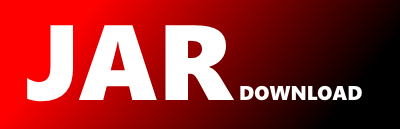
io.vertx.rxjava3.ext.mongo.MongoClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.mongo;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A Vert.x service used to interact with MongoDB server instances.
*
* Some of the operations might change _id field of passed document.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.mongo.MongoClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.mongo.MongoClient.class)
public class MongoClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MongoClient that = (MongoClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new MongoClient((io.vertx.ext.mongo.MongoClient) obj),
MongoClient::getDelegate
);
private final io.vertx.ext.mongo.MongoClient delegate;
public MongoClient(io.vertx.ext.mongo.MongoClient delegate) {
this.delegate = delegate;
}
public MongoClient(Object delegate) {
this.delegate = (io.vertx.ext.mongo.MongoClient)delegate;
}
public io.vertx.ext.mongo.MongoClient getDelegate() {
return delegate;
}
/**
* Create a Mongo client which maintains its own data source.
* @param vertx the Vert.x instance
* @param config the configuration
* @return the client
*/
public static io.vertx.rxjava3.ext.mongo.MongoClient create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.core.json.JsonObject config) {
io.vertx.rxjava3.ext.mongo.MongoClient ret = io.vertx.rxjava3.ext.mongo.MongoClient.newInstance((io.vertx.ext.mongo.MongoClient)io.vertx.ext.mongo.MongoClient.create(vertx.getDelegate(), config));
return ret;
}
/**
* Create a Mongo client which shares its data source with any other Mongo clients created with the same
* data source name
* @param vertx the Vert.x instance
* @param config the configuration
* @param dataSourceName the data source name
* @return the client
*/
public static io.vertx.rxjava3.ext.mongo.MongoClient createShared(io.vertx.rxjava3.core.Vertx vertx, io.vertx.core.json.JsonObject config, java.lang.String dataSourceName) {
io.vertx.rxjava3.ext.mongo.MongoClient ret = io.vertx.rxjava3.ext.mongo.MongoClient.newInstance((io.vertx.ext.mongo.MongoClient)io.vertx.ext.mongo.MongoClient.createShared(vertx.getDelegate(), config, dataSourceName));
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.mongo.MongoClient#createShared} but with the default data source name
* @param vertx the Vert.x instance
* @param config the configuration
* @return the client
*/
public static io.vertx.rxjava3.ext.mongo.MongoClient createShared(io.vertx.rxjava3.core.Vertx vertx, io.vertx.core.json.JsonObject config) {
io.vertx.rxjava3.ext.mongo.MongoClient ret = io.vertx.rxjava3.ext.mongo.MongoClient.newInstance((io.vertx.ext.mongo.MongoClient)io.vertx.ext.mongo.MongoClient.createShared(vertx.getDelegate(), config));
return ret;
}
/**
* Save a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
*/
public io.reactivex.rxjava3.core.Maybe save(java.lang.String collection, io.vertx.core.json.JsonObject document) {
io.reactivex.rxjava3.core.Maybe ret = rxSave(collection, document);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Save a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxSave(java.lang.String collection, io.vertx.core.json.JsonObject document) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.save(collection, document, resultHandler);
});
}
/**
* Save a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe saveWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
io.reactivex.rxjava3.core.Maybe ret = rxSaveWithOptions(collection, document, writeOption);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Save a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxSaveWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.saveWithOptions(collection, document, writeOption, resultHandler);
});
}
/**
* Insert a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
*/
public io.reactivex.rxjava3.core.Maybe insert(java.lang.String collection, io.vertx.core.json.JsonObject document) {
io.reactivex.rxjava3.core.Maybe ret = rxInsert(collection, document);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Insert a document in the specified collection
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxInsert(java.lang.String collection, io.vertx.core.json.JsonObject document) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.insert(collection, document, resultHandler);
});
}
/**
* Insert a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe insertWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
io.reactivex.rxjava3.core.Maybe ret = rxInsertWithOptions(collection, document, writeOption);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Insert a document in the specified collection with the specified write option
*
* This operation might change _id field of document parameter
* @param collection the collection
* @param document the document
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxInsertWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject document, io.vertx.ext.mongo.WriteOption writeOption) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.insertWithOptions(collection, document, writeOption, resultHandler);
});
}
/**
* Update matching documents in the specified collection and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
*/
public io.reactivex.rxjava3.core.Maybe updateCollection(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update) {
io.reactivex.rxjava3.core.Maybe ret = rxUpdateCollection(collection, query, update);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Update matching documents in the specified collection and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxUpdateCollection(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.updateCollection(collection, query, update, resultHandler);
});
}
/**
* Use an aggregation pipeline to update documents in the specified collection and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
*/
public io.reactivex.rxjava3.core.Maybe updateCollection(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonArray update) {
io.reactivex.rxjava3.core.Maybe ret = rxUpdateCollection(collection, query, update);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Use an aggregation pipeline to update documents in the specified collection and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxUpdateCollection(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonArray update) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.updateCollection(collection, query, update, resultHandler);
});
}
/**
* Update matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe updateCollectionWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update, io.vertx.ext.mongo.UpdateOptions options) {
io.reactivex.rxjava3.core.Maybe ret = rxUpdateCollectionWithOptions(collection, query, update, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Update matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update used to describe how the documents will be updated
* @param options options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxUpdateCollectionWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update, io.vertx.ext.mongo.UpdateOptions options) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.updateCollectionWithOptions(collection, query, update, options, resultHandler);
});
}
/**
* Use an aggregation pipeline to update documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update aggregation pipeline used to describe how documents will be updated
* @param options options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe updateCollectionWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonArray update, io.vertx.ext.mongo.UpdateOptions options) {
io.reactivex.rxjava3.core.Maybe ret = rxUpdateCollectionWithOptions(collection, query, update, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Use an aggregation pipeline to update documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param update aggregation pipeline used to describe how documents will be updated
* @param options options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxUpdateCollectionWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonArray update, io.vertx.ext.mongo.UpdateOptions options) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.updateCollectionWithOptions(collection, query, update, options, resultHandler);
});
}
/**
* Replace matching documents in the specified collection and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @return
*/
public io.reactivex.rxjava3.core.Maybe replaceDocuments(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace) {
io.reactivex.rxjava3.core.Maybe ret = rxReplaceDocuments(collection, query, replace);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Replace matching documents in the specified collection and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxReplaceDocuments(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.replaceDocuments(collection, query, replace, resultHandler);
});
}
/**
* Replace matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @return
*/
public io.reactivex.rxjava3.core.Maybe replaceDocumentsWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace, io.vertx.ext.mongo.UpdateOptions options) {
io.reactivex.rxjava3.core.Maybe ret = rxReplaceDocumentsWithOptions(collection, query, replace, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Replace matching documents in the specified collection, specifying options and return the handler with MongoClientUpdateResult
result
* @param collection the collection
* @param query query used to match the documents
* @param replace all matching documents will be replaced with this
* @param options options to configure the replace
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxReplaceDocumentsWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace, io.vertx.ext.mongo.UpdateOptions options) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.replaceDocumentsWithOptions(collection, query, replace, options, resultHandler);
});
}
/**
* Execute a bulk operation. Can insert, update, replace, and/or delete multiple documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @return
*/
public io.reactivex.rxjava3.core.Maybe bulkWrite(java.lang.String collection, java.util.List operations) {
io.reactivex.rxjava3.core.Maybe ret = rxBulkWrite(collection, operations);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Execute a bulk operation. Can insert, update, replace, and/or delete multiple documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxBulkWrite(java.lang.String collection, java.util.List operations) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.bulkWrite(collection, operations, resultHandler);
});
}
/**
* Execute a bulk operation with the specified write options. Can insert, update, replace, and/or delete multiple
* documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @param bulkWriteOptions the write options
* @return
*/
public io.reactivex.rxjava3.core.Maybe bulkWriteWithOptions(java.lang.String collection, java.util.List operations, io.vertx.ext.mongo.BulkWriteOptions bulkWriteOptions) {
io.reactivex.rxjava3.core.Maybe ret = rxBulkWriteWithOptions(collection, operations, bulkWriteOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Execute a bulk operation with the specified write options. Can insert, update, replace, and/or delete multiple
* documents with one request.
* @param collection the collection
* @param operations the operations to execute
* @param bulkWriteOptions the write options
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxBulkWriteWithOptions(java.lang.String collection, java.util.List operations, io.vertx.ext.mongo.BulkWriteOptions bulkWriteOptions) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.bulkWriteWithOptions(collection, operations, bulkWriteOptions, resultHandler);
});
}
/**
* Find matching documents in the specified collection
* @param collection the collection
* @param query query used to match documents
* @return
*/
public io.reactivex.rxjava3.core.Single> find(java.lang.String collection, io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Single> ret = rxFind(collection, query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Find matching documents in the specified collection
* @param collection the collection
* @param query query used to match documents
* @return
*/
public io.reactivex.rxjava3.core.Single> rxFind(java.lang.String collection, io.vertx.core.json.JsonObject query) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.find(collection, query, resultHandler);
});
}
/**
* Find matching documents in the specified collection.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @return a emitting found documents
*/
public io.vertx.rxjava3.core.streams.ReadStream findBatch(java.lang.String collection, io.vertx.core.json.JsonObject query) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.findBatch(collection, query), TypeArg.unknown());
return ret;
}
/**
* Find matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return
*/
public io.reactivex.rxjava3.core.Single> findWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.FindOptions options) {
io.reactivex.rxjava3.core.Single> ret = rxFindWithOptions(collection, query, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Find matching documents in the specified collection, specifying options
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return
*/
public io.reactivex.rxjava3.core.Single> rxFindWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.FindOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.findWithOptions(collection, query, options, resultHandler);
});
}
/**
* Find matching documents in the specified collection, specifying options.
* This method use batchCursor for returning each found document.
* @param collection the collection
* @param query query used to match documents
* @param options options to configure the find
* @return a emitting found documents
*/
public io.vertx.rxjava3.core.streams.ReadStream findBatchWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.FindOptions options) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.findBatchWithOptions(collection, query, options), TypeArg.unknown());
return ret;
}
/**
* Find a single matching document in the specified collection
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param fields the fields
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOne(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject fields) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOne(collection, query, fields);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param fields the fields
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOne(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject fields) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOne(collection, query, fields, resultHandler);
});
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOneAndUpdate(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOneAndUpdate(collection, query, update);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOneAndUpdate(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOneAndUpdate(collection, query, update, resultHandler);
});
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOneAndUpdateWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection and update it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param update used to describe how the documents will be updated
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOneAndUpdateWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject update, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOneAndUpdateWithOptions(collection, query, update, findOptions, updateOptions, resultHandler);
});
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOneAndReplace(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOneAndReplace(collection, query, replace);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOneAndReplace(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOneAndReplace(collection, query, replace, resultHandler);
});
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOneAndReplaceWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOneAndReplaceWithOptions(collection, query, replace, findOptions, updateOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection and replace it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param replace the replacement document
* @param findOptions options to configure the find
* @param updateOptions options to configure the update
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOneAndReplaceWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.core.json.JsonObject replace, io.vertx.ext.mongo.FindOptions findOptions, io.vertx.ext.mongo.UpdateOptions updateOptions) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOneAndReplaceWithOptions(collection, query, replace, findOptions, updateOptions, resultHandler);
});
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOneAndDelete(java.lang.String collection, io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOneAndDelete(collection, query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOneAndDelete(java.lang.String collection, io.vertx.core.json.JsonObject query) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOneAndDelete(collection, query, resultHandler);
});
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param findOptions options to configure the find
* @return
*/
public io.reactivex.rxjava3.core.Maybe findOneAndDeleteWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.FindOptions findOptions) {
io.reactivex.rxjava3.core.Maybe ret = rxFindOneAndDeleteWithOptions(collection, query, findOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Find a single matching document in the specified collection and delete it.
*
* This operation might change _id field of query parameter
* @param collection the collection
* @param query the query used to match the document
* @param findOptions options to configure the find
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxFindOneAndDeleteWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.FindOptions findOptions) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.findOneAndDeleteWithOptions(collection, query, findOptions, resultHandler);
});
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @return
*/
public io.reactivex.rxjava3.core.Single count(java.lang.String collection, io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Single ret = rxCount(collection, query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @return
*/
public io.reactivex.rxjava3.core.Single rxCount(java.lang.String collection, io.vertx.core.json.JsonObject query) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.count(collection, query, resultHandler);
});
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @param countOptions
* @return
*/
public io.reactivex.rxjava3.core.Single countWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.CountOptions countOptions) {
io.reactivex.rxjava3.core.Single ret = rxCountWithOptions(collection, query, countOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Count matching documents in a collection.
* @param collection the collection
* @param query query used to match documents
* @param countOptions
* @return
*/
public io.reactivex.rxjava3.core.Single rxCountWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.CountOptions countOptions) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.countWithOptions(collection, query, countOptions, resultHandler);
});
}
/**
* Remove matching documents from a collection and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match documents
* @return
*/
public io.reactivex.rxjava3.core.Maybe removeDocuments(java.lang.String collection, io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Maybe ret = rxRemoveDocuments(collection, query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Remove matching documents from a collection and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match documents
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxRemoveDocuments(java.lang.String collection, io.vertx.core.json.JsonObject query) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.removeDocuments(collection, query, resultHandler);
});
}
/**
* Remove matching documents from a collection with the specified write option and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe removeDocumentsWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
io.reactivex.rxjava3.core.Maybe ret = rxRemoveDocumentsWithOptions(collection, query, writeOption);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Remove matching documents from a collection with the specified write option and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match documents
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxRemoveDocumentsWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.removeDocumentsWithOptions(collection, query, writeOption, resultHandler);
});
}
/**
* Remove a single matching document from a collection and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match document
* @return
*/
public io.reactivex.rxjava3.core.Maybe removeDocument(java.lang.String collection, io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Maybe ret = rxRemoveDocument(collection, query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Remove a single matching document from a collection and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match document
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxRemoveDocument(java.lang.String collection, io.vertx.core.json.JsonObject query) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.removeDocument(collection, query, resultHandler);
});
}
/**
* Remove a single matching document from a collection with the specified write option and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe removeDocumentWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
io.reactivex.rxjava3.core.Maybe ret = rxRemoveDocumentWithOptions(collection, query, writeOption);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Remove a single matching document from a collection with the specified write option and return the handler with MongoClientDeleteResult
result
* @param collection the collection
* @param query query used to match document
* @param writeOption the write option to use
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxRemoveDocumentWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.WriteOption writeOption) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.removeDocumentWithOptions(collection, query, writeOption, resultHandler);
});
}
/**
* Create a new collection
* @param collectionName the name of the collection
* @return
*/
public io.reactivex.rxjava3.core.Completable createCollection(java.lang.String collectionName) {
io.reactivex.rxjava3.core.Completable ret = rxCreateCollection(collectionName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create a new collection
* @param collectionName the name of the collection
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreateCollection(java.lang.String collectionName) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.createCollection(collectionName, resultHandler);
});
}
/**
* Create a new collection with options
* @param collectionName the name of the collection
* @param collectionOptions options of the collection
* @return
*/
public io.reactivex.rxjava3.core.Completable createCollectionWithOptions(java.lang.String collectionName, io.vertx.ext.mongo.CreateCollectionOptions collectionOptions) {
io.reactivex.rxjava3.core.Completable ret = rxCreateCollectionWithOptions(collectionName, collectionOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Create a new collection with options
* @param collectionName the name of the collection
* @param collectionOptions options of the collection
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreateCollectionWithOptions(java.lang.String collectionName, io.vertx.ext.mongo.CreateCollectionOptions collectionOptions) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.createCollectionWithOptions(collectionName, collectionOptions, resultHandler);
});
}
/**
* Get a list of all collections in the database.
* @return
*/
public io.reactivex.rxjava3.core.Single> getCollections() {
io.reactivex.rxjava3.core.Single> ret = rxGetCollections();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get a list of all collections in the database.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetCollections() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getCollections(resultHandler);
});
}
/**
* Drop a collection
* @param collection the collection
* @return
*/
public io.reactivex.rxjava3.core.Completable dropCollection(java.lang.String collection) {
io.reactivex.rxjava3.core.Completable ret = rxDropCollection(collection);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Drop a collection
* @param collection the collection
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDropCollection(java.lang.String collection) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.dropCollection(collection, resultHandler);
});
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @return
*/
public io.reactivex.rxjava3.core.Completable createIndex(java.lang.String collection, io.vertx.core.json.JsonObject key) {
io.reactivex.rxjava3.core.Completable ret = rxCreateIndex(collection, key);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreateIndex(java.lang.String collection, io.vertx.core.json.JsonObject key) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.createIndex(collection, key, resultHandler);
});
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @param options the options for the index
* @return
*/
public io.reactivex.rxjava3.core.Completable createIndexWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject key, io.vertx.ext.mongo.IndexOptions options) {
io.reactivex.rxjava3.core.Completable ret = rxCreateIndexWithOptions(collection, key, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates an index.
* @param collection the collection
* @param key A document that contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @param options the options for the index
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreateIndexWithOptions(java.lang.String collection, io.vertx.core.json.JsonObject key, io.vertx.ext.mongo.IndexOptions options) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.createIndexWithOptions(collection, key, options, resultHandler);
});
}
/**
* creates an indexes
* @param collection the collection
* @param indexes A model that contains pairs of document and indexOptions, document contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @return
*/
public io.reactivex.rxjava3.core.Completable createIndexes(java.lang.String collection, java.util.List indexes) {
io.reactivex.rxjava3.core.Completable ret = rxCreateIndexes(collection, indexes);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* creates an indexes
* @param collection the collection
* @param indexes A model that contains pairs of document and indexOptions, document contains the field and value pairs where the field is the index key and the value describes the type of index for that field. For an ascending index on a field, specify a value of 1; for descending index, specify a value of -1.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreateIndexes(java.lang.String collection, java.util.List indexes) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.createIndexes(collection, indexes, resultHandler);
});
}
/**
* Get all the indexes in this collection.
* @param collection the collection
* @return
*/
public io.reactivex.rxjava3.core.Single listIndexes(java.lang.String collection) {
io.reactivex.rxjava3.core.Single ret = rxListIndexes(collection);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get all the indexes in this collection.
* @param collection the collection
* @return
*/
public io.reactivex.rxjava3.core.Single rxListIndexes(java.lang.String collection) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.listIndexes(collection, resultHandler);
});
}
/**
* Drops the index given its name.
* @param collection the collection
* @param indexName the name of the index to remove
* @return
*/
public io.reactivex.rxjava3.core.Completable dropIndex(java.lang.String collection, java.lang.String indexName) {
io.reactivex.rxjava3.core.Completable ret = rxDropIndex(collection, indexName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Drops the index given its name.
* @param collection the collection
* @param indexName the name of the index to remove
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDropIndex(java.lang.String collection, java.lang.String indexName) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.dropIndex(collection, indexName, resultHandler);
});
}
/**
* Run an arbitrary MongoDB command.
* @param commandName the name of the command
* @param command the command
* @return
*/
public io.reactivex.rxjava3.core.Maybe runCommand(java.lang.String commandName, io.vertx.core.json.JsonObject command) {
io.reactivex.rxjava3.core.Maybe ret = rxRunCommand(commandName, command);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Run an arbitrary MongoDB command.
* @param commandName the name of the command
* @param command the command
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxRunCommand(java.lang.String commandName, io.vertx.core.json.JsonObject command) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.runCommand(commandName, command, resultHandler);
});
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return
*/
public io.reactivex.rxjava3.core.Single distinct(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname) {
io.reactivex.rxjava3.core.Single ret = rxDistinct(collection, fieldName, resultClassname);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return
*/
public io.reactivex.rxjava3.core.Single rxDistinct(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.distinct(collection, fieldName, resultClassname, resultHandler);
});
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param distinctOptions options (e.g. collation)
* @return
*/
public io.reactivex.rxjava3.core.Single distinct(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
io.reactivex.rxjava3.core.Single ret = rxDistinct(collection, fieldName, resultClassname, distinctOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Gets the distinct values of the specified field name.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param distinctOptions options (e.g. collation)
* @return
*/
public io.reactivex.rxjava3.core.Single rxDistinct(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.distinct(collection, fieldName, resultClassname, distinctOptions, resultHandler);
});
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @return
*/
public io.reactivex.rxjava3.core.Single distinctWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Single ret = rxDistinctWithQuery(collection, fieldName, resultClassname, query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @return
*/
public io.reactivex.rxjava3.core.Single rxDistinctWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.distinctWithQuery(collection, fieldName, resultClassname, query, resultHandler);
});
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @param distinctOptions options (e.g. collation)
* @return
*/
public io.reactivex.rxjava3.core.Single distinctWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
io.reactivex.rxjava3.core.Single ret = rxDistinctWithQuery(collection, fieldName, resultClassname, query, distinctOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* Return a JsonArray containing distinct values (eg: [ 1 , 89 ])
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @param distinctOptions options (e.g. collation)
* @return
*/
public io.reactivex.rxjava3.core.Single rxDistinctWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.distinctWithQuery(collection, fieldName, resultClassname, query, distinctOptions, resultHandler);
});
}
/**
* Gets the distinct values of the specified field name.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @return a emitting json fragments
*/
public io.vertx.rxjava3.core.streams.ReadStream distinctBatch(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.distinctBatch(collection, fieldName, resultClassname), TypeArg.unknown());
return ret;
}
/**
* Gets the distinct values of the specified field name.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param distinctOptions options (e.g. collation)
* @return a emitting json fragments
*/
public io.vertx.rxjava3.core.streams.ReadStream distinctBatch(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.distinctBatch(collection, fieldName, resultClassname, distinctOptions), TypeArg.unknown());
return ret;
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @return a emitting json fragments
*/
public io.vertx.rxjava3.core.streams.ReadStream distinctBatchWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.distinctBatchWithQuery(collection, fieldName, resultClassname, query), TypeArg.unknown());
return ret;
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @param distinctOptions options (e.g. collation)
* @return a emitting json fragments
*/
public io.vertx.rxjava3.core.streams.ReadStream distinctBatchWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.distinctBatchWithQuery(collection, fieldName, resultClassname, query, distinctOptions), TypeArg.unknown());
return ret;
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @param batchSize the number of documents to load in a batch
* @return a emitting json fragments
*/
public io.vertx.rxjava3.core.streams.ReadStream distinctBatchWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query, int batchSize) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.distinctBatchWithQuery(collection, fieldName, resultClassname, query, batchSize), TypeArg.unknown());
return ret;
}
/**
* Gets the distinct values of the specified field name filtered by specified query.
* This method use batchCursor for returning each found value.
* Each value is a json fragment with fieldName key (eg: {"num": 1}).
* @param collection the collection
* @param fieldName the field name
* @param resultClassname
* @param query the query
* @param batchSize the number of documents to load in a batch
* @param distinctOptions options (e.g. collation)
* @return a emitting json fragments
*/
public io.vertx.rxjava3.core.streams.ReadStream distinctBatchWithQuery(java.lang.String collection, java.lang.String fieldName, java.lang.String resultClassname, io.vertx.core.json.JsonObject query, int batchSize, io.vertx.ext.mongo.DistinctOptions distinctOptions) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.distinctBatchWithQuery(collection, fieldName, resultClassname, query, batchSize, distinctOptions), TypeArg.unknown());
return ret;
}
/**
* Run aggregate MongoDB command with default {@link io.vertx.ext.mongo.AggregateOptions}.
* @param collection the collection
* @param pipeline aggregation pipeline to be executed
* @return
*/
public io.vertx.rxjava3.core.streams.ReadStream aggregate(java.lang.String collection, io.vertx.core.json.JsonArray pipeline) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.aggregate(collection, pipeline), TypeArg.unknown());
return ret;
}
/**
* Run aggregate MongoDB command.
* @param collection the collection
* @param pipeline aggregation pipeline to be executed
* @param options options to configure the aggregation command
* @return
*/
public io.vertx.rxjava3.core.streams.ReadStream aggregateWithOptions(java.lang.String collection, io.vertx.core.json.JsonArray pipeline, io.vertx.ext.mongo.AggregateOptions options) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.aggregateWithOptions(collection, pipeline, options), TypeArg.unknown());
return ret;
}
/**
* Creates a {@link io.vertx.rxjava3.ext.mongo.MongoGridFsClient} used to interact with Mongo GridFS.
* @return
*/
public io.reactivex.rxjava3.core.Single createDefaultGridFsBucketService() {
io.reactivex.rxjava3.core.Single ret = rxCreateDefaultGridFsBucketService();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a {@link io.vertx.rxjava3.ext.mongo.MongoGridFsClient} used to interact with Mongo GridFS.
* @return
*/
public io.reactivex.rxjava3.core.Single rxCreateDefaultGridFsBucketService() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.createDefaultGridFsBucketService(new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.ext.mongo.MongoGridFsClient.newInstance((io.vertx.ext.mongo.MongoGridFsClient)event))));
});
}
/**
* Creates a {@link io.vertx.rxjava3.ext.mongo.MongoGridFsClient} used to interact with Mongo GridFS.
* @param bucketName the name of the GridFS bucket
* @return
*/
public io.reactivex.rxjava3.core.Single createGridFsBucketService(java.lang.String bucketName) {
io.reactivex.rxjava3.core.Single ret = rxCreateGridFsBucketService(bucketName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Creates a {@link io.vertx.rxjava3.ext.mongo.MongoGridFsClient} used to interact with Mongo GridFS.
* @param bucketName the name of the GridFS bucket
* @return
*/
public io.reactivex.rxjava3.core.Single rxCreateGridFsBucketService(java.lang.String bucketName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.createGridFsBucketService(bucketName, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.ext.mongo.MongoGridFsClient.newInstance((io.vertx.ext.mongo.MongoGridFsClient)event))));
});
}
/**
* Close the client and release its resources
* @return
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Close the client and release its resources
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.close(handler);
});
}
/**
* Watch the collection change.
* @param collection the collection
* @param pipeline watching pipeline to be executed
* @param withUpdatedDoc whether to get updated fullDocument for "update" operation
* @param batchSize the number of documents to load in a batch
* @return
*/
public io.vertx.rxjava3.core.streams.ReadStream> watch(java.lang.String collection, io.vertx.core.json.JsonArray pipeline, boolean withUpdatedDoc, int batchSize) {
io.vertx.rxjava3.core.streams.ReadStream> ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.watch(collection, pipeline, withUpdatedDoc, batchSize), TypeArg.unknown());
return ret;
}
/**
* The name of the default pool
*/
public static final java.lang.String DEFAULT_POOL_NAME = io.vertx.ext.mongo.MongoClient.DEFAULT_POOL_NAME;
/**
* The name of the default database
*/
public static final java.lang.String DEFAULT_DB_NAME = io.vertx.ext.mongo.MongoClient.DEFAULT_DB_NAME;
public static MongoClient newInstance(io.vertx.ext.mongo.MongoClient arg) {
return arg != null ? new MongoClient(arg) : null;
}
}