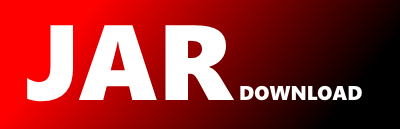
io.vertx.rxjava3.core.shareddata.LocalMap Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.shareddata;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Local maps can be used to share data safely in a single Vert.x instance.
*
* By default the map allows immutable keys and values.
* Custom keys and values should implement {@link io.vertx.core.shareddata.Shareable} interface. The map returns their copies.
*
* This ensures there is no shared access to mutable state from different threads (e.g. different event loops) in the
* Vert.x instance, and means you don't have to protect access to that state using synchronization or locks.
*
*
* Since the version 3.4, this class extends the interface. However some methods are only accessible in Java.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.shareddata.LocalMap original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.shareddata.LocalMap.class)
public class LocalMap {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
LocalMap that = (LocalMap) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new LocalMap((io.vertx.core.shareddata.LocalMap) obj),
LocalMap::getDelegate
);
private final io.vertx.core.shareddata.LocalMap delegate;
public final TypeArg __typeArg_0;
public final TypeArg __typeArg_1;
public LocalMap(io.vertx.core.shareddata.LocalMap delegate) {
this.delegate = delegate;
this.__typeArg_0 = TypeArg.unknown(); this.__typeArg_1 = TypeArg.unknown(); }
public LocalMap(Object delegate, TypeArg typeArg_0, TypeArg typeArg_1) {
this.delegate = (io.vertx.core.shareddata.LocalMap)delegate;
this.__typeArg_0 = typeArg_0;
this.__typeArg_1 = typeArg_1;
}
public io.vertx.core.shareddata.LocalMap getDelegate() {
return delegate;
}
/**
* Get a value from the map
* @param key the key
* @return the value, or null if none
*/
public V get(java.lang.Object key) {
V ret = (V)__typeArg_1.wrap(delegate.get(key));
return ret;
}
/**
* Put an entry in the map
* @param key the key
* @param value the value
* @return return the old value, or null if none
*/
public V put(K key, V value) {
V ret = (V)__typeArg_1.wrap(delegate.put(__typeArg_0.unwrap(key), __typeArg_1.unwrap(value)));
return ret;
}
/**
* Remove an entry from the map
* @param key the key
* @return the old value
*/
public V remove(java.lang.Object key) {
V ret = (V)__typeArg_1.wrap(delegate.remove(key));
return ret;
}
/**
* Clear all entries in the map
*/
public void clear() {
delegate.clear();
}
/**
* Get the size of the map
* @return the number of entries in the map
*/
public int size() {
int ret = delegate.size();
return ret;
}
/**
* @return true if there are zero entries in the map
*/
public boolean isEmpty() {
boolean ret = delegate.isEmpty();
return ret;
}
/**
* Put the entry only if there is no existing entry for that key
* @param key the key
* @param value the value
* @return the old value or null, if none
*/
public V putIfAbsent(K key, V value) {
V ret = (V)__typeArg_1.wrap(delegate.putIfAbsent(__typeArg_0.unwrap(key), __typeArg_1.unwrap(value)));
return ret;
}
/**
* Remove the entry only if there is an entry with the specified key and value.
*
* This method is the poyglot version of {@link io.vertx.rxjava3.core.shareddata.LocalMap#remove}.
* @param key the key
* @param value the value
* @return true if removed
*/
public boolean removeIfPresent(K key, V value) {
boolean ret = delegate.removeIfPresent(__typeArg_0.unwrap(key), __typeArg_1.unwrap(value));
return ret;
}
/**
* Replace the entry only if there is an existing entry with the specified key and value.
*
* This method is the polyglot version of {@link io.vertx.rxjava3.core.shareddata.LocalMap#replace}.
* @param key the key
* @param oldValue the old value
* @param newValue the new value
* @return true if removed
*/
public boolean replaceIfPresent(K key, V oldValue, V newValue) {
boolean ret = delegate.replaceIfPresent(__typeArg_0.unwrap(key), __typeArg_1.unwrap(oldValue), __typeArg_1.unwrap(newValue));
return ret;
}
/**
* Replace the entry only if there is an existing entry with the key
* @param key the key
* @param value the new value
* @return the old value
*/
public V replace(K key, V value) {
V ret = (V)__typeArg_1.wrap(delegate.replace(__typeArg_0.unwrap(key), __typeArg_1.unwrap(value)));
return ret;
}
/**
* Close and release the map
*/
public void close() {
delegate.close();
}
/**
* Returns true
if this map contains a mapping for the specified
* key.
* @param key key whose presence in this map is to be tested
* @return true
if this map contains a mapping for the specified key
*/
public boolean containsKey(java.lang.Object key) {
boolean ret = delegate.containsKey(key);
return ret;
}
/**
* Returns @{code true} if this map maps one or more keys to the
* specified value.
* @param value value whose presence in this map is to be tested
* @return @{code true} if this map maps one or more keys to the specified value
*/
public boolean containsValue(java.lang.Object value) {
boolean ret = delegate.containsValue(value);
return ret;
}
/**
* Returns the value to which the specified key is mapped, or
* defaultValue
if this map contains no mapping for the key.
* @param key the key whose associated value is to be returned
* @param defaultValue the default mapping of the key
* @return the value to which the specified key is mapped, or defaultValue
if this map contains no mapping for the key
*/
public V getOrDefault(java.lang.Object key, V defaultValue) {
V ret = (V)__typeArg_1.wrap(delegate.getOrDefault(key, __typeArg_1.unwrap(defaultValue)));
return ret;
}
public static LocalMap newInstance(io.vertx.core.shareddata.LocalMap arg) {
return arg != null ? new LocalMap(arg) : null;
}
public static LocalMap newInstance(io.vertx.core.shareddata.LocalMap arg, TypeArg __typeArg_K, TypeArg __typeArg_V) {
return arg != null ? new LocalMap(arg, __typeArg_K, __typeArg_V) : null;
}
}