}
}