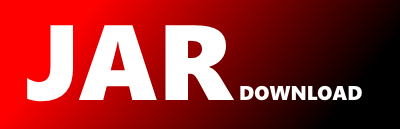
io.vertx.rxjava3.ext.unit.TestContext Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.unit;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* The test context is used for performing test assertions and manage the completion of the test. This context
* is provided by vertx-unit as argument of the test case.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.unit.TestContext original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.unit.TestContext.class)
public class TestContext {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
TestContext that = (TestContext) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new TestContext((io.vertx.ext.unit.TestContext) obj),
TestContext::getDelegate
);
private final io.vertx.ext.unit.TestContext delegate;
public TestContext(io.vertx.ext.unit.TestContext delegate) {
this.delegate = delegate;
}
public TestContext(Object delegate) {
this.delegate = (io.vertx.ext.unit.TestContext)delegate;
}
public io.vertx.ext.unit.TestContext getDelegate() {
return delegate;
}
/**
* Get some data from the context.
* @param key the key of the data
* @return the data
*/
public T get(java.lang.String key) {
T ret = (T) delegate.get(key);
return ret;
}
/**
* Put some data in the context.
*
* This can be used to share data between different tests and before/after phases.
* @param key the key of the data
* @param value the data
* @return the previous object when it exists
*/
public T put(java.lang.String key, java.lang.Object value) {
T ret = (T) delegate.put(key, value);
return ret;
}
/**
* Remove some data from the context.
* @param key the key to remove
* @return the removed object when it exists
*/
public T remove(java.lang.String key) {
T ret = (T) delegate.remove(key);
return ret;
}
/**
* Assert the expected
argument is null
. If the argument is not, an assertion error is thrown
* otherwise the execution continue.
* @param expected the argument being asserted to be null
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertNull(java.lang.Object expected) {
delegate.assertNull(expected);
return this;
}
/**
* Assert the expected
argument is null
. If the argument is not, an assertion error is thrown
* otherwise the execution continue.
* @param expected the argument being asserted to be null
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertNull(java.lang.Object expected, java.lang.String message) {
delegate.assertNull(expected, message);
return this;
}
/**
* Assert the expected
argument is not null
. If the argument is null
, an assertion error is thrown
* otherwise the execution continue.
* @param expected the argument being asserted to be not null
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertNotNull(java.lang.Object expected) {
delegate.assertNotNull(expected);
return this;
}
/**
* Assert the expected
argument is not null
. If the argument is null
, an assertion error is thrown
* otherwise the execution continue.
* @param expected the argument being asserted to be not null
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertNotNull(java.lang.Object expected, java.lang.String message) {
delegate.assertNotNull(expected, message);
return this;
}
/**
* Assert the specified condition
is true
. If the condition is false
, an assertion error is thrown
* otherwise the execution continue.
* @param condition the condition to assert
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertTrue(boolean condition) {
delegate.assertTrue(condition);
return this;
}
/**
* Assert the specified condition
is true
. If the condition is false
, an assertion error is thrown
* otherwise the execution continue.
* @param condition the condition to assert
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertTrue(boolean condition, java.lang.String message) {
delegate.assertTrue(condition, message);
return this;
}
/**
* Assert the specified condition
is false
. If the condition is true
, an assertion error is thrown
* otherwise the execution continue.
* @param condition the condition to assert
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertFalse(boolean condition) {
delegate.assertFalse(condition);
return this;
}
/**
* Assert the specified condition
is false
. If the condition is true
, an assertion error is thrown
* otherwise the execution continue.
* @param condition the condition to assert
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertFalse(boolean condition, java.lang.String message) {
delegate.assertFalse(condition, message);
return this;
}
/**
* Assert the expected
argument is equals to the actual
argument. If the arguments are not equals
* an assertion error is thrown otherwise the execution continue.
* @param expected the object the actual object is supposedly equals to
* @param actual the actual object to test
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertEquals(java.lang.Object expected, java.lang.Object actual) {
delegate.assertEquals(expected, actual);
return this;
}
/**
* Assert the expected
argument is equals to the actual
argument. If the arguments are not equals
* an assertion error is thrown otherwise the execution continue.
* @param expected the object the actual object is supposedly equals to
* @param actual the actual object to test
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertEquals(java.lang.Object expected, java.lang.Object actual, java.lang.String message) {
delegate.assertEquals(expected, actual, message);
return this;
}
/**
* Asserts that the expected
double argument is equals to the actual
double argument
* within a positive delta. If the arguments do not satisfy this, an assertion error is thrown otherwise
* the execution continue.
* @param expected the object the actual object is supposedly equals to
* @param actual the actual object to test
* @param delta the maximum delta
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertInRange(double expected, double actual, double delta) {
delegate.assertInRange(expected, actual, delta);
return this;
}
/**
* Asserts that the expected
double argument is equals to the actual
double argument
* within a positive delta. If the arguments do not satisfy this, an assertion error is thrown otherwise
* the execution continue.
* @param expected the object the actual object is supposedly equals to
* @param actual the actual object to test
* @param delta the maximum delta
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertInRange(double expected, double actual, double delta, java.lang.String message) {
delegate.assertInRange(expected, actual, delta, message);
return this;
}
/**
* Assert the first
argument is not equals to the second
argument. If the arguments are equals
* an assertion error is thrown otherwise the execution continue.
* @param first the first object to test
* @param second the second object to test
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertNotEquals(java.lang.Object first, java.lang.Object second) {
delegate.assertNotEquals(first, second);
return this;
}
/**
* Assert the first
argument is not equals to the second
argument. If the arguments are equals
* an assertion error is thrown otherwise the execution continue.
* @param first the first object to test
* @param second the second object to test
* @param message the failure message
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext assertNotEquals(java.lang.Object first, java.lang.Object second, java.lang.String message) {
delegate.assertNotEquals(first, second, message);
return this;
}
/**
* Execute the provided handler, which may contain assertions, possibly from any third-party assertion framework.
* Any {@link java.lang.AssertionError} thrown will be caught (and propagated) in order to fulfill potential expected async
* completeness.
* @param block block of code to be executed
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.unit.TestContext verify(io.vertx.core.Handler block) {
delegate.verify(block);
return this;
}
/**
* Throw a failure.
*/
public void fail() {
delegate.fail();
}
/**
* Throw a failure with the specified failure message
.
* @param message the failure message
*/
public void fail(java.lang.String message) {
delegate.fail(message);
}
/**
* Throw a failure with the specified failure cause
.
* @param cause the failure cause
*/
public void fail(java.lang.Throwable cause) {
delegate.fail(cause);
}
/**
* Create and returns a new async object, the returned async controls the completion of the test. Calling the
* {@link io.vertx.rxjava3.ext.unit.Async#complete} completes the async operation.
*
* The test case will complete when all the async objects have their {@link io.vertx.rxjava3.ext.unit.Async#complete}
* method called at least once.
*
* This method shall be used for creating asynchronous exit points for the executed test.
* @return the async instance
*/
public io.vertx.rxjava3.ext.unit.Async async() {
io.vertx.rxjava3.ext.unit.Async ret = io.vertx.rxjava3.ext.unit.Async.newInstance((io.vertx.ext.unit.Async)delegate.async());
return ret;
}
/**
* Create and returns a new async object, the returned async controls the completion of the test. This async operation
* completes when the {@link io.vertx.rxjava3.ext.unit.Async#countDown} is called count
times.
*
* The test case will complete when all the async objects have their {@link io.vertx.rxjava3.ext.unit.Async#complete}
* method called at least once.
*
* This method shall be used for creating asynchronous exit points for the executed test.
* @param count
* @return the async instance
*/
public io.vertx.rxjava3.ext.unit.Async async(int count) {
io.vertx.rxjava3.ext.unit.Async ret = io.vertx.rxjava3.ext.unit.Async.newInstance((io.vertx.ext.unit.Async)delegate.async(count));
return ret;
}
/**
* Create and returns a new async object, the returned async controls the completion of the test.
* This async operation completes when the {@link io.vertx.rxjava3.ext.unit.Async#countDown} is called count
times.
* If {@link io.vertx.rxjava3.ext.unit.Async#countDown} is called more than count
times, an {@link java.lang.IllegalStateException} is thrown.
*
* The test case will complete when all the async objects have their {@link io.vertx.rxjava3.ext.unit.Async#complete}
* method called at least once.
*
* This method shall be used for creating asynchronous exit points for the executed test.
* @param count
* @return the async instance
*/
public io.vertx.rxjava3.ext.unit.Async strictAsync(int count) {
io.vertx.rxjava3.ext.unit.Async ret = io.vertx.rxjava3.ext.unit.Async.newInstance((io.vertx.ext.unit.Async)delegate.strictAsync(count));
return ret;
}
/**
* Creates and returns a new async handler, the returned handler controls the completion of the test.
*
* When the returned handler is called back with a succeeded result it completes the async operation.
*
* When the returned handler is called back with a failed result it fails the test with the cause of the failure.
* @return the async result handler
*/
public io.vertx.core.Handler> asyncAssertSuccess() {
io.vertx.core.Handler> ret = delegate.asyncAssertSuccess();
return ret;
}
/**
* Creates and returns a new async handler, the returned handler controls the completion of the test.
*
* When the returned handler is called back with a succeeded result it invokes the resultHandler
argument
* with the async result. The test completes after the result handler is invoked and does not fails.
*
* When the returned handler is called back with a failed result it fails the test with the cause of the failure.
*
* Note that the result handler can create other async objects during its invocation that would postpone
* the completion of the test case until those objects are resolved.
* @param resultHandler the result handler
* @return the async result handler
*/
public io.vertx.core.Handler> asyncAssertSuccess(io.vertx.core.Handler resultHandler) {
io.vertx.core.Handler> ret = delegate.asyncAssertSuccess(resultHandler);
return ret;
}
/**
* Creates and returns a new async handler, the returned handler controls the completion of the test.
*
* When the returned handler is called back with a failed result it completes the async operation.
*
* When the returned handler is called back with a succeeded result it fails the test.
* @return the async result handler
*/
public io.vertx.core.Handler> asyncAssertFailure() {
io.vertx.core.Handler> ret = delegate.asyncAssertFailure();
return ret;
}
/**
* Creates and returns a new async handler, the returned handler controls the completion of the test.
*
* When the returned handler is called back with a failed result it completes the async operation.
*
* When the returned handler is called back with a succeeded result it fails the test.
* @param causeHandler the cause handler
* @return the async result handler
*/
public io.vertx.core.Handler> asyncAssertFailure(io.vertx.core.Handler causeHandler) {
io.vertx.core.Handler> ret = delegate.asyncAssertFailure(causeHandler);
return ret;
}
/**
* @return an exception handler that will fail this context
*/
public io.vertx.core.Handler exceptionHandler() {
io.vertx.core.Handler ret = delegate.exceptionHandler();
return ret;
}
public static TestContext newInstance(io.vertx.ext.unit.TestContext arg) {
return arg != null ? new TestContext(arg) : null;
}
}