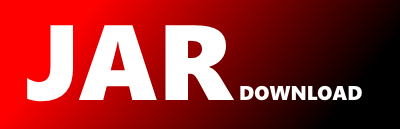
io.vertx.rxjava3.ext.auth.webauthn.WebAuthn Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.auth.webauthn;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Factory interface for creating WebAuthN based {@link io.vertx.rxjava3.ext.auth.authentication.AuthenticationProvider} instances.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.webauthn.WebAuthn original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.auth.webauthn.WebAuthn.class)
public class WebAuthn extends io.vertx.rxjava3.ext.auth.authentication.AuthenticationProvider {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
WebAuthn that = (WebAuthn) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new WebAuthn((io.vertx.ext.auth.webauthn.WebAuthn) obj),
WebAuthn::getDelegate
);
private final io.vertx.ext.auth.webauthn.WebAuthn delegate;
public WebAuthn(io.vertx.ext.auth.webauthn.WebAuthn delegate) {
super(delegate);
this.delegate = delegate;
}
public WebAuthn(Object delegate) {
super((io.vertx.ext.auth.webauthn.WebAuthn)delegate);
this.delegate = (io.vertx.ext.auth.webauthn.WebAuthn)delegate;
}
public io.vertx.ext.auth.webauthn.WebAuthn getDelegate() {
return delegate;
}
/**
* Create a WebAuthN auth provider
* @param vertx the Vertx instance.
* @return the auth provider.
*/
public static io.vertx.rxjava3.ext.auth.webauthn.WebAuthn create(io.vertx.rxjava3.core.Vertx vertx) {
io.vertx.rxjava3.ext.auth.webauthn.WebAuthn ret = io.vertx.rxjava3.ext.auth.webauthn.WebAuthn.newInstance((io.vertx.ext.auth.webauthn.WebAuthn)io.vertx.ext.auth.webauthn.WebAuthn.create(vertx.getDelegate()));
return ret;
}
/**
* Create a WebAuthN auth provider
* @param vertx the Vertx instance.
* @param options the custom options to the provider.
* @return the auth provider.
*/
public static io.vertx.rxjava3.ext.auth.webauthn.WebAuthn create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.ext.auth.webauthn.WebAuthnOptions options) {
io.vertx.rxjava3.ext.auth.webauthn.WebAuthn ret = io.vertx.rxjava3.ext.auth.webauthn.WebAuthn.newInstance((io.vertx.ext.auth.webauthn.WebAuthn)io.vertx.ext.auth.webauthn.WebAuthn.create(vertx.getDelegate(), options));
return ret;
}
/**
* Gets a challenge and any other parameters for the navigator.credentials.create()
call.
*
* The object being returned is described here https://w3c.github.io/webauthn/#dictdef-publickeycredentialcreationoptions
* @param user - the user object with name and optionally displayName and icon
* @return fluent self
*/
public io.reactivex.rxjava3.core.Single createCredentialsOptions(io.vertx.core.json.JsonObject user) {
io.reactivex.rxjava3.core.Single ret = rxCreateCredentialsOptions(user);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Gets a challenge and any other parameters for the navigator.credentials.create()
call.
*
* The object being returned is described here https://w3c.github.io/webauthn/#dictdef-publickeycredentialcreationoptions
* @param user - the user object with name and optionally displayName and icon
* @return fluent self
*/
public io.reactivex.rxjava3.core.Single rxCreateCredentialsOptions(io.vertx.core.json.JsonObject user) {
return AsyncResultSingle.toSingle( handler -> {
delegate.createCredentialsOptions(user, handler);
});
}
/**
* Creates an assertion challenge and any other parameters for the navigator.credentials.get()
call.
* If the auth provider is configured with RequireResidentKey
and the username is null then the
* generated assertion will be a RK assertion (Usernameless).
*
* The object being returned is described here https://w3c.github.io/webauthn/#dictdef-publickeycredentialcreationoptions
* @param name the unique user identified
* @return fluent self.
*/
public io.reactivex.rxjava3.core.Single getCredentialsOptions(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxGetCredentialsOptions(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates an assertion challenge and any other parameters for the navigator.credentials.get()
call.
* If the auth provider is configured with RequireResidentKey
and the username is null then the
* generated assertion will be a RK assertion (Usernameless).
*
* The object being returned is described here https://w3c.github.io/webauthn/#dictdef-publickeycredentialcreationoptions
* @param name the unique user identified
* @return fluent self.
*/
public io.reactivex.rxjava3.core.Single rxGetCredentialsOptions(java.lang.String name) {
return AsyncResultSingle.toSingle( handler -> {
delegate.getCredentialsOptions(name, handler);
});
}
/**
* Provide a that can fetch {@link io.vertx.ext.auth.webauthn.Authenticator}s from a backend given the incomplete
* {@link io.vertx.ext.auth.webauthn.Authenticator} argument.
*
* The implementation must consider the following fields exclusively, while performing the lookup:
*
* - {@link io.vertx.ext.auth.webauthn.Authenticator}
* - {@link io.vertx.ext.auth.webauthn.Authenticator} ()}
*
*
* It may return more than 1 result, for example when a user can be identified using different modalities.
* To signal that a user is not allowed/present on the system, a failure should be returned, not null
.
*
* The function signature is as follows:
*
* (Authenticator) -> Future>>
*
*
* - {@link io.vertx.ext.auth.webauthn.Authenticator} the incomplete authenticator data to lookup.
* - async result with a list of authenticators.
*
* @param fetcher fetcher function.
* @return fluent self.
*/
public io.vertx.rxjava3.ext.auth.webauthn.WebAuthn authenticatorFetcher(java.util.function.Function>> fetcher) {
delegate.authenticatorFetcher(new Function>>() {
public io.vertx.core.Future> apply(io.vertx.ext.auth.webauthn.Authenticator arg) {
io.reactivex.rxjava3.core.Single> ret = fetcher.apply(arg);
return io.vertx.rxjava3.SingleHelper.toFuture(ret, obj -> obj);
}
});
return this;
}
/**
* Provide a that can update or insert a {@link io.vertx.ext.auth.webauthn.Authenticator}.
* The function should store a given authenticator to a persistence storage.
*
* When an authenticator is already present, this method must at least update
* {@link io.vertx.ext.auth.webauthn.Authenticator}, and is not required to perform any other update.
*
* For new authenticators, the whole object data must be persisted.
*
* The function signature is as follows:
*
* (Authenticator) -> Future
*
*
* - {@link io.vertx.ext.auth.webauthn.Authenticator} the authenticator data to update.
* - async result of the operation.
*
* @param updater updater function.
* @return fluent self.
*/
public io.vertx.rxjava3.ext.auth.webauthn.WebAuthn authenticatorUpdater(java.util.function.Function updater) {
delegate.authenticatorUpdater(new Function>() {
public io.vertx.core.Future apply(io.vertx.ext.auth.webauthn.Authenticator arg) {
io.reactivex.rxjava3.core.Completable ret = updater.apply(arg);
return io.vertx.rxjava3.CompletableHelper.toFuture(ret);
}
});
return this;
}
/**
* Getter to the instance FIDO2 Meta Data Service.
* @return the MDS instance.
*/
public io.vertx.rxjava3.ext.auth.webauthn.MetaDataService metaDataService() {
io.vertx.rxjava3.ext.auth.webauthn.MetaDataService ret = io.vertx.rxjava3.ext.auth.webauthn.MetaDataService.newInstance((io.vertx.ext.auth.webauthn.MetaDataService)delegate.metaDataService());
return ret;
}
public static WebAuthn newInstance(io.vertx.ext.auth.webauthn.WebAuthn arg) {
return arg != null ? new WebAuthn(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy