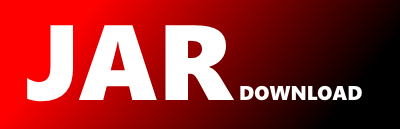
io.vertx.rxjava3.ext.mongo.MongoGridFsClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.mongo;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
@RxGen(io.vertx.ext.mongo.MongoGridFsClient.class)
public class MongoGridFsClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MongoGridFsClient that = (MongoGridFsClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new MongoGridFsClient((io.vertx.ext.mongo.MongoGridFsClient) obj),
MongoGridFsClient::getDelegate
);
private final io.vertx.ext.mongo.MongoGridFsClient delegate;
public MongoGridFsClient(io.vertx.ext.mongo.MongoGridFsClient delegate) {
this.delegate = delegate;
}
public MongoGridFsClient(Object delegate) {
this.delegate = (io.vertx.ext.mongo.MongoGridFsClient)delegate;
}
public io.vertx.ext.mongo.MongoGridFsClient getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)o1), o1 -> o1.getDelegate());
/**
* Deletes a file by it's ID
* @param id the identifier of the file
* @return
*/
public io.reactivex.rxjava3.core.Completable delete(java.lang.String id) {
io.reactivex.rxjava3.core.Completable ret = rxDelete(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Deletes a file by it's ID
* @param id the identifier of the file
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDelete(java.lang.String id) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.delete(id, resultHandler);
});
}
/**
* Read file by name to ReadStream
* @param fileName
* @return
*/
public io.vertx.rxjava3.core.streams.ReadStream readByFileName(java.lang.String fileName) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.readByFileName(fileName), TYPE_ARG_0);
return ret;
}
/**
* Read file by name to ReadStream with options
* @param fileName
* @param options
* @return
*/
public io.vertx.rxjava3.core.streams.ReadStream readByFileNameWithOptions(java.lang.String fileName, io.vertx.ext.mongo.GridFsDownloadOptions options) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.readByFileNameWithOptions(fileName, options), TYPE_ARG_1);
return ret;
}
/**
* Read file by id to ReadStream
* @param id
* @return
*/
public io.vertx.rxjava3.core.streams.ReadStream readById(java.lang.String id) {
io.vertx.rxjava3.core.streams.ReadStream ret = io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)delegate.readById(id), TYPE_ARG_2);
return ret;
}
public io.reactivex.rxjava3.core.Single downloadByFileName(io.vertx.rxjava3.core.streams.WriteStream stream, java.lang.String fileName) {
io.reactivex.rxjava3.core.Single ret = rxDownloadByFileName(stream, fileName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single rxDownloadByFileName(io.vertx.rxjava3.core.streams.WriteStream stream, java.lang.String fileName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.downloadByFileName(stream.getDelegate(), fileName, resultHandler);
});
}
public io.reactivex.rxjava3.core.Single downloadByFileNameWithOptions(io.vertx.rxjava3.core.streams.WriteStream stream, java.lang.String fileName, io.vertx.ext.mongo.GridFsDownloadOptions options) {
io.reactivex.rxjava3.core.Single ret = rxDownloadByFileNameWithOptions(stream, fileName, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single rxDownloadByFileNameWithOptions(io.vertx.rxjava3.core.streams.WriteStream stream, java.lang.String fileName, io.vertx.ext.mongo.GridFsDownloadOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.downloadByFileNameWithOptions(stream.getDelegate(), fileName, options, resultHandler);
});
}
public io.reactivex.rxjava3.core.Single downloadById(io.vertx.rxjava3.core.streams.WriteStream stream, java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxDownloadById(stream, id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single rxDownloadById(io.vertx.rxjava3.core.streams.WriteStream stream, java.lang.String id) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.downloadById(stream.getDelegate(), id, resultHandler);
});
}
/**
* Downloads a file.
* @param fileName the name of the file to download
* @return
*/
public io.reactivex.rxjava3.core.Single downloadFile(java.lang.String fileName) {
io.reactivex.rxjava3.core.Single ret = rxDownloadFile(fileName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Downloads a file.
* @param fileName the name of the file to download
* @return
*/
public io.reactivex.rxjava3.core.Single rxDownloadFile(java.lang.String fileName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.downloadFile(fileName, resultHandler);
});
}
/**
* Downloads a file and gives it a new name.
* @param fileName the name of the file to download
* @param newFileName the name the file should be saved as
* @return
*/
public io.reactivex.rxjava3.core.Single downloadFileAs(java.lang.String fileName, java.lang.String newFileName) {
io.reactivex.rxjava3.core.Single ret = rxDownloadFileAs(fileName, newFileName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Downloads a file and gives it a new name.
* @param fileName the name of the file to download
* @param newFileName the name the file should be saved as
* @return
*/
public io.reactivex.rxjava3.core.Single rxDownloadFileAs(java.lang.String fileName, java.lang.String newFileName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.downloadFileAs(fileName, newFileName, resultHandler);
});
}
/**
* Downloads a file using the ID generated by GridFs.
* @param id the GridFs Object ID of the file to download
* @param fileName
* @return
*/
public io.reactivex.rxjava3.core.Single downloadFileByID(java.lang.String id, java.lang.String fileName) {
io.reactivex.rxjava3.core.Single ret = rxDownloadFileByID(id, fileName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Downloads a file using the ID generated by GridFs.
* @param id the GridFs Object ID of the file to download
* @param fileName
* @return
*/
public io.reactivex.rxjava3.core.Single rxDownloadFileByID(java.lang.String id, java.lang.String fileName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.downloadFileByID(id, fileName, resultHandler);
});
}
/**
* Drops the entire file bucket with all of its contents
* @return
*/
public io.reactivex.rxjava3.core.Completable drop() {
io.reactivex.rxjava3.core.Completable ret = rxDrop();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Drops the entire file bucket with all of its contents
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDrop() {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.drop(resultHandler);
});
}
/**
* Finds all file ids in the bucket
* @return
*/
public io.reactivex.rxjava3.core.Single> findAllIds() {
io.reactivex.rxjava3.core.Single> ret = rxFindAllIds();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Finds all file ids in the bucket
* @return
*/
public io.reactivex.rxjava3.core.Single> rxFindAllIds() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.findAllIds(resultHandler);
});
}
/**
* Finds all file ids that match a query.
* @param query a bson query expressed as json that will be used to match files
* @return
*/
public io.reactivex.rxjava3.core.Single> findIds(io.vertx.core.json.JsonObject query) {
io.reactivex.rxjava3.core.Single> ret = rxFindIds(query);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Finds all file ids that match a query.
* @param query a bson query expressed as json that will be used to match files
* @return
*/
public io.reactivex.rxjava3.core.Single> rxFindIds(io.vertx.core.json.JsonObject query) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.findIds(query, resultHandler);
});
}
public io.reactivex.rxjava3.core.Single uploadByFileName(io.reactivex.rxjava3.core.Flowable stream, java.lang.String fileName) {
io.reactivex.rxjava3.core.Single ret = rxUploadByFileName(stream, fileName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single rxUploadByFileName(io.reactivex.rxjava3.core.Flowable stream, java.lang.String fileName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.uploadByFileName(io.vertx.rxjava3.impl.ReadStreamSubscriber.asReadStream(stream, obj -> obj.getDelegate()).resume(), fileName, resultHandler);
});
}
public io.reactivex.rxjava3.core.Single uploadByFileNameWithOptions(io.reactivex.rxjava3.core.Flowable stream, java.lang.String fileName, io.vertx.ext.mongo.GridFsUploadOptions options) {
io.reactivex.rxjava3.core.Single ret = rxUploadByFileNameWithOptions(stream, fileName, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single rxUploadByFileNameWithOptions(io.reactivex.rxjava3.core.Flowable stream, java.lang.String fileName, io.vertx.ext.mongo.GridFsUploadOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.uploadByFileNameWithOptions(io.vertx.rxjava3.impl.ReadStreamSubscriber.asReadStream(stream, obj -> obj.getDelegate()).resume(), fileName, options, resultHandler);
});
}
/**
* Upload a file to gridfs
* @param fileName the name of the file to store in gridfs
* @return
*/
public io.reactivex.rxjava3.core.Single uploadFile(java.lang.String fileName) {
io.reactivex.rxjava3.core.Single ret = rxUploadFile(fileName);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Upload a file to gridfs
* @param fileName the name of the file to store in gridfs
* @return
*/
public io.reactivex.rxjava3.core.Single rxUploadFile(java.lang.String fileName) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.uploadFile(fileName, resultHandler);
});
}
/**
* Upload a file to gridfs with options
* @param fileName the name of the file to store in gridfs
* @param options {@link io.vertx.ext.mongo.GridFsUploadOptions} for specifying metadata and chunk size
* @return
*/
public io.reactivex.rxjava3.core.Single uploadFileWithOptions(java.lang.String fileName, io.vertx.ext.mongo.GridFsUploadOptions options) {
io.reactivex.rxjava3.core.Single ret = rxUploadFileWithOptions(fileName, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Upload a file to gridfs with options
* @param fileName the name of the file to store in gridfs
* @param options {@link io.vertx.ext.mongo.GridFsUploadOptions} for specifying metadata and chunk size
* @return
*/
public io.reactivex.rxjava3.core.Single rxUploadFileWithOptions(java.lang.String fileName, io.vertx.ext.mongo.GridFsUploadOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.uploadFileWithOptions(fileName, options, resultHandler);
});
}
/**
* Close the client and release its resources
*/
public void close() {
delegate.close();
}
public static MongoGridFsClient newInstance(io.vertx.ext.mongo.MongoGridFsClient arg) {
return arg != null ? new MongoGridFsClient(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy