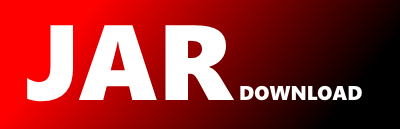
io.vertx.rxjava3.ext.stomp.StompClientConnection Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.stomp;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Once a connection to the STOMP server has been made, client receives a {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}, that let
* send and receive STOMP frames.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.stomp.StompClientConnection original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.stomp.StompClientConnection.class)
public class StompClientConnection {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
StompClientConnection that = (StompClientConnection) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new StompClientConnection((io.vertx.ext.stomp.StompClientConnection) obj),
StompClientConnection::getDelegate
);
private final io.vertx.ext.stomp.StompClientConnection delegate;
public StompClientConnection(io.vertx.ext.stomp.StompClientConnection delegate) {
this.delegate = delegate;
}
public StompClientConnection(Object delegate) {
this.delegate = (io.vertx.ext.stomp.StompClientConnection)delegate;
}
public io.vertx.ext.stomp.StompClientConnection getDelegate() {
return delegate;
}
/**
* @return the session id.
*/
public java.lang.String session() {
java.lang.String ret = delegate.session();
return ret;
}
/**
* @return the STOMP protocol version negotiated with the server.
*/
public java.lang.String version() {
java.lang.String ret = delegate.version();
return ret;
}
/**
* Closes the connection without sending the DISCONNECT
frame.
*/
public void close() {
delegate.close();
}
/**
* @return the server name.
*/
public java.lang.String server() {
java.lang.String ret = delegate.server();
return ret;
}
/**
* Sends a SEND
frame to the server.
* @param headers the headers, must not be null
* @param body the body, may be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single send(java.util.Map headers, io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Single ret = rxSend(headers, body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends a SEND
frame to the server.
* @param headers the headers, must not be null
* @param body the body, may be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxSend(java.util.Map headers, io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.send(headers, body.getDelegate(), receiptHandler);
});
}
/**
* Sends a SEND
frame to the server to the given destination. The message does not have any other header.
* @param destination the destination, must not be null
* @param body the body, may be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single send(java.lang.String destination, io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Single ret = rxSend(destination, body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends a SEND
frame to the server to the given destination. The message does not have any other header.
* @param destination the destination, must not be null
* @param body the body, may be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxSend(java.lang.String destination, io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.send(destination, body.getDelegate(), receiptHandler);
});
}
/**
* Sends the given frame to the server.
* @param frame the frame
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single send(io.vertx.ext.stomp.Frame frame) {
io.reactivex.rxjava3.core.Single ret = rxSend(frame);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends the given frame to the server.
* @param frame the frame
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxSend(io.vertx.ext.stomp.Frame frame) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.send(frame, receiptHandler);
});
}
/**
* Sends a SEND
frame to the server to the given destination.
* @param destination the destination, must not be null
* @param headers the header. The destination
header is replaced by the value given to the destination
parameter
* @param body the body, may be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single send(java.lang.String destination, java.util.Map headers, io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Single ret = rxSend(destination, headers, body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends a SEND
frame to the server to the given destination.
* @param destination the destination, must not be null
* @param headers the header. The destination
header is replaced by the value given to the destination
parameter
* @param body the body, may be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxSend(java.lang.String destination, java.util.Map headers, io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.send(destination, headers, body.getDelegate(), receiptHandler);
});
}
/**
* Subscribes to the given destination. This destination is used as subscription id.
* @param destination the destination, must not be null
* @param handler the handler invoked when a message is received on the given destination. Must not be null
.
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single subscribe(java.lang.String destination, io.vertx.core.Handler handler) {
io.reactivex.rxjava3.core.Single ret = rxSubscribe(destination, handler);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Subscribes to the given destination. This destination is used as subscription id.
* @param destination the destination, must not be null
* @param handler the handler invoked when a message is received on the given destination. Must not be null
.
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxSubscribe(java.lang.String destination, io.vertx.core.Handler handler) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.subscribe(destination, handler, receiptHandler);
});
}
/**
* Subscribes to the given destination.
* @param destination the destination, must not be null
* @param headers the headers to configure the subscription. It may contain the ack
header to configure the acknowledgment policy. If the given set of headers contains the id
header, this value is used as subscription id.
* @param handler the handler invoked when a message is received on the given destination. Must not be null
.
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single subscribe(java.lang.String destination, java.util.Map headers, io.vertx.core.Handler handler) {
io.reactivex.rxjava3.core.Single ret = rxSubscribe(destination, headers, handler);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Subscribes to the given destination.
* @param destination the destination, must not be null
* @param headers the headers to configure the subscription. It may contain the ack
header to configure the acknowledgment policy. If the given set of headers contains the id
header, this value is used as subscription id.
* @param handler the handler invoked when a message is received on the given destination. Must not be null
.
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxSubscribe(java.lang.String destination, java.util.Map headers, io.vertx.core.Handler handler) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.subscribe(destination, headers, handler, receiptHandler);
});
}
/**
* Un-subscribes from the given destination. This method only works if the subscription did not specifies a
* subscription id (using the id
header).
* @param destination the destination
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single unsubscribe(java.lang.String destination) {
io.reactivex.rxjava3.core.Single ret = rxUnsubscribe(destination);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Un-subscribes from the given destination. This method only works if the subscription did not specifies a
* subscription id (using the id
header).
* @param destination the destination
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxUnsubscribe(java.lang.String destination) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.unsubscribe(destination, receiptHandler);
});
}
/**
* Un-subscribes from the given destination. This method computes the subscription id as follows. If the given
* headers contains the id
header, the header value is used. Otherwise the destination is used.
* @param destination the destination
* @param headers the headers
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single unsubscribe(java.lang.String destination, java.util.Map headers) {
io.reactivex.rxjava3.core.Single ret = rxUnsubscribe(destination, headers);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Un-subscribes from the given destination. This method computes the subscription id as follows. If the given
* headers contains the id
header, the header value is used. Otherwise the destination is used.
* @param destination the destination
* @param headers the headers
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxUnsubscribe(java.lang.String destination, java.util.Map headers) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.unsubscribe(destination, headers, receiptHandler);
});
}
/**
* Sets a handler notified when an ERROR
frame is received by the client. The handler receives the ERROR
frame and a reference on the {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}.
* @param handler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection errorHandler(io.vertx.core.Handler handler) {
io.vertx.rxjava3.ext.stomp.StompClientConnection ret = io.vertx.rxjava3.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)delegate.errorHandler(handler));
return ret;
}
/**
* Sets a handler notified when the STOMP connection is closed.
* @param handler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection closeHandler(io.vertx.core.Handler handler) {
delegate.closeHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)event)));
return this;
}
/**
* Sets a handler notified when the server does not respond to a ping
request in time. In other
* words, this handler is invoked when the heartbeat has detected a connection failure with the server.
* The handler can decide to reconnect to the server.
* @param handler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection} receiving the dropped connection.
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection connectionDroppedHandler(io.vertx.core.Handler handler) {
delegate.connectionDroppedHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)event)));
return this;
}
/**
* Sets a handler that let customize the behavior when a ping needs to be sent to the server. Be aware that
* changing the default behavior may break the compliance with the STOMP specification.
* @param handler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection pingHandler(io.vertx.core.Handler handler) {
delegate.pingHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.ext.stomp.StompClientConnection.newInstance((io.vertx.ext.stomp.StompClientConnection)event)));
return this;
}
/**
* Begins a transaction.
* @param id the transaction id, must not be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single beginTX(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxBeginTX(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Begins a transaction.
* @param id the transaction id, must not be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxBeginTX(java.lang.String id) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.beginTX(id, receiptHandler);
});
}
/**
* Begins a transaction.
* @param id the transaction id, must not be null
* @param headers additional headers to send to the server. The transaction
header is replaced by the value passed in the @{code id} parameter
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single beginTX(java.lang.String id, java.util.Map headers) {
io.reactivex.rxjava3.core.Single ret = rxBeginTX(id, headers);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Begins a transaction.
* @param id the transaction id, must not be null
* @param headers additional headers to send to the server. The transaction
header is replaced by the value passed in the @{code id} parameter
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxBeginTX(java.lang.String id, java.util.Map headers) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.beginTX(id, headers, receiptHandler);
});
}
/**
* Commits a transaction.
* @param id the transaction id, must not be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single commit(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxCommit(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Commits a transaction.
* @param id the transaction id, must not be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxCommit(java.lang.String id) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.commit(id, receiptHandler);
});
}
/**
* Commits a transaction.
* @param id the transaction id, must not be null
* @param headers additional headers to send to the server. The transaction
header is replaced by the value passed in the @{code id} parameter
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single commit(java.lang.String id, java.util.Map headers) {
io.reactivex.rxjava3.core.Single ret = rxCommit(id, headers);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Commits a transaction.
* @param id the transaction id, must not be null
* @param headers additional headers to send to the server. The transaction
header is replaced by the value passed in the @{code id} parameter
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxCommit(java.lang.String id, java.util.Map headers) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.commit(id, headers, receiptHandler);
});
}
/**
* Aborts a transaction.
* @param id the transaction id, must not be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single abort(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxAbort(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Aborts a transaction.
* @param id the transaction id, must not be null
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxAbort(java.lang.String id) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.abort(id, receiptHandler);
});
}
/**
* Aborts a transaction.
* @param id the transaction id, must not be null
* @param headers additional headers to send to the server. The transaction
header is replaced by the value passed in the @{code id} parameter
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single abort(java.lang.String id, java.util.Map headers) {
io.reactivex.rxjava3.core.Single ret = rxAbort(id, headers);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Aborts a transaction.
* @param id the transaction id, must not be null
* @param headers additional headers to send to the server. The transaction
header is replaced by the value passed in the @{code id} parameter
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxAbort(java.lang.String id, java.util.Map headers) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.abort(id, headers, receiptHandler);
});
}
/**
* Disconnects the client. Unlike the {@link io.vertx.rxjava3.ext.stomp.StompClientConnection#close} method, this method send the DISCONNECT
frame to the
* server.
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single disconnect() {
io.reactivex.rxjava3.core.Single ret = rxDisconnect();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Disconnects the client. Unlike the {@link io.vertx.rxjava3.ext.stomp.StompClientConnection#close} method, this method send the DISCONNECT
frame to the
* server.
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxDisconnect() {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.disconnect(receiptHandler);
});
}
/**
* Disconnects the client. Unlike the {@link io.vertx.rxjava3.ext.stomp.StompClientConnection#close} method, this method send the DISCONNECT
frame to the
* server. This method lets you customize the DISCONNECT
frame.
* @param frame the DISCONNECT
frame.
* @return a future resolved with the sent frame when the RECEIPT
frame associated with the sent frame has been received
*/
public io.reactivex.rxjava3.core.Single disconnect(io.vertx.ext.stomp.Frame frame) {
io.reactivex.rxjava3.core.Single ret = rxDisconnect(frame);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Disconnects the client. Unlike the {@link io.vertx.rxjava3.ext.stomp.StompClientConnection#close} method, this method send the DISCONNECT
frame to the
* server. This method lets you customize the DISCONNECT
frame.
* @param frame the DISCONNECT
frame.
* @return a future resolved with the sent frame when the RECEIPT
frame associated with the sent frame has been received
*/
public io.reactivex.rxjava3.core.Single rxDisconnect(io.vertx.ext.stomp.Frame frame) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.disconnect(frame, receiptHandler);
});
}
/**
* Sends an acknowledgement for a specific message. It means that the message has been handled and processed by the
* client. The id
parameter is the message id received in the frame.
* @param id the message id of the message to acknowledge
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single ack(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxAck(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends an acknowledgement for a specific message. It means that the message has been handled and processed by the
* client. The id
parameter is the message id received in the frame.
* @param id the message id of the message to acknowledge
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxAck(java.lang.String id) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.ack(id, receiptHandler);
});
}
/**
* Sends a non-acknowledgement for the given message. It means that the message has not been handled by the client.
* The id
parameter is the message id received in the frame.
* @param id the message id of the message to acknowledge
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single nack(java.lang.String id) {
io.reactivex.rxjava3.core.Single ret = rxNack(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends a non-acknowledgement for the given message. It means that the message has not been handled by the client.
* The id
parameter is the message id received in the frame.
* @param id the message id of the message to acknowledge
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxNack(java.lang.String id) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.nack(id, receiptHandler);
});
}
/**
* Sends an acknowledgement for the given frame. It means that the frame has been handled and processed by the
* client. The sent acknowledgement is part of the transaction identified by the given id.
* @param id the message id of the message to acknowledge
* @param txId the transaction id
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single ack(java.lang.String id, java.lang.String txId) {
io.reactivex.rxjava3.core.Single ret = rxAck(id, txId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends an acknowledgement for the given frame. It means that the frame has been handled and processed by the
* client. The sent acknowledgement is part of the transaction identified by the given id.
* @param id the message id of the message to acknowledge
* @param txId the transaction id
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxAck(java.lang.String id, java.lang.String txId) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.ack(id, txId, receiptHandler);
});
}
/**
* Sends a non-acknowledgement for the given frame. It means that the frame has not been handled by the client.
* The sent non-acknowledgement is part of the transaction identified by the given id.
* @param id the message id of the message to acknowledge
* @param txId the transaction id
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single nack(java.lang.String id, java.lang.String txId) {
io.reactivex.rxjava3.core.Single ret = rxNack(id, txId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends a non-acknowledgement for the given frame. It means that the frame has not been handled by the client.
* The sent non-acknowledgement is part of the transaction identified by the given id.
* @param id the message id of the message to acknowledge
* @param txId the transaction id
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.reactivex.rxjava3.core.Single rxNack(java.lang.String id, java.lang.String txId) {
return AsyncResultSingle.toSingle( receiptHandler -> {
delegate.nack(id, txId, receiptHandler);
});
}
/**
* Configures a received handler that get notified when a STOMP frame is received by the client.
* This handler can be used for logging, debugging or ad-hoc behavior. The frame can still be modified by the handler.
*
* Unlike {@link io.vertx.rxjava3.ext.stomp.StompClient#receivedFrameHandler}, the given handler won't receive the CONNECTED
frame. If a received frame handler is set on the {@link io.vertx.rxjava3.ext.stomp.StompClient}, it will be used by all
* clients connection, so calling this method is useless, except if you want to use a different handler.
* @param handler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection receivedFrameHandler(io.vertx.core.Handler handler) {
delegate.receivedFrameHandler(handler);
return this;
}
/**
* Configures a handler notified when a frame is going to be written on the wire. This handler can be used from
* logging, debugging. The handler can modify the received frame.
*
* If a writing frame handler is set on the {@link io.vertx.rxjava3.ext.stomp.StompClient}, it will be used by all
* clients connection, so calling this method is useless, except if you want to use a different handler.
* @param handler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection writingFrameHandler(io.vertx.core.Handler handler) {
delegate.writingFrameHandler(handler);
return this;
}
/**
* Configures the exception handler notified upon TCP-level errors.
* @param exceptionHandler the handler
* @return the current {@link io.vertx.rxjava3.ext.stomp.StompClientConnection}
*/
public io.vertx.rxjava3.ext.stomp.StompClientConnection exceptionHandler(io.vertx.core.Handler exceptionHandler) {
delegate.exceptionHandler(exceptionHandler);
return this;
}
/**
* Returns whether or not the `CONNECTED` frame has been receive meaning that the Stomp connection is established.
* @return true
if the connection is established, false
otherwise
*/
public boolean isConnected() {
boolean ret = delegate.isConnected();
return ret;
}
public static StompClientConnection newInstance(io.vertx.ext.stomp.StompClientConnection arg) {
return arg != null ? new StompClientConnection(arg) : null;
}
}