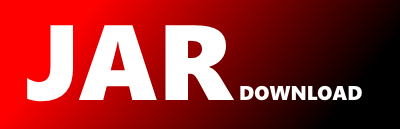
io.vertx.rxjava3.core.buffer.Buffer Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.buffer;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Most data is shuffled around inside Vert.x using buffers.
*
* A buffer is a sequence of zero or more bytes that can read from or written to and which expands automatically as
* necessary to accommodate any bytes written to it. You can perhaps think of a buffer as smart byte array.
*
* Please consult the documentation for more information on buffers.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.buffer.Buffer original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.buffer.Buffer.class)
public class Buffer implements io.vertx.core.shareddata.impl.ClusterSerializable {
@Override
public void writeToBuffer(io.vertx.core.buffer.Buffer buffer) {
delegate.writeToBuffer(buffer);
}
@Override
public int readFromBuffer(int pos, io.vertx.core.buffer.Buffer buffer) {
return delegate.readFromBuffer(pos, buffer);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Buffer that = (Buffer) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new Buffer((io.vertx.core.buffer.Buffer) obj),
Buffer::getDelegate
);
private final io.vertx.core.buffer.Buffer delegate;
public Buffer(io.vertx.core.buffer.Buffer delegate) {
this.delegate = delegate;
}
public Buffer(Object delegate) {
this.delegate = (io.vertx.core.buffer.Buffer)delegate;
}
public io.vertx.core.buffer.Buffer getDelegate() {
return delegate;
}
/**
* Create a new, empty buffer.
* @return the buffer
*/
public static io.vertx.rxjava3.core.buffer.Buffer buffer() {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)io.vertx.core.buffer.Buffer.buffer());
return ret;
}
/**
* Create a new buffer given the initial size hint.
*
* If you know the buffer will require a certain size, providing the hint can prevent unnecessary re-allocations
* as the buffer is written to and resized.
* @param initialSizeHint the hint, in bytes
* @return the buffer
*/
public static io.vertx.rxjava3.core.buffer.Buffer buffer(int initialSizeHint) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)io.vertx.core.buffer.Buffer.buffer(initialSizeHint));
return ret;
}
/**
* Create a new buffer from a string. The string will be UTF-8 encoded into the buffer.
* @param string the string
* @return the buffer
*/
public static io.vertx.rxjava3.core.buffer.Buffer buffer(java.lang.String string) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)io.vertx.core.buffer.Buffer.buffer(string));
return ret;
}
/**
* Create a new buffer from a string and using the specified encoding.
* The string will be encoded into the buffer using the specified encoding.
* @param string the string
* @param enc
* @return the buffer
*/
public static io.vertx.rxjava3.core.buffer.Buffer buffer(java.lang.String string, java.lang.String enc) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)io.vertx.core.buffer.Buffer.buffer(string, enc));
return ret;
}
/**
* Returns a String
representation of the Buffer with the UTF-8
encoding
* @return
*/
public java.lang.String toString() {
java.lang.String ret = delegate.toString();
return ret;
}
/**
* Returns a String
representation of the Buffer with the encoding specified by enc
* @param enc
* @return
*/
public java.lang.String toString(java.lang.String enc) {
java.lang.String ret = delegate.toString(enc);
return ret;
}
/**
* Returns a representation of this buffer's content.
* @return
*/
public io.vertx.core.json.JsonObject toJsonObject() {
io.vertx.core.json.JsonObject ret = delegate.toJsonObject();
return ret;
}
/**
* Returns a representation of this buffer's content.
* @return
*/
public io.vertx.core.json.JsonArray toJsonArray() {
io.vertx.core.json.JsonArray ret = delegate.toJsonArray();
return ret;
}
/**
* Returns a Json value representation of this buffer's content.
* @return a Json value which can be a , , {@link java.lang.String}, ... if the buffer contains an array, object, string, ...etc
*/
public java.lang.Object toJsonValue() {
java.lang.Object ret = (Object) delegate.toJsonValue();
return ret;
}
/**
* @return
*/
@Deprecated()
public java.lang.Object toJson() {
java.lang.Object ret = (Object) delegate.toJson();
return ret;
}
/**
* Returns the byte
at position pos
in the Buffer.
* @param pos
* @return
*/
public byte getByte(int pos) {
byte ret = delegate.getByte(pos);
return ret;
}
/**
* Returns the unsigned byte
at position pos
in the Buffer, as a short
.
* @param pos
* @return
*/
public short getUnsignedByte(int pos) {
short ret = delegate.getUnsignedByte(pos);
return ret;
}
/**
* Returns the int
at position pos
in the Buffer.
* @param pos
* @return
*/
public int getInt(int pos) {
int ret = delegate.getInt(pos);
return ret;
}
/**
* Gets a 32-bit integer at the specified absolute index
in this buffer with Little Endian Byte Order.
* @param pos
* @return
*/
public int getIntLE(int pos) {
int ret = delegate.getIntLE(pos);
return ret;
}
/**
* Returns the unsigned int
at position pos
in the Buffer, as a long
.
* @param pos
* @return
*/
public long getUnsignedInt(int pos) {
long ret = delegate.getUnsignedInt(pos);
return ret;
}
/**
* Returns the unsigned int
at position pos
in the Buffer, as a long
in Little Endian Byte Order.
* @param pos
* @return
*/
public long getUnsignedIntLE(int pos) {
long ret = delegate.getUnsignedIntLE(pos);
return ret;
}
/**
* Returns the long
at position pos
in the Buffer.
* @param pos
* @return
*/
public long getLong(int pos) {
long ret = delegate.getLong(pos);
return ret;
}
/**
* Gets a 64-bit long integer at the specified absolute index
in this buffer in Little Endian Byte Order.
* @param pos
* @return
*/
public long getLongLE(int pos) {
long ret = delegate.getLongLE(pos);
return ret;
}
/**
* Returns the double
at position pos
in the Buffer.
* @param pos
* @return
*/
public double getDouble(int pos) {
double ret = delegate.getDouble(pos);
return ret;
}
/**
* Returns the float
at position pos
in the Buffer.
* @param pos
* @return
*/
public float getFloat(int pos) {
float ret = delegate.getFloat(pos);
return ret;
}
/**
* Returns the short
at position pos
in the Buffer.
* @param pos
* @return
*/
public short getShort(int pos) {
short ret = delegate.getShort(pos);
return ret;
}
/**
* Gets a 16-bit short integer at the specified absolute index
in this buffer in Little Endian Byte Order.
* @param pos
* @return
*/
public short getShortLE(int pos) {
short ret = delegate.getShortLE(pos);
return ret;
}
/**
* Returns the unsigned short
at position pos
in the Buffer, as an int
.
* @param pos
* @return
*/
public int getUnsignedShort(int pos) {
int ret = delegate.getUnsignedShort(pos);
return ret;
}
/**
* Gets an unsigned 16-bit short integer at the specified absolute index
in this buffer in Little Endian Byte Order.
* @param pos
* @return
*/
public int getUnsignedShortLE(int pos) {
int ret = delegate.getUnsignedShortLE(pos);
return ret;
}
/**
* Gets a 24-bit medium integer at the specified absolute index
in this buffer.
* @param pos
* @return
*/
public int getMedium(int pos) {
int ret = delegate.getMedium(pos);
return ret;
}
/**
* Gets a 24-bit medium integer at the specified absolute index
in this buffer in the Little Endian Byte Order.
* @param pos
* @return
*/
public int getMediumLE(int pos) {
int ret = delegate.getMediumLE(pos);
return ret;
}
/**
* Gets an unsigned 24-bit medium integer at the specified absolute index
in this buffer.
* @param pos
* @return
*/
public int getUnsignedMedium(int pos) {
int ret = delegate.getUnsignedMedium(pos);
return ret;
}
/**
* Gets an unsigned 24-bit medium integer at the specified absolute index
in this buffer in Little Endian Byte Order.
* @param pos
* @return
*/
public int getUnsignedMediumLE(int pos) {
int ret = delegate.getUnsignedMediumLE(pos);
return ret;
}
/**
* Returns a copy of a sub-sequence the Buffer as a {@link io.vertx.rxjava3.core.buffer.Buffer} starting at position start
* and ending at position end - 1
* @param start
* @param end
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer getBuffer(int start, int end) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.getBuffer(start, end));
return ret;
}
/**
* Returns a copy of a sub-sequence the Buffer as a String
starting at position start
* and ending at position end - 1
interpreted as a String in the specified encoding
* @param start
* @param end
* @param enc
* @return
*/
public java.lang.String getString(int start, int end, java.lang.String enc) {
java.lang.String ret = delegate.getString(start, end, enc);
return ret;
}
/**
* Returns a copy of a sub-sequence the Buffer as a String
starting at position start
* and ending at position end - 1
interpreted as a String in UTF-8 encoding
* @param start
* @param end
* @return
*/
public java.lang.String getString(int start, int end) {
java.lang.String ret = delegate.getString(start, end);
return ret;
}
/**
* Appends the specified Buffer
to the end of this Buffer. The buffer will expand as necessary to accommodate
* any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param buff
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendBuffer(io.vertx.rxjava3.core.buffer.Buffer buff) {
delegate.appendBuffer(buff.getDelegate());
return this;
}
/**
* Appends the specified Buffer
starting at the offset
using len
to the end of this Buffer. The buffer will expand as necessary to accommodate
* any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param buff
* @param offset
* @param len
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendBuffer(io.vertx.rxjava3.core.buffer.Buffer buff, int offset, int len) {
delegate.appendBuffer(buff.getDelegate(), offset, len);
return this;
}
/**
* Appends the specified byte
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendByte(byte b) {
delegate.appendByte(b);
return this;
}
/**
* Appends the specified unsigned byte
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendUnsignedByte(short b) {
delegate.appendUnsignedByte(b);
return this;
}
/**
* Appends the specified int
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendInt(int i) {
delegate.appendInt(i);
return this;
}
/**
* Appends the specified int
to the end of the Buffer in the Little Endian Byte Order. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendIntLE(int i) {
delegate.appendIntLE(i);
return this;
}
/**
* Appends the specified unsigned int
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendUnsignedInt(long i) {
delegate.appendUnsignedInt(i);
return this;
}
/**
* Appends the specified unsigned int
to the end of the Buffer in the Little Endian Byte Order. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendUnsignedIntLE(long i) {
delegate.appendUnsignedIntLE(i);
return this;
}
/**
* Appends the specified 24bit int
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendMedium(int i) {
delegate.appendMedium(i);
return this;
}
/**
* Appends the specified 24bit int
to the end of the Buffer in the Little Endian Byte Order. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendMediumLE(int i) {
delegate.appendMediumLE(i);
return this;
}
/**
* Appends the specified long
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param l
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendLong(long l) {
delegate.appendLong(l);
return this;
}
/**
* Appends the specified long
to the end of the Buffer in the Little Endian Byte Order. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param l
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendLongLE(long l) {
delegate.appendLongLE(l);
return this;
}
/**
* Appends the specified short
to the end of the Buffer.The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendShort(short s) {
delegate.appendShort(s);
return this;
}
/**
* Appends the specified short
to the end of the Buffer in the Little Endian Byte Order.The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendShortLE(short s) {
delegate.appendShortLE(s);
return this;
}
/**
* Appends the specified unsigned short
to the end of the Buffer.The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendUnsignedShort(int s) {
delegate.appendUnsignedShort(s);
return this;
}
/**
* Appends the specified unsigned short
to the end of the Buffer in the Little Endian Byte Order.The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendUnsignedShortLE(int s) {
delegate.appendUnsignedShortLE(s);
return this;
}
/**
* Appends the specified float
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param f
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendFloat(float f) {
delegate.appendFloat(f);
return this;
}
/**
* Appends the specified double
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param d
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendDouble(double d) {
delegate.appendDouble(d);
return this;
}
/**
* Appends the specified String
to the end of the Buffer with the encoding as specified by enc
.
* The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param str
* @param enc
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendString(java.lang.String str, java.lang.String enc) {
delegate.appendString(str, enc);
return this;
}
/**
* Appends the specified String str
to the end of the Buffer with UTF-8 encoding.
* The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together
* @param str
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendString(java.lang.String str) {
delegate.appendString(str);
return this;
}
/**
* Sets the byte
at position pos
in the Buffer to the value b
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setByte(int pos, byte b) {
delegate.setByte(pos, b);
return this;
}
/**
* Sets the unsigned byte
at position pos
in the Buffer to the value b
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setUnsignedByte(int pos, short b) {
delegate.setUnsignedByte(pos, b);
return this;
}
/**
* Sets the int
at position pos
in the Buffer to the value i
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setInt(int pos, int i) {
delegate.setInt(pos, i);
return this;
}
/**
* Sets the int
at position pos
in the Buffer to the value i
in the Little Endian Byte Order.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setIntLE(int pos, int i) {
delegate.setIntLE(pos, i);
return this;
}
/**
* Sets the unsigned int
at position pos
in the Buffer to the value i
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setUnsignedInt(int pos, long i) {
delegate.setUnsignedInt(pos, i);
return this;
}
/**
* Sets the unsigned int
at position pos
in the Buffer to the value i
in the Little Endian Byte Order.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setUnsignedIntLE(int pos, long i) {
delegate.setUnsignedIntLE(pos, i);
return this;
}
/**
* Sets the 24bit int
at position pos
in the Buffer to the value i
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setMedium(int pos, int i) {
delegate.setMedium(pos, i);
return this;
}
/**
* Sets the 24bit int
at position pos
in the Buffer to the value i
. in the Little Endian Byte Order
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param i
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setMediumLE(int pos, int i) {
delegate.setMediumLE(pos, i);
return this;
}
/**
* Sets the long
at position pos
in the Buffer to the value l
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param l
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setLong(int pos, long l) {
delegate.setLong(pos, l);
return this;
}
/**
* Sets the long
at position pos
in the Buffer to the value l
in the Little Endian Byte Order.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param l
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setLongLE(int pos, long l) {
delegate.setLongLE(pos, l);
return this;
}
/**
* Sets the double
at position pos
in the Buffer to the value d
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param d
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setDouble(int pos, double d) {
delegate.setDouble(pos, d);
return this;
}
/**
* Sets the float
at position pos
in the Buffer to the value f
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param f
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setFloat(int pos, float f) {
delegate.setFloat(pos, f);
return this;
}
/**
* Sets the short
at position pos
in the Buffer to the value s
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setShort(int pos, short s) {
delegate.setShort(pos, s);
return this;
}
/**
* Sets the short
at position pos
in the Buffer to the value s
in the Little Endian Byte Order.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setShortLE(int pos, short s) {
delegate.setShortLE(pos, s);
return this;
}
/**
* Sets the unsigned short
at position pos
in the Buffer to the value s
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setUnsignedShort(int pos, int s) {
delegate.setUnsignedShort(pos, s);
return this;
}
/**
* Sets the unsigned short
at position pos
in the Buffer to the value s
in the Little Endian Byte Order.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param s
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setUnsignedShortLE(int pos, int s) {
delegate.setUnsignedShortLE(pos, s);
return this;
}
/**
* Sets the bytes at position pos
in the Buffer to the bytes represented by the Buffer b
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setBuffer(int pos, io.vertx.rxjava3.core.buffer.Buffer b) {
delegate.setBuffer(pos, b.getDelegate());
return this;
}
/**
* Sets the bytes at position pos
in the Buffer to the bytes represented by the Buffer b
on the given offset
and len
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @param offset
* @param len
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setBuffer(int pos, io.vertx.rxjava3.core.buffer.Buffer b, int offset, int len) {
delegate.setBuffer(pos, b.getDelegate(), offset, len);
return this;
}
/**
* Sets the bytes at position pos
in the Buffer to the value of str
encoded in UTF-8.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param str
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setString(int pos, java.lang.String str) {
delegate.setString(pos, str);
return this;
}
/**
* Sets the bytes at position pos
in the Buffer to the value of str
encoded in encoding enc
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param str
* @param enc
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setString(int pos, java.lang.String str, java.lang.String enc) {
delegate.setString(pos, str, enc);
return this;
}
/**
* Returns the length of the buffer, measured in bytes.
* All positions are indexed from zero.
* @return
*/
public int length() {
int ret = delegate.length();
return ret;
}
/**
* Returns a copy of the entire Buffer.
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer copy() {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.copy());
return ret;
}
/**
* Returns a slice of this buffer. Modifying the content
* of the returned buffer or this buffer affects each other's content
* while they maintain separate indexes and marks.
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer slice() {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.slice());
return ret;
}
/**
* Returns a slice of this buffer. Modifying the content
* of the returned buffer or this buffer affects each other's content
* while they maintain separate indexes and marks.
* @param start
* @param end
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer slice(int start, int end) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.slice(start, end));
return ret;
}
/**
* Create a new buffer from a byte[]. The byte[] will be copied to form the buffer.
* @param bytes the byte array
* @return the buffer
*/
public static io.vertx.rxjava3.core.buffer.Buffer buffer(byte[] bytes) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)io.vertx.core.buffer.Buffer.buffer(bytes));
return ret;
}
/**
*
* Create a new buffer from a Netty ByteBuf
.
* Note that the returned buffer is backed by given Netty ByteBuf,
* so changes in the returned buffer are reflected in given Netty ByteBuf, and vice-versa.
*
*
* For example, both buffers in the code below share their data:
*
*
* Buffer src = Buffer.buffer();
* Buffer clone = Buffer.buffer(src.getByteBuf());
*
* @param byteBuf the Netty ByteBuf
* @return the buffer
*/
@Deprecated()
public static io.vertx.rxjava3.core.buffer.Buffer buffer(io.netty.buffer.ByteBuf byteBuf) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)io.vertx.core.buffer.Buffer.buffer(byteBuf));
return ret;
}
/**
* Returns a String
representation of the Buffer with the encoding specified by enc
* @param enc
* @return
*/
public java.lang.String toString(java.nio.charset.Charset enc) {
java.lang.String ret = delegate.toString(enc);
return ret;
}
/**
* Returns a copy of the entire Buffer as a byte[]
* @return
*/
public byte[] getBytes() {
byte[] ret = delegate.getBytes();
return ret;
}
/**
* Returns a copy of a sub-sequence the Buffer as a byte[]
starting at position start
* and ending at position end - 1
* @param start
* @param end
* @return
*/
public byte[] getBytes(int start, int end) {
byte[] ret = delegate.getBytes(start, end);
return ret;
}
/**
* Transfers the content of the Buffer into a byte[]
.
* @param dst the destination byte array
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer getBytes(byte[] dst) {
delegate.getBytes(dst);
return this;
}
/**
* Transfers the content of the Buffer into a byte[]
at the specific destination.
* @param dst the destination byte array
* @param dstIndex
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer getBytes(byte[] dst, int dstIndex) {
delegate.getBytes(dst, dstIndex);
return this;
}
/**
* Transfers the content of the Buffer starting at position start
and ending at position end - 1
* into a byte[]
.
* @param start
* @param end
* @param dst the destination byte array
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer getBytes(int start, int end, byte[] dst) {
delegate.getBytes(start, end, dst);
return this;
}
/**
* Transfers the content of the Buffer starting at position start
and ending at position end - 1
* into a byte[]
at the specific destination.
* @param start
* @param end
* @param dst the destination byte array
* @param dstIndex
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer getBytes(int start, int end, byte[] dst, int dstIndex) {
delegate.getBytes(start, end, dst, dstIndex);
return this;
}
/**
* Appends the specified byte[]
to the end of the Buffer. The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param bytes
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendBytes(byte[] bytes) {
delegate.appendBytes(bytes);
return this;
}
/**
* Appends the specified number of bytes from byte[]
to the end of the Buffer, starting at the given offset.
* The buffer will expand as necessary to accommodate any bytes written.
* Returns a reference to this
so multiple operations can be appended together.
* @param bytes
* @param offset
* @param len
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer appendBytes(byte[] bytes, int offset, int len) {
delegate.appendBytes(bytes, offset, len);
return this;
}
/**
* Sets the bytes at position pos
in the Buffer to the bytes represented by the ByteBuffer b
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setBytes(int pos, java.nio.ByteBuffer b) {
delegate.setBytes(pos, b);
return this;
}
/**
* Sets the bytes at position pos
in the Buffer to the bytes represented by the byte[] b
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setBytes(int pos, byte[] b) {
delegate.setBytes(pos, b);
return this;
}
/**
* Sets the given number of bytes at position pos
in the Buffer to the bytes represented by the byte[] b
.
* The buffer will expand as necessary to accommodate any value written.
* @param pos
* @param b
* @param offset
* @param len
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer setBytes(int pos, byte[] b, int offset, int len) {
delegate.setBytes(pos, b, offset, len);
return this;
}
/**
* Returns the Buffer as a Netty ByteBuf
.
*
* The returned buffer is a duplicate that maintain its own indices.
* @return
*/
@Deprecated()
public io.netty.buffer.ByteBuf getByteBuf() {
io.netty.buffer.ByteBuf ret = delegate.getByteBuf();
return ret;
}
public static Buffer newInstance(io.vertx.core.buffer.Buffer arg) {
return arg != null ? new Buffer(arg) : null;
}
}