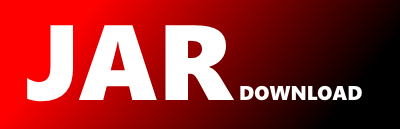
io.vertx.rxjava3.core.shareddata.SharedData Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.core.shareddata;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Shared data allows you to share data safely between different parts of your application in a safe way.
*
* Shared data provides:
*
* - synchronous shared maps (local)
* - asynchronous maps (local or cluster-wide)
* - asynchronous locks (local or cluster-wide)
* - asynchronous counters (local or cluster-wide)
*
*
*
* WARNING: In clustered mode, asynchronous maps/locks/counters rely on distributed data structures provided by the cluster manager.
* Beware that the latency relative to asynchronous maps/locks/counters operations can be much higher in clustered than in local mode.
*
* Please see the documentation for more information.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.core.shareddata.SharedData original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.core.shareddata.SharedData.class)
public class SharedData {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SharedData that = (SharedData) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new SharedData((io.vertx.core.shareddata.SharedData) obj),
SharedData::getDelegate
);
private final io.vertx.core.shareddata.SharedData delegate;
public SharedData(io.vertx.core.shareddata.SharedData delegate) {
this.delegate = delegate;
}
public SharedData(Object delegate) {
this.delegate = (io.vertx.core.shareddata.SharedData)delegate;
}
public io.vertx.core.shareddata.SharedData getDelegate() {
return delegate;
}
/**
* Get the cluster wide map with the specified name. The map is accessible to all nodes in the cluster and data
* put into the map from any node is visible to to any other node.
* @param name the name of the map
* @return
*/
public io.reactivex.rxjava3.core.Single> getClusterWideMap(java.lang.String name) {
io.reactivex.rxjava3.core.Single> ret = rxGetClusterWideMap(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the cluster wide map with the specified name. The map is accessible to all nodes in the cluster and data
* put into the map from any node is visible to to any other node.
* @param name the name of the map
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetClusterWideMap(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getClusterWideMap(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.AsyncMap.newInstance((io.vertx.core.shareddata.AsyncMap)event, TypeArg.unknown(), TypeArg.unknown()))));
});
}
/**
* Get the {@link io.vertx.rxjava3.core.shareddata.AsyncMap} with the specified name. When clustered, the map is accessible to all nodes in the cluster
* and data put into the map from any node is visible to to any other node.
*
* WARNING: In clustered mode, asynchronous shared maps rely on distributed data structures provided by the cluster manager.
* Beware that the latency relative to asynchronous shared maps operations can be much higher in clustered than in local mode.
*
* @param name the name of the map
* @return
*/
public io.reactivex.rxjava3.core.Single> getAsyncMap(java.lang.String name) {
io.reactivex.rxjava3.core.Single> ret = rxGetAsyncMap(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the {@link io.vertx.rxjava3.core.shareddata.AsyncMap} with the specified name. When clustered, the map is accessible to all nodes in the cluster
* and data put into the map from any node is visible to to any other node.
*
* WARNING: In clustered mode, asynchronous shared maps rely on distributed data structures provided by the cluster manager.
* Beware that the latency relative to asynchronous shared maps operations can be much higher in clustered than in local mode.
*
* @param name the name of the map
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetAsyncMap(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getAsyncMap(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.AsyncMap.newInstance((io.vertx.core.shareddata.AsyncMap)event, TypeArg.unknown(), TypeArg.unknown()))));
});
}
/**
* Get the {@link io.vertx.rxjava3.core.shareddata.AsyncMap} with the specified name.
*
* When clustered, the map is NOT accessible to all nodes in the cluster.
* Only the instance which created the map can put and retrieve data from this map.
* @param name the name of the map
* @return
*/
public io.reactivex.rxjava3.core.Single> getLocalAsyncMap(java.lang.String name) {
io.reactivex.rxjava3.core.Single> ret = rxGetLocalAsyncMap(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the {@link io.vertx.rxjava3.core.shareddata.AsyncMap} with the specified name.
*
* When clustered, the map is NOT accessible to all nodes in the cluster.
* Only the instance which created the map can put and retrieve data from this map.
* @param name the name of the map
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetLocalAsyncMap(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getLocalAsyncMap(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.AsyncMap.newInstance((io.vertx.core.shareddata.AsyncMap)event, TypeArg.unknown(), TypeArg.unknown()))));
});
}
/**
* Get an asynchronous lock with the specified name. The lock will be passed to the handler when it is available.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @return
*/
public io.reactivex.rxjava3.core.Single getLock(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxGetLock(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get an asynchronous lock with the specified name. The lock will be passed to the handler when it is available.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetLock(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getLock(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.Lock.newInstance((io.vertx.core.shareddata.Lock)event))));
});
}
/**
* Like {@link io.vertx.rxjava3.core.shareddata.SharedData#getLock} but specifying a timeout. If the lock is not obtained within the timeout
* a failure will be sent to the handler.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @param timeout the timeout in ms
* @return
*/
public io.reactivex.rxjava3.core.Single getLockWithTimeout(java.lang.String name, long timeout) {
io.reactivex.rxjava3.core.Single ret = rxGetLockWithTimeout(name, timeout);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.shareddata.SharedData#getLock} but specifying a timeout. If the lock is not obtained within the timeout
* a failure will be sent to the handler.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @param timeout the timeout in ms
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetLockWithTimeout(java.lang.String name, long timeout) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getLockWithTimeout(name, timeout, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.Lock.newInstance((io.vertx.core.shareddata.Lock)event))));
});
}
/**
* Get an asynchronous local lock with the specified name. The lock will be passed to the handler when it is available.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @return
*/
public io.reactivex.rxjava3.core.Single getLocalLock(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxGetLocalLock(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get an asynchronous local lock with the specified name. The lock will be passed to the handler when it is available.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetLocalLock(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getLocalLock(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.Lock.newInstance((io.vertx.core.shareddata.Lock)event))));
});
}
/**
* Like {@link io.vertx.rxjava3.core.shareddata.SharedData#getLocalLock} but specifying a timeout. If the lock is not obtained within the timeout
* a failure will be sent to the handler.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @param timeout the timeout in ms
* @return
*/
public io.reactivex.rxjava3.core.Single getLocalLockWithTimeout(java.lang.String name, long timeout) {
io.reactivex.rxjava3.core.Single ret = rxGetLocalLockWithTimeout(name, timeout);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.core.shareddata.SharedData#getLocalLock} but specifying a timeout. If the lock is not obtained within the timeout
* a failure will be sent to the handler.
*
* In general lock acquision is unordered, so that sequential attempts to acquire a lock,
* even from a single thread, can happen in non-sequential order.
*
* @param name the name of the lock
* @param timeout the timeout in ms
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetLocalLockWithTimeout(java.lang.String name, long timeout) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getLocalLockWithTimeout(name, timeout, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.Lock.newInstance((io.vertx.core.shareddata.Lock)event))));
});
}
/**
* Get an asynchronous counter. The counter will be passed to the handler.
* @param name the name of the counter.
* @return
*/
public io.reactivex.rxjava3.core.Single getCounter(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxGetCounter(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get an asynchronous counter. The counter will be passed to the handler.
* @param name the name of the counter.
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetCounter(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getCounter(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.Counter.newInstance((io.vertx.core.shareddata.Counter)event))));
});
}
/**
* Get an asynchronous local counter. The counter will be passed to the handler.
* @param name the name of the counter.
* @return
*/
public io.reactivex.rxjava3.core.Single getLocalCounter(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxGetLocalCounter(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get an asynchronous local counter. The counter will be passed to the handler.
* @param name the name of the counter.
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetLocalCounter(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getLocalCounter(name, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.shareddata.Counter.newInstance((io.vertx.core.shareddata.Counter)event))));
});
}
/**
* Return a LocalMap
with the specific name
.
* @param name the name of the map
* @return the map
*/
public io.vertx.rxjava3.core.shareddata.LocalMap getLocalMap(java.lang.String name) {
io.vertx.rxjava3.core.shareddata.LocalMap ret = io.vertx.rxjava3.core.shareddata.LocalMap.newInstance((io.vertx.core.shareddata.LocalMap)delegate.getLocalMap(name), TypeArg.unknown(), TypeArg.unknown());
return ret;
}
public static SharedData newInstance(io.vertx.core.shareddata.SharedData arg) {
return arg != null ? new SharedData(arg) : null;
}
}