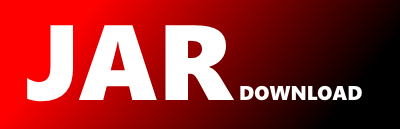
io.vertx.rxjava3.core.file.FileSystem Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.file;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Contains a broad set of operations for manipulating files on the file system.
*
* A (potential) blocking and non blocking version of each operation is provided.
*
* The non blocking versions take a handler which is called when the operation completes or an error occurs.
*
* The blocking versions are named xxxBlocking
and return the results, or throw exceptions directly.
* In many cases, depending on the operating system and file system some of the potentially blocking operations
* can return quickly, which is why we provide them, but it's highly recommended that you test how long they take to
* return in your particular application before using them on an event loop.
*
* Please consult the documentation for more information on file system support.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.file.FileSystem original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.file.FileSystem.class)
public class FileSystem {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FileSystem that = (FileSystem) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new FileSystem((io.vertx.core.file.FileSystem) obj),
FileSystem::getDelegate
);
private final io.vertx.core.file.FileSystem delegate;
public FileSystem(io.vertx.core.file.FileSystem delegate) {
this.delegate = delegate;
}
public FileSystem(Object delegate) {
this.delegate = (io.vertx.core.file.FileSystem)delegate;
}
public io.vertx.core.file.FileSystem getDelegate() {
return delegate;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* The copy will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable copy(java.lang.String from, java.lang.String to) {
io.reactivex.rxjava3.core.Completable ret = rxCopy(from, to);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* The copy will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxCopy(java.lang.String from, java.lang.String to) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.copy(from, to, handler);
});
}
/**
* Copy a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable copy(java.lang.String from, java.lang.String to, io.vertx.core.file.CopyOptions options) {
io.reactivex.rxjava3.core.Completable ret = rxCopy(from, to, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxCopy(java.lang.String from, java.lang.String to, io.vertx.core.file.CopyOptions options) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.copy(from, to, options, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#copy}
* @param from
* @param to
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem copyBlocking(java.lang.String from, java.lang.String to) {
delegate.copyBlocking(from, to);
return this;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* If recursive
is true
and from
represents a directory, then the directory and its contents
* will be copied recursively to the destination to
.
*
* The copy will fail if the destination if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param recursive
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable copyRecursive(java.lang.String from, java.lang.String to, boolean recursive) {
io.reactivex.rxjava3.core.Completable ret = rxCopyRecursive(from, to, recursive);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Copy a file from the path from
to path to
, asynchronously.
*
* If recursive
is true
and from
represents a directory, then the directory and its contents
* will be copied recursively to the destination to
.
*
* The copy will fail if the destination if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @param recursive
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxCopyRecursive(java.lang.String from, java.lang.String to, boolean recursive) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.copyRecursive(from, to, recursive, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#copyRecursive}
* @param from
* @param to
* @param recursive
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem copyRecursiveBlocking(java.lang.String from, java.lang.String to, boolean recursive) {
delegate.copyRecursiveBlocking(from, to, recursive);
return this;
}
/**
* Move a file from the path from
to path to
, asynchronously.
*
* The move will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable move(java.lang.String from, java.lang.String to) {
io.reactivex.rxjava3.core.Completable ret = rxMove(from, to);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Move a file from the path from
to path to
, asynchronously.
*
* The move will fail if the destination already exists.
* @param from the path to copy from
* @param to the path to copy to
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxMove(java.lang.String from, java.lang.String to) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.move(from, to, handler);
});
}
/**
* Move a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable move(java.lang.String from, java.lang.String to, io.vertx.core.file.CopyOptions options) {
io.reactivex.rxjava3.core.Completable ret = rxMove(from, to, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Move a file from the path from
to path to
, asynchronously.
* @param from the path to copy from
* @param to the path to copy to
* @param options options describing how the file should be copied
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxMove(java.lang.String from, java.lang.String to, io.vertx.core.file.CopyOptions options) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.move(from, to, options, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#move}
* @param from
* @param to
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem moveBlocking(java.lang.String from, java.lang.String to) {
delegate.moveBlocking(from, to);
return this;
}
/**
* Truncate the file represented by path
to length len
in bytes, asynchronously.
*
* The operation will fail if the file does not exist or len
is less than zero
.
* @param path the path to the file
* @param len the length to truncate it to
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable truncate(java.lang.String path, long len) {
io.reactivex.rxjava3.core.Completable ret = rxTruncate(path, len);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Truncate the file represented by path
to length len
in bytes, asynchronously.
*
* The operation will fail if the file does not exist or len
is less than zero
.
* @param path the path to the file
* @param len the length to truncate it to
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxTruncate(java.lang.String path, long len) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.truncate(path, len, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#truncate}
* @param path
* @param len
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem truncateBlocking(java.lang.String path, long len) {
delegate.truncateBlocking(path, len);
return this;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
*
* The permission String takes the form rwxr-x--- as
* specified here.
* @param path the path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable chmod(java.lang.String path, java.lang.String perms) {
io.reactivex.rxjava3.core.Completable ret = rxChmod(path, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
*
* The permission String takes the form rwxr-x--- as
* specified here.
* @param path the path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxChmod(java.lang.String path, java.lang.String perms) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.chmod(path, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem #chmod(String, String, Handler)}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem chmodBlocking(java.lang.String path, java.lang.String perms) {
delegate.chmodBlocking(path, perms);
return this;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
* The permission String takes the form rwxr-x--- as
* specified in {here}.
*
* If the file is directory then all contents will also have their permissions changed recursively. Any directory permissions will
* be set to dirPerms
, whilst any normal file permissions will be set to perms
.
* @param path the path to the file
* @param perms the permissions string
* @param dirPerms the directory permissions
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable chmodRecursive(java.lang.String path, java.lang.String perms, java.lang.String dirPerms) {
io.reactivex.rxjava3.core.Completable ret = rxChmodRecursive(path, perms, dirPerms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Change the permissions on the file represented by path
to perms
, asynchronously.
* The permission String takes the form rwxr-x--- as
* specified in {here}.
*
* If the file is directory then all contents will also have their permissions changed recursively. Any directory permissions will
* be set to dirPerms
, whilst any normal file permissions will be set to perms
.
* @param path the path to the file
* @param perms the permissions string
* @param dirPerms the directory permissions
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxChmodRecursive(java.lang.String path, java.lang.String perms, java.lang.String dirPerms) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.chmodRecursive(path, perms, dirPerms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#chmodRecursive}
* @param path
* @param perms
* @param dirPerms
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem chmodRecursiveBlocking(java.lang.String path, java.lang.String perms, java.lang.String dirPerms) {
delegate.chmodRecursiveBlocking(path, perms, dirPerms);
return this;
}
/**
* Change the ownership on the file represented by path
to user
and {code group}, asynchronously.
* @param path the path to the file
* @param user the user name, null
will not change the user name
* @param group the user group, null
will not change the user group name
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable chown(java.lang.String path, java.lang.String user, java.lang.String group) {
io.reactivex.rxjava3.core.Completable ret = rxChown(path, user, group);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Change the ownership on the file represented by path
to user
and {code group}, asynchronously.
* @param path the path to the file
* @param user the user name, null
will not change the user name
* @param group the user group, null
will not change the user group name
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxChown(java.lang.String path, java.lang.String user, java.lang.String group) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.chown(path, user, group, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#chown}
*
* @param path
* @param user
* @param group
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem chownBlocking(java.lang.String path, java.lang.String user, java.lang.String group) {
delegate.chownBlocking(path, user, group);
return this;
}
/**
* Obtain properties for the file represented by path
, asynchronously.
*
* If the file is a link, the link will be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single props(java.lang.String path) {
io.reactivex.rxjava3.core.Single ret = rxProps(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Obtain properties for the file represented by path
, asynchronously.
*
* If the file is a link, the link will be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxProps(java.lang.String path) {
return AsyncResultSingle.toSingle( handler -> {
delegate.props(path, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)event))));
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#props}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileProps propsBlocking(java.lang.String path) {
io.vertx.rxjava3.core.file.FileProps ret = io.vertx.rxjava3.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)delegate.propsBlocking(path));
return ret;
}
/**
* Obtain properties for the link represented by path
, asynchronously.
*
* The link will not be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single lprops(java.lang.String path) {
io.reactivex.rxjava3.core.Single ret = rxLprops(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Obtain properties for the link represented by path
, asynchronously.
*
* The link will not be followed.
* @param path the path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxLprops(java.lang.String path) {
return AsyncResultSingle.toSingle( handler -> {
delegate.lprops(path, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)event))));
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#lprops}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileProps lpropsBlocking(java.lang.String path) {
io.vertx.rxjava3.core.file.FileProps ret = io.vertx.rxjava3.core.file.FileProps.newInstance((io.vertx.core.file.FileProps)delegate.lpropsBlocking(path));
return ret;
}
/**
* Create a hard link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable link(java.lang.String link, java.lang.String existing) {
io.reactivex.rxjava3.core.Completable ret = rxLink(link, existing);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create a hard link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxLink(java.lang.String link, java.lang.String existing) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.link(link, existing, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#link}
* @param link
* @param existing
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem linkBlocking(java.lang.String link, java.lang.String existing) {
delegate.linkBlocking(link, existing);
return this;
}
/**
* Create a symbolic link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable symlink(java.lang.String link, java.lang.String existing) {
io.reactivex.rxjava3.core.Completable ret = rxSymlink(link, existing);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create a symbolic link on the file system from link
to existing
, asynchronously.
* @param link the link
* @param existing the link destination
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxSymlink(java.lang.String link, java.lang.String existing) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.symlink(link, existing, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#link}
* @param link
* @param existing
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem symlinkBlocking(java.lang.String link, java.lang.String existing) {
delegate.symlinkBlocking(link, existing);
return this;
}
/**
* Unlinks the link on the file system represented by the path link
, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable unlink(java.lang.String link) {
io.reactivex.rxjava3.core.Completable ret = rxUnlink(link);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Unlinks the link on the file system represented by the path link
, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxUnlink(java.lang.String link) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.unlink(link, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#unlink}
* @param link
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem unlinkBlocking(java.lang.String link) {
delegate.unlinkBlocking(link);
return this;
}
/**
* Returns the path representing the file that the symbolic link specified by link
points to, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single readSymlink(java.lang.String link) {
io.reactivex.rxjava3.core.Single ret = rxReadSymlink(link);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the path representing the file that the symbolic link specified by link
points to, asynchronously.
* @param link the link
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxReadSymlink(java.lang.String link) {
return AsyncResultSingle.toSingle( handler -> {
delegate.readSymlink(link, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#readSymlink}
* @param link
* @return
*/
public java.lang.String readSymlinkBlocking(java.lang.String link) {
java.lang.String ret = delegate.readSymlinkBlocking(link);
return ret;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable delete(java.lang.String path) {
io.reactivex.rxjava3.core.Completable ret = rxDelete(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxDelete(java.lang.String path) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.delete(path, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#delete}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem deleteBlocking(java.lang.String path) {
delegate.deleteBlocking(path);
return this;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
*
* If the path represents a directory and recursive = true
then the directory and its contents will be
* deleted recursively.
* @param path path to the file
* @param recursive delete recursively?
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable deleteRecursive(java.lang.String path, boolean recursive) {
io.reactivex.rxjava3.core.Completable ret = rxDeleteRecursive(path, recursive);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Deletes the file represented by the specified path
, asynchronously.
*
* If the path represents a directory and recursive = true
then the directory and its contents will be
* deleted recursively.
* @param path path to the file
* @param recursive delete recursively?
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxDeleteRecursive(java.lang.String path, boolean recursive) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.deleteRecursive(path, recursive, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#deleteRecursive}
* @param path
* @param recursive
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem deleteRecursiveBlocking(java.lang.String path, boolean recursive) {
delegate.deleteRecursiveBlocking(path, recursive);
return this;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable mkdir(java.lang.String path) {
io.reactivex.rxjava3.core.Completable ret = rxMkdir(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxMkdir(java.lang.String path) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.mkdir(path, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#mkdir}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem mkdirBlocking(java.lang.String path) {
delegate.mkdirBlocking(path);
return this;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable mkdir(java.lang.String path, java.lang.String perms) {
io.reactivex.rxjava3.core.Completable ret = rxMkdir(path, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create the directory represented by path
, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the directory already exists.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxMkdir(java.lang.String path, java.lang.String perms) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.mkdir(path, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#mkdir}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem mkdirBlocking(java.lang.String path, java.lang.String perms) {
delegate.mkdirBlocking(path, perms);
return this;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable mkdirs(java.lang.String path) {
io.reactivex.rxjava3.core.Completable ret = rxMkdirs(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxMkdirs(java.lang.String path) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.mkdirs(path, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#mkdirs}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem mkdirsBlocking(java.lang.String path) {
delegate.mkdirsBlocking(path);
return this;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable mkdirs(java.lang.String path, java.lang.String perms) {
io.reactivex.rxjava3.core.Completable ret = rxMkdirs(path, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create the directory represented by path
and any non existent parents, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
* The operation will fail if the path
already exists but is not a directory.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxMkdirs(java.lang.String path, java.lang.String perms) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.mkdirs(path, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#mkdirs}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem mkdirsBlocking(java.lang.String path, java.lang.String perms) {
delegate.mkdirsBlocking(path, perms);
return this;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single> readDir(java.lang.String path) {
io.reactivex.rxjava3.core.Single> ret = rxReadDir(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single> rxReadDir(java.lang.String path) {
return AsyncResultSingle.toSingle( handler -> {
delegate.readDir(path, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#readDir}
* @param path
* @return
*/
public java.util.List readDirBlocking(java.lang.String path) {
java.util.List ret = delegate.readDirBlocking(path);
return ret;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The parameter filter
is a regular expression. If filter
is specified then only the paths that
* match @{filter}will be returned.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the directory
* @param filter the filter expression
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single> readDir(java.lang.String path, java.lang.String filter) {
io.reactivex.rxjava3.core.Single> ret = rxReadDir(path, filter);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Read the contents of the directory specified by path
, asynchronously.
*
* The parameter filter
is a regular expression. If filter
is specified then only the paths that
* match @{filter}will be returned.
*
* The result is an array of String representing the paths of the files inside the directory.
* @param path path to the directory
* @param filter the filter expression
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single> rxReadDir(java.lang.String path, java.lang.String filter) {
return AsyncResultSingle.toSingle( handler -> {
delegate.readDir(path, filter, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#readDir}
* @param path
* @param filter
* @return
*/
public java.util.List readDirBlocking(java.lang.String path, java.lang.String filter) {
java.util.List ret = delegate.readDirBlocking(path, filter);
return ret;
}
/**
* Reads the entire file as represented by the path path
as a , asynchronously.
*
* Do not use this method to read very large files or you risk running out of available RAM.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single readFile(java.lang.String path) {
io.reactivex.rxjava3.core.Single ret = rxReadFile(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Reads the entire file as represented by the path path
as a , asynchronously.
*
* Do not use this method to read very large files or you risk running out of available RAM.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxReadFile(java.lang.String path) {
return AsyncResultSingle.toSingle( handler -> {
delegate.readFile(path, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)event))));
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#readFile}
* @param path
* @return
*/
public io.vertx.rxjava3.core.buffer.Buffer readFileBlocking(java.lang.String path) {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.readFileBlocking(path));
return ret;
}
/**
* Creates the file, and writes the specified Buffer data
to the file represented by the path path
,
* asynchronously.
* @param path path to the file
* @param data
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable writeFile(java.lang.String path, io.vertx.rxjava3.core.buffer.Buffer data) {
io.reactivex.rxjava3.core.Completable ret = rxWriteFile(path, data);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates the file, and writes the specified Buffer data
to the file represented by the path path
,
* asynchronously.
* @param path path to the file
* @param data
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxWriteFile(java.lang.String path, io.vertx.rxjava3.core.buffer.Buffer data) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.writeFile(path, data.getDelegate(), handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#writeFile}
* @param path
* @param data
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem writeFileBlocking(java.lang.String path, io.vertx.rxjava3.core.buffer.Buffer data) {
delegate.writeFileBlocking(path, data.getDelegate());
return this;
}
/**
* Open the file represented by path
, asynchronously.
*
* The file is opened for both reading and writing. If the file does not already exist it will be created.
* @param path path to the file
* @param options options describing how the file should be opened
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single open(java.lang.String path, io.vertx.core.file.OpenOptions options) {
io.reactivex.rxjava3.core.Single ret = rxOpen(path, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Open the file represented by path
, asynchronously.
*
* The file is opened for both reading and writing. If the file does not already exist it will be created.
* @param path path to the file
* @param options options describing how the file should be opened
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxOpen(java.lang.String path, io.vertx.core.file.OpenOptions options) {
return AsyncResultSingle.toSingle( handler -> {
delegate.open(path, options, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.file.AsyncFile.newInstance((io.vertx.core.file.AsyncFile)event))));
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#open}
* @param path
* @param options
* @return
*/
public io.vertx.rxjava3.core.file.AsyncFile openBlocking(java.lang.String path, io.vertx.core.file.OpenOptions options) {
io.vertx.rxjava3.core.file.AsyncFile ret = io.vertx.rxjava3.core.file.AsyncFile.newInstance((io.vertx.core.file.AsyncFile)delegate.openBlocking(path, options));
return ret;
}
/**
* Creates an empty file with the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable createFile(java.lang.String path) {
io.reactivex.rxjava3.core.Completable ret = rxCreateFile(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates an empty file with the specified path
, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxCreateFile(java.lang.String path) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.createFile(path, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createFile}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem createFileBlocking(java.lang.String path) {
delegate.createFileBlocking(path);
return this;
}
/**
* Creates an empty file with the specified path
and permissions perms
, asynchronously.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable createFile(java.lang.String path, java.lang.String perms) {
io.reactivex.rxjava3.core.Completable ret = rxCreateFile(path, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates an empty file with the specified path
and permissions perms
, asynchronously.
* @param path path to the file
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxCreateFile(java.lang.String path, java.lang.String perms) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.createFile(path, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createFile}
* @param path
* @param perms
* @return
*/
public io.vertx.rxjava3.core.file.FileSystem createFileBlocking(java.lang.String path, java.lang.String perms) {
delegate.createFileBlocking(path, perms);
return this;
}
/**
* Determines whether the file as specified by the path path
exists, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single exists(java.lang.String path) {
io.reactivex.rxjava3.core.Single ret = rxExists(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Determines whether the file as specified by the path path
exists, asynchronously.
* @param path path to the file
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxExists(java.lang.String path) {
return AsyncResultSingle.toSingle( handler -> {
delegate.exists(path, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#exists}
* @param path
* @return
*/
public boolean existsBlocking(java.lang.String path) {
boolean ret = delegate.existsBlocking(path);
return ret;
}
/**
* Returns properties of the file-system being used by the specified path
, asynchronously.
* @param path path to anywhere on the filesystem
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single fsProps(java.lang.String path) {
io.reactivex.rxjava3.core.Single ret = rxFsProps(path);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns properties of the file-system being used by the specified path
, asynchronously.
* @param path path to anywhere on the filesystem
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxFsProps(java.lang.String path) {
return AsyncResultSingle.toSingle( handler -> {
delegate.fsProps(path, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.file.FileSystemProps.newInstance((io.vertx.core.file.FileSystemProps)event))));
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#fsProps}
* @param path
* @return
*/
public io.vertx.rxjava3.core.file.FileSystemProps fsPropsBlocking(java.lang.String path) {
io.vertx.rxjava3.core.file.FileSystemProps ret = io.vertx.rxjava3.core.file.FileSystemProps.newInstance((io.vertx.core.file.FileSystemProps)delegate.fsPropsBlocking(path));
return ret;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single createTempDirectory(java.lang.String prefix) {
io.reactivex.rxjava3.core.Single ret = rxCreateTempDirectory(prefix);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxCreateTempDirectory(java.lang.String prefix) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createTempDirectory(prefix, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createTempDirectory}
* @param prefix
* @return
*/
public java.lang.String createTempDirectoryBlocking(java.lang.String prefix) {
java.lang.String ret = delegate.createTempDirectoryBlocking(prefix);
return ret;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single createTempDirectory(java.lang.String prefix, java.lang.String perms) {
io.reactivex.rxjava3.core.Single ret = rxCreateTempDirectory(prefix, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new directory in the default temporary-file directory, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxCreateTempDirectory(java.lang.String prefix, java.lang.String perms) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createTempDirectory(prefix, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createTempDirectory}
* @param prefix
* @param perms
* @return
*/
public java.lang.String createTempDirectoryBlocking(java.lang.String prefix, java.lang.String perms) {
java.lang.String ret = delegate.createTempDirectoryBlocking(prefix, perms);
return ret;
}
/**
* Creates a new directory in the directory provided by the path path
, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single createTempDirectory(java.lang.String dir, java.lang.String prefix, java.lang.String perms) {
io.reactivex.rxjava3.core.Single ret = rxCreateTempDirectory(dir, prefix, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new directory in the directory provided by the path path
, using the given
* prefix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxCreateTempDirectory(java.lang.String dir, java.lang.String prefix, java.lang.String perms) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createTempDirectory(dir, prefix, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createTempDirectory}
* @param dir
* @param prefix
* @param perms
* @return
*/
public java.lang.String createTempDirectoryBlocking(java.lang.String dir, java.lang.String prefix, java.lang.String perms) {
java.lang.String ret = delegate.createTempDirectoryBlocking(dir, prefix, perms);
return ret;
}
/**
* Creates a new file in the default temporary-file directory, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single createTempFile(java.lang.String prefix, java.lang.String suffix) {
io.reactivex.rxjava3.core.Single ret = rxCreateTempFile(prefix, suffix);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new file in the default temporary-file directory, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxCreateTempFile(java.lang.String prefix, java.lang.String suffix) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createTempFile(prefix, suffix, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createTempFile}
* @param prefix
* @param suffix
* @return
*/
public java.lang.String createTempFileBlocking(java.lang.String prefix, java.lang.String suffix) {
java.lang.String ret = delegate.createTempFileBlocking(prefix, suffix);
return ret;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single createTempFile(java.lang.String prefix, java.lang.String suffix, java.lang.String perms) {
io.reactivex.rxjava3.core.Single ret = rxCreateTempFile(prefix, suffix, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxCreateTempFile(java.lang.String prefix, java.lang.String suffix, java.lang.String perms) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createTempFile(prefix, suffix, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createTempFile}
* @param prefix
* @param suffix
* @param perms
* @return
*/
public java.lang.String createTempFileBlocking(java.lang.String prefix, java.lang.String suffix, java.lang.String perms) {
java.lang.String ret = delegate.createTempFileBlocking(prefix, suffix, perms);
return ret;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single createTempFile(java.lang.String dir, java.lang.String prefix, java.lang.String suffix, java.lang.String perms) {
io.reactivex.rxjava3.core.Single ret = rxCreateTempFile(dir, prefix, suffix, perms);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new file in the directory provided by the path dir
, using the given
* prefix and suffix to generate its name, asynchronously.
*
* The new directory will be created with permissions as specified by perms
.
*
* The permission String takes the form rwxr-x--- as specified
* in here.
*
*
* As with the File.createTempFile
methods, this method is only
* part of a temporary-file facility.A {@link java.lang.Runtime},
* or the {@link java.io.File} mechanism may be used to delete the directory automatically.
*
* @param dir the path to directory in which to create the directory
* @param prefix the prefix string to be used in generating the directory's name; may be null
* @param suffix the suffix string to be used in generating the file's name; may be null
, in which case ".tmp
" is used
* @param perms the permissions string
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxCreateTempFile(java.lang.String dir, java.lang.String prefix, java.lang.String suffix, java.lang.String perms) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createTempFile(dir, prefix, suffix, perms, handler);
});
}
/**
* Blocking version of {@link io.vertx.rxjava3.core.file.FileSystem#createTempFile}
* @param dir
* @param prefix
* @param suffix
* @param perms
* @return
*/
public java.lang.String createTempFileBlocking(java.lang.String dir, java.lang.String prefix, java.lang.String suffix, java.lang.String perms) {
java.lang.String ret = delegate.createTempFileBlocking(dir, prefix, suffix, perms);
return ret;
}
public static FileSystem newInstance(io.vertx.core.file.FileSystem arg) {
return arg != null ? new FileSystem(arg) : null;
}
}