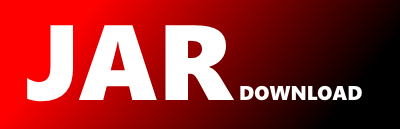
io.vertx.rxjava3.ext.consul.ConsulClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.consul;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A Vert.x service used to interact with Consul.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.consul.ConsulClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.consul.ConsulClient.class)
public class ConsulClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ConsulClient that = (ConsulClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new ConsulClient((io.vertx.ext.consul.ConsulClient) obj),
ConsulClient::getDelegate
);
private final io.vertx.ext.consul.ConsulClient delegate;
public ConsulClient(io.vertx.ext.consul.ConsulClient delegate) {
this.delegate = delegate;
}
public ConsulClient(Object delegate) {
this.delegate = (io.vertx.ext.consul.ConsulClient)delegate;
}
public io.vertx.ext.consul.ConsulClient getDelegate() {
return delegate;
}
/**
* Create a Consul client with default options.
* @param vertx the Vert.x instance
* @return the client
*/
public static io.vertx.rxjava3.ext.consul.ConsulClient create(io.vertx.rxjava3.core.Vertx vertx) {
io.vertx.rxjava3.ext.consul.ConsulClient ret = io.vertx.rxjava3.ext.consul.ConsulClient.newInstance((io.vertx.ext.consul.ConsulClient)io.vertx.ext.consul.ConsulClient.create(vertx.getDelegate()));
return ret;
}
/**
* Create a Consul client.
* @param vertx the Vert.x instance
* @param options the options
* @return the client
*/
public static io.vertx.rxjava3.ext.consul.ConsulClient create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.ext.consul.ConsulClientOptions options) {
io.vertx.rxjava3.ext.consul.ConsulClient ret = io.vertx.rxjava3.ext.consul.ConsulClient.newInstance((io.vertx.ext.consul.ConsulClient)io.vertx.ext.consul.ConsulClient.create(vertx.getDelegate(), options));
return ret;
}
/**
* Returns the configuration and member information of the local agent
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single agentInfo() {
io.reactivex.rxjava3.core.Single ret = rxAgentInfo();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the configuration and member information of the local agent
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxAgentInfo() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.agentInfo(resultHandler);
});
}
/**
* Returns the LAN network coordinates for all nodes in a given DC
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single coordinateNodes() {
io.reactivex.rxjava3.core.Single ret = rxCoordinateNodes();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the LAN network coordinates for all nodes in a given DC
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCoordinateNodes() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.coordinateNodes(resultHandler);
});
}
/**
* Returns the LAN network coordinates for all nodes in a given DC
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#coordinateNodes}
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single coordinateNodesWithOptions(io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCoordinateNodesWithOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the LAN network coordinates for all nodes in a given DC
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#coordinateNodes}
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCoordinateNodesWithOptions(io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.coordinateNodesWithOptions(options, resultHandler);
});
}
/**
* Returns the WAN network coordinates for all Consul servers, organized by DCs
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> coordinateDatacenters() {
io.reactivex.rxjava3.core.Single> ret = rxCoordinateDatacenters();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the WAN network coordinates for all Consul servers, organized by DCs
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxCoordinateDatacenters() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.coordinateDatacenters(resultHandler);
});
}
/**
* Returns the list of keys that corresponding to the specified key prefix.
* @param keyPrefix the prefix
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> getKeys(java.lang.String keyPrefix) {
io.reactivex.rxjava3.core.Single> ret = rxGetKeys(keyPrefix);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the list of keys that corresponding to the specified key prefix.
* @param keyPrefix the prefix
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxGetKeys(java.lang.String keyPrefix) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getKeys(keyPrefix, resultHandler);
});
}
/**
* Returns the list of keys that corresponding to the specified key prefix.
* @param keyPrefix the prefix
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> getKeysWithOptions(java.lang.String keyPrefix, io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single> ret = rxGetKeysWithOptions(keyPrefix, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the list of keys that corresponding to the specified key prefix.
* @param keyPrefix the prefix
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxGetKeysWithOptions(java.lang.String keyPrefix, io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getKeysWithOptions(keyPrefix, options, resultHandler);
});
}
/**
* Returns key/value pair that corresponding to the specified key.
* An empty {@link io.vertx.ext.consul.KeyValue} object will be returned if no such key is found.
* @param key the key
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single getValue(java.lang.String key) {
io.reactivex.rxjava3.core.Single ret = rxGetValue(key);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns key/value pair that corresponding to the specified key.
* An empty {@link io.vertx.ext.consul.KeyValue} object will be returned if no such key is found.
* @param key the key
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxGetValue(java.lang.String key) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getValue(key, resultHandler);
});
}
/**
* Returns key/value pair that corresponding to the specified key.
* An empty {@link io.vertx.ext.consul.KeyValue} object will be returned if no such key is found.
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#getValue}
* @param key the key
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single getValueWithOptions(java.lang.String key, io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxGetValueWithOptions(key, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns key/value pair that corresponding to the specified key.
* An empty {@link io.vertx.ext.consul.KeyValue} object will be returned if no such key is found.
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#getValue}
* @param key the key
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxGetValueWithOptions(java.lang.String key, io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getValueWithOptions(key, options, resultHandler);
});
}
/**
* Remove the key/value pair that corresponding to the specified key
* @param key the key
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable deleteValue(java.lang.String key) {
io.reactivex.rxjava3.core.Completable ret = rxDeleteValue(key);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Remove the key/value pair that corresponding to the specified key
* @param key the key
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDeleteValue(java.lang.String key) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.deleteValue(key, resultHandler);
});
}
/**
* Returns the list of key/value pairs that corresponding to the specified key prefix.
* An empty {@link io.vertx.ext.consul.KeyValueList} object will be returned if no such key prefix is found.
* @param keyPrefix the prefix
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single getValues(java.lang.String keyPrefix) {
io.reactivex.rxjava3.core.Single ret = rxGetValues(keyPrefix);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the list of key/value pairs that corresponding to the specified key prefix.
* An empty {@link io.vertx.ext.consul.KeyValueList} object will be returned if no such key prefix is found.
* @param keyPrefix the prefix
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxGetValues(java.lang.String keyPrefix) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getValues(keyPrefix, resultHandler);
});
}
/**
* Returns the list of key/value pairs that corresponding to the specified key prefix.
* An empty {@link io.vertx.ext.consul.KeyValueList} object will be returned if no such key prefix is found.
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#getValues}
* @param keyPrefix the prefix
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single getValuesWithOptions(java.lang.String keyPrefix, io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxGetValuesWithOptions(keyPrefix, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the list of key/value pairs that corresponding to the specified key prefix.
* An empty {@link io.vertx.ext.consul.KeyValueList} object will be returned if no such key prefix is found.
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#getValues}
* @param keyPrefix the prefix
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxGetValuesWithOptions(java.lang.String keyPrefix, io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getValuesWithOptions(keyPrefix, options, resultHandler);
});
}
/**
* Removes all the key/value pair that corresponding to the specified key prefix
* @param keyPrefix the prefix
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable deleteValues(java.lang.String keyPrefix) {
io.reactivex.rxjava3.core.Completable ret = rxDeleteValues(keyPrefix);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Removes all the key/value pair that corresponding to the specified key prefix
* @param keyPrefix the prefix
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDeleteValues(java.lang.String keyPrefix) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.deleteValues(keyPrefix, resultHandler);
});
}
/**
* Adds specified key/value pair
* @param key the key
* @param value the value
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single putValue(java.lang.String key, java.lang.String value) {
io.reactivex.rxjava3.core.Single ret = rxPutValue(key, value);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Adds specified key/value pair
* @param key the key
* @param value the value
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxPutValue(java.lang.String key, java.lang.String value) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.putValue(key, value, resultHandler);
});
}
/**
* @param key the key
* @param value the value
* @param options options used to push pair
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single putValueWithOptions(java.lang.String key, java.lang.String value, io.vertx.ext.consul.KeyValueOptions options) {
io.reactivex.rxjava3.core.Single ret = rxPutValueWithOptions(key, value, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* @param key the key
* @param value the value
* @param options options used to push pair
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxPutValueWithOptions(java.lang.String key, java.lang.String value, io.vertx.ext.consul.KeyValueOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.putValueWithOptions(key, value, options, resultHandler);
});
}
/**
* Manages multiple operations inside a single, atomic transaction.
* @param request transaction request
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single transaction(io.vertx.ext.consul.TxnRequest request) {
io.reactivex.rxjava3.core.Single ret = rxTransaction(request);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Manages multiple operations inside a single, atomic transaction.
* @param request transaction request
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxTransaction(io.vertx.ext.consul.TxnRequest request) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.transaction(request, resultHandler);
});
}
/**
* Creates a new ACL policy
* @param policy properties of policy
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single createAclPolicy(io.vertx.ext.consul.policy.AclPolicy policy) {
io.reactivex.rxjava3.core.Single ret = rxCreateAclPolicy(policy);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a new ACL policy
* @param policy properties of policy
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCreateAclPolicy(io.vertx.ext.consul.policy.AclPolicy policy) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.createAclPolicy(policy, resultHandler);
});
}
/**
* This endpoint reads an ACL policy with the given ID
* @param id uuid policy
* @return a reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single readPolicy(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxReadPolicy(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* This endpoint reads an ACL policy with the given ID
* @param id uuid policy
* @return a reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxReadPolicy(java.lang.String id) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.readPolicy(id, resultHandler);
});
}
/**
* This endpoint reads an ACL policy with the given name
* @param name unique name of created policy
* @return a reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single readPolicyByName(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxReadPolicyByName(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* This endpoint reads an ACL policy with the given name
* @param name unique name of created policy
* @return a reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxReadPolicyByName(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.readPolicyByName(name, resultHandler);
});
}
/**
* This endpoint updates an existing ACL policy
* @param id uuid of existing policy
* @param policy options that will be applied to the existing policy
* @return a reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single updatePolicy(java.lang.String id, io.vertx.ext.consul.policy.AclPolicy policy) {
io.reactivex.rxjava3.core.Single ret = rxUpdatePolicy(id, policy);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* This endpoint updates an existing ACL policy
* @param id uuid of existing policy
* @param policy options that will be applied to the existing policy
* @return a reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxUpdatePolicy(java.lang.String id, io.vertx.ext.consul.policy.AclPolicy policy) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.updatePolicy(id, policy, resultHandler);
});
}
/**
* This endpoint deletes an ACL policy
* @param id uuid of existing policy
* @param resultHandler will be provided with result of policy deleting
* @return a reference to this, for fluency
*/
public io.vertx.rxjava3.ext.consul.ConsulClient deletePolicy(java.lang.String id, io.vertx.core.Handler> resultHandler) {
io.vertx.rxjava3.ext.consul.ConsulClient ret = io.vertx.rxjava3.ext.consul.ConsulClient.newInstance((io.vertx.ext.consul.ConsulClient)delegate.deletePolicy(id, resultHandler));
return ret;
}
/**
* This endpoint lists all the ACL policies.
* Note - The policies rules are not included in the listing and must be retrieved by the policy reading endpoint
* @param resultHandler will be provided with result of policy deleting
* @return a reference to this, for fluency
*/
public io.vertx.rxjava3.ext.consul.ConsulClient getAclPolicies(io.vertx.core.Handler>> resultHandler) {
io.vertx.rxjava3.ext.consul.ConsulClient ret = io.vertx.rxjava3.ext.consul.ConsulClient.newInstance((io.vertx.ext.consul.ConsulClient)delegate.getAclPolicies(resultHandler));
return ret;
}
/**
* Create an Acl token
* @param token properties of the token
* @return reference to this, for fluency {@link io.vertx.ext.consul.token.AclToken} accessorId - required in the URL path or JSON body for getting, updating and cloning token. {@link io.vertx.ext.consul.token.AclToken} secretId - using in {@link io.vertx.ext.consul.ConsulClientOptions}.
*/
public io.reactivex.rxjava3.core.Single createAclToken(io.vertx.ext.consul.token.AclToken token) {
io.reactivex.rxjava3.core.Single ret = rxCreateAclToken(token);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create an Acl token
* @param token properties of the token
* @return reference to this, for fluency {@link io.vertx.ext.consul.token.AclToken} accessorId - required in the URL path or JSON body for getting, updating and cloning token. {@link io.vertx.ext.consul.token.AclToken} secretId - using in {@link io.vertx.ext.consul.ConsulClientOptions}.
*/
public io.reactivex.rxjava3.core.Single rxCreateAclToken(io.vertx.ext.consul.token.AclToken token) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.createAclToken(token, resultHandler);
});
}
/**
* Update an existing Acl token
* @param accessorId uuid of the token
* @param token properties of the token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single updateAclToken(java.lang.String accessorId, io.vertx.ext.consul.token.AclToken token) {
io.reactivex.rxjava3.core.Single ret = rxUpdateAclToken(accessorId, token);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Update an existing Acl token
* @param accessorId uuid of the token
* @param token properties of the token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxUpdateAclToken(java.lang.String accessorId, io.vertx.ext.consul.token.AclToken token) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.updateAclToken(accessorId, token, resultHandler);
});
}
/**
* Clones an existing ACL token
* @param accessorId uuid of the token
* @param cloneAclToken properties of cloned token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single cloneAclToken(java.lang.String accessorId, io.vertx.ext.consul.token.CloneAclTokenOptions cloneAclToken) {
io.reactivex.rxjava3.core.Single ret = rxCloneAclToken(accessorId, cloneAclToken);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Clones an existing ACL token
* @param accessorId uuid of the token
* @param cloneAclToken properties of cloned token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCloneAclToken(java.lang.String accessorId, io.vertx.ext.consul.token.CloneAclTokenOptions cloneAclToken) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.cloneAclToken(accessorId, cloneAclToken, resultHandler);
});
}
/**
* Get list of Acl token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> getAclTokens() {
io.reactivex.rxjava3.core.Single> ret = rxGetAclTokens();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get list of Acl token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxGetAclTokens() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getAclTokens(resultHandler);
});
}
/**
* Reads an ACL token with the given Accessor ID
* @param accessorId uuid of token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single readAclToken(java.lang.String accessorId) {
io.reactivex.rxjava3.core.Single ret = rxReadAclToken(accessorId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Reads an ACL token with the given Accessor ID
* @param accessorId uuid of token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxReadAclToken(java.lang.String accessorId) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.readAclToken(accessorId, resultHandler);
});
}
/**
* Deletes an ACL token
* @param accessorId uuid of token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single deleteAclToken(java.lang.String accessorId) {
io.reactivex.rxjava3.core.Single ret = rxDeleteAclToken(accessorId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Deletes an ACL token
* @param accessorId uuid of token
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxDeleteAclToken(java.lang.String accessorId) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.deleteAclToken(accessorId, resultHandler);
});
}
/**
* Legacy create new Acl token
* @param token properties of the token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single createAclToken(io.vertx.ext.consul.AclToken token) {
io.reactivex.rxjava3.core.Single ret = rxCreateAclToken(token);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Legacy create new Acl token
* @param token properties of the token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxCreateAclToken(io.vertx.ext.consul.AclToken token) {
return AsyncResultSingle.toSingle( idHandler -> {
delegate.createAclToken(token, idHandler);
});
}
/**
* Update Acl token
* @param token properties of the token to be updated
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single updateAclToken(io.vertx.ext.consul.AclToken token) {
io.reactivex.rxjava3.core.Single ret = rxUpdateAclToken(token);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Update Acl token
* @param token properties of the token to be updated
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxUpdateAclToken(io.vertx.ext.consul.AclToken token) {
return AsyncResultSingle.toSingle( idHandler -> {
delegate.updateAclToken(token, idHandler);
});
}
/**
* Clone Acl token
* @param id the ID of token to be cloned
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single cloneAclToken(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxCloneAclToken(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Clone Acl token
* @param id the ID of token to be cloned
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxCloneAclToken(java.lang.String id) {
return AsyncResultSingle.toSingle( idHandler -> {
delegate.cloneAclToken(id, idHandler);
});
}
/**
* Get list of Acl token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single> listAclTokens() {
io.reactivex.rxjava3.core.Single> ret = rxListAclTokens();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get list of Acl token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single> rxListAclTokens() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listAclTokens(resultHandler);
});
}
/**
* Get info of Acl token
* @param id the ID of token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single infoAclToken(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxInfoAclToken(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get info of Acl token
* @param id the ID of token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxInfoAclToken(java.lang.String id) {
return AsyncResultSingle.toSingle( tokenHandler -> {
delegate.infoAclToken(id, tokenHandler);
});
}
/**
* Destroy Acl token
* @param id the ID of token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Completable destroyAclToken(java.lang.String id) {
io.reactivex.rxjava3.core.Completable ret = rxDestroyAclToken(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Destroy Acl token
* @param id the ID of token
* @return reference to this, for fluency
*/
@Deprecated()
public io.reactivex.rxjava3.core.Completable rxDestroyAclToken(java.lang.String id) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.destroyAclToken(id, resultHandler);
});
}
/**
* Fires a new user event
* @param name name of event
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single fireEvent(java.lang.String name) {
io.reactivex.rxjava3.core.Single ret = rxFireEvent(name);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Fires a new user event
* @param name name of event
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxFireEvent(java.lang.String name) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.fireEvent(name, resultHandler);
});
}
/**
* Fires a new user event
* @param name name of event
* @param options options used to create event
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single fireEventWithOptions(java.lang.String name, io.vertx.ext.consul.EventOptions options) {
io.reactivex.rxjava3.core.Single ret = rxFireEventWithOptions(name, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Fires a new user event
* @param name name of event
* @param options options used to create event
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxFireEventWithOptions(java.lang.String name, io.vertx.ext.consul.EventOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.fireEventWithOptions(name, options, resultHandler);
});
}
/**
* Returns the most recent events known by the agent
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single listEvents() {
io.reactivex.rxjava3.core.Single ret = rxListEvents();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the most recent events known by the agent
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxListEvents() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listEvents(resultHandler);
});
}
/**
* Returns the most recent events known by the agent.
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#listEvents}. However, the semantics of this endpoint
* are slightly different. Most blocking queries provide a monotonic index and block until a newer index is available.
* This can be supported as a consequence of the total ordering of the consensus protocol. With gossip,
* there is no ordering, and instead X-Consul-Index
maps to the newest event that matches the query.
*
* In practice, this means the index is only useful when used against a single agent and has no meaning globally.
* Because Consul defines the index as being opaque, clients should not be expecting a natural ordering either.
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single listEventsWithOptions(io.vertx.ext.consul.EventListOptions options) {
io.reactivex.rxjava3.core.Single ret = rxListEventsWithOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the most recent events known by the agent.
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#listEvents}. However, the semantics of this endpoint
* are slightly different. Most blocking queries provide a monotonic index and block until a newer index is available.
* This can be supported as a consequence of the total ordering of the consensus protocol. With gossip,
* there is no ordering, and instead X-Consul-Index
maps to the newest event that matches the query.
*
* In practice, this means the index is only useful when used against a single agent and has no meaning globally.
* Because Consul defines the index as being opaque, clients should not be expecting a natural ordering either.
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxListEventsWithOptions(io.vertx.ext.consul.EventListOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listEventsWithOptions(options, resultHandler);
});
}
/**
* Adds a new service, with an optional health check, to the local agent.
* @param serviceOptions the options of new service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable registerService(io.vertx.ext.consul.ServiceOptions serviceOptions) {
io.reactivex.rxjava3.core.Completable ret = rxRegisterService(serviceOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Adds a new service, with an optional health check, to the local agent.
* @param serviceOptions the options of new service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxRegisterService(io.vertx.ext.consul.ServiceOptions serviceOptions) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.registerService(serviceOptions, resultHandler);
});
}
/**
* Places a given service into "maintenance mode"
* @param maintenanceOptions the maintenance options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable maintenanceService(io.vertx.ext.consul.MaintenanceOptions maintenanceOptions) {
io.reactivex.rxjava3.core.Completable ret = rxMaintenanceService(maintenanceOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Places a given service into "maintenance mode"
* @param maintenanceOptions the maintenance options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxMaintenanceService(io.vertx.ext.consul.MaintenanceOptions maintenanceOptions) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.maintenanceService(maintenanceOptions, resultHandler);
});
}
/**
* Remove a service from the local agent. The agent will take care of deregistering the service with the Catalog.
* If there is an associated check, that is also deregistered.
* @param id the ID of service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable deregisterService(java.lang.String id) {
io.reactivex.rxjava3.core.Completable ret = rxDeregisterService(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Remove a service from the local agent. The agent will take care of deregistering the service with the Catalog.
* If there is an associated check, that is also deregistered.
* @param id the ID of service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDeregisterService(java.lang.String id) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.deregisterService(id, resultHandler);
});
}
/**
* Returns the nodes providing a service
* @param service name of service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogServiceNodes(java.lang.String service) {
io.reactivex.rxjava3.core.Single ret = rxCatalogServiceNodes(service);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the nodes providing a service
* @param service name of service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogServiceNodes(java.lang.String service) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogServiceNodes(service, resultHandler);
});
}
/**
* Returns the nodes providing a service
* @param service name of service
* @param options options used to request services
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogServiceNodesWithOptions(java.lang.String service, io.vertx.ext.consul.ServiceQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCatalogServiceNodesWithOptions(service, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the nodes providing a service
* @param service name of service
* @param options options used to request services
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogServiceNodesWithOptions(java.lang.String service, io.vertx.ext.consul.ServiceQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogServiceNodesWithOptions(service, options, resultHandler);
});
}
/**
* Return all the datacenters that are known by the Consul server
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> catalogDatacenters() {
io.reactivex.rxjava3.core.Single> ret = rxCatalogDatacenters();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Return all the datacenters that are known by the Consul server
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxCatalogDatacenters() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogDatacenters(resultHandler);
});
}
/**
* Returns the nodes registered in a datacenter
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogNodes() {
io.reactivex.rxjava3.core.Single ret = rxCatalogNodes();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the nodes registered in a datacenter
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogNodes() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogNodes(resultHandler);
});
}
/**
* Returns the nodes registered in a datacenter
* @param options options used to request nodes
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogNodesWithOptions(io.vertx.ext.consul.NodeQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCatalogNodesWithOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the nodes registered in a datacenter
* @param options options used to request nodes
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogNodesWithOptions(io.vertx.ext.consul.NodeQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogNodesWithOptions(options, resultHandler);
});
}
/**
* Returns the checks associated with the service
* @param service the service name
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single healthChecks(java.lang.String service) {
io.reactivex.rxjava3.core.Single ret = rxHealthChecks(service);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the checks associated with the service
* @param service the service name
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxHealthChecks(java.lang.String service) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthChecks(service, resultHandler);
});
}
/**
* Returns the checks associated with the service
* @param service the service name
* @param options options used to request checks
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single healthChecksWithOptions(java.lang.String service, io.vertx.ext.consul.CheckQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxHealthChecksWithOptions(service, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the checks associated with the service
* @param service the service name
* @param options options used to request checks
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxHealthChecksWithOptions(java.lang.String service, io.vertx.ext.consul.CheckQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthChecksWithOptions(service, options, resultHandler);
});
}
/**
* Returns the checks in the specified status
* @param healthState the health state
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single healthState(io.vertx.ext.consul.HealthState healthState) {
io.reactivex.rxjava3.core.Single ret = rxHealthState(healthState);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the checks in the specified status
* @param healthState the health state
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxHealthState(io.vertx.ext.consul.HealthState healthState) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthState(healthState, resultHandler);
});
}
/**
* Returns the checks in the specified status
* @param healthState the health state
* @param options options used to request checks
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single healthStateWithOptions(io.vertx.ext.consul.HealthState healthState, io.vertx.ext.consul.CheckQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxHealthStateWithOptions(healthState, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the checks in the specified status
* @param healthState the health state
* @param options options used to request checks
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxHealthStateWithOptions(io.vertx.ext.consul.HealthState healthState, io.vertx.ext.consul.CheckQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthStateWithOptions(healthState, options, resultHandler);
});
}
/**
* Returns the nodes providing the service. This endpoint is very similar to the {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogServiceNodes} endpoint;
* however, this endpoint automatically returns the status of the associated health check as well as any system level health checks.
* @param service the service name
* @param passing if true, filter results to only nodes with all checks in the passing state
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single healthServiceNodes(java.lang.String service, boolean passing) {
io.reactivex.rxjava3.core.Single ret = rxHealthServiceNodes(service, passing);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the nodes providing the service. This endpoint is very similar to the {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogServiceNodes} endpoint;
* however, this endpoint automatically returns the status of the associated health check as well as any system level health checks.
* @param service the service name
* @param passing if true, filter results to only nodes with all checks in the passing state
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxHealthServiceNodes(java.lang.String service, boolean passing) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthServiceNodes(service, passing, resultHandler);
});
}
/**
* Returns the nodes providing the service. This endpoint is very similar to the {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogServiceNodesWithOptions} endpoint;
* however, this endpoint automatically returns the status of the associated health check as well as any system level health checks.
* @param service the service name
* @param passing if true, filter results to only nodes with all checks in the passing state
* @param options options used to request services
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single healthServiceNodesWithOptions(java.lang.String service, boolean passing, io.vertx.ext.consul.ServiceQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxHealthServiceNodesWithOptions(service, passing, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the nodes providing the service. This endpoint is very similar to the {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogServiceNodesWithOptions} endpoint;
* however, this endpoint automatically returns the status of the associated health check as well as any system level health checks.
* @param service the service name
* @param passing if true, filter results to only nodes with all checks in the passing state
* @param options options used to request services
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxHealthServiceNodesWithOptions(java.lang.String service, boolean passing, io.vertx.ext.consul.ServiceQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthServiceNodesWithOptions(service, passing, options, resultHandler);
});
}
/**
* Returns the checks specific to the node provided on the path.
* @param node the node name or ID
* @param options options used to request node health checks
* @return a future provided with list of services
*/
public io.reactivex.rxjava3.core.Single healthNodesWithOptions(java.lang.String node, io.vertx.ext.consul.CheckQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxHealthNodesWithOptions(node, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the checks specific to the node provided on the path.
* @param node the node name or ID
* @param options options used to request node health checks
* @return a future provided with list of services
*/
public io.reactivex.rxjava3.core.Single rxHealthNodesWithOptions(java.lang.String node, io.vertx.ext.consul.CheckQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.healthNodesWithOptions(node, options, resultHandler);
});
}
/**
* Returns the services registered in a datacenter
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogServices() {
io.reactivex.rxjava3.core.Single ret = rxCatalogServices();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the services registered in a datacenter
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogServices() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogServices(resultHandler);
});
}
/**
* Returns the services registered in a datacenter
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogServices}
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogServicesWithOptions(io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCatalogServicesWithOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the services registered in a datacenter
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogServices}
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogServicesWithOptions(io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogServicesWithOptions(options, resultHandler);
});
}
/**
* Returns the node's registered services
* @param node node name
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogNodeServices(java.lang.String node) {
io.reactivex.rxjava3.core.Single ret = rxCatalogNodeServices(node);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the node's registered services
* @param node node name
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogNodeServices(java.lang.String node) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogNodeServices(node, resultHandler);
});
}
/**
* Returns the node's registered services
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogNodeServices}
* @param node node name
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single catalogNodeServicesWithOptions(java.lang.String node, io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCatalogNodeServicesWithOptions(node, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the node's registered services
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#catalogNodeServices}
* @param node node name
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCatalogNodeServicesWithOptions(java.lang.String node, io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.catalogNodeServicesWithOptions(node, options, resultHandler);
});
}
/**
* Returns list of services registered with the local agent.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> localServices() {
io.reactivex.rxjava3.core.Single> ret = rxLocalServices();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns list of services registered with the local agent.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxLocalServices() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.localServices(resultHandler);
});
}
/**
* Return all the checks that are registered with the local agent.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> localChecks() {
io.reactivex.rxjava3.core.Single> ret = rxLocalChecks();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Return all the checks that are registered with the local agent.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxLocalChecks() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.localChecks(resultHandler);
});
}
/**
* Add a new check to the local agent. The agent is responsible for managing the status of the check
* and keeping the Catalog in sync.
* @param checkOptions options used to register new check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable registerCheck(io.vertx.ext.consul.CheckOptions checkOptions) {
io.reactivex.rxjava3.core.Completable ret = rxRegisterCheck(checkOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Add a new check to the local agent. The agent is responsible for managing the status of the check
* and keeping the Catalog in sync.
* @param checkOptions options used to register new check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxRegisterCheck(io.vertx.ext.consul.CheckOptions checkOptions) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.registerCheck(checkOptions, resultHandler);
});
}
/**
* Remove a check from the local agent. The agent will take care of deregistering the check from the Catalog.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable deregisterCheck(java.lang.String checkId) {
io.reactivex.rxjava3.core.Completable ret = rxDeregisterCheck(checkId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Remove a check from the local agent. The agent will take care of deregistering the check from the Catalog.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDeregisterCheck(java.lang.String checkId) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.deregisterCheck(checkId, resultHandler);
});
}
/**
* Set status of the check to "passing". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable passCheck(java.lang.String checkId) {
io.reactivex.rxjava3.core.Completable ret = rxPassCheck(checkId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to "passing". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxPassCheck(java.lang.String checkId) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.passCheck(checkId, resultHandler);
});
}
/**
* Set status of the check to "passing". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable passCheckWithNote(java.lang.String checkId, java.lang.String note) {
io.reactivex.rxjava3.core.Completable ret = rxPassCheckWithNote(checkId, note);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to "passing". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxPassCheckWithNote(java.lang.String checkId, java.lang.String note) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.passCheckWithNote(checkId, note, resultHandler);
});
}
/**
* Set status of the check to "warning". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable warnCheck(java.lang.String checkId) {
io.reactivex.rxjava3.core.Completable ret = rxWarnCheck(checkId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to "warning". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxWarnCheck(java.lang.String checkId) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.warnCheck(checkId, resultHandler);
});
}
/**
* Set status of the check to "warning". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable warnCheckWithNote(java.lang.String checkId, java.lang.String note) {
io.reactivex.rxjava3.core.Completable ret = rxWarnCheckWithNote(checkId, note);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to "warning". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxWarnCheckWithNote(java.lang.String checkId, java.lang.String note) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.warnCheckWithNote(checkId, note, resultHandler);
});
}
/**
* Set status of the check to "critical". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable failCheck(java.lang.String checkId) {
io.reactivex.rxjava3.core.Completable ret = rxFailCheck(checkId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to "critical". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxFailCheck(java.lang.String checkId) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.failCheck(checkId, resultHandler);
});
}
/**
* Set status of the check to "critical". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable failCheckWithNote(java.lang.String checkId, java.lang.String note) {
io.reactivex.rxjava3.core.Completable ret = rxFailCheckWithNote(checkId, note);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to "critical". Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxFailCheckWithNote(java.lang.String checkId, java.lang.String note) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.failCheckWithNote(checkId, note, resultHandler);
});
}
/**
* Set status of the check to given status. Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param status new status of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable updateCheck(java.lang.String checkId, io.vertx.ext.consul.CheckStatus status) {
io.reactivex.rxjava3.core.Completable ret = rxUpdateCheck(checkId, status);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to given status. Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param status new status of check
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxUpdateCheck(java.lang.String checkId, io.vertx.ext.consul.CheckStatus status) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.updateCheck(checkId, status, resultHandler);
});
}
/**
* Set status of the check to given status. Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param status new status of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable updateCheckWithNote(java.lang.String checkId, io.vertx.ext.consul.CheckStatus status, java.lang.String note) {
io.reactivex.rxjava3.core.Completable ret = rxUpdateCheckWithNote(checkId, status, note);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set status of the check to given status. Used with a check that is of the TTL type. The TTL clock will be reset.
* @param checkId the ID of check
* @param status new status of check
* @param note specifies a human-readable message. This will be passed through to the check's Output
field.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxUpdateCheckWithNote(java.lang.String checkId, io.vertx.ext.consul.CheckStatus status, java.lang.String note) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.updateCheckWithNote(checkId, status, note, resultHandler);
});
}
/**
* Get the Raft leader for the datacenter in which the agent is running.
* It returns an address in format "10.1.10.12:8300
"
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single leaderStatus() {
io.reactivex.rxjava3.core.Single ret = rxLeaderStatus();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the Raft leader for the datacenter in which the agent is running.
* It returns an address in format "10.1.10.12:8300
"
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxLeaderStatus() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.leaderStatus(resultHandler);
});
}
/**
* Retrieves the Raft peers for the datacenter in which the the agent is running.
* It returns a list of addresses "10.1.10.12:8300
", "10.1.10.13:8300
"
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> peersStatus() {
io.reactivex.rxjava3.core.Single> ret = rxPeersStatus();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Retrieves the Raft peers for the datacenter in which the the agent is running.
* It returns a list of addresses "10.1.10.12:8300
", "10.1.10.13:8300
"
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxPeersStatus() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.peersStatus(resultHandler);
});
}
/**
* Initialize a new session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single createSession() {
io.reactivex.rxjava3.core.Single ret = rxCreateSession();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Initialize a new session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCreateSession() {
return AsyncResultSingle.toSingle( idHandler -> {
delegate.createSession(idHandler);
});
}
/**
* Initialize a new session
* @param options options used to create session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single createSessionWithOptions(io.vertx.ext.consul.SessionOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCreateSessionWithOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Initialize a new session
* @param options options used to create session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCreateSessionWithOptions(io.vertx.ext.consul.SessionOptions options) {
return AsyncResultSingle.toSingle( idHandler -> {
delegate.createSessionWithOptions(options, idHandler);
});
}
/**
* Returns the requested session information
* @param id the ID of requested session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single infoSession(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxInfoSession(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the requested session information
* @param id the ID of requested session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxInfoSession(java.lang.String id) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.infoSession(id, resultHandler);
});
}
/**
* Returns the requested session information
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#infoSession}
* @param id the ID of requested session
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single infoSessionWithOptions(java.lang.String id, io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxInfoSessionWithOptions(id, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the requested session information
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#infoSession}
* @param id the ID of requested session
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxInfoSessionWithOptions(java.lang.String id, io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.infoSessionWithOptions(id, options, resultHandler);
});
}
/**
* Renews the given session. This is used with sessions that have a TTL, and it extends the expiration by the TTL
* @param id the ID of session that should be renewed
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single renewSession(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxRenewSession(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Renews the given session. This is used with sessions that have a TTL, and it extends the expiration by the TTL
* @param id the ID of session that should be renewed
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxRenewSession(java.lang.String id) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.renewSession(id, resultHandler);
});
}
/**
* Returns the active sessions
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single listSessions() {
io.reactivex.rxjava3.core.Single ret = rxListSessions();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the active sessions
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxListSessions() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listSessions(resultHandler);
});
}
/**
* Returns the active sessions
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#listSessions}
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single listSessionsWithOptions(io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxListSessionsWithOptions(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the active sessions
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#listSessions}
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxListSessionsWithOptions(io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listSessionsWithOptions(options, resultHandler);
});
}
/**
* Returns the active sessions for a given node
* @param nodeId the ID of node
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single listNodeSessions(java.lang.String nodeId) {
io.reactivex.rxjava3.core.Single ret = rxListNodeSessions(nodeId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the active sessions for a given node
* @param nodeId the ID of node
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxListNodeSessions(java.lang.String nodeId) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listNodeSessions(nodeId, resultHandler);
});
}
/**
* Returns the active sessions for a given node
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#listNodeSessions}
* @param nodeId the ID of node
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single listNodeSessionsWithOptions(java.lang.String nodeId, io.vertx.ext.consul.BlockingQueryOptions options) {
io.reactivex.rxjava3.core.Single ret = rxListNodeSessionsWithOptions(nodeId, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the active sessions for a given node
* This is blocking query unlike {@link io.vertx.rxjava3.ext.consul.ConsulClient#listNodeSessions}
* @param nodeId the ID of node
* @param options the blocking options
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxListNodeSessionsWithOptions(java.lang.String nodeId, io.vertx.ext.consul.BlockingQueryOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listNodeSessionsWithOptions(nodeId, options, resultHandler);
});
}
/**
* Destroys the given session
* @param id the ID of session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable destroySession(java.lang.String id) {
io.reactivex.rxjava3.core.Completable ret = rxDestroySession(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Destroys the given session
* @param id the ID of session
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDestroySession(java.lang.String id) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.destroySession(id, resultHandler);
});
}
/**
* @param definition definition of the prepare query
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single createPreparedQuery(io.vertx.ext.consul.PreparedQueryDefinition definition) {
io.reactivex.rxjava3.core.Single ret = rxCreatePreparedQuery(definition);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* @param definition definition of the prepare query
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxCreatePreparedQuery(io.vertx.ext.consul.PreparedQueryDefinition definition) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.createPreparedQuery(definition, resultHandler);
});
}
/**
* Returns an existing prepared query
* @param id the id of the query to read
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single getPreparedQuery(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxGetPreparedQuery(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns an existing prepared query
* @param id the id of the query to read
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxGetPreparedQuery(java.lang.String id) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getPreparedQuery(id, resultHandler);
});
}
/**
* Returns a list of all prepared queries.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> getAllPreparedQueries() {
io.reactivex.rxjava3.core.Single> ret = rxGetAllPreparedQueries();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns a list of all prepared queries.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single> rxGetAllPreparedQueries() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getAllPreparedQueries(resultHandler);
});
}
/**
* @param definition definition of the prepare query
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable updatePreparedQuery(io.vertx.ext.consul.PreparedQueryDefinition definition) {
io.reactivex.rxjava3.core.Completable ret = rxUpdatePreparedQuery(definition);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* @param definition definition of the prepare query
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxUpdatePreparedQuery(io.vertx.ext.consul.PreparedQueryDefinition definition) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.updatePreparedQuery(definition, resultHandler);
});
}
/**
* Deletes an existing prepared query
* @param id the id of the query to delete
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable deletePreparedQuery(java.lang.String id) {
io.reactivex.rxjava3.core.Completable ret = rxDeletePreparedQuery(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Deletes an existing prepared query
* @param id the id of the query to delete
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDeletePreparedQuery(java.lang.String id) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.deletePreparedQuery(id, resultHandler);
});
}
/**
* Executes an existing prepared query.
* @param query the ID of the query to execute. This can also be the name of an existing prepared query, or a name that matches a prefix name for a prepared query template.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single executePreparedQuery(java.lang.String query) {
io.reactivex.rxjava3.core.Single ret = rxExecutePreparedQuery(query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Executes an existing prepared query.
* @param query the ID of the query to execute. This can also be the name of an existing prepared query, or a name that matches a prefix name for a prepared query template.
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxExecutePreparedQuery(java.lang.String query) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.executePreparedQuery(query, resultHandler);
});
}
/**
* Executes an existing prepared query.
* @param query the ID of the query to execute. This can also be the name of an existing prepared query, or a name that matches a prefix name for a prepared query template.
* @param options the options used to execute prepared query
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single executePreparedQueryWithOptions(java.lang.String query, io.vertx.ext.consul.PreparedQueryExecuteOptions options) {
io.reactivex.rxjava3.core.Single ret = rxExecutePreparedQueryWithOptions(query, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Executes an existing prepared query.
* @param query the ID of the query to execute. This can also be the name of an existing prepared query, or a name that matches a prefix name for a prepared query template.
* @param options the options used to execute prepared query
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Single rxExecutePreparedQueryWithOptions(java.lang.String query, io.vertx.ext.consul.PreparedQueryExecuteOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.executePreparedQueryWithOptions(query, options, resultHandler);
});
}
/**
* Register node with external service
* @param nodeOptions the options of new node
* @param serviceOptions the options of new service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable registerCatalogService(io.vertx.ext.consul.Node nodeOptions, io.vertx.ext.consul.ServiceOptions serviceOptions) {
io.reactivex.rxjava3.core.Completable ret = rxRegisterCatalogService(nodeOptions, serviceOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Register node with external service
* @param nodeOptions the options of new node
* @param serviceOptions the options of new service
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxRegisterCatalogService(io.vertx.ext.consul.Node nodeOptions, io.vertx.ext.consul.ServiceOptions serviceOptions) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.registerCatalogService(nodeOptions, serviceOptions, resultHandler);
});
}
/**
* Deregister entities from the node or deregister the node itself.
* @param nodeId the ID of node
* @param serviceId the ID of the service to de-registered; if it is null, the node itself will be de-registered (as well as the entities that belongs to that node)
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable deregisterCatalogService(java.lang.String nodeId, java.lang.String serviceId) {
io.reactivex.rxjava3.core.Completable ret = rxDeregisterCatalogService(nodeId, serviceId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Deregister entities from the node or deregister the node itself.
* @param nodeId the ID of node
* @param serviceId the ID of the service to de-registered; if it is null, the node itself will be de-registered (as well as the entities that belongs to that node)
* @return reference to this, for fluency
*/
public io.reactivex.rxjava3.core.Completable rxDeregisterCatalogService(java.lang.String nodeId, java.lang.String serviceId) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.deregisterCatalogService(nodeId, serviceId, resultHandler);
});
}
/**
* Close the client and release its resources
*/
public void close() {
delegate.close();
}
public static ConsulClient newInstance(io.vertx.ext.consul.ConsulClient arg) {
return arg != null ? new ConsulClient(arg) : null;
}
}