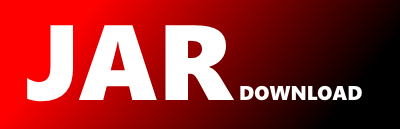
io.vertx.rxjava3.core.shareddata.AsyncMap Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.shareddata;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* An asynchronous map.
*
* {@link io.vertx.rxjava3.core.shareddata.AsyncMap} does not allow null
to be used as a key or value.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.shareddata.AsyncMap original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.shareddata.AsyncMap.class)
public class AsyncMap {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AsyncMap that = (AsyncMap) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new AsyncMap((io.vertx.core.shareddata.AsyncMap) obj),
AsyncMap::getDelegate
);
private final io.vertx.core.shareddata.AsyncMap delegate;
public final TypeArg __typeArg_0;
public final TypeArg __typeArg_1;
public AsyncMap(io.vertx.core.shareddata.AsyncMap delegate) {
this.delegate = delegate;
this.__typeArg_0 = TypeArg.unknown(); this.__typeArg_1 = TypeArg.unknown(); }
public AsyncMap(Object delegate, TypeArg typeArg_0, TypeArg typeArg_1) {
this.delegate = (io.vertx.core.shareddata.AsyncMap)delegate;
this.__typeArg_0 = typeArg_0;
this.__typeArg_1 = typeArg_1;
}
public io.vertx.core.shareddata.AsyncMap getDelegate() {
return delegate;
}
/**
* Get a value from the map, asynchronously.
* @param k the key
* @return
*/
public io.reactivex.rxjava3.core.Maybe get(K k) {
io.reactivex.rxjava3.core.Maybe ret = rxGet(k);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Get a value from the map, asynchronously.
* @param k the key
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxGet(K k) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.get(__typeArg_0.unwrap(k), new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> (V)__typeArg_1.wrap(event))));
});
}
/**
* Put a value in the map, asynchronously.
* @param k the key
* @param v the value
* @return
*/
public io.reactivex.rxjava3.core.Completable put(K k, V v) {
io.reactivex.rxjava3.core.Completable ret = rxPut(k, v);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Put a value in the map, asynchronously.
* @param k the key
* @param v the value
* @return
*/
public io.reactivex.rxjava3.core.Completable rxPut(K k, V v) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.put(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), completionHandler);
});
}
/**
* Like {@link io.vertx.rxjava3.core.shareddata.AsyncMap#put} but specifying a time to live for the entry. Entry will expire and get evicted after the
* ttl.
* @param k the key
* @param v the value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Completable put(K k, V v, long ttl) {
io.reactivex.rxjava3.core.Completable ret = rxPut(k, v, ttl);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.shareddata.AsyncMap#put} but specifying a time to live for the entry. Entry will expire and get evicted after the
* ttl.
* @param k the key
* @param v the value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Completable rxPut(K k, V v, long ttl) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.put(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), ttl, completionHandler);
});
}
/**
* Put the entry only if there is no entry with the key already present. If key already present then the existing
* value will be returned to the handler, otherwise null.
* @param k the key
* @param v the value
* @return
*/
public io.reactivex.rxjava3.core.Maybe putIfAbsent(K k, V v) {
io.reactivex.rxjava3.core.Maybe ret = rxPutIfAbsent(k, v);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Put the entry only if there is no entry with the key already present. If key already present then the existing
* value will be returned to the handler, otherwise null.
* @param k the key
* @param v the value
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxPutIfAbsent(K k, V v) {
return AsyncResultMaybe.toMaybe( completionHandler -> {
delegate.putIfAbsent(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> (V)__typeArg_1.wrap(event))));
});
}
/**
* Link {@link io.vertx.rxjava3.core.shareddata.AsyncMap#putIfAbsent} but specifying a time to live for the entry. Entry will expire and get evicted
* after the ttl.
* @param k the key
* @param v the value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Maybe putIfAbsent(K k, V v, long ttl) {
io.reactivex.rxjava3.core.Maybe ret = rxPutIfAbsent(k, v, ttl);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Link {@link io.vertx.rxjava3.core.shareddata.AsyncMap#putIfAbsent} but specifying a time to live for the entry. Entry will expire and get evicted
* after the ttl.
* @param k the key
* @param v the value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxPutIfAbsent(K k, V v, long ttl) {
return AsyncResultMaybe.toMaybe( completionHandler -> {
delegate.putIfAbsent(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), ttl, new io.vertx.lang.rx.DelegatingHandler<>(completionHandler, ar -> ar.map(event -> (V)__typeArg_1.wrap(event))));
});
}
/**
* Remove a value from the map, asynchronously.
* @param k the key
* @return
*/
public io.reactivex.rxjava3.core.Maybe remove(K k) {
io.reactivex.rxjava3.core.Maybe ret = rxRemove(k);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Remove a value from the map, asynchronously.
* @param k the key
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxRemove(K k) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.remove(__typeArg_0.unwrap(k), new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> (V)__typeArg_1.wrap(event))));
});
}
/**
* Remove a value from the map, only if entry already exists with same value.
* @param k the key
* @param v the value
* @return
*/
public io.reactivex.rxjava3.core.Single removeIfPresent(K k, V v) {
io.reactivex.rxjava3.core.Single ret = rxRemoveIfPresent(k, v);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Remove a value from the map, only if entry already exists with same value.
* @param k the key
* @param v the value
* @return
*/
public io.reactivex.rxjava3.core.Single rxRemoveIfPresent(K k, V v) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.removeIfPresent(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), resultHandler);
});
}
/**
* Replace the entry only if it is currently mapped to some value
* @param k the key
* @param v the new value
* @return
*/
public io.reactivex.rxjava3.core.Maybe replace(K k, V v) {
io.reactivex.rxjava3.core.Maybe ret = rxReplace(k, v);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Replace the entry only if it is currently mapped to some value
* @param k the key
* @param v the new value
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxReplace(K k, V v) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.replace(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> (V)__typeArg_1.wrap(event))));
});
}
/**
* Replace the entry only if it is currently mapped to some value
* @param k the key
* @param v the new value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Maybe replace(K k, V v, long ttl) {
io.reactivex.rxjava3.core.Maybe ret = rxReplace(k, v, ttl);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Replace the entry only if it is currently mapped to some value
* @param k the key
* @param v the new value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxReplace(K k, V v, long ttl) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.replace(__typeArg_0.unwrap(k), __typeArg_1.unwrap(v), ttl, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> (V)__typeArg_1.wrap(event))));
});
}
/**
* Replace the entry only if it is currently mapped to a specific value
* @param k the key
* @param oldValue the existing value
* @param newValue the new value
* @return
*/
public io.reactivex.rxjava3.core.Single replaceIfPresent(K k, V oldValue, V newValue) {
io.reactivex.rxjava3.core.Single ret = rxReplaceIfPresent(k, oldValue, newValue);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Replace the entry only if it is currently mapped to a specific value
* @param k the key
* @param oldValue the existing value
* @param newValue the new value
* @return
*/
public io.reactivex.rxjava3.core.Single rxReplaceIfPresent(K k, V oldValue, V newValue) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.replaceIfPresent(__typeArg_0.unwrap(k), __typeArg_1.unwrap(oldValue), __typeArg_1.unwrap(newValue), resultHandler);
});
}
/**
* Replace the entry only if it is currently mapped to a specific value
* @param k the key
* @param oldValue the existing value
* @param newValue the new value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Single replaceIfPresent(K k, V oldValue, V newValue, long ttl) {
io.reactivex.rxjava3.core.Single ret = rxReplaceIfPresent(k, oldValue, newValue, ttl);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Replace the entry only if it is currently mapped to a specific value
* @param k the key
* @param oldValue the existing value
* @param newValue the new value
* @param ttl The time to live (in ms) for the entry
* @return
*/
public io.reactivex.rxjava3.core.Single rxReplaceIfPresent(K k, V oldValue, V newValue, long ttl) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.replaceIfPresent(__typeArg_0.unwrap(k), __typeArg_1.unwrap(oldValue), __typeArg_1.unwrap(newValue), ttl, resultHandler);
});
}
/**
* Clear all entries in the map
* @return
*/
public io.reactivex.rxjava3.core.Completable clear() {
io.reactivex.rxjava3.core.Completable ret = rxClear();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Clear all entries in the map
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClear() {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.clear(resultHandler);
});
}
/**
* Provide the number of entries in the map
* @return
*/
public io.reactivex.rxjava3.core.Single size() {
io.reactivex.rxjava3.core.Single ret = rxSize();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Provide the number of entries in the map
* @return
*/
public io.reactivex.rxjava3.core.Single rxSize() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.size(resultHandler);
});
}
/**
* Get the keys of the map, asynchronously.
*
* Use this method with care as the map may contain a large number of keys,
* which may not fit entirely in memory of a single node.
* In this case, the invocation will result in an {@link java.lang.OutOfMemoryError}.
* @return
*/
public io.reactivex.rxjava3.core.Single> keys() {
io.reactivex.rxjava3.core.Single> ret = rxKeys();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the keys of the map, asynchronously.
*
* Use this method with care as the map may contain a large number of keys,
* which may not fit entirely in memory of a single node.
* In this case, the invocation will result in an {@link java.lang.OutOfMemoryError}.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxKeys() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.keys(new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> event.stream().map(elt -> (K)__typeArg_0.wrap(elt)).collect(Collectors.toSet()))));
});
}
/**
* Get the values of the map, asynchronously.
*
* Use this method with care as the map may contain a large number of values,
* which may not fit entirely in memory of a single node.
* In this case, the invocation will result in an {@link java.lang.OutOfMemoryError}.
* @return
*/
public io.reactivex.rxjava3.core.Single> values() {
io.reactivex.rxjava3.core.Single> ret = rxValues();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the values of the map, asynchronously.
*
* Use this method with care as the map may contain a large number of values,
* which may not fit entirely in memory of a single node.
* In this case, the invocation will result in an {@link java.lang.OutOfMemoryError}.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxValues() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.values(new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> event.stream().map(elt -> (V)__typeArg_1.wrap(elt)).collect(Collectors.toList()))));
});
}
public static AsyncMap newInstance(io.vertx.core.shareddata.AsyncMap arg) {
return arg != null ? new AsyncMap(arg) : null;
}
public static AsyncMap newInstance(io.vertx.core.shareddata.AsyncMap arg, TypeArg __typeArg_K, TypeArg __typeArg_V) {
return arg != null ? new AsyncMap(arg, __typeArg_K, __typeArg_V) : null;
}
}