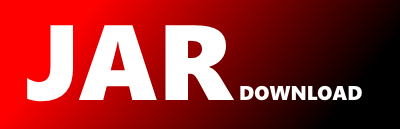
io.vertx.rxjava3.core.http.HttpClientResponse Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.http;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents a client-side HTTP response.
*
* Vert.x provides you with one of these via the handler that was provided when creating the {@link io.vertx.rxjava3.core.http.HttpClientRequest}
* or that was set on the {@link io.vertx.rxjava3.core.http.HttpClientRequest} instance.
*
* It implements {@link io.vertx.rxjava3.core.streams.ReadStream} so it can be used with
* {@link io.vertx.rxjava3.core.streams.Pipe} to pipe data with flow control.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.http.HttpClientResponse original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.http.HttpClientResponse.class)
public class HttpClientResponse implements io.vertx.rxjava3.core.streams.ReadStream, io.vertx.rxjava3.core.http.HttpResponseHead {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpClientResponse that = (HttpClientResponse) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpClientResponse((io.vertx.core.http.HttpClientResponse) obj),
HttpClientResponse::getDelegate
);
private final io.vertx.core.http.HttpClientResponse delegate;
public HttpClientResponse(io.vertx.core.http.HttpClientResponse delegate) {
this.delegate = delegate;
}
public HttpClientResponse(Object delegate) {
this.delegate = (io.vertx.core.http.HttpClientResponse)delegate;
}
public io.vertx.core.http.HttpClientResponse getDelegate() {
return delegate;
}
private io.reactivex.rxjava3.core.Observable observable;
private io.reactivex.rxjava3.core.Flowable flowable;
public synchronized io.reactivex.rxjava3.core.Observable toObservable() {
if (observable == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::newInstance;
observable = ObservableHelper.toObservable(delegate, conv);
}
return observable;
}
public synchronized io.reactivex.rxjava3.core.Flowable toFlowable() {
if (flowable == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::newInstance;
flowable = FlowableHelper.toFlowable(delegate, conv);
}
return flowable;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
/**
* @return the version of the response
*/
public io.vertx.core.http.HttpVersion version() {
io.vertx.core.http.HttpVersion ret = delegate.version();
return ret;
}
/**
* @return the status code of the response
*/
public int statusCode() {
int ret = delegate.statusCode();
return ret;
}
/**
* @return the status message of the response
*/
public java.lang.String statusMessage() {
java.lang.String ret = delegate.statusMessage();
return ret;
}
/**
* @return the headers
*/
public io.vertx.rxjava3.core.MultiMap headers() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.headers());
cached_0 = ret;
return ret;
}
/**
* Return the first header value with the specified name
* @param headerName the header name
* @return the header value
*/
public java.lang.String getHeader(java.lang.String headerName) {
java.lang.String ret = delegate.getHeader(headerName);
return ret;
}
/**
* @return the Set-Cookie headers (including trailers)
*/
public java.util.List cookies() {
if (cached_1 != null) {
return cached_1;
}
java.util.List ret = delegate.cookies();
cached_1 = ret;
return ret;
}
/**
* Pause this stream and return a to transfer the elements of this stream to a destination .
*
* The stream will be resumed when the pipe will be wired to a WriteStream
.
* @return a pipe
*/
public io.vertx.rxjava3.core.streams.Pipe pipe() {
io.vertx.rxjava3.core.streams.Pipe ret = io.vertx.rxjava3.core.streams.Pipe.newInstance((io.vertx.core.streams.Pipe)delegate.pipe(), TYPE_ARG_0);
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
*
* Once this stream has ended or failed, the write stream will be ended and the handler
will be
* called with the result.
* @param dst the destination write stream
* @return
*/
public io.reactivex.rxjava3.core.Completable pipeTo(io.vertx.rxjava3.core.streams.WriteStream dst) {
io.reactivex.rxjava3.core.Completable ret = rxPipeTo(dst);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
*
* Once this stream has ended or failed, the write stream will be ended and the handler
will be
* called with the result.
* @param dst the destination write stream
* @return
*/
public io.reactivex.rxjava3.core.Completable rxPipeTo(io.vertx.rxjava3.core.streams.WriteStream dst) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.pipeTo(dst.getDelegate(), handler);
});
}
public io.vertx.rxjava3.core.http.HttpClientResponse fetch(long amount) {
delegate.fetch(amount);
return this;
}
public io.vertx.rxjava3.core.http.HttpClientResponse resume() {
delegate.resume();
return this;
}
public io.vertx.rxjava3.core.http.HttpClientResponse exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.rxjava3.core.http.HttpClientResponse handler(io.vertx.core.Handler handler) {
delegate.handler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)event)));
return this;
}
public io.vertx.rxjava3.core.http.HttpClientResponse pause() {
delegate.pause();
return this;
}
public io.vertx.rxjava3.core.http.HttpClientResponse endHandler(io.vertx.core.Handler endHandler) {
delegate.endHandler(endHandler);
return this;
}
/**
* @return a NetSocket
facade to interact with the HTTP client response.
*/
public io.vertx.rxjava3.core.net.NetSocket netSocket() {
if (cached_2 != null) {
return cached_2;
}
io.vertx.rxjava3.core.net.NetSocket ret = io.vertx.rxjava3.core.net.NetSocket.newInstance((io.vertx.core.net.NetSocket)delegate.netSocket());
cached_2 = ret;
return ret;
}
/**
* Return the first trailer value with the specified name
* @param trailerName the trailer name
* @return the trailer value
*/
public java.lang.String getTrailer(java.lang.String trailerName) {
java.lang.String ret = delegate.getTrailer(trailerName);
return ret;
}
/**
* @return the trailers
*/
public io.vertx.rxjava3.core.MultiMap trailers() {
if (cached_3 != null) {
return cached_3;
}
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.trailers());
cached_3 = ret;
return ret;
}
/**
* Convenience method for receiving the entire request body in one piece.
*
* This saves you having to manually set a dataHandler and an endHandler and append the chunks of the body until
* the whole body received. Don't use this if your request body is large - you could potentially run out of RAM.
* @param bodyHandler This handler will be called after all the body has been received
* @return
*/
public io.vertx.rxjava3.core.http.HttpClientResponse bodyHandler(io.vertx.core.Handler bodyHandler) {
delegate.bodyHandler(new io.vertx.lang.rx.DelegatingHandler<>(bodyHandler, event -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)event)));
return this;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientResponse#body} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Single body() {
io.reactivex.rxjava3.core.Single ret = rxBody();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientResponse#body} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Single rxBody() {
return AsyncResultSingle.toSingle( handler -> {
delegate.body(new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)event))));
});
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientResponse#end} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Completable end() {
io.reactivex.rxjava3.core.Completable ret = rxEnd();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.http.HttpClientResponse#end} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(handler);
});
}
/**
* Set an custom frame handler. The handler will get notified when the http stream receives an custom HTTP/2
* frame. HTTP/2 permits extension of the protocol.
* @param handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.http.HttpClientResponse customFrameHandler(io.vertx.core.Handler handler) {
delegate.customFrameHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.core.http.HttpFrame.newInstance((io.vertx.core.http.HttpFrame)event)));
return this;
}
/**
* @return the corresponding request
*/
public io.vertx.rxjava3.core.http.HttpClientRequest request() {
if (cached_4 != null) {
return cached_4;
}
io.vertx.rxjava3.core.http.HttpClientRequest ret = io.vertx.rxjava3.core.http.HttpClientRequest.newInstance((io.vertx.core.http.HttpClientRequest)delegate.request());
cached_4 = ret;
return ret;
}
/**
* Set an handler for stream priority changes.
*
* This is not implemented for HTTP/1.x.
* @param handler the handler to be called when the stream priority changes
* @return
*/
public io.vertx.rxjava3.core.http.HttpClientResponse streamPriorityHandler(io.vertx.core.Handler handler) {
delegate.streamPriorityHandler(handler);
return this;
}
/**
* Return the first header value with the specified name
* @param headerName the header name
* @return the header value
*/
public java.lang.String getHeader(java.lang.CharSequence headerName) {
java.lang.String ret = delegate.getHeader(headerName);
return ret;
}
private io.vertx.rxjava3.core.MultiMap cached_0;
private java.util.List cached_1;
private io.vertx.rxjava3.core.net.NetSocket cached_2;
private io.vertx.rxjava3.core.MultiMap cached_3;
private io.vertx.rxjava3.core.http.HttpClientRequest cached_4;
public static HttpClientResponse newInstance(io.vertx.core.http.HttpClientResponse arg) {
return arg != null ? new HttpClientResponse(arg) : null;
}
}