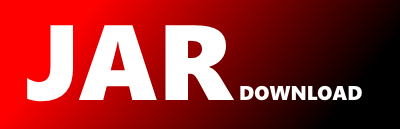
io.vertx.rxjava3.core.http.HttpClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.http;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* An asynchronous HTTP client.
*
* It allows you to make requests to HTTP servers, and a single client can make requests to any server.
*
* This gives the benefits of keep alive when the client is loaded but means we don't keep connections hanging around
* unnecessarily when there would be no benefits anyway.
*
* The client is designed to be reused between requests.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.HttpClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.HttpClient.class)
public class HttpClient implements io.vertx.rxjava3.core.metrics.Measured {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpClient that = (HttpClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpClient((io.vertx.core.http.HttpClient) obj),
HttpClient::getDelegate
);
private final io.vertx.core.http.HttpClient delegate;
public HttpClient(io.vertx.core.http.HttpClient delegate) {
this.delegate = delegate;
}
public HttpClient(Object delegate) {
this.delegate = (io.vertx.core.http.HttpClient)delegate;
}
public io.vertx.core.http.HttpClient getDelegate() {
return delegate;
}
/**
* Whether the metrics are enabled for this measured object
* @return true
if metrics are enabled
*/
public boolean isMetricsEnabled() {
boolean ret = delegate.isMetricsEnabled();
return ret;
}
/**
* Create an HTTP request to send to the server. The handler
* is called when the request is ready to be sent.
* @param options the request options
* @return
*/
public io.reactivex.rxjava3.core.Single request(io.vertx.core.http.RequestOptions options) {
io.reactivex.rxjava3.core.Single ret = rxRequest(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create an HTTP request to send to the server. The handler
* is called when the request is ready to be sent.
* @param options the request options
* @return
*/
public io.reactivex.rxjava3.core.Single rxRequest(io.vertx.core.http.RequestOptions options) {
return AsyncResultSingle.toSingle( handler -> {
delegate.request(options, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)event))));
});
}
/**
* Create an HTTP request to send to the server at the host
and port
. The handler
* is called when the request is ready to be sent.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return
*/
public io.reactivex.rxjava3.core.Single request(io.vertx.core.http.HttpMethod method, int port, java.lang.String host, java.lang.String requestURI) {
io.reactivex.rxjava3.core.Single ret = rxRequest(method, port, host, requestURI);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create an HTTP request to send to the server at the host
and port
. The handler
* is called when the request is ready to be sent.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return
*/
public io.reactivex.rxjava3.core.Single rxRequest(io.vertx.core.http.HttpMethod method, int port, java.lang.String host, java.lang.String requestURI) {
return AsyncResultSingle.toSingle( handler -> {
delegate.request(method, port, host, requestURI, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)event))));
});
}
/**
* Create an HTTP request to send to the server at the host
and default port. The handler
* is called when the request is ready to be sent.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return
*/
public io.reactivex.rxjava3.core.Single request(io.vertx.core.http.HttpMethod method, java.lang.String host, java.lang.String requestURI) {
io.reactivex.rxjava3.core.Single ret = rxRequest(method, host, requestURI);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create an HTTP request to send to the server at the host
and default port. The handler
* is called when the request is ready to be sent.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return
*/
public io.reactivex.rxjava3.core.Single rxRequest(io.vertx.core.http.HttpMethod method, java.lang.String host, java.lang.String requestURI) {
return AsyncResultSingle.toSingle( handler -> {
delegate.request(method, host, requestURI, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)event))));
});
}
/**
* Create an HTTP request to send to the server at the default host and port. The handler
* is called when the request is ready to be sent.
* @param method the HTTP method
* @param requestURI the relative URI
* @return
*/
public io.reactivex.rxjava3.core.Single request(io.vertx.core.http.HttpMethod method, java.lang.String requestURI) {
io.reactivex.rxjava3.core.Single ret = rxRequest(method, requestURI);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create an HTTP request to send to the server at the default host and port. The handler
* is called when the request is ready to be sent.
* @param method the HTTP method
* @param requestURI the relative URI
* @return
*/
public io.reactivex.rxjava3.core.Single rxRequest(io.vertx.core.http.HttpMethod method, java.lang.String requestURI) {
return AsyncResultSingle.toSingle( handler -> {
delegate.request(method, requestURI, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)event))));
});
}
/**
* Connect a WebSocket to the specified port, host and relative request URI
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single webSocket(int port, java.lang.String host, java.lang.String requestURI) {
io.reactivex.rxjava3.core.Single ret = rxWebSocket(port, host, requestURI);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Connect a WebSocket to the specified port, host and relative request URI
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxWebSocket(int port, java.lang.String host, java.lang.String requestURI) {
return AsyncResultSingle.toSingle( handler -> {
delegate.webSocket(port, host, requestURI, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.WebSocket.newInstance((io.vertx.core.http.WebSocket)event))));
});
}
/**
* Connect a WebSocket to the host and relative request URI and default port
* @param host the host
* @param requestURI the relative URI
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single webSocket(java.lang.String host, java.lang.String requestURI) {
io.reactivex.rxjava3.core.Single ret = rxWebSocket(host, requestURI);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Connect a WebSocket to the host and relative request URI and default port
* @param host the host
* @param requestURI the relative URI
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxWebSocket(java.lang.String host, java.lang.String requestURI) {
return AsyncResultSingle.toSingle( handler -> {
delegate.webSocket(host, requestURI, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.WebSocket.newInstance((io.vertx.core.http.WebSocket)event))));
});
}
/**
* Connect a WebSocket at the relative request URI using the default host and port
* @param requestURI the relative URI
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single webSocket(java.lang.String requestURI) {
io.reactivex.rxjava3.core.Single ret = rxWebSocket(requestURI);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Connect a WebSocket at the relative request URI using the default host and port
* @param requestURI the relative URI
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxWebSocket(java.lang.String requestURI) {
return AsyncResultSingle.toSingle( handler -> {
delegate.webSocket(requestURI, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.WebSocket.newInstance((io.vertx.core.http.WebSocket)event))));
});
}
/**
* Connect a WebSocket with the specified options.
* @param options the request options
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single webSocket(io.vertx.core.http.WebSocketConnectOptions options) {
io.reactivex.rxjava3.core.Single ret = rxWebSocket(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Connect a WebSocket with the specified options.
* @param options the request options
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxWebSocket(io.vertx.core.http.WebSocketConnectOptions options) {
return AsyncResultSingle.toSingle( handler -> {
delegate.webSocket(options, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.WebSocket.newInstance((io.vertx.core.http.WebSocket)event))));
});
}
/**
* Connect a WebSocket with the specified absolute url, with the specified headers, using
* the specified version of WebSockets, and the specified WebSocket sub protocols.
* @param url the absolute url
* @param headers the headers
* @param version the WebSocket version
* @param subProtocols the subprotocols to use
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single webSocketAbs(java.lang.String url, io.vertx.rxjava3.core.MultiMap headers, io.vertx.core.http.WebsocketVersion version, java.util.List subProtocols) {
io.reactivex.rxjava3.core.Single ret = rxWebSocketAbs(url, headers, version, subProtocols);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Connect a WebSocket with the specified absolute url, with the specified headers, using
* the specified version of WebSockets, and the specified WebSocket sub protocols.
* @param url the absolute url
* @param headers the headers
* @param version the WebSocket version
* @param subProtocols the subprotocols to use
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxWebSocketAbs(java.lang.String url, io.vertx.rxjava3.core.MultiMap headers, io.vertx.core.http.WebsocketVersion version, java.util.List subProtocols) {
return AsyncResultSingle.toSingle( handler -> {
delegate.webSocketAbs(url, headers.getDelegate(), version, subProtocols, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.WebSocket.newInstance((io.vertx.core.http.WebSocket)event))));
});
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClient#updateSSLOptions} but supplying a handler that will be called when the update
* happened (or has failed).
* @param options the new SSL options
* @return
*/
public io.reactivex.rxjava3.core.Single updateSSLOptions(io.vertx.core.net.SSLOptions options) {
io.reactivex.rxjava3.core.Single ret = rxUpdateSSLOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClient#updateSSLOptions} but supplying a handler that will be called when the update
* happened (or has failed).
* @param options the new SSL options
* @return
*/
public io.reactivex.rxjava3.core.Single rxUpdateSSLOptions(io.vertx.core.net.SSLOptions options) {
return AsyncResultSingle.toSingle( handler -> {
delegate.updateSSLOptions(options, handler);
});
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClient#updateSSLOptions} but supplying a handler that will be called when the update
* happened (or has failed).
* @param options the new SSL options
* @param force force the update when options are equals
* @return
*/
public io.reactivex.rxjava3.core.Single updateSSLOptions(io.vertx.core.net.SSLOptions options, boolean force) {
io.reactivex.rxjava3.core.Single ret = rxUpdateSSLOptions(options, force);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClient#updateSSLOptions} but supplying a handler that will be called when the update
* happened (or has failed).
* @param options the new SSL options
* @param force force the update when options are equals
* @return
*/
public io.reactivex.rxjava3.core.Single rxUpdateSSLOptions(io.vertx.core.net.SSLOptions options, boolean force) {
return AsyncResultSingle.toSingle( handler -> {
delegate.updateSSLOptions(options, force, handler);
});
}
/**
* Set a connection handler for the client. This handler is called when a new connection is established.
* @param handler
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.core.http.HttpClient connectionHandler(io.vertx.core.Handler handler) {
delegate.connectionHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.core.http.HttpConnection.newInstance((io.vertx.core.http.HttpConnection)event)));
return this;
}
/**
* Set a redirect handler for the http client.
*
* The redirect handler is called when a 3xx
response is received and the request is configured to
* follow redirects with {@link io.vertx.rxjava3.core.http.HttpClientRequest#setFollowRedirects}.
*
* The redirect handler is passed the {@link io.vertx.rxjava3.core.http.HttpClientResponse}, it can return an {@link io.vertx.rxjava3.core.http.HttpClientRequest} or null
.
*
* - when null is returned, the original response is processed by the original request response handler
* - when a new
Future
is returned, the client will send this new request
*
* The new request will get a copy of the previous request headers unless headers are set. In this case,
* the client assumes that the redirect handler exclusively managers the headers of the new request.
*
* The handler must return a Future
unsent so the client can further configure it and send it.
* @param handler the new redirect handler
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.core.http.HttpClient redirectHandler(java.util.function.Function> handler) {
delegate.redirectHandler(new Function>() {
public io.vertx.core.Future apply(io.vertx.core.http.HttpClientResponse arg) {
io.reactivex.rxjava3.core.Single ret = handler.apply(io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)arg));
return io.vertx.rxjava3.SingleHelper.toFuture(ret, obj -> obj);
}
});
return this;
}
/**
* Close the client. Closing will close down any pooled connections.
* Clients should always be closed after use.
* @return
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Close the client. Closing will close down any pooled connections.
* Clients should always be closed after use.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.close(handler);
});
}
public static HttpClient newInstance(io.vertx.core.http.HttpClient arg) {
return arg != null ? new HttpClient(arg) : null;
}
}