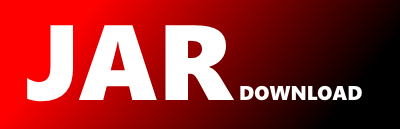
io.vertx.rxjava3.core.net.NetSocket Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.net;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents a socket-like interface to a TCP connection on either the
* client or the server side.
*
* Instances of this class are created on the client side by an {@link io.vertx.rxjava3.core.net.NetClient}
* when a connection to a server is made, or on the server side by a {@link io.vertx.rxjava3.core.net.NetServer}
* when a server accepts a connection.
*
* It implements both and so it can be used with
* {@link io.vertx.rxjava3.core.streams.Pipe} to pipe data with flow control.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.net.NetSocket original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.net.NetSocket.class)
public class NetSocket implements io.vertx.rxjava3.core.streams.ReadStream, io.vertx.rxjava3.core.streams.WriteStream {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
NetSocket that = (NetSocket) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new NetSocket((io.vertx.core.net.NetSocket) obj),
NetSocket::getDelegate
);
private final io.vertx.core.net.NetSocket delegate;
public NetSocket(io.vertx.core.net.NetSocket delegate) {
this.delegate = delegate;
}
public NetSocket(Object delegate) {
this.delegate = (io.vertx.core.net.NetSocket)delegate;
}
public io.vertx.core.net.NetSocket getDelegate() {
return delegate;
}
private io.reactivex.rxjava3.core.Observable observable;
private io.reactivex.rxjava3.core.Flowable flowable;
public synchronized io.reactivex.rxjava3.core.Observable toObservable() {
if (observable == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::newInstance;
observable = ObservableHelper.toObservable(delegate, conv);
}
return observable;
}
public synchronized io.reactivex.rxjava3.core.Flowable toFlowable() {
if (flowable == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::newInstance;
flowable = FlowableHelper.toFlowable(delegate, conv);
}
return flowable;
}
private WriteStreamObserver observer;
private WriteStreamSubscriber subscriber;
public synchronized WriteStreamObserver toObserver() {
if (observer == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::getDelegate;
observer = RxHelper.toObserver(getDelegate(), conv);
}
return observer;
}
public synchronized WriteStreamSubscriber toSubscriber() {
if (subscriber == null) {
Function conv = io.vertx.rxjava3.core.buffer.Buffer::getDelegate;
subscriber = RxHelper.toSubscriber(getDelegate(), conv);
}
return subscriber;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
/**
* Pause this stream and return a to transfer the elements of this stream to a destination .
*
* The stream will be resumed when the pipe will be wired to a WriteStream
.
* @return a pipe
*/
public io.vertx.rxjava3.core.streams.Pipe pipe() {
io.vertx.rxjava3.core.streams.Pipe ret = io.vertx.rxjava3.core.streams.Pipe.newInstance((io.vertx.core.streams.Pipe)delegate.pipe(), TYPE_ARG_0);
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
*
* Once this stream has ended or failed, the write stream will be ended and the handler
will be
* called with the result.
* @param dst the destination write stream
* @return
*/
public io.reactivex.rxjava3.core.Completable pipeTo(io.vertx.rxjava3.core.streams.WriteStream dst) {
io.reactivex.rxjava3.core.Completable ret = rxPipeTo(dst);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
*
* Once this stream has ended or failed, the write stream will be ended and the handler
will be
* called with the result.
* @param dst the destination write stream
* @return
*/
public io.reactivex.rxjava3.core.Completable rxPipeTo(io.vertx.rxjava3.core.streams.WriteStream dst) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.pipeTo(dst.getDelegate(), handler);
});
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable end(io.vertx.rxjava3.core.buffer.Buffer data) {
io.reactivex.rxjava3.core.Completable ret = rxEnd(data);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd(io.vertx.rxjava3.core.buffer.Buffer data) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(data.getDelegate(), handler);
});
}
/**
* This will return true
if there are more bytes in the write queue than the value set using {@link io.vertx.rxjava3.core.net.NetSocket#setWriteQueueMaxSize}
* @return true
if write queue is full
*/
public boolean writeQueueFull() {
boolean ret = delegate.writeQueueFull();
return ret;
}
public io.vertx.rxjava3.core.net.NetSocket exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.rxjava3.core.net.NetSocket handler(io.vertx.core.Handler handler) {
delegate.handler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)event)));
return this;
}
public io.vertx.rxjava3.core.net.NetSocket pause() {
delegate.pause();
return this;
}
public io.vertx.rxjava3.core.net.NetSocket resume() {
delegate.resume();
return this;
}
public io.vertx.rxjava3.core.net.NetSocket fetch(long amount) {
delegate.fetch(amount);
return this;
}
/**
*
*
* This handler might be called after the close handler when the socket is paused and there are still
* buffers to deliver.
* @param endHandler
* @return
*/
public io.vertx.rxjava3.core.net.NetSocket endHandler(io.vertx.core.Handler endHandler) {
delegate.endHandler(endHandler);
return this;
}
public io.vertx.rxjava3.core.net.NetSocket setWriteQueueMaxSize(int maxSize) {
delegate.setWriteQueueMaxSize(maxSize);
return this;
}
public io.vertx.rxjava3.core.net.NetSocket drainHandler(io.vertx.core.Handler handler) {
delegate.drainHandler(handler);
return this;
}
/**
* When a NetSocket
is created, it may register an event handler with the event bus, the ID of that
* handler is given by writeHandlerID
.
*
* By default, no handler is registered, the feature must be enabled via {@link io.vertx.core.net.NetClientOptions} or {@link io.vertx.core.net.NetServerOptions}.
*
* Given this ID, a different event loop can send a buffer to that event handler using the event bus and
* that buffer will be received by this instance in its own event loop and written to the underlying connection. This
* allows you to write data to other connections which are owned by different event loops.
* @return the write handler ID
*/
public java.lang.String writeHandlerID() {
java.lang.String ret = delegate.writeHandlerID();
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#write} but with an handler
called when the operation completes
* @param str
* @return
*/
public io.reactivex.rxjava3.core.Completable write(java.lang.String str) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(str);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#write} but with an handler
called when the operation completes
* @param str
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(java.lang.String str) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(str, handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#write} but with an handler
called when the operation completes
* @param str
* @param enc
* @return
*/
public io.reactivex.rxjava3.core.Completable write(java.lang.String str, java.lang.String enc) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(str, enc);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#write} but with an handler
called when the operation completes
* @param str
* @param enc
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(java.lang.String str, java.lang.String enc) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(str, enc, handler);
});
}
/**
* Like {@link io.vertx.rxjava3.core.streams.WriteStream#write} but with an handler
called when the message has been written
* or failed to be written.
* @param message
* @return
*/
public io.reactivex.rxjava3.core.Completable write(io.vertx.rxjava3.core.buffer.Buffer message) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(message);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.streams.WriteStream#write} but with an handler
called when the message has been written
* or failed to be written.
* @param message
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(io.vertx.rxjava3.core.buffer.Buffer message) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(message.getDelegate(), handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#sendFile} but also takes a handler that will be called when the send has completed or
* a failure has occurred
* @param filename file name of the file to send
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable sendFile(java.lang.String filename) {
io.reactivex.rxjava3.core.Completable ret = rxSendFile(filename);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#sendFile} but also takes a handler that will be called when the send has completed or
* a failure has occurred
* @param filename file name of the file to send
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxSendFile(java.lang.String filename) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.sendFile(filename, resultHandler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#sendFile} but also takes a handler that will be called when the send has completed or
* a failure has occurred
* @param filename file name of the file to send
* @param offset offset
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable sendFile(java.lang.String filename, long offset) {
io.reactivex.rxjava3.core.Completable ret = rxSendFile(filename, offset);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#sendFile} but also takes a handler that will be called when the send has completed or
* a failure has occurred
* @param filename file name of the file to send
* @param offset offset
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxSendFile(java.lang.String filename, long offset) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.sendFile(filename, offset, resultHandler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#sendFile} but also takes a handler that will be called when the send has completed or
* a failure has occurred
* @param filename file name of the file to send
* @param offset offset
* @param length length
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable sendFile(java.lang.String filename, long offset, long length) {
io.reactivex.rxjava3.core.Completable ret = rxSendFile(filename, offset, length);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.net.NetSocket#sendFile} but also takes a handler that will be called when the send has completed or
* a failure has occurred
* @param filename file name of the file to send
* @param offset offset
* @param length length
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxSendFile(java.lang.String filename, long offset, long length) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.sendFile(filename, offset, length, resultHandler);
});
}
/**
* @return the remote address for this connection, possibly null
(e.g a server bound on a domain socket). If useProxyProtocol
is set to true
, the address returned will be of the actual connecting client.
*/
public io.vertx.rxjava3.core.net.SocketAddress remoteAddress() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.rxjava3.core.net.SocketAddress ret = io.vertx.rxjava3.core.net.SocketAddress.newInstance((io.vertx.core.net.SocketAddress)delegate.remoteAddress());
cached_0 = ret;
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.net.NetSocket#remoteAddress} but returns the proxy remote address when real
is true
* @param real
* @return
*/
public io.vertx.rxjava3.core.net.SocketAddress remoteAddress(boolean real) {
io.vertx.rxjava3.core.net.SocketAddress ret = io.vertx.rxjava3.core.net.SocketAddress.newInstance((io.vertx.core.net.SocketAddress)delegate.remoteAddress(real));
return ret;
}
/**
* @return the local address for this connection, possibly null
(e.g a server bound on a domain socket) If useProxyProtocol
is set to true
, the address returned will be of the proxy.
*/
public io.vertx.rxjava3.core.net.SocketAddress localAddress() {
if (cached_1 != null) {
return cached_1;
}
io.vertx.rxjava3.core.net.SocketAddress ret = io.vertx.rxjava3.core.net.SocketAddress.newInstance((io.vertx.core.net.SocketAddress)delegate.localAddress());
cached_1 = ret;
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.net.NetSocket#localAddress} ()} but returns the server local address when real
is true
* @param real
* @return
*/
public io.vertx.rxjava3.core.net.SocketAddress localAddress(boolean real) {
io.vertx.rxjava3.core.net.SocketAddress ret = io.vertx.rxjava3.core.net.SocketAddress.newInstance((io.vertx.core.net.SocketAddress)delegate.localAddress(real));
return ret;
}
/**
* Calls {@link io.vertx.rxjava3.core.net.NetSocket#end}.
* @return
*/
public io.reactivex.rxjava3.core.Completable end() {
io.reactivex.rxjava3.core.Completable ret = rxEnd();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Calls {@link io.vertx.rxjava3.core.net.NetSocket#end}.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(handler);
});
}
/**
* Close the NetSocket and notify the handler
when the operation completes.
* @return
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Close the NetSocket and notify the handler
when the operation completes.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.close(handler);
});
}
/**
* Set a handler that will be called when the NetSocket is closed
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.core.net.NetSocket closeHandler(io.vertx.core.Handler handler) {
delegate.closeHandler(handler);
return this;
}
/**
* Upgrade channel to use SSL/TLS. Be aware that for this to work SSL must be configured.
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable upgradeToSsl() {
io.reactivex.rxjava3.core.Completable ret = rxUpgradeToSsl();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Upgrade channel to use SSL/TLS. Be aware that for this to work SSL must be configured.
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxUpgradeToSsl() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.upgradeToSsl(handler);
});
}
/**
* Upgrade channel to use SSL/TLS. Be aware that for this to work SSL must be configured.
* @param serverName the server name
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable upgradeToSsl(java.lang.String serverName) {
io.reactivex.rxjava3.core.Completable ret = rxUpgradeToSsl(serverName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Upgrade channel to use SSL/TLS. Be aware that for this to work SSL must be configured.
* @param serverName the server name
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxUpgradeToSsl(java.lang.String serverName) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.upgradeToSsl(serverName, handler);
});
}
/**
* @return true if this {@link io.vertx.rxjava3.core.net.NetSocket} is encrypted via SSL/TLS.
*/
public boolean isSsl() {
boolean ret = delegate.isSsl();
return ret;
}
/**
* Returns the SNI server name presented during the SSL handshake by the client.
* @return the indicated server name
*/
public java.lang.String indicatedServerName() {
java.lang.String ret = delegate.indicatedServerName();
return ret;
}
/**
* @return the application-level protocol negotiated during the TLS handshake
*/
public java.lang.String applicationLayerProtocol() {
java.lang.String ret = delegate.applicationLayerProtocol();
return ret;
}
/**
* @return SSLSession associated with the underlying socket. Returns null if connection is not SSL.
*/
public javax.net.ssl.SSLSession sslSession() {
javax.net.ssl.SSLSession ret = delegate.sslSession();
return ret;
}
private io.vertx.rxjava3.core.net.SocketAddress cached_0;
private io.vertx.rxjava3.core.net.SocketAddress cached_1;
public static NetSocket newInstance(io.vertx.core.net.NetSocket arg) {
return arg != null ? new NetSocket(arg) : null;
}
}