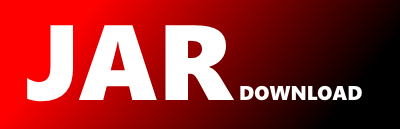
io.vertx.rxjava3.core.http.HttpClientRequest Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.http;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents a client-side HTTP request.
*
* Instances are created by an {@link io.vertx.rxjava3.core.http.HttpClient} instance, via one of the methods corresponding to the
* specific HTTP methods, or the generic request methods. On creation the request will not have been written to the
* wire.
*
* Once a request has been obtained, headers can be set on it, and data can be written to its body if required. Once
* you are ready to send the request, one of the {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} methods should be called.
*
* Nothing is actually sent until the request has been internally assigned an HTTP connection.
*
* The {@link io.vertx.rxjava3.core.http.HttpClient} instance will return an instance of this class immediately, even if there are no HTTP
* connections available in the pool. Any requests sent before a connection is assigned will be queued
* internally and actually sent when an HTTP connection becomes available from the pool.
*
* The headers of the request are queued for writing either when the {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} method is called, or, when the first
* part of the body is written, whichever occurs first.
*
* This class supports both chunked and non-chunked HTTP.
*
* It implements {@link io.vertx.rxjava3.core.streams.WriteStream} so it can be used with
* {@link io.vertx.rxjava3.core.streams.Pipe} to pipe data with flow control.
*
* An example of using this class is as follows:
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.HttpClientRequest original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.HttpClientRequest.class)
public class HttpClientRequest implements io.vertx.rxjava3.core.streams.WriteStream {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpClientRequest that = (HttpClientRequest) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpClientRequest((io.vertx.core.http.HttpClientRequest) obj),
HttpClientRequest::getDelegate
);
private final io.vertx.core.http.HttpClientRequest delegate;
public HttpClientRequest(io.vertx.core.http.HttpClientRequest delegate) {
this.delegate = delegate;
}
public HttpClientRequest(Object delegate) {
this.delegate = (io.vertx.core.http.HttpClientRequest)delegate;
}
public io.vertx.core.http.HttpClientRequest getDelegate() {
return delegate;
}
private WriteStreamObserver observer;
private WriteStreamSubscriber subscriber;
public synchronized WriteStreamObserver toObserver() {
if (observer == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::getDelegate;
observer = RxHelper.toObserver(getDelegate(), conv);
}
return observer;
}
public synchronized WriteStreamSubscriber toSubscriber() {
if (subscriber == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::getDelegate;
subscriber = RxHelper.toSubscriber(getDelegate(), conv);
}
return subscriber;
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable write(io.vertx.rxjava3.core.buffer.Buffer data) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(data);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(io.vertx.rxjava3.core.buffer.Buffer data) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(data.getDelegate(), handler);
});
}
/**
* This will return true
if there are more bytes in the write queue than the value set using {@link io.vertx.rxjava3.core.http.HttpClientRequest#setWriteQueueMaxSize}
* @return true
if write queue is full
*/
public boolean writeQueueFull() {
boolean ret = delegate.writeQueueFull();
return ret;
}
public io.vertx.rxjava3.core.http.HttpClientRequest exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.rxjava3.core.http.HttpClientRequest setWriteQueueMaxSize(int maxSize) {
delegate.setWriteQueueMaxSize(maxSize);
return this;
}
public io.vertx.rxjava3.core.http.HttpClientRequest drainHandler(io.vertx.core.Handler handler) {
delegate.drainHandler(handler);
return this;
}
/**
* Override the request authority, when using HTTP/1.x this overrides the request host
header, when using
* HTTP/2 this sets the authority
pseudo header. When the port is a negative value, the default
* scheme port will be used.
*
* The default request authority is the server host and port when connecting to the server.
* @param authority override the request authority
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest authority(io.vertx.rxjava3.core.net.HostAndPort authority) {
delegate.authority(authority.getDelegate());
return this;
}
/**
* Set the host value of the HTTP/1.1 host
header or HTTP/2 authority
pseudo header
*
The initial value is the same as the server socket address host.
*
Keep in mind that changing this value won't change the actual server socket address for this request.
* @param host the host part of the HTTP/1.1 host
header or HTTP/2 authority
pseudo header
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.core.http.HttpClientRequest setHost(java.lang.String host) {
delegate.setHost(host);
return this;
}
/**
* @return the host value of the HTTP/1.1 host
header or HTTP/2 authority
pseudo header
*/
@Deprecated()
public java.lang.String getHost() {
java.lang.String ret = delegate.getHost();
return ret;
}
/**
* Set the port value of the HTTP/1.1 host
header or HTTP/2 authority
pseudo header
*
*
Keep in mind that this won't change the actual server socket address for this request.
*
The initial value is the same than the server socket address port.
* @param port the port part of the HTTP/1.1 host
header or HTTP/2 authority
pseudo header
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.core.http.HttpClientRequest setPort(int port) {
delegate.setPort(port);
return this;
}
/**
* @return the port value of the HTTP/1.1 host
header or HTTP/2 authority
pseudo header
*/
@Deprecated()
public int getPort() {
int ret = delegate.getPort();
return ret;
}
/**
* Set the request to follow HTTP redirects up to {@link io.vertx.core.http.HttpClientOptions}.
* @param followRedirects true
to follow HTTP redirects
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest setFollowRedirects(boolean followRedirects) {
delegate.setFollowRedirects(followRedirects);
return this;
}
/**
* @return whether HTTP redirections should be followed
*/
public boolean isFollowRedirects() {
boolean ret = delegate.isFollowRedirects();
return ret;
}
/**
* Set the max number of HTTP redirects this request will follow. The default is 0
which means
* no redirects.
* @param maxRedirects the number of HTTP redirect to follow
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest setMaxRedirects(int maxRedirects) {
delegate.setMaxRedirects(maxRedirects);
return this;
}
/**
* @return the maximum number of HTTP redirections to follow
*/
public int getMaxRedirects() {
int ret = delegate.getMaxRedirects();
return ret;
}
/**
* @return the number of followed redirections for the current HTTP request
*/
public int numberOfRedirections() {
int ret = delegate.numberOfRedirections();
return ret;
}
/**
* If chunked is true then the request will be set into HTTP chunked mode
* @param chunked true if chunked encoding
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest setChunked(boolean chunked) {
delegate.setChunked(chunked);
return this;
}
/**
* @return Is the request chunked?
*/
public boolean isChunked() {
boolean ret = delegate.isChunked();
return ret;
}
/**
* The HTTP method for the request.
* @return
*/
public io.vertx.core.http.HttpMethod getMethod() {
io.vertx.core.http.HttpMethod ret = delegate.getMethod();
return ret;
}
/**
* Set the HTTP method for this request.
* @param method the HTTP method
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest setMethod(io.vertx.core.http.HttpMethod method) {
delegate.setMethod(method);
return this;
}
/**
* @return the absolute URI corresponding to the HTTP request
*/
public java.lang.String absoluteURI() {
java.lang.String ret = delegate.absoluteURI();
return ret;
}
/**
* @return The URI of the request.
*/
public java.lang.String getURI() {
java.lang.String ret = delegate.getURI();
return ret;
}
/**
* Set the request uri.
* @param uri the request uri
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest setURI(java.lang.String uri) {
delegate.setURI(uri);
return this;
}
/**
* @return The path part of the uri. For example /somepath/somemorepath/someresource.foo
*/
public java.lang.String path() {
java.lang.String ret = delegate.path();
return ret;
}
/**
* @return the query part of the uri. For example someparam=32&someotherparam=x
*/
public java.lang.String query() {
java.lang.String ret = delegate.query();
return ret;
}
/**
* @return The HTTP headers
*/
public io.vertx.rxjava3.core.MultiMap headers() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.headers());
cached_0 = ret;
return ret;
}
/**
* Put an HTTP header
* @param name The header name
* @param value The header value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest putHeader(java.lang.String name, java.lang.String value) {
delegate.putHeader(name, value);
return this;
}
/**
* Set the trace operation of this request.
* @param op the operation
* @return @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest traceOperation(java.lang.String op) {
io.vertx.rxjava3.core.http.HttpClientRequest ret = io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)delegate.traceOperation(op));
return ret;
}
/**
* @return the trace operation of this request
*/
public java.lang.String traceOperation() {
java.lang.String ret = delegate.traceOperation();
return ret;
}
/**
* @return the HTTP version for this request
*/
public io.vertx.core.http.HttpVersion version() {
io.vertx.core.http.HttpVersion ret = delegate.version();
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#write} but with an handler
called when the operation completes
* @param chunk
* @return
*/
public io.reactivex.rxjava3.core.Completable write(java.lang.String chunk) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(chunk);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#write} but with an handler
called when the operation completes
* @param chunk
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(java.lang.String chunk) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(chunk, handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#write} but with an handler
called when the operation completes
* @param chunk
* @param enc
* @return
*/
public io.reactivex.rxjava3.core.Completable write(java.lang.String chunk, java.lang.String enc) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(chunk, enc);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#write} but with an handler
called when the operation completes
* @param chunk
* @param enc
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(java.lang.String chunk, java.lang.String enc) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(chunk, enc, handler);
});
}
/**
* If you send an HTTP request with the header Expect
set to the value 100-continue
* and the server responds with an interim HTTP response with a status code of 100
and a Continue handler
* has been set using this method, then the handler
will be called.
*
* You can then continue to write data to the request body and later end it. This is normally used in conjunction with
* the {@link io.vertx.rxjava3.core.http.HttpClientRequest#sendHead} method to force the request header to be written before the request has ended.
* @param handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest continueHandler(io.vertx.core.Handler handler) {
delegate.continueHandler(handler);
return this;
}
/**
* If the server responds with an interim HTTP response with a status code of 103
and a Early Hints handler
* has been set using this method, then the handler
will be called.
* @param handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest earlyHintsHandler(io.vertx.core.Handler handler) {
delegate.earlyHintsHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)event)));
return this;
}
public io.vertx.rxjava3.core.http.HttpClientRequest redirectHandler(java.util.function.Function> handler) {
delegate.redirectHandler(new Function>() {
public io.vertx.core.Future apply(io.vertx.core.http.HttpClientResponse arg) {
io.reactivex.rxjava3.core.Single ret = handler.apply(io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)arg));
return io.vertx.rxjava3.SingleHelper.toFuture(ret, obj -> obj.getDelegate());
}
});
return this;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClientRequest#sendHead} but with an handler after headers have been sent. The handler will be called with
* the {@link io.vertx.core.http.HttpVersion} if it can be determined or null otherwise.
* @return
*/
public io.reactivex.rxjava3.core.Completable sendHead() {
io.reactivex.rxjava3.core.Completable ret = rxSendHead();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClientRequest#sendHead} but with an handler after headers have been sent. The handler will be called with
* the {@link io.vertx.core.http.HttpVersion} if it can be determined or null otherwise.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxSendHead() {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.sendHead(completionHandler);
});
}
/**
* Create an HTTP tunnel to the server.
*
*
Send HTTP request headers to the server, then configures the transport to exchange
* raw buffers when the server replies with an appropriate response:
*
*
* 200
for HTTP CONNECT
method
* 101
for HTTP/1.1 GET
with Upgrade
connection
header
*
*
* The handler
is called after response headers are received.
*
*
Use {@link io.vertx.rxjava3.core.http.HttpClientResponse#netSocket} to get a for interacting
* more conveniently with the server.
*
*
HTTP/1.1 pipe-lined requests are not supported.f
* @return
*/
public io.reactivex.rxjava3.core.Single connect() {
io.reactivex.rxjava3.core.Single ret = rxConnect();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create an HTTP tunnel to the server.
*
* Send HTTP request headers to the server, then configures the transport to exchange
* raw buffers when the server replies with an appropriate response:
*
*
* 200
for HTTP CONNECT
method
* 101
for HTTP/1.1 GET
with Upgrade
connection
header
*
*
* The handler
is called after response headers are received.
*
*
Use {@link io.vertx.rxjava3.core.http.HttpClientResponse#netSocket} to get a for interacting
* more conveniently with the server.
*
*
HTTP/1.1 pipe-lined requests are not supported.f
* @return
*/
public io.reactivex.rxjava3.core.Single rxConnect() {
return AsyncResultSingle.toSingle( handler -> {
delegate.connect(new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)event))));
});
}
/**
* Set a callback for the associated {@link io.vertx.rxjava3.core.http.HttpClientResponse}.
*
* This method does not modify the current request being sent.
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single response() {
io.reactivex.rxjava3.core.Single ret = rxResponse();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Set a callback for the associated {@link io.vertx.rxjava3.core.http.HttpClientResponse}.
*
* This method does not modify the current request being sent.
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Single rxResponse() {
return AsyncResultSingle.toSingle( handler -> {
delegate.response(new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)event))));
});
}
/**
* Send the request with an empty body.
* @return
*/
public io.reactivex.rxjava3.core.Single send() {
io.reactivex.rxjava3.core.Single ret = rxSend();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Send the request with an empty body.
* @return
*/
public io.reactivex.rxjava3.core.Single rxSend() {
return AsyncResultSingle.toSingle( handler -> {
delegate.send(new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)event))));
});
}
/**
* Send the request with a string body
.
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Single send(java.lang.String body) {
io.reactivex.rxjava3.core.Single ret = rxSend(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Send the request with a string body
.
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Single rxSend(java.lang.String body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.send(body, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)event))));
});
}
/**
* Send the request with a buffer body
.
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Single send(io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Single ret = rxSend(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Send the request with a buffer body
.
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Single rxSend(io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.send(body.getDelegate(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)event))));
});
}
/**
* Send the request with a stream body
.
*
* If the {@link io.vertx.rxjava3.core.http.HttpHeaders} is set then the request assumes this is the
* length of the {stream}, otherwise the request will set a chunked {@link io.vertx.rxjava3.core.http.HttpHeaders}.
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Single send(io.reactivex.rxjava3.core.Flowable body) {
io.reactivex.rxjava3.core.Single ret = rxSend(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Send the request with a stream body
.
*
* If the {@link io.vertx.rxjava3.core.http.HttpHeaders} is set then the request assumes this is the
* length of the {stream}, otherwise the request will set a chunked {@link io.vertx.rxjava3.core.http.HttpHeaders}.
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Single rxSend(io.reactivex.rxjava3.core.Flowable body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.send(io.vertx.rxjava3.impl.ReadStreamSubscriber.asReadStream(body, obj -> obj.getDelegate()).resume(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.http.HttpClientResponse.newInstance((io.vertx.core.http.HttpClientResponse)event))));
});
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @param chunk
* @return
*/
public io.reactivex.rxjava3.core.Completable end(java.lang.String chunk) {
io.reactivex.rxjava3.core.Completable ret = rxEnd(chunk);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @param chunk
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd(java.lang.String chunk) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(chunk, handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @param chunk
* @param enc
* @return
*/
public io.reactivex.rxjava3.core.Completable end(java.lang.String chunk, java.lang.String enc) {
io.reactivex.rxjava3.core.Completable ret = rxEnd(chunk, enc);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @param chunk
* @param enc
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd(java.lang.String chunk, java.lang.String enc) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(chunk, enc, handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @param chunk
* @return
*/
public io.reactivex.rxjava3.core.Completable end(io.vertx.rxjava3.core.buffer.Buffer chunk) {
io.reactivex.rxjava3.core.Completable ret = rxEnd(chunk);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @param chunk
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd(io.vertx.rxjava3.core.buffer.Buffer chunk) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(chunk.getDelegate(), handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Completable end() {
io.reactivex.rxjava3.core.Completable ret = rxEnd();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientRequest#end} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(handler);
});
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClientRequest#idleTimeout} but with a confusing name (hence the deprecation).
* @param timeout
* @return
*/
@Deprecated()
public io.vertx.rxjava3.core.http.HttpClientRequest setTimeout(long timeout) {
delegate.setTimeout(timeout);
return this;
}
/**
* Sets the amount of time after which, if the request does not return any data within the timeout period,
* the request/response is closed and the related futures are failed with a {@link java.util.concurrent.TimeoutException},
* e.g. Future
or Future
response body.
* @param timeout the amount of time in milliseconds.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest idleTimeout(long timeout) {
delegate.idleTimeout(timeout);
return this;
}
/**
* Set a push handler for this request.
*
* The handler is called when the client receives a push promise from the server. The handler can be called
* multiple times, for each push promise.
*
* The handler is called with a read-only {@link io.vertx.rxjava3.core.http.HttpClientRequest}, the following methods can be called:
*
*
* - {@link io.vertx.rxjava3.core.http.HttpClientRequest#getMethod}
* - {@link io.vertx.rxjava3.core.http.HttpClientRequest#getURI}
* - {@link io.vertx.rxjava3.core.http.HttpClientRequest#headers}
*
*
* In addition the handler should call the {@link io.vertx.rxjava3.core.http.HttpClientRequest#response} method to set an handler to
* process the response.
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest pushHandler(io.vertx.core.Handler handler) {
delegate.pushHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)event)));
return this;
}
/**
* Reset this stream with the error code 0
.
* @return
*/
public boolean reset() {
boolean ret = delegate.reset();
return ret;
}
/**
* Reset this request:
*
*
* - for HTTP/2, this performs send an HTTP/2 reset frame with the specified error
code
* - for HTTP/1.x, this closes the connection when the current request is inflight
*
*
* When the request has not yet been sent, the request will be aborted and false is returned as indicator.
*
* @param code the error code
* @return true
when reset has been performed
*/
public boolean reset(long code) {
boolean ret = delegate.reset(code);
return ret;
}
/**
* Reset this request:
*
*
* - for HTTP/2, send an HTTP/2 reset frame with the specified error
code
* - for HTTP/1.x, close the connection when the current request is inflight
*
*
* When the request has not yet been sent, the request will be aborted and false
is returned as indicator.
*
* @param code the error code
* @param cause an optional cause that can be attached to the error code
* @return true when reset has been performed
*/
public boolean reset(long code, java.lang.Throwable cause) {
boolean ret = delegate.reset(code, cause);
return ret;
}
/**
* @return the {@link io.vertx.rxjava3.core.http.HttpConnection} associated with this request
*/
public io.vertx.rxjava3.core.http.HttpConnection connection() {
if (cached_1 != null) {
return cached_1;
}
io.vertx.rxjava3.core.http.HttpConnection ret = io.vertx.rxjava3.core.http.HttpConnection.newInstance((io.vertx.core.http.HttpConnection)delegate.connection());
cached_1 = ret;
return ret;
}
/**
* Write an HTTP/2 frame to the request, allowing to extend the HTTP/2 protocol.
*
* The frame is sent immediatly and is not subject to flow control.
*
* This method must be called after the request headers have been sent and only for the protocol HTTP/2.
* The {@link io.vertx.rxjava3.core.http.HttpClientRequest#sendHead} should be used for this purpose.
* @param type the 8-bit frame type
* @param flags the 8-bit frame flags
* @param payload the frame payload
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest writeCustomFrame(int type, int flags, io.vertx.rxjava3.core.buffer.Buffer payload) {
delegate.writeCustomFrame(type, flags, payload.getDelegate());
return this;
}
/**
* @return the id of the stream of this response, when it is not yet determined, i.e the request has not been yet sent or it is not supported HTTP/1.x
*/
public int streamId() {
int ret = delegate.streamId();
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClientRequest#writeCustomFrame} but with an {@link io.vertx.rxjava3.core.http.HttpFrame}.
* @param frame the frame to write
* @return
*/
public io.vertx.rxjava3.core.http.HttpClientRequest writeCustomFrame(io.vertx.rxjava3.core.http.HttpFrame frame) {
delegate.writeCustomFrame(frame.getDelegate());
return this;
}
/**
* Sets the priority of the associated stream.
*
* This is not implemented for HTTP/1.x.
* @param streamPriority the priority of this request's stream
* @return
*/
public io.vertx.rxjava3.core.http.HttpClientRequest setStreamPriority(io.vertx.core.http.StreamPriority streamPriority) {
delegate.setStreamPriority(streamPriority);
return this;
}
/**
* @return the priority of the associated HTTP/2 stream for HTTP/2 otherwise null
*/
public io.vertx.core.http.StreamPriority getStreamPriority() {
io.vertx.core.http.StreamPriority ret = delegate.getStreamPriority();
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClientRequest#putHeader} but using CharSequence
* @param name
* @param value
* @return
*/
public io.vertx.rxjava3.core.http.HttpClientRequest putHeader(java.lang.CharSequence name, java.lang.CharSequence value) {
delegate.putHeader(name, value);
return this;
}
/**
* Put an HTTP header with multiple values
* @param name The header name
* @param values The header values
* @return @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientRequest putHeader(java.lang.String name, java.lang.Iterable values) {
delegate.putHeader(name, values);
return this;
}
/**
* Like {@link io.vertx.rxjava3.core.http.HttpClientRequest#putHeader} but using CharSequence
* @param name
* @param values
* @return
*/
public io.vertx.rxjava3.core.http.HttpClientRequest putHeader(java.lang.CharSequence name, java.lang.Iterable values) {
delegate.putHeader(name, values);
return this;
}
private io.vertx.rxjava3.core.MultiMap cached_0;
private io.vertx.rxjava3.core.http.HttpConnection cached_1;
public static HttpClientRequest newInstance(io.vertx.core.http.HttpClientRequest arg) {
return arg != null ? new HttpClientRequest(arg) : null;
}
}